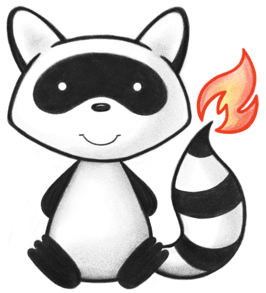
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum ResearchStudyStatus { 037 038 /** 039 * Study is opened for accrual. 040 */ 041 ACTIVE, 042 /** 043 * Study is completed prematurely and will not resume; patients are no longer 044 * examined nor treated. 045 */ 046 ADMINISTRATIVELYCOMPLETED, 047 /** 048 * Protocol is approved by the review board. 049 */ 050 APPROVED, 051 /** 052 * Study is closed for accrual; patients can be examined and treated. 053 */ 054 CLOSEDTOACCRUAL, 055 /** 056 * Study is closed to accrual and intervention, i.e. the study is closed to 057 * enrollment, all study subjects have completed treatment or intervention but 058 * are still being followed according to the primary objective of the study. 059 */ 060 CLOSEDTOACCRUALANDINTERVENTION, 061 /** 062 * Study is closed to accrual and intervention, i.e. the study is closed to 063 * enrollment, all study subjects have completed treatment or intervention but 064 * are still being followed according to the primary objective of the study. 065 */ 066 COMPLETED, 067 /** 068 * Protocol was disapproved by the review board. 069 */ 070 DISAPPROVED, 071 /** 072 * Protocol is submitted to the review board for approval. 073 */ 074 INREVIEW, 075 /** 076 * Study is temporarily closed for accrual; can be potentially resumed in the 077 * future; patients can be examined and treated. 078 */ 079 TEMPORARILYCLOSEDTOACCRUAL, 080 /** 081 * Study is temporarily closed for accrual and intervention and potentially can 082 * be resumed in the future. 083 */ 084 TEMPORARILYCLOSEDTOACCRUALANDINTERVENTION, 085 /** 086 * Protocol was withdrawn by the lead organization. 087 */ 088 WITHDRAWN, 089 /** 090 * added to help the parsers 091 */ 092 NULL; 093 094 public static ResearchStudyStatus fromCode(String codeString) throws FHIRException { 095 if (codeString == null || "".equals(codeString)) 096 return null; 097 if ("active".equals(codeString)) 098 return ACTIVE; 099 if ("administratively-completed".equals(codeString)) 100 return ADMINISTRATIVELYCOMPLETED; 101 if ("approved".equals(codeString)) 102 return APPROVED; 103 if ("closed-to-accrual".equals(codeString)) 104 return CLOSEDTOACCRUAL; 105 if ("closed-to-accrual-and-intervention".equals(codeString)) 106 return CLOSEDTOACCRUALANDINTERVENTION; 107 if ("completed".equals(codeString)) 108 return COMPLETED; 109 if ("disapproved".equals(codeString)) 110 return DISAPPROVED; 111 if ("in-review".equals(codeString)) 112 return INREVIEW; 113 if ("temporarily-closed-to-accrual".equals(codeString)) 114 return TEMPORARILYCLOSEDTOACCRUAL; 115 if ("temporarily-closed-to-accrual-and-intervention".equals(codeString)) 116 return TEMPORARILYCLOSEDTOACCRUALANDINTERVENTION; 117 if ("withdrawn".equals(codeString)) 118 return WITHDRAWN; 119 throw new FHIRException("Unknown ResearchStudyStatus code '" + codeString + "'"); 120 } 121 122 public String toCode() { 123 switch (this) { 124 case ACTIVE: 125 return "active"; 126 case ADMINISTRATIVELYCOMPLETED: 127 return "administratively-completed"; 128 case APPROVED: 129 return "approved"; 130 case CLOSEDTOACCRUAL: 131 return "closed-to-accrual"; 132 case CLOSEDTOACCRUALANDINTERVENTION: 133 return "closed-to-accrual-and-intervention"; 134 case COMPLETED: 135 return "completed"; 136 case DISAPPROVED: 137 return "disapproved"; 138 case INREVIEW: 139 return "in-review"; 140 case TEMPORARILYCLOSEDTOACCRUAL: 141 return "temporarily-closed-to-accrual"; 142 case TEMPORARILYCLOSEDTOACCRUALANDINTERVENTION: 143 return "temporarily-closed-to-accrual-and-intervention"; 144 case WITHDRAWN: 145 return "withdrawn"; 146 case NULL: 147 return null; 148 default: 149 return "?"; 150 } 151 } 152 153 public String getSystem() { 154 return "http://hl7.org/fhir/research-study-status"; 155 } 156 157 public String getDefinition() { 158 switch (this) { 159 case ACTIVE: 160 return "Study is opened for accrual."; 161 case ADMINISTRATIVELYCOMPLETED: 162 return "Study is completed prematurely and will not resume; patients are no longer examined nor treated."; 163 case APPROVED: 164 return "Protocol is approved by the review board."; 165 case CLOSEDTOACCRUAL: 166 return "Study is closed for accrual; patients can be examined and treated."; 167 case CLOSEDTOACCRUALANDINTERVENTION: 168 return "Study is closed to accrual and intervention, i.e. the study is closed to enrollment, all study subjects have completed treatment or intervention but are still being followed according to the primary objective of the study."; 169 case COMPLETED: 170 return "Study is closed to accrual and intervention, i.e. the study is closed to enrollment, all study subjects have completed treatment\nor intervention but are still being followed according to the primary objective of the study."; 171 case DISAPPROVED: 172 return "Protocol was disapproved by the review board."; 173 case INREVIEW: 174 return "Protocol is submitted to the review board for approval."; 175 case TEMPORARILYCLOSEDTOACCRUAL: 176 return "Study is temporarily closed for accrual; can be potentially resumed in the future; patients can be examined and treated."; 177 case TEMPORARILYCLOSEDTOACCRUALANDINTERVENTION: 178 return "Study is temporarily closed for accrual and intervention and potentially can be resumed in the future."; 179 case WITHDRAWN: 180 return "Protocol was withdrawn by the lead organization."; 181 case NULL: 182 return null; 183 default: 184 return "?"; 185 } 186 } 187 188 public String getDisplay() { 189 switch (this) { 190 case ACTIVE: 191 return "Active"; 192 case ADMINISTRATIVELYCOMPLETED: 193 return "Administratively Completed"; 194 case APPROVED: 195 return "Approved"; 196 case CLOSEDTOACCRUAL: 197 return "Closed to Accrual"; 198 case CLOSEDTOACCRUALANDINTERVENTION: 199 return "Closed to Accrual and Intervention"; 200 case COMPLETED: 201 return "Completed"; 202 case DISAPPROVED: 203 return "Disapproved"; 204 case INREVIEW: 205 return "In Review"; 206 case TEMPORARILYCLOSEDTOACCRUAL: 207 return "Temporarily Closed to Accrual"; 208 case TEMPORARILYCLOSEDTOACCRUALANDINTERVENTION: 209 return "Temporarily Closed to Accrual and Intervention"; 210 case WITHDRAWN: 211 return "Withdrawn"; 212 case NULL: 213 return null; 214 default: 215 return "?"; 216 } 217 } 218 219}