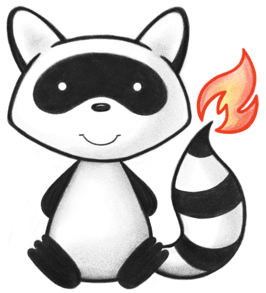
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum Program { 037 038 /** 039 * null 040 */ 041 _1, 042 /** 043 * null 044 */ 045 _2, 046 /** 047 * null 048 */ 049 _3, 050 /** 051 * null 052 */ 053 _4, 054 /** 055 * null 056 */ 057 _5, 058 /** 059 * null 060 */ 061 _6, 062 /** 063 * null 064 */ 065 _7, 066 /** 067 * null 068 */ 069 _8, 070 /** 071 * null 072 */ 073 _9, 074 /** 075 * null 076 */ 077 _10, 078 /** 079 * null 080 */ 081 _11, 082 /** 083 * null 084 */ 085 _12, 086 /** 087 * null 088 */ 089 _13, 090 /** 091 * null 092 */ 093 _14, 094 /** 095 * null 096 */ 097 _15, 098 /** 099 * null 100 */ 101 _16, 102 /** 103 * null 104 */ 105 _17, 106 /** 107 * null 108 */ 109 _18, 110 /** 111 * null 112 */ 113 _19, 114 /** 115 * null 116 */ 117 _20, 118 /** 119 * null 120 */ 121 _21, 122 /** 123 * null 124 */ 125 _22, 126 /** 127 * null 128 */ 129 _23, 130 /** 131 * null 132 */ 133 _24, 134 /** 135 * null 136 */ 137 _25, 138 /** 139 * null 140 */ 141 _26, 142 /** 143 * null 144 */ 145 _27, 146 /** 147 * null 148 */ 149 _28, 150 /** 151 * null 152 */ 153 _29, 154 /** 155 * null 156 */ 157 _30, 158 /** 159 * null 160 */ 161 _31, 162 /** 163 * null 164 */ 165 _32, 166 /** 167 * null 168 */ 169 _33, 170 /** 171 * null 172 */ 173 _34, 174 /** 175 * null 176 */ 177 _35, 178 /** 179 * null 180 */ 181 _36, 182 /** 183 * null 184 */ 185 _37, 186 /** 187 * null 188 */ 189 _38, 190 /** 191 * null 192 */ 193 _39, 194 /** 195 * null 196 */ 197 _40, 198 /** 199 * null 200 */ 201 _41, 202 /** 203 * null 204 */ 205 _42, 206 /** 207 * null 208 */ 209 _43, 210 /** 211 * null 212 */ 213 _44, 214 /** 215 * null 216 */ 217 _45, 218 /** 219 * added to help the parsers 220 */ 221 NULL; 222 223 public static Program fromCode(String codeString) throws FHIRException { 224 if (codeString == null || "".equals(codeString)) 225 return null; 226 if ("1".equals(codeString)) 227 return _1; 228 if ("2".equals(codeString)) 229 return _2; 230 if ("3".equals(codeString)) 231 return _3; 232 if ("4".equals(codeString)) 233 return _4; 234 if ("5".equals(codeString)) 235 return _5; 236 if ("6".equals(codeString)) 237 return _6; 238 if ("7".equals(codeString)) 239 return _7; 240 if ("8".equals(codeString)) 241 return _8; 242 if ("9".equals(codeString)) 243 return _9; 244 if ("10".equals(codeString)) 245 return _10; 246 if ("11".equals(codeString)) 247 return _11; 248 if ("12".equals(codeString)) 249 return _12; 250 if ("13".equals(codeString)) 251 return _13; 252 if ("14".equals(codeString)) 253 return _14; 254 if ("15".equals(codeString)) 255 return _15; 256 if ("16".equals(codeString)) 257 return _16; 258 if ("17".equals(codeString)) 259 return _17; 260 if ("18".equals(codeString)) 261 return _18; 262 if ("19".equals(codeString)) 263 return _19; 264 if ("20".equals(codeString)) 265 return _20; 266 if ("21".equals(codeString)) 267 return _21; 268 if ("22".equals(codeString)) 269 return _22; 270 if ("23".equals(codeString)) 271 return _23; 272 if ("24".equals(codeString)) 273 return _24; 274 if ("25".equals(codeString)) 275 return _25; 276 if ("26".equals(codeString)) 277 return _26; 278 if ("27".equals(codeString)) 279 return _27; 280 if ("28".equals(codeString)) 281 return _28; 282 if ("29".equals(codeString)) 283 return _29; 284 if ("30".equals(codeString)) 285 return _30; 