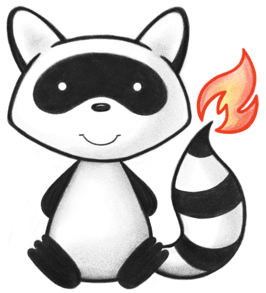
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum ServicePlace { 037 038 /** 039 * A facility or location where drugs and other medically related items and 040 * services are sold, dispensed, or otherwise provided directly to patients. 041 */ 042 _01, 043 /** 044 * A facility whose primary purpose is education. 045 */ 046 _03, 047 /** 048 * A facility or location whose primary purpose is to provide temporary housing 049 * to homeless individuals (e.g., emergency shelters, individual or family 050 * shelters). 051 */ 052 _04, 053 /** 054 * A facility or location, owned and operated by the Indian Health Service, 055 * which provides diagnostic, therapeutic (surgical and nonsurgical), and 056 * rehabilitation services to American Indians and Alaska Natives who do not 057 * require hospitalization. 058 */ 059 _05, 060 /** 061 * A facility or location, owned and operated by the Indian Health Service, 062 * which provides diagnostic, therapeutic (surgical and nonsurgical), and 063 * rehabilitation services rendered by, or under the supervision of, physicians 064 * to American Indians and Alaska Natives admitted as inpatients or outpatients. 065 */ 066 _06, 067 /** 068 * A facility or location owned and operated by a federally recognized American 069 * Indian or Alaska Native tribe or tribal organization under a 638 agreement, 070 * which provides diagnostic, therapeutic (surgical and nonsurgical), and 071 * rehabilitation services to tribal members who do not require hospitalization. 072 */ 073 _07, 074 /** 075 * A facility or location owned and operated by a federally recognized American 076 * Indian or Alaska Native tribe or tribal organization under a 638 agreement, 077 * which provides diagnostic, therapeutic (surgical and nonsurgical), and 078 * rehabilitation services to tribal members admitted as inpatients or 079 * outpatients. 080 */ 081 _08, 082 /** 083 * A prison, jail, reformatory, work farm, detention center, or any other 084 * similar facility maintained by either Federal, State or local authorities for 085 * the purpose of confinement or rehabilitation of adult or juvenile criminal 086 * offenders. 087 */ 088 _09, 089 /** 090 * Location, other than a hospital, skilled nursing facility (SNF), military 091 * treatment facility, community health center, State or local public health 092 * clinic, or intermediate care facility (ICF), where the health professional 093 * routinely provides health examinations, diagnosis, and treatment of illness 094 * or injury on an ambulatory basis. 095 */ 096 _11, 097 /** 098 * Location, other than a hospital or other facility, where the patient receives 099 * care in a private residence. 100 */ 101 _12, 102 /** 103 * Congregate residential facility with self-contained living units providing 104 * assessment of each resident's needs and on-site support 24 hours a day, 7 105 * days a week, with the capacity to deliver or arrange for services including 106 * some health care and other services. 107 */ 108 _13, 109 /** 110 * A residence, with shared living areas, where clients receive supervision and 111 * other services such as social and/or behavioral services, custodial service, 112 * and minimal services (e.g., medication administration). 113 */ 114 _14, 115 /** 116 * A facility/unit that moves from place-to-place equipped to provide 117 * preventive, screening, diagnostic, and/or treatment services. 118 */ 119 _15, 120 /** 121 * portion of an off-campus hospital provider-based department which provides 122 * diagnostic, therapeutic (both surgical and nonsurgical), and rehabilitation 123 * services to sick or injured persons who do not require hospitalization or 124 * institutionalization. 125 */ 126 _19, 127 /** 128 * Location, distinct from a hospital emergency room, an office, or a clinic, 129 * whose purpose is to diagnose and treat illness or injury for unscheduled, 130 * ambulatory patients seeking immediate medical attention. 131 */ 132 _20, 133 /** 134 * A facility, other than psychiatric, which primarily provides diagnostic, 135 * therapeutic (both surgical and nonsurgical), and rehabilitation services by, 136 * or under, the supervision of physicians to patients admitted for a variety of 137 * medical conditions. 138 */ 139 _21, 140 /** 141 * A land vehicle specifically designed, equipped and staffed for lifesaving and 142 * transporting the sick or injured. 143 */ 144 _41, 145 /** 146 * added to help the parsers 147 */ 148 NULL; 149 150 public static ServicePlace fromCode(String codeString) throws FHIRException { 151 if (codeString == null || "".equals(codeString)) 152 return null; 153 if ("01".equals(codeString)) 154 return _01; 155 if ("03".equals(codeString)) 156 return _03; 157 if ("04".equals(codeString)) 158 return _04; 159 if ("05".equals(codeString)) 160 return _05; 161 if ("06".equals(codeString)) 162 return _06; 163 if ("07".equals(codeString)) 164 return _07; 165 if ("08".equals(codeString)) 166 return _08; 167 if ("09".equals(codeString)) 168 return _09; 169 if ("11".equals(codeString)) 170 return _11; 171 if ("12".equals(codeString)) 172 return _12; 173 if ("13".equals(codeString)) 174 return _13; 175 if ("14".equals(codeString)) 176 return _14; 177 if ("15".equals(codeString)) 178 return _15; 179 if ("19".equals(codeString)) 180 return _19; 181 if ("20".equals(codeString)) 182 return _20; 183 if ("21".equals(codeString)) 184 return _21; 185 if ("41".equals(codeString)) 186 return _41; 187 throw new FHIRException("Unknown ServicePlace code '" + codeString + "'"); 188 } 189 190 public String toCode() { 191 switch (this) { 192 case _01: 193 return "01"; 194 case _03: 195 return "03"; 196 case _04: 197 return "04"; 198 case _05: 199 return "05"; 