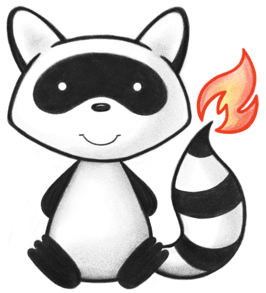
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum TaskCode { 037 038 /** 039 * Take what actions are needed to transition the focus resource from 'draft' to 040 * 'active' or 'in-progress', as appropriate for the resource type. This may 041 * involve additing additional content, approval, validation, etc. 042 */ 043 APPROVE, 044 /** 045 * Act to perform the actions defined in the focus request. This might result in 046 * a 'more assertive' request (order for a plan or proposal, filler order for a 047 * placer order), but is intend to eventually result in events. The degree of 048 * fulfillment requested might be limited by Task.restriction. 049 */ 050 FULFILL, 051 /** 052 * Abort, cancel or withdraw the focal resource, as appropriate for the type of 053 * resource. 054 */ 055 ABORT, 056 /** 057 * Replace the focal resource with the specified input resource 058 */ 059 REPLACE, 060 /** 061 * Update the focal resource of the owning system to reflect the content 062 * specified as the Task.focus 063 */ 064 CHANGE, 065 /** 066 * Transition the focal resource from 'active' or 'in-progress' to 'suspended' 067 */ 068 SUSPEND, 069 /** 070 * Transition the focal resource from 'suspended' to 'active' or 'in-progress' 071 * as appropriate for the resource type. 072 */ 073 RESUME, 074 /** 075 * added to help the parsers 076 */ 077 NULL; 078 079 public static TaskCode fromCode(String codeString) throws FHIRException { 080 if (codeString == null || "".equals(codeString)) 081 return null; 082 if ("approve".equals(codeString)) 083 return APPROVE; 084 if ("fulfill".equals(codeString)) 085 return FULFILL; 086 if ("abort".equals(codeString)) 087 return ABORT; 088 if ("replace".equals(codeString)) 089 return REPLACE; 090 if ("change".equals(codeString)) 091 return CHANGE; 092 if ("suspend".equals(codeString)) 093 return SUSPEND; 094 if ("resume".equals(codeString)) 095 return RESUME; 096 throw new FHIRException("Unknown TaskCode code '" + codeString + "'"); 097 } 098 099 public String toCode() { 100 switch (this) { 101 case APPROVE: 102 return "approve"; 103 case FULFILL: 104 return "fulfill"; 105 case ABORT: 106 return "abort"; 107 case REPLACE: 108 return "replace"; 109 case CHANGE: 110 return "change"; 111 case SUSPEND: 112 return "suspend"; 113 case RESUME: 114 return "resume"; 115 case NULL: 116 return null; 117 default: 118 return "?"; 119 } 120 } 121 122 public String getSystem() { 123 return "http://hl7.org/fhir/CodeSystem/task-code"; 124 } 125 126 public String getDefinition() { 127 switch (this) { 128 case APPROVE: 129 return "Take what actions are needed to transition the focus resource from 'draft' to 'active' or 'in-progress', as appropriate for the resource type. This may involve additing additional content, approval, validation, etc."; 130 case FULFILL: 131 return "Act to perform the actions defined in the focus request. This might result in a 'more assertive' request (order for a plan or proposal, filler order for a placer order), but is intend to eventually result in events. The degree of fulfillment requested might be limited by Task.restriction."; 132 case ABORT: 133 return "Abort, cancel or withdraw the focal resource, as appropriate for the type of resource."; 134 case REPLACE: 135 return "Replace the focal resource with the specified input resource"; 136 case CHANGE: 137 return "Update the focal resource of the owning system to reflect the content specified as the Task.focus"; 138 case SUSPEND: 139 return "Transition the focal resource from 'active' or 'in-progress' to 'suspended'"; 140 case RESUME: 141 return "Transition the focal resource from 'suspended' to 'active' or 'in-progress' as appropriate for the resource type."; 142 case NULL: 143 return null; 144 default: 145 return "?"; 146 } 147 } 148 149 public String getDisplay() { 150 switch (this) { 151 case APPROVE: 152 return "Activate/approve the focal resource"; 153 case FULFILL: 154 return "Fulfill the focal request"; 155 case ABORT: 156 return "Mark the focal resource as no longer active"; 157 case REPLACE: 158 return "Replace the focal resource with the input resource"; 159 case CHANGE: 160 return "Change the focal resource"; 161 case SUSPEND: 162 return "Suspend the focal resource"; 163 case RESUME: 164 return "Re-activate the focal resource"; 165 case NULL: 166 return null; 167 default: 168 return "?"; 169 } 170 } 171 172}