286 if ("31".equals(codeString)) 287 return _31; 288 if ("32".equals(codeString)) 289 return _32; 290 if ("33".equals(codeString)) 291 return _33; 292 if ("34".equals(codeString)) 293 return _34; 294 if ("35".equals(codeString)) 295 return _35; 296 if ("36".equals(codeString)) 297 return _36; 298 if ("37".equals(codeString)) 299 return _37; 300 if ("38".equals(codeString)) 301 return _38; 302 if ("39".equals(codeString)) 303 return _39; 304 if ("40".equals(codeString)) 305 return _40; 306 if ("41".equals(codeString)) 307 return _41; 308 if ("42".equals(codeString)) 309 return _42; 310 if ("43".equals(codeString)) 311 return _43; 312 if ("44".equals(codeString)) 313 return _44; 314 if ("45".equals(codeString)) 315 return _45; 316 throw new FHIRException("Unknown Program code '" + codeString + "'"); 317 } 318 319 public String toCode() { 320 switch (this) { 321 case _1: 322 return "1"; 323 case _2: 324 return "2"; 325 case _3: 326 return "3"; 327 case _4: 328 return "4"; 329 case _5: 330 return "5"; 331 case _6: 332 return "6"; 333 case _7: 334 return "7"; 335 case _8: 336 return "8"; 337 case _9: 338 return "9"; 339 case _10: 340 return "10"; 341 case _11: 342 return "11"; 343 case _12: 344 return "12"; 345 case _13: 346 return "13"; 347 case _14: 348 return "14"; 349 case _15: 350 return "15"; 351 case _16: 352 return "16"; 353 case _17: 354 return "17"; 355 case _18: 356 return "18"; 357 case _19: 358 return "19"; 359 case _20: 360 return "20"; 361 case _21: 362 return "21"; 363 case _22: 364 return "22"; 365 case _23: 366 return "23"; 367 case _24: 368 return "24"; 369 case _25: 370 return "25"; 371 case _26: 372 return "26"; 373 case _27: 374 return "27"; 375 case _28: 376 return "28"; 377 case _29: 378 return "29"; 379 case _30: 380 return "30"; 381 case _31: 382 return "31"; 383 case _32: 384 return "32"; 385 case _33: 386 return "33"; 387 case _34: 388 return "34"; 389 case _35: 390 return "35"; 391 case _36: 392 return "36"; 393 case _37: 394 return "37"; 395 case _38: 396 return "38"; 397 case _39: 398 return "39"; 399 case _40: 400 return "40"; 401 case _41: 402 return "41"; 403 case _42: 404 return "42"; 405 case _43: 406 return "43"; 407 case _44: 408 return "44"; 409 case _45: 410 return "45"; 411 case NULL: 412 return null; 413 default: 414 return "?"; 415 } 416 } 417 418 public String getSystem() { 419 return "http://terminology.hl7.org/CodeSystem/program"; 420 } 421 422 public String getDefinition() { 423 switch (this) { 424 case _1: 425 return ""; 426 case _2: 427 return ""; 428 case _3: 429 return ""; 430 case _4: 431 return ""; 432 case _5: 433 return ""; 434 case _6: 435 return ""; 436 case _7: 437 return ""; 438 case _8: 439 return ""; 440 case _9: 441 return ""; 442 case _10: 443 return ""; 444 case _11: 445 return ""; 446 case _12: 447 return ""; 448 case _13: 449 return ""; 450 case _14: 451 return ""; 452 case _15: 453 return ""; 454 case _16: 455 return ""; 456 case _17: 457 return ""; 458 case _18: 459 return ""; 460 case _19: 461 return ""; 462 case _20: 463 return ""; 464 case _21: 465 return ""; 466 case _22: 467 return ""; 468 case _23: 469 return ""; 470 case _24: 471 return ""; 472 case _25: 473 return ""; 474 case _26: 475 return ""; 476 case _27: 477 return ""; 478 case _28: 479 return ""; 480 case _29: 481 return ""; 482 case _30: 483 return ""; 484 case _31: 485 return ""; 486 case _32: 487 return ""; 488 case _33: 489 return ""; 490 case _34: 491 return ""; 492 case _35: 493 return ""; 494 case _36: 495 return ""; 496 case _37: 497 return ""; 498 case _38: 499 return ""; 500 case _39: 501 return ""; 502 case _40: 503 return ""; 504 case _41: 505 return ""; 506 case _42: 507 return ""; 508 case _43: 509 return ""; 510 case _44: 511 return ""; 512 case _45: 513 return ""; 514 case NULL: 515 return null; 516 default: 517 return "?"; 518 } 519 } 520 521 public String getDisplay() { 522 switch (this) { 523 case _1: 524 return "Acquired Brain Injury (ABI) Program "; 525 case _2: 526 return "ABI Slow To Recover (ABI STR) Program"; 527 case _3: 528 return "Access Programs"; 529 case _4: 530 return "Adult and Further Education (ACFE) Program"; 531 case _5: 532 return "Adult Day Activity and Support Services (ADASS) Program"; 533 case _6: 534 return "Adult Day Care Program"; 535 case _7: 536 return "ATSS (Adult Training Support Service)"; 537 case _8: 538 return "Community Aged Care Packages (CACP)"; 539 case _9: 540 return "Care Coordination & Supplementary Services (CCSS)"; 541 case _10: 542 return "Cognitive Dementia Memory Service (CDAMS)"; 543 case _11: 544 return "ChildFIRST"; 545 case _12: 546 return "Children's Contact Services"; 547 case _13: 548 return "Community Visitors Scheme"; 549 case _14: 550 return "CPP (Community Partners Program)"; 551 case _15: 552 return "Closing the Gap (CTG)"; 553 case _16: 554 return "Coordinated Veterans' Care (CVC) Program"; 555 case _17: 556 return "Day Program"; 557 case _18: 558 return "Drop In Program"; 559 case _19: 560 return "Early Years Program"; 561 case _20: 562 return "Employee Assistance Program"; 563 case _21: 564 return "Home And Community Care (HACC)"; 565 case _22: 566 return "Hospital Admission Risk Program (HARP)"; 567 case _23: 568 return "Hospital in the Home (HITH) Program"; 569 case _24: 570 return "ICTP (Intensive Community Treatment Program)"; 571 case _25: 572 return "IFSS (Intensive Family Support Program)"; 573 case _26: 574 return "JPET (Job Placement, Education and Training)"; 575 case _27: 576 return "Koori Juvenile Justice Program"; 577 case _28: 578 return "Language Literacy and Numeracy Program"; 579 case _29: 580 return "Life Skills Program"; 581 case _30: 582 return "LMP (Lifestyle Modification Program)"; 583 case _31: 584 return "MedsCheck Program"; 585 case _32: 586 return "Methadone/Buprenorphine Program"; 587 case _33: 588 return "National Disabilities Insurance Scheme (NDIS)"; 589 case _34: 590 return "National Diabetes Services Scheme (NDSS)"; 591 case _35: 592 return "Needle/Syringe Program"; 593 case _36: 594 return "nPEP Program"; 595 case _37: 596 return "Personal Support Program"; 597 case _38: 598 return "Partners in Recovery (PIR) Program"; 599 case _39: 600 return "Pre-employment Program"; 601 case _40: 602 return "Reconnect Program"; 603 case _41: 604 return "Sexual Abuse Counselling and Prevention Program (SACPP)"; 605 case _42: 606 return "Social Support Programs"; 607 case _43: 608 return "Supported Residential Service (SRS)"; 609 case _44: 610 return "Tasmanian Aboriginal Centre (TAC)"; 611 case _45: 612 return "Victim's Assistance Program"; 613 case NULL: 614 return null; 615 default: 616 return "?"; 617 } 618 } 619 620}