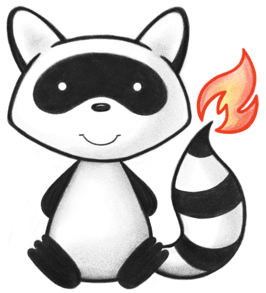
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum Teeth { 037 038 /** 039 * Upper Right Tooth 1 from the central axis, permanent dentition. 040 */ 041 _11, 042 /** 043 * Upper Right Tooth 2 from the central axis, permanent dentition. 044 */ 045 _12, 046 /** 047 * Upper Right Tooth 3 from the central axis, permanent dentition. 048 */ 049 _13, 050 /** 051 * Upper Right Tooth 4 from the central axis, permanent dentition. 052 */ 053 _14, 054 /** 055 * Upper Right Tooth 5 from the central axis, permanent dentition. 056 */ 057 _15, 058 /** 059 * Upper Right Tooth 6 from the central axis, permanent dentition. 060 */ 061 _16, 062 /** 063 * Upper Right Tooth 7 from the central axis, permanent dentition. 064 */ 065 _17, 066 /** 067 * Upper Right Tooth 8 from the central axis, permanent dentition. 068 */ 069 _18, 070 /** 071 * Upper Left Tooth 1 from the central axis, permanent dentition. 072 */ 073 _21, 074 /** 075 * Upper Left Tooth 2 from the central axis, permanent dentition. 076 */ 077 _22, 078 /** 079 * Upper Left Tooth 3 from the central axis, permanent dentition. 080 */ 081 _23, 082 /** 083 * Upper Left Tooth 4 from the central axis, permanent dentition. 084 */ 085 _24, 086 /** 087 * Upper Left Tooth 5 from the central axis, permanent dentition. 088 */ 089 _25, 090 /** 091 * Upper Left Tooth 6 from the central axis, permanent dentition. 092 */ 093 _26, 094 /** 095 * Upper Left Tooth 7 from the central axis, permanent dentition. 096 */ 097 _27, 098 /** 099 * Upper Left Tooth 8 from the central axis, permanent dentition. 100 */ 101 _28, 102 /** 103 * Lower Left Tooth 1 from the central axis, permanent dentition. 104 */ 105 _31, 106 /** 107 * Lower Left Tooth 2 from the central axis, permanent dentition. 108 */ 109 _32, 110 /** 111 * Lower Left Tooth 3 from the central axis, permanent dentition. 112 */ 113 _33, 114 /** 115 * Lower Left Tooth 4 from the central axis, permanent dentition. 116 */ 117 _34, 118 /** 119 * Lower Left Tooth 5 from the central axis, permanent dentition. 120 */ 121 _35, 122 /** 123 * Lower Left Tooth 6 from the central axis, permanent dentition. 124 */ 125 _36, 126 /** 127 * Lower Left Tooth 7 from the central axis, permanent dentition. 128 */ 129 _37, 130 /** 131 * Lower Left Tooth 8 from the central axis, permanent dentition. 132 */ 133 _38, 134 /** 135 * Lower Right Tooth 1 from the central axis, permanent dentition. 136 */ 137 _41, 138 /** 139 * Lower Right Tooth 2 from the central axis, permanent dentition. 140 */ 141 _42, 142 /** 143 * Lower Right Tooth 3 from the central axis, permanent dentition. 144 */ 145 _43, 146 /** 147 * Lower Right Tooth 4 from the central axis, permanent dentition. 148 */ 149 _44, 150 /** 151 * Lower Right Tooth 5 from the central axis, permanent dentition. 152 */ 153 _45, 154 /** 155 * Lower Right Tooth 6 from the central axis, permanent dentition. 156 */ 157 _46, 158 /** 159 * Lower Right Tooth 7 from the central axis, permanent dentition. 160 */ 161 _47, 162 /** 163 * Lower Right Tooth 8 from the central axis, permanent dentition. 164 */ 165 _48, 166 /** 167 * added to help the parsers 168 */ 169 NULL; 170 171 public static Teeth fromCode(String codeString) throws FHIRException { 172 if (codeString == null || "".equals(codeString)) 173 return null; 174 if ("11".equals(codeString)) 175 return _11; 176 if ("12".equals(codeString)) 177 return _12; 178 if ("13".equals(codeString)) 179 return _13; 180 if ("14".equals(codeString)) 181 return _14; 182 if ("15".equals(codeString)) 183 return _15; 184 if ("16".equals(codeString)) 185 return _16; 186 if ("17".equals(codeString)) 187 return _17; 188 if ("18".equals(codeString)) 189 return _18; 190 if ("21".equals(codeString)) 191 return _21; 192 if ("22".equals(codeString)) 193 return _22; 194 if ("23".equals(codeString)) 195 return _23; 196 if ("24".equals(codeString)) 197 return _24; 198 if ("25".equals(codeString)) 199 return _25; 200 if ("26".equals(codeString)) 201 return _26; 202 if ("27".equals(codeString)) 203 return _27; 204 if ("28".equals(codeString)) 205 return _28; 206 if ("31".equals(codeString)) 207 return _31; 208 if ("32".equals(codeString)) 209 return _32; 210 if ("33".equals(codeString)) 211 return _33; 212 if ("34".equals(codeString)) 213 return _34; 214 if ("35".equals(codeString)) 215 return _35; 216 if ("36".equals(codeString)) 217 return _36; 218 if ("37".equals(codeString)) 219 return _37; 220 if ("38".equals(codeString)) 221 return _38; 222 if ("41".equals(codeString)) 223 return _41; 224 if ("42".equals(codeString)) 225 return _42; 226 if ("43".equals(codeString)) 227 return _43; 228 if ("44".equals(codeString)) 229 return _44; 230 if ("45".equals(codeString)) 231 return _45; 232 if ("46".equals(codeString)) 233 return _46; 234 if ("47".equals(codeString)) 235 return _47; 