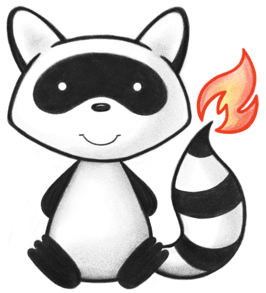
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum TaskStatus { 037 038 /** 039 * The task is not yet ready to be acted upon. 040 */ 041 DRAFT, 042 /** 043 * The task is ready to be acted upon and action is sought. 044 */ 045 REQUESTED, 046 /** 047 * A potential performer has claimed ownership of the task and is evaluating 048 * whether to perform it. 049 */ 050 RECEIVED, 051 /** 052 * The potential performer has agreed to execute the task but has not yet 053 * started work. 054 */ 055 ACCEPTED, 056 /** 057 * The potential performer who claimed ownership of the task has decided not to 058 * execute it prior to performing any action. 059 */ 060 REJECTED, 061 /** 062 * The task is ready to be performed, but no action has yet been taken. Used in 063 * place of requested/received/accepted/rejected when request assignment and 064 * acceptance is a given. 065 */ 066 READY, 067 /** 068 * The task was not completed. 069 */ 070 CANCELLED, 071 /** 072 * The task has been started but is not yet complete. 073 */ 074 INPROGRESS, 075 /** 076 * The task has been started but work has been paused. 077 */ 078 ONHOLD, 079 /** 080 * The task was attempted but could not be completed due to some error. 081 */ 082 FAILED, 083 /** 084 * The task has been completed. 085 */ 086 COMPLETED, 087 /** 088 * The task should never have existed and is retained only because of the 089 * possibility it may have used. 090 */ 091 ENTEREDINERROR, 092 /** 093 * added to help the parsers 094 */ 095 NULL; 096 097 public static TaskStatus fromCode(String codeString) throws FHIRException { 098 if (codeString == null || "".equals(codeString)) 099 return null; 100 if ("draft".equals(codeString)) 101 return DRAFT; 102 if ("requested".equals(codeString)) 103 return REQUESTED; 104 if ("received".equals(codeString)) 105 return RECEIVED; 106 if ("accepted".equals(codeString)) 107 return ACCEPTED; 108 if ("rejected".equals(codeString)) 109 return REJECTED; 110 if ("ready".equals(codeString)) 111 return READY; 112 if ("cancelled".equals(codeString)) 113 return CANCELLED; 114 if ("in-progress".equals(codeString)) 115 return INPROGRESS; 116 if ("on-hold".equals(codeString)) 117 return ONHOLD; 118 if ("failed".equals(codeString)) 119 return FAILED; 120 if ("completed".equals(codeString)) 121 return COMPLETED; 122 if ("entered-in-error".equals(codeString)) 123 return ENTEREDINERROR; 124 throw new FHIRException("Unknown TaskStatus code '" + codeString + "'"); 125 } 126 127 public String toCode() { 128 switch (this) { 129 case DRAFT: 130 return "draft"; 131 case REQUESTED: 132 return "requested"; 133 case RECEIVED: 134 return "received"; 135 case ACCEPTED: 136 return "accepted"; 137 case REJECTED: 138 return "rejected"; 139 case READY: 140 return "ready"; 141 case CANCELLED: 142 return "cancelled"; 143 case INPROGRESS: 144 return "in-progress"; 145 case ONHOLD: 146 return "on-hold"; 147 case FAILED: 148 return "failed"; 149 case COMPLETED: 150 return "completed"; 151 case ENTEREDINERROR: 152 return "entered-in-error"; 153 case NULL: 154 return null; 155 default: 156 return "?"; 157 } 158 } 159 160 public String getSystem() { 161 return "http://hl7.org/fhir/task-status"; 162 } 163 164 public String getDefinition() { 165 switch (this) { 166 case DRAFT: 167 return "The task is not yet ready to be acted upon."; 168 case REQUESTED: 169 return "The task is ready to be acted upon and action is sought."; 170 case RECEIVED: 171 return "A potential performer has claimed ownership of the task and is evaluating whether to perform it."; 172 case ACCEPTED: 173 return "The potential performer has agreed to execute the task but has not yet started work."; 174 case REJECTED: 175 return "The potential performer who claimed ownership of the task has decided not to execute it prior to performing any action."; 176 case READY: 177 return "The task is ready to be performed, but no action has yet been taken. Used in place of requested/received/accepted/rejected when request assignment and acceptance is a given."; 178 case CANCELLED: 179 return "The task was not completed."; 180 case INPROGRESS: 181 return "The task has been started but is not yet complete."; 182 case ONHOLD: 183 return "The task has been started but work has been paused."; 184 case FAILED: 185 return "The task was attempted but could not be completed due to some error."; 186 case COMPLETED: 187 return "The task has been completed."; 188 case ENTEREDINERROR: 189 return "The task should never have existed and is retained only because of the possibility it may have used."; 190 case NULL: 191 return null; 192 default: 193 return "?"; 194 } 195 } 196 197 public String getDisplay() { 198 switch (this) { 199 case DRAFT: 200 return "Draft"; 201 case REQUESTED: 202 return "Requested"; 203 case RECEIVED: 204 return "Received"; 205 case ACCEPTED: 206 return "Accepted"; 207 case REJECTED: 208 return "Rejected"; 209 case READY: 210 return "Ready"; 211 case CANCELLED: 212 return "Cancelled"; 213 case INPROGRESS: 214 return "In Progress"; 215 case ONHOLD: 216 return "On Hold"; 217 case FAILED: 218 return "Failed"; 219 case COMPLETED: 220 return "Completed"; 221 case ENTEREDINERROR: 222 return "Entered in Error"; 223 case NULL: 224 return null; 225 default: 226 return "?"; 227 } 228 } 229 230}