200 case _06: 201 return "06"; 202 case _07: 203 return "07"; 204 case _08: 205 return "08"; 206 case _09: 207 return "09"; 208 case _11: 209 return "11"; 210 case _12: 211 return "12"; 212 case _13: 213 return "13"; 214 case _14: 215 return "14"; 216 case _15: 217 return "15"; 218 case _19: 219 return "19"; 220 case _20: 221 return "20"; 222 case _21: 223 return "21"; 224 case _41: 225 return "41"; 226 case NULL: 227 return null; 228 default: 229 return "?"; 230 } 231 } 232 233 public String getSystem() { 234 return "http://terminology.hl7.org/CodeSystem/ex-serviceplace"; 235 } 236 237 public String getDefinition() { 238 switch (this) { 239 case _01: 240 return "A facility or location where drugs and other medically related items and services are sold, dispensed, or otherwise provided directly to patients."; 241 case _03: 242 return "A facility whose primary purpose is education."; 243 case _04: 244 return "A facility or location whose primary purpose is to provide temporary housing to homeless individuals (e.g., emergency shelters, individual or family shelters)."; 245 case _05: 246 return "A facility or location, owned and operated by the Indian Health Service, which provides diagnostic, therapeutic (surgical and nonsurgical), and rehabilitation services to American Indians and Alaska Natives who do not require hospitalization."; 247 case _06: 248 return "A facility or location, owned and operated by the Indian Health Service, which provides diagnostic, therapeutic (surgical and nonsurgical), and rehabilitation services rendered by, or under the supervision of, physicians to American Indians and Alaska Natives admitted as inpatients or outpatients."; 249 case _07: 250 return "A facility or location owned and operated by a federally recognized American Indian or Alaska Native tribe or tribal organization under a 638 agreement, which provides diagnostic, therapeutic (surgical and nonsurgical), and rehabilitation services to tribal members who do not require hospitalization."; 251 case _08: 252 return "A facility or location owned and operated by a federally recognized American Indian or Alaska Native tribe or tribal organization under a 638 agreement, which provides diagnostic, therapeutic (surgical and nonsurgical), and rehabilitation services to tribal members admitted as inpatients or outpatients."; 253 case _09: 254 return "A prison, jail, reformatory, work farm, detention center, or any other similar facility maintained by either Federal, State or local authorities for the purpose of confinement or rehabilitation of adult or juvenile criminal offenders."; 255 case _11: 256 return "Location, other than a hospital, skilled nursing facility (SNF), military treatment facility, community health center, State or local public health clinic, or intermediate care facility (ICF), where the health professional routinely provides health examinations, diagnosis, and treatment of illness or injury on an ambulatory basis."; 257 case _12: 258 return "Location, other than a hospital or other facility, where the patient receives care in a private residence."; 259 case _13: 260 return "Congregate residential facility with self-contained living units providing assessment of each resident's needs and on-site support 24 hours a day, 7 days a week, with the capacity to deliver or arrange for services including some health care and other services."; 261 case _14: 262 return "A residence, with shared living areas, where clients receive supervision and other services such as social and/or behavioral services, custodial service, and minimal services (e.g., medication administration)."; 263 case _15: 264 return "A facility/unit that moves from place-to-place equipped to provide preventive, screening, diagnostic, and/or treatment services."; 265 case _19: 266 return "portion of an off-campus hospital provider-based department which provides diagnostic, therapeutic (both surgical and nonsurgical), and rehabilitation services to sick or injured persons who do not require hospitalization or institutionalization."; 267 case _20: 268 return "Location, distinct from a hospital emergency room, an office, or a clinic, whose purpose is to diagnose and treat illness or injury for unscheduled, ambulatory patients seeking immediate medical attention."; 269 case _21: 270 return "A facility, other than psychiatric, which primarily provides diagnostic, therapeutic (both surgical and nonsurgical), and rehabilitation services by, or under, the supervision of physicians to patients admitted for a variety of medical conditions."; 271 case _41: 272 return "A land vehicle specifically designed, equipped and staffed for lifesaving and transporting the sick or injured."; 273 case NULL: 274 return null; 275 default: 276 return "?"; 277 } 278 } 279 280 public String getDisplay() { 281 switch (this) { 282 case _01: 283 return "Pharmacy"; 284 case _03: 285 return "School"; 286 case _04: 287 return "Homeless Shelter"; 288 case _05: 289 return "Indian Health Service Free-standing Facility"; 290 case _06: 291 return "Indian Health Service Provider-based Facility"; 292 case _07: 293 return "Tribal 638 Free-Standing Facility"; 294 case _08: 295 return "Tribal 638 Provider-Based Facility"; 296 case _09: 297 return "Prison/Correctional Facility"; 298 case _11: 299 return "Office"; 300 case _12: 301 return "Home"; 302 case _13: 303 return "Assisted Living Fa"; 304 case _14: 305 return "Group Home"; 306 case _15: 307 return "Mobile Unit"; 308 case _19: 309 return "Off Campus-Outpatient Hospital"; 310 case _20: 311 return "Urgent Care Facility"; 312 case _21: 313 return "Inpatient Hospital"; 314 case _41: 315 return "Ambulance?Land"; 316 case NULL: 317 return null; 318 default: 319 return "?"; 320 } 321 } 322 323}