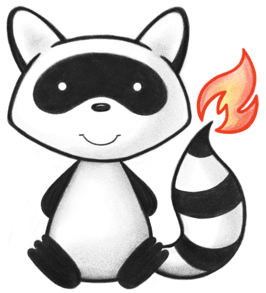
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum SearchComparator { 037 038 /** 039 * the value for the parameter in the resource is equal to the provided value. 040 */ 041 EQ, 042 /** 043 * the value for the parameter in the resource is not equal to the provided 044 * value. 045 */ 046 NE, 047 /** 048 * the value for the parameter in the resource is greater than the provided 049 * value. 050 */ 051 GT, 052 /** 053 * the value for the parameter in the resource is less than the provided value. 054 */ 055 LT, 056 /** 057 * the value for the parameter in the resource is greater or equal to the 058 * provided value. 059 */ 060 GE, 061 /** 062 * the value for the parameter in the resource is less or equal to the provided 063 * value. 064 */ 065 LE, 066 /** 067 * the value for the parameter in the resource starts after the provided value. 068 */ 069 SA, 070 /** 071 * the value for the parameter in the resource ends before the provided value. 072 */ 073 EB, 074 /** 075 * the value for the parameter in the resource is approximately the same to the 076 * provided value. 077 */ 078 AP, 079 /** 080 * added to help the parsers 081 */ 082 NULL; 083 084 public static SearchComparator fromCode(String codeString) throws FHIRException { 085 if (codeString == null || "".equals(codeString)) 086 return null; 087 if ("eq".equals(codeString)) 088 return EQ; 089 if ("ne".equals(codeString)) 090 return NE; 091 if ("gt".equals(codeString)) 092 return GT; 093 if ("lt".equals(codeString)) 094 return LT; 095 if ("ge".equals(codeString)) 096 return GE; 097 if ("le".equals(codeString)) 098 return LE; 099 if ("sa".equals(codeString)) 100 return SA; 101 if ("eb".equals(codeString)) 102 return EB; 103 if ("ap".equals(codeString)) 104 return AP; 105 throw new FHIRException("Unknown SearchComparator code '" + codeString + "'"); 106 } 107 108 public String toCode() { 109 switch (this) { 110 case EQ: 111 return "eq"; 112 case NE: 113 return "ne"; 114 case GT: 115 return "gt"; 116 case LT: 117 return "lt"; 118 case GE: 119 return "ge"; 120 case LE: 121 return "le"; 122 case SA: 123 return "sa"; 124 case EB: 125 return "eb"; 126 case AP: 127 return "ap"; 128 case NULL: 129 return null; 130 default: 131 return "?"; 132 } 133 } 134 135 public String getSystem() { 136 return "http://hl7.org/fhir/search-comparator"; 137 } 138 139 public String getDefinition() { 140 switch (this) { 141 case EQ: 142 return "the value for the parameter in the resource is equal to the provided value."; 143 case NE: 144 return "the value for the parameter in the resource is not equal to the provided value."; 145 case GT: 146 return "the value for the parameter in the resource is greater than the provided value."; 147 case LT: 148 return "the value for the parameter in the resource is less than the provided value."; 149 case GE: 150 return "the value for the parameter in the resource is greater or equal to the provided value."; 151 case LE: 152 return "the value for the parameter in the resource is less or equal to the provided value."; 153 case SA: 154 return "the value for the parameter in the resource starts after the provided value."; 155 case EB: 156 return "the value for the parameter in the resource ends before the provided value."; 157 case AP: 158 return "the value for the parameter in the resource is approximately the same to the provided value."; 159 case NULL: 160 return null; 161 default: 162 return "?"; 163 } 164 } 165 166 public String getDisplay() { 167 switch (this) { 168 case EQ: 169 return "Equals"; 170 case NE: 171 return "Not Equals"; 172 case GT: 173 return "Greater Than"; 174 case LT: 175 return "Less Than"; 176 case GE: 177 return "Greater or Equals"; 178 case LE: 179 return "Less of Equal"; 180 case SA: 181 return "Starts After"; 182 case EB: 183 return "Ends Before"; 184 case AP: 185 return "Approximately"; 186 case NULL: 187 return null; 188 default: 189 return "?"; 190 } 191 } 192 193}