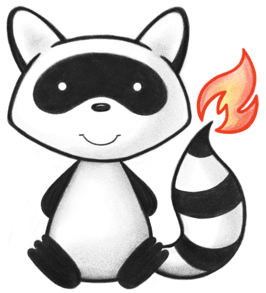
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum RestfulInteraction { 037 038 /** 039 * Read the current state of the resource. 040 */ 041 READ, 042 /** 043 * Read the state of a specific version of the resource. 044 */ 045 VREAD, 046 /** 047 * Update an existing resource by its id (or create it if it is new). 048 */ 049 UPDATE, 050 /** 051 * Update an existing resource by posting a set of changes to it. 052 */ 053 PATCH, 054 /** 055 * Delete a resource. 056 */ 057 DELETE, 058 /** 059 * Retrieve the change history for a particular resource, type of resource, or 060 * the entire system. 061 */ 062 HISTORY, 063 /** 064 * Retrieve the change history for a particular resource. 065 */ 066 HISTORYINSTANCE, 067 /** 068 * Retrieve the change history for all resources of a particular type. 069 */ 070 HISTORYTYPE, 071 /** 072 * Retrieve the change history for all resources on a system. 073 */ 074 HISTORYSYSTEM, 075 /** 076 * Create a new resource with a server assigned id. 077 */ 078 CREATE, 079 /** 080 * Search a resource type or all resources based on some filter criteria. 081 */ 082 SEARCH, 083 /** 084 * Search all resources of the specified type based on some filter criteria. 085 */ 086 SEARCHTYPE, 087 /** 088 * Search all resources based on some filter criteria. 089 */ 090 SEARCHSYSTEM, 091 /** 092 * Get a Capability Statement for the system. 093 */ 094 CAPABILITIES, 095 /** 096 * Update, create or delete a set of resources as a single transaction. 097 */ 098 TRANSACTION, 099 /** 100 * perform a set of a separate interactions in a single http operation 101 */ 102 BATCH, 103 /** 104 * Perform an operation as defined by an OperationDefinition. 105 */ 106 OPERATION, 107 /** 108 * added to help the parsers 109 */ 110 NULL; 111 112 public static RestfulInteraction fromCode(String codeString) throws FHIRException { 113 if (codeString == null || "".equals(codeString)) 114 return null; 115 if ("read".equals(codeString)) 116 return READ; 117 if ("vread".equals(codeString)) 118 return VREAD; 119 if ("update".equals(codeString)) 120 return UPDATE; 121 if ("patch".equals(codeString)) 122 return PATCH; 123 if ("delete".equals(codeString)) 124 return DELETE; 125 if ("history".equals(codeString)) 126 return HISTORY; 127 if ("history-instance".equals(codeString)) 128 return HISTORYINSTANCE; 129 if ("history-type".equals(codeString)) 130 return HISTORYTYPE; 131 if ("history-system".equals(codeString)) 132 return HISTORYSYSTEM; 133 if ("create".equals(codeString)) 134 return CREATE; 135 if ("search".equals(codeString)) 136 return SEARCH; 137 if ("search-type".equals(codeString)) 138 return SEARCHTYPE; 139 if ("search-system".equals(codeString)) 140 return SEARCHSYSTEM; 141 if ("capabilities".equals(codeString)) 142 return CAPABILITIES; 143 if ("transaction".equals(codeString)) 144 return TRANSACTION; 145 if ("batch".equals(codeString)) 146 return BATCH; 147 if ("operation".equals(codeString)) 148 return OPERATION; 149 throw new FHIRException("Unknown RestfulInteraction code '" + codeString + "'"); 150 } 151 152 public String toCode() { 153 switch (this) { 154 case READ: 155 return "read"; 156 case VREAD: 157 return "vread"; 158 case UPDATE: 159 return "update"; 160 case PATCH: 161 return "patch"; 162 case DELETE: 163 return "delete"; 164 case HISTORY: 165 return "history"; 166 case HISTORYINSTANCE: 167 return "history-instance"; 168 case HISTORYTYPE: 169 return "history-type"; 170 case HISTORYSYSTEM: 171 return "history-system"; 172 case CREATE: 173 return "create"; 174 case SEARCH: 175 return "search"; 176 case SEARCHTYPE: 177 return "search-type"; 178 case SEARCHSYSTEM: 179 return "search-system"; 180 case CAPABILITIES: 181 return "capabilities"; 182 case TRANSACTION: 183 return "transaction"; 184 case BATCH: 185 return "batch"; 186 case OPERATION: 187 return "operation"; 188 case NULL: 189 return null; 190 default: 191 return "?"; 192 } 193 } 194 195 public String getSystem() { 196 return "http://hl7.org/fhir/restful-interaction"; 197 } 198 199 public String getDefinition() { 200 switch (this) { 201 case READ: 202 return "Read the current state of the resource."; 203 case VREAD: 204 return "Read the state of a specific version of the resource."; 205 case UPDATE: 206 return "Update an existing resource by its id (or create it if it is new)."; 207 case PATCH: 208 return "Update an existing resource by posting a set of changes to it."; 209 case DELETE: 210 return "Delete a resource."; 211 case HISTORY: 212 return "Retrieve the change history for a particular resource, type of resource, or the entire system."; 213 case HISTORYINSTANCE: 214 return "Retrieve the change history for a particular resource."; 215 case HISTORYTYPE: 216 return "Retrieve the change history for all resources of a particular type."; 217 case HISTORYSYSTEM: 218 return "Retrieve the change history for all resources on a system."; 219 case CREATE: 220 return "Create a new resource with a server assigned id."; 221 case SEARCH: 222 return "Search a resource type or all resources based on some filter criteria."; 223 case SEARCHTYPE: 224 return "Search all resources of the specified type based on some filter criteria."; 225 case SEARCHSYSTEM: 226 return "Search all resources based on some filter criteria."; 227 case CAPABILITIES: 228 return "Get a Capability Statement for the system."; 229 case TRANSACTION: 230 return "Update, create or delete a set of resources as a single transaction."; 231 case BATCH: 232 return "perform a set of a separate interactions in a single http operation"; 233 case OPERATION: 234 return "Perform an operation as defined by an OperationDefinition."; 235 case NULL: 236 return null; 237 default: 238 return "?"; 239 } 240 } 241 242 public String getDisplay() { 243 switch (this) { 244 case READ: 245 return "read"; 246 case VREAD: 247 return "vread"; 248 case UPDATE: 249 return "update"; 250 case PATCH: 251 return "patch"; 252 case DELETE: 253 return "delete"; 254 case HISTORY: 255 return "history"; 256 case HISTORYINSTANCE: 257 return "history-instance"; 258 case HISTORYTYPE: 259 return "history-type"; 260 case HISTORYSYSTEM: 261 return "history-system"; 262 case CREATE: 263 return "create"; 264 case SEARCH: 265 return "search"; 266 case SEARCHTYPE: 267 return "search-type"; 268 case SEARCHSYSTEM: 269 return "search-system"; 270 case CAPABILITIES: 271 return "capabilities"; 272 case TRANSACTION: 273 return "transaction"; 274 case BATCH: 275 return "batch"; 276 case OPERATION: 277 return "operation"; 278 case NULL: 279 return null; 280 default: 281 return "?"; 282 } 283 } 284 285}