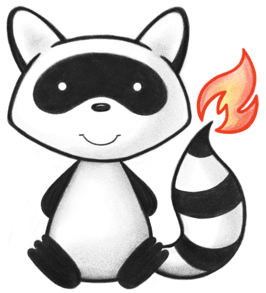
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum QuestionnaireEnableOperator { 037 038 /** 039 * True if whether an answer exists is equal to the enableWhen answer (which 040 * must be a boolean). 041 */ 042 EXISTS, 043 /** 044 * True if whether at least one answer has a value that is equal to the 045 * enableWhen answer. 046 */ 047 EQUAL, 048 /** 049 * True if whether at least no answer has a value that is equal to the 050 * enableWhen answer. 051 */ 052 NOT_EQUAL, 053 /** 054 * True if whether at least no answer has a value that is greater than the 055 * enableWhen answer. 056 */ 057 GREATER_THAN, 058 /** 059 * True if whether at least no answer has a value that is less than the 060 * enableWhen answer. 061 */ 062 LESS_THAN, 063 /** 064 * True if whether at least no answer has a value that is greater or equal to 065 * the enableWhen answer. 066 */ 067 GREATER_OR_EQUAL, 068 /** 069 * True if whether at least no answer has a value that is less or equal to the 070 * enableWhen answer. 071 */ 072 LESS_OR_EQUAL, 073 /** 074 * added to help the parsers 075 */ 076 NULL; 077 078 public static QuestionnaireEnableOperator fromCode(String codeString) throws FHIRException { 079 if (codeString == null || "".equals(codeString)) 080 return null; 081 if ("exists".equals(codeString)) 082 return EXISTS; 083 if ("=".equals(codeString)) 084 return EQUAL; 085 if ("!=".equals(codeString)) 086 return NOT_EQUAL; 087 if (">".equals(codeString)) 088 return GREATER_THAN; 089 if ("<".equals(codeString)) 090 return LESS_THAN; 091 if (">=".equals(codeString)) 092 return GREATER_OR_EQUAL; 093 if ("<=".equals(codeString)) 094 return LESS_OR_EQUAL; 095 throw new FHIRException("Unknown QuestionnaireEnableOperator code '" + codeString + "'"); 096 } 097 098 public String toCode() { 099 switch (this) { 100 case EXISTS: 101 return "exists"; 102 case EQUAL: 103 return "="; 104 case NOT_EQUAL: 105 return "!="; 106 case GREATER_THAN: 107 return ">"; 108 case LESS_THAN: 109 return "<"; 110 case GREATER_OR_EQUAL: 111 return ">="; 112 case LESS_OR_EQUAL: 113 return "<="; 114 case NULL: 115 return null; 116 default: 117 return "?"; 118 } 119 } 120 121 public String getSystem() { 122 return "http://hl7.org/fhir/questionnaire-enable-operator"; 123 } 124 125 public String getDefinition() { 126 switch (this) { 127 case EXISTS: 128 return "True if whether an answer exists is equal to the enableWhen answer (which must be a boolean)."; 129 case EQUAL: 130 return "True if whether at least one answer has a value that is equal to the enableWhen answer."; 131 case NOT_EQUAL: 132 return "True if whether at least no answer has a value that is equal to the enableWhen answer."; 133 case GREATER_THAN: 134 return "True if whether at least no answer has a value that is greater than the enableWhen answer."; 135 case LESS_THAN: 136 return "True if whether at least no answer has a value that is less than the enableWhen answer."; 137 case GREATER_OR_EQUAL: 138 return "True if whether at least no answer has a value that is greater or equal to the enableWhen answer."; 139 case LESS_OR_EQUAL: 140 return "True if whether at least no answer has a value that is less or equal to the enableWhen answer."; 141 case NULL: 142 return null; 143 default: 144 return "?"; 145 } 146 } 147 148 public String getDisplay() { 149 switch (this) { 150 case EXISTS: 151 return "Exists"; 152 case EQUAL: 153 return "Equals"; 154 case NOT_EQUAL: 155 return "Not Equals"; 156 case GREATER_THAN: 157 return "Greater Than"; 158 case LESS_THAN: 159 return "Less Than"; 160 case GREATER_OR_EQUAL: 161 return "Greater or Equals"; 162 case LESS_OR_EQUAL: 163 return "Less or Equals"; 164 case NULL: 165 return null; 166 default: 167 return "?"; 168 } 169 } 170 171}