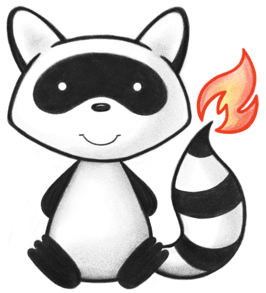
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum ResearchSubjectStatus { 037 038 /** 039 * An identified person that can be considered for inclusion in a study. 040 */ 041 CANDIDATE, 042 /** 043 * A person that has met the eligibility criteria for inclusion in a study. 044 */ 045 ELIGIBLE, 046 /** 047 * A person is no longer receiving study intervention and/or being evaluated 048 * with tests and procedures according to the protocol, but they are being 049 * monitored on a protocol-prescribed schedule. 050 */ 051 FOLLOWUP, 052 /** 053 * A person who did not meet one or more criteria required for participation in 054 * a study is considered to have failed screening or is ineligible for the 055 * study. 056 */ 057 INELIGIBLE, 058 /** 059 * A person for whom registration was not completed. 060 */ 061 NOTREGISTERED, 062 /** 063 * A person that has ended their participation on a study either because their 064 * treatment/observation is complete or through not responding, withdrawal, 065 * non-compliance and/or adverse event. 066 */ 067 OFFSTUDY, 068 /** 069 * A person that is enrolled or registered on a study. 070 */ 071 ONSTUDY, 072 /** 073 * The person is receiving the treatment or participating in an activity (e.g. 074 * yoga, diet, etc.) that the study is evaluating. 075 */ 076 ONSTUDYINTERVENTION, 077 /** 078 * The subject is being evaluated via tests and assessments according to the 079 * study calendar, but is not receiving any intervention. Note that this state 080 * is study-dependent and might not exist in all studies. A synonym for this is 081 * "short-term follow-up". 082 */ 083 ONSTUDYOBSERVATION, 084 /** 085 * A person is pre-registered for a study. 086 */ 087 PENDINGONSTUDY, 088 /** 089 * A person that is potentially eligible for participation in the study. 090 */ 091 POTENTIALCANDIDATE, 092 /** 093 * A person who is being evaluated for eligibility for a study. 094 */ 095 SCREENING, 096 /** 097 * The person has withdrawn their participation in the study before 098 * registration. 099 */ 100 WITHDRAWN, 101 /** 102 * added to help the parsers 103 */ 104 NULL; 105 106 public static ResearchSubjectStatus fromCode(String codeString) throws FHIRException { 107 if (codeString == null || "".equals(codeString)) 108 return null; 109 if ("candidate".equals(codeString)) 110 return CANDIDATE; 111 if ("eligible".equals(codeString)) 112 return ELIGIBLE; 113 if ("follow-up".equals(codeString)) 114 return FOLLOWUP; 115 if ("ineligible".equals(codeString)) 116 return INELIGIBLE; 117 if ("not-registered".equals(codeString)) 118 return NOTREGISTERED; 119 if ("off-study".equals(codeString)) 120 return OFFSTUDY; 121 if ("on-study".equals(codeString)) 122 return ONSTUDY; 123 if ("on-study-intervention".equals(codeString)) 124 return ONSTUDYINTERVENTION; 125 if ("on-study-observation".equals(codeString)) 126 return ONSTUDYOBSERVATION; 127 if ("pending-on-study".equals(codeString)) 128 return PENDINGONSTUDY; 129 if ("potential-candidate".equals(codeString)) 130 return POTENTIALCANDIDATE; 131 if ("screening".equals(codeString)) 132 return SCREENING; 133 if ("withdrawn".equals(codeString)) 134 return WITHDRAWN; 135 throw new FHIRException("Unknown ResearchSubjectStatus code '" + codeString + "'"); 136 } 137 138 public String toCode() { 139 switch (this) { 140 case CANDIDATE: 141 return "candidate"; 142 case ELIGIBLE: 143 return "eligible"; 144 case FOLLOWUP: 145 return "follow-up"; 146 case INELIGIBLE: 147 return "ineligible"; 148 case NOTREGISTERED: 149 return "not-registered"; 150 case OFFSTUDY: 151 return "off-study"; 152 case ONSTUDY: 153 return "on-study"; 154 case ONSTUDYINTERVENTION: 155 return "on-study-intervention"; 156 case ONSTUDYOBSERVATION: 157 return "on-study-observation"; 158 case PENDINGONSTUDY: 159 return "pending-on-study"; 160 case POTENTIALCANDIDATE: 161 return "potential-candidate"; 162 case SCREENING: 163 return "screening"; 164 case WITHDRAWN: 165 return "withdrawn"; 166 case NULL: 167 return null; 168 default: 169 return "?"; 170 } 171 } 172 173 public String getSystem() { 174 return "http://hl7.org/fhir/research-subject-status"; 175 } 176 177 public String getDefinition() { 178 switch (this) { 179 case CANDIDATE: 180 return "An identified person that can be considered for inclusion in a study."; 181 case ELIGIBLE: 182 return "A person that has met the eligibility criteria for inclusion in a study."; 183 case FOLLOWUP: 184 return "A person is no longer receiving study intervention and/or being evaluated with tests and procedures according to the protocol, but they are being monitored on a protocol-prescribed schedule."; 185 case INELIGIBLE: 186 return "A person who did not meet one or more criteria required for participation in a study is considered to have failed screening or\nis ineligible for the study."; 187 case NOTREGISTERED: 188 return "A person for whom registration was not completed."; 189 case OFFSTUDY: 190 return "A person that has ended their participation on a study either because their treatment/observation is complete or through not\nresponding, withdrawal, non-compliance and/or adverse event."; 191 case ONSTUDY: 192 return "A person that is enrolled or registered on a study."; 193 case ONSTUDYINTERVENTION: 194 return "The person is receiving the treatment or participating in an activity (e.g. yoga, diet, etc.) that the study is evaluating."; 195 case ONSTUDYOBSERVATION: 196 return "The subject is being evaluated via tests and assessments according to the study calendar, but is not receiving any intervention. Note that this state is study-dependent and might not exist in all studies. A synonym for this is \"short-term follow-up\"."; 197 case PENDINGONSTUDY: 198 return "A person is pre-registered for a study."; 199 case POTENTIALCANDIDATE: 200 return "A person that is potentially eligible for participation in the study."; 201 case SCREENING: 202 return "A person who is being evaluated for eligibility for a study."; 203 case WITHDRAWN: 204 return "The person has withdrawn their participation in the study before registration."; 205 case NULL: 206 return null; 207 default: 208 return "?"; 209 } 210 } 211 212 public String getDisplay() { 213 switch (this) { 214 case CANDIDATE: 215 return "Candidate"; 216 case ELIGIBLE: 217 return "Eligible"; 218 case FOLLOWUP: 219 return "Follow-up"; 220 case INELIGIBLE: 221 return "Ineligible"; 222 case NOTREGISTERED: 223 return "Not Registered"; 224 case OFFSTUDY: 225 return "Off-study"; 226 case ONSTUDY: 227 return "On-study"; 228 case ONSTUDYINTERVENTION: 229 return "On-study-intervention"; 230 case ONSTUDYOBSERVATION: 231 return "On-study-observation"; 232 case PENDINGONSTUDY: 233 return "Pending on-study"; 234 case POTENTIALCANDIDATE: 235 return "Potential Candidate"; 236 case SCREENING: 237 return "Screening"; 238 case WITHDRAWN: 239 return "Withdrawn"; 240 case NULL: 241 return null; 242 default: 243 return "?"; 244 } 245 } 246 247}