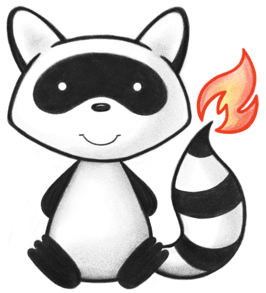
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum StandardsStatus { 037 038 /** 039 * This portion of the specification is not considered to be complete enough or 040 * sufficiently reviewed to be safe for implementation. It may have known issues 041 * or still be in the "in development" stage. It is included in the publication 042 * as a place-holder, to solicit feedback from the implementation community 043 * and/or to give implementers some insight as to functionality likely to be 044 * included in future versions of the specification. Content at this level 045 * should only be implemented by the brave or desperate and is very much "use at 046 * your own risk". The content that is Draft that will usually be elevated to 047 * Trial Use once review and correction is complete after it has been subjected 048 * to ballot. 049 */ 050 DRAFT, 051 /** 052 * This content has been subject to review and production implementation in a 053 * wide variety of environments. The content is considered to be stable and has 054 * been 'locked', subjecting it to FHIR Inter-version Compatibility Rules. While 055 * changes are possible, they are expected to be infrequent and are tightly 056 * constrained. Compatibility Rules. 057 */ 058 NORMATIVE, 059 /** 060 * This content has been well reviewed and is considered by the authors to be 061 * ready for use in production systems. It has been subjected to ballot and 062 * approved as an official standard. However, it has not yet seen widespread use 063 * in production across the full spectrum of environments it is intended to be 064 * used in. In some cases, there may be documented known issues that require 065 * implementation experience to determine appropriate resolutions for. 066 * 067 * Future versions of FHIR may make significant changes to Trial Use content 068 * that are not compatible with previously published content. 069 */ 070 TRIALUSE, 071 /** 072 * This portion of the specification is provided for implementer assistance, and 073 * does not make rules that implementers are required to follow. Typical 074 * examples of this content in the FHIR specification are tables of contents, 075 * registries, examples, and implementer advice. 076 */ 077 INFORMATIVE, 078 /** 079 * This portion of the specification is provided for implementer assistance, and 080 * does not make rules that implementers are required to follow. Typical 081 * examples of this content in the FHIR specification are tables of contents, 082 * registries, examples, and implementer advice. 083 */ 084 DEPRECATED, 085 /** 086 * This is content that is managed outside the FHIR Specification, but included 087 * for implementer convenience. 088 */ 089 EXTERNAL, 090 /** 091 * added to help the parsers 092 */ 093 NULL; 094 095 public static StandardsStatus fromCode(String codeString) throws FHIRException { 096 if (codeString == null || "".equals(codeString)) 097 return null; 098 if ("draft".equals(codeString)) 099 return DRAFT; 100 if ("normative".equals(codeString)) 101 return NORMATIVE; 102 if ("trial-use".equals(codeString)) 103 return TRIALUSE; 104 if ("informative".equals(codeString)) 105 return INFORMATIVE; 106 if ("deprecated".equals(codeString)) 107 return DEPRECATED; 108 if ("external".equals(codeString)) 109 return EXTERNAL; 110 throw new FHIRException("Unknown StandardsStatus code '" + codeString + "'"); 111 } 112 113 public String toCode() { 114 switch (this) { 115 case DRAFT: 116 return "draft"; 117 case NORMATIVE: 118 return "normative"; 119 case TRIALUSE: 120 return "trial-use"; 121 case INFORMATIVE: 122 return "informative"; 123 case DEPRECATED: 124 return "deprecated"; 125 case EXTERNAL: 126 return "external"; 127 case NULL: 128 return null; 129 default: 130 return "?"; 131 } 132 } 133 134 public String getSystem() { 135 return "http://terminology.hl7.org/CodeSystem/standards-status"; 136 } 137 138 public String getDefinition() { 139 switch (this) { 140 case DRAFT: 141 return "This portion of the specification is not considered to be complete enough or sufficiently reviewed to be safe for implementation. It may have known issues or still be in the \"in development\" stage. It is included in the publication as a place-holder, to solicit feedback from the implementation community and/or to give implementers some insight as to functionality likely to be included in future versions of the specification. Content at this level should only be implemented by the brave or desperate and is very much \"use at your own risk\". The content that is Draft that will usually be elevated to Trial Use once review and correction is complete after it has been subjected to ballot."; 142 case NORMATIVE: 143 return "This content has been subject to review and production implementation in a wide variety of environments. The content is considered to be stable and has been 'locked', subjecting it to FHIR Inter-version Compatibility Rules. While changes are possible, they are expected to be infrequent and are tightly constrained. Compatibility Rules."; 144 case TRIALUSE: 145 return "This content has been well reviewed and is considered by the authors to be ready for use in production systems. It has been subjected to ballot and approved as an official standard. However, it has not yet seen widespread use in production across the full spectrum of environments it is intended to be used in. In some cases, there may be documented known issues that require implementation experience to determine appropriate resolutions for.\n\nFuture versions of FHIR may make significant changes to Trial Use content that are not compatible with previously published content."; 146 case INFORMATIVE: 147 return "This portion of the specification is provided for implementer assistance, and does not make rules that implementers are required to follow. Typical examples of this content in the FHIR specification are tables of contents, registries, examples, and implementer advice."; 148 case DEPRECATED: 149 return "This portion of the specification is provided for implementer assistance, and does not make rules that implementers are required to follow. Typical examples of this content in the FHIR specification are tables of contents, registries, examples, and implementer advice."; 150 case EXTERNAL: 151 return "This is content that is managed outside the FHIR Specification, but included for implementer convenience."; 152 case NULL: 153 return null; 154 default: 155 return "?"; 156 } 157 } 158 159 public String getDisplay() { 160 switch (this) { 161 case DRAFT: 162 return "Draft"; 163 case NORMATIVE: 164 return "Normative"; 165 case TRIALUSE: 166 return "Trial-Use"; 167 case INFORMATIVE: 168 return "Informative"; 169 case DEPRECATED: 170 return "Deprecated"; 171 case EXTERNAL: 172 return "External"; 173 case NULL: 174 return null; 175 default: 176 return "?"; 177 } 178 } 179 180}