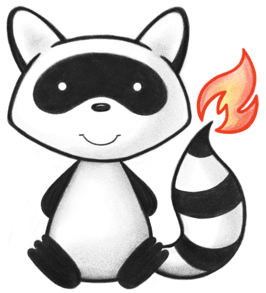
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum SearchModifierCode { 037 038 /** 039 * The search parameter returns resources that have a value or not. 040 */ 041 MISSING, 042 /** 043 * The search parameter returns resources that have a value that exactly matches 044 * the supplied parameter (the whole string, including casing and accents). 045 */ 046 EXACT, 047 /** 048 * The search parameter returns resources that include the supplied parameter 049 * value anywhere within the field being searched. 050 */ 051 CONTAINS, 052 /** 053 * The search parameter returns resources that do not contain a match. 054 */ 055 NOT, 056 /** 057 * The search parameter is processed as a string that searches text associated 058 * with the code/value - either CodeableConcept.text, Coding.display, or 059 * Identifier.type.text. 060 */ 061 TEXT, 062 /** 063 * The search parameter is a URI (relative or absolute) that identifies a value 064 * set, and the search parameter tests whether the coding is in the specified 065 * value set. 066 */ 067 IN, 068 /** 069 * The search parameter is a URI (relative or absolute) that identifies a value 070 * set, and the search parameter tests whether the coding is not in the 071 * specified value set. 072 */ 073 NOTIN, 074 /** 075 * The search parameter tests whether the value in a resource is subsumed by the 076 * specified value (is-a, or hierarchical relationships). 077 */ 078 BELOW, 079 /** 080 * The search parameter tests whether the value in a resource subsumes the 081 * specified value (is-a, or hierarchical relationships). 082 */ 083 ABOVE, 084 /** 085 * The search parameter only applies to the Resource Type specified as a 086 * modifier (e.g. the modifier is not actually :type, but :Patient etc.). 087 */ 088 TYPE, 089 /** 090 * The search parameter applies to the identifier on the resource, not the 091 * reference. 092 */ 093 IDENTIFIER, 094 /** 095 * The search parameter has the format system|code|value, where the system and 096 * code refer to an Identifier.type.coding.system and .code, and match if any of 097 * the type codes match. All 3 parts must be present. 098 */ 099 OFTYPE, 100 /** 101 * added to help the parsers 102 */ 103 NULL; 104 105 public static SearchModifierCode fromCode(String codeString) throws FHIRException { 106 if (codeString == null || "".equals(codeString)) 107 return null; 108 if ("missing".equals(codeString)) 109 return MISSING; 110 if ("exact".equals(codeString)) 111 return EXACT; 112 if ("contains".equals(codeString)) 113 return CONTAINS; 114 if ("not".equals(codeString)) 115 return NOT; 116 if ("text".equals(codeString)) 117 return TEXT; 118 if ("in".equals(codeString)) 119 return IN; 120 if ("not-in".equals(codeString)) 121 return NOTIN; 122 if ("below".equals(codeString)) 123 return BELOW; 124 if ("above".equals(codeString)) 125 return ABOVE; 126 if ("type".equals(codeString)) 127 return TYPE; 128 if ("identifier".equals(codeString)) 129 return IDENTIFIER; 130 if ("ofType".equals(codeString)) 131 return OFTYPE; 132 throw new FHIRException("Unknown SearchModifierCode code '" + codeString + "'"); 133 } 134 135 public String toCode() { 136 switch (this) { 137 case MISSING: 138 return "missing"; 139 case EXACT: 140 return "exact"; 141 case CONTAINS: 142 return "contains"; 143 case NOT: 144 return "not"; 145 case TEXT: 146 return "text"; 147 case IN: 148 return "in"; 149 case NOTIN: 150 return "not-in"; 151 case BELOW: 152 return "below"; 153 case ABOVE: 154 return "above"; 155 case TYPE: 156 return "type"; 157 case IDENTIFIER: 158 return "identifier"; 159 case OFTYPE: 160 return "ofType"; 161 case NULL: 162 return null; 163 default: 164 return "?"; 165 } 166 } 167 168 public String getSystem() { 169 return "http://hl7.org/fhir/search-modifier-code"; 170 } 171 172 public String getDefinition() { 173 switch (this) { 174 case MISSING: 175 return "The search parameter returns resources that have a value or not."; 176 case EXACT: 177 return "The search parameter returns resources that have a value that exactly matches the supplied parameter (the whole string, including casing and accents)."; 178 case CONTAINS: 179 return "The search parameter returns resources that include the supplied parameter value anywhere within the field being searched."; 180 case NOT: 181 return "The search parameter returns resources that do not contain a match."; 182 case TEXT: 183 return "The search parameter is processed as a string that searches text associated with the code/value - either CodeableConcept.text, Coding.display, or Identifier.type.text."; 184 case IN: 185 return "The search parameter is a URI (relative or absolute) that identifies a value set, and the search parameter tests whether the coding is in the specified value set."; 186 case NOTIN: 187 return "The search parameter is a URI (relative or absolute) that identifies a value set, and the search parameter tests whether the coding is not in the specified value set."; 188 case BELOW: 189 return "The search parameter tests whether the value in a resource is subsumed by the specified value (is-a, or hierarchical relationships)."; 190 case ABOVE: 191 return "The search parameter tests whether the value in a resource subsumes the specified value (is-a, or hierarchical relationships)."; 192 case TYPE: 193 return "The search parameter only applies to the Resource Type specified as a modifier (e.g. the modifier is not actually :type, but :Patient etc.)."; 194 case IDENTIFIER: 195 return "The search parameter applies to the identifier on the resource, not the reference."; 196 case OFTYPE: 197 return "The search parameter has the format system|code|value, where the system and code refer to an Identifier.type.coding.system and .code, and match if any of the type codes match. All 3 parts must be present."; 198 case NULL: 199 return null; 200 default: 201 return "?"; 202 } 203 } 204 205 public String getDisplay() { 206 switch (this) { 207 case MISSING: 208 return "Missing"; 209 case EXACT: 210 return "Exact"; 211 case CONTAINS: 212 return "Contains"; 213 case NOT: 214 return "Not"; 215 case TEXT: 216 return "Text"; 217 case IN: 218 return "In"; 219 case NOTIN: 220 return "Not In"; 221 case BELOW: 222 return "Below"; 223 case ABOVE: 224 return "Above"; 225 case TYPE: 226 return "Type"; 227 case IDENTIFIER: 228 return "Identifier"; 229 case OFTYPE: 230 return "Of Type"; 231 case NULL: 232 return null; 233 default: 234 return "?"; 235 } 236 } 237 238}