236 if ("48".equals(codeString)) 237 return _48; 238 throw new FHIRException("Unknown Teeth code '" + codeString + "'"); 239 } 240 241 public String toCode() { 242 switch (this) { 243 case _11: 244 return "11"; 245 case _12: 246 return "12"; 247 case _13: 248 return "13"; 249 case _14: 250 return "14"; 251 case _15: 252 return "15"; 253 case _16: 254 return "16"; 255 case _17: 256 return "17"; 257 case _18: 258 return "18"; 259 case _21: 260 return "21"; 261 case _22: 262 return "22"; 263 case _23: 264 return "23"; 265 case _24: 266 return "24"; 267 case _25: 268 return "25"; 269 case _26: 270 return "26"; 271 case _27: 272 return "27"; 273 case _28: 274 return "28"; 275 case _31: 276 return "31"; 277 case _32: 278 return "32"; 279 case _33: 280 return "33"; 281 case _34: 282 return "34"; 283 case _35: 284 return "35"; 285 case _36: 286 return "36"; 287 case _37: 288 return "37"; 289 case _38: 290 return "38"; 291 case _41: 292 return "41"; 293 case _42: 294 return "42"; 295 case _43: 296 return "43"; 297 case _44: 298 return "44"; 299 case _45: 300 return "45"; 301 case _46: 302 return "46"; 303 case _47: 304 return "47"; 305 case _48: 306 return "48"; 307 case NULL: 308 return null; 309 default: 310 return "?"; 311 } 312 } 313 314 public String getSystem() { 315 return "http://hl7.org/fhir/ex-fdi"; 316 } 317 318 public String getDefinition() { 319 switch (this) { 320 case _11: 321 return "Upper Right Tooth 1 from the central axis, permanent dentition."; 322 case _12: 323 return "Upper Right Tooth 2 from the central axis, permanent dentition."; 324 case _13: 325 return "Upper Right Tooth 3 from the central axis, permanent dentition."; 326 case _14: 327 return "Upper Right Tooth 4 from the central axis, permanent dentition."; 328 case _15: 329 return "Upper Right Tooth 5 from the central axis, permanent dentition."; 330 case _16: 331 return "Upper Right Tooth 6 from the central axis, permanent dentition."; 332 case _17: 333 return "Upper Right Tooth 7 from the central axis, permanent dentition."; 334 case _18: 335 return "Upper Right Tooth 8 from the central axis, permanent dentition."; 336 case _21: 337 return "Upper Left Tooth 1 from the central axis, permanent dentition."; 338 case _22: 339 return "Upper Left Tooth 2 from the central axis, permanent dentition."; 340 case _23: 341 return "Upper Left Tooth 3 from the central axis, permanent dentition."; 342 case _24: 343 return "Upper Left Tooth 4 from the central axis, permanent dentition."; 344 case _25: 345 return "Upper Left Tooth 5 from the central axis, permanent dentition."; 346 case _26: 347 return "Upper Left Tooth 6 from the central axis, permanent dentition."; 348 case _27: 349 return "Upper Left Tooth 7 from the central axis, permanent dentition."; 350 case _28: 351 return "Upper Left Tooth 8 from the central axis, permanent dentition."; 352 case _31: 353 return "Lower Left Tooth 1 from the central axis, permanent dentition."; 354 case _32: 355 return "Lower Left Tooth 2 from the central axis, permanent dentition."; 356 case _33: 357 return "Lower Left Tooth 3 from the central axis, permanent dentition."; 358 case _34: 359 return "Lower Left Tooth 4 from the central axis, permanent dentition."; 360 case _35: 361 return "Lower Left Tooth 5 from the central axis, permanent dentition."; 362 case _36: 363 return "Lower Left Tooth 6 from the central axis, permanent dentition."; 364 case _37: 365 return "Lower Left Tooth 7 from the central axis, permanent dentition."; 366 case _38: 367 return "Lower Left Tooth 8 from the central axis, permanent dentition."; 368 case _41: 369 return "Lower Right Tooth 1 from the central axis, permanent dentition."; 370 case _42: 371 return "Lower Right Tooth 2 from the central axis, permanent dentition."; 372 case _43: 373 return "Lower Right Tooth 3 from the central axis, permanent dentition."; 374 case _44: 375 return "Lower Right Tooth 4 from the central axis, permanent dentition."; 376 case _45: 377 return "Lower Right Tooth 5 from the central axis, permanent dentition."; 378 case _46: 379 return "Lower Right Tooth 6 from the central axis, permanent dentition."; 380 case _47: 381 return "Lower Right Tooth 7 from the central axis, permanent dentition."; 382 case _48: 383 return "Lower Right Tooth 8 from the central axis, permanent dentition."; 384 case NULL: 385 return null; 386 default: 387 return "?"; 388 } 389 } 390 391 public String getDisplay() { 392 switch (this) { 393 case _11: 394 return "11"; 395 case _12: 396 return "12"; 397 case _13: 398 return "13"; 399 case _14: 400 return "14"; 401 case _15: 402 return "15"; 403 case _16: 404 return "16"; 405 case _17: 406 return "17"; 407 case _18: 408 return "18"; 409 case _21: 410 return "21"; 411 case _22: 412 return "22"; 413 case _23: 414 return "23"; 415 case _24: 416 return "24"; 417 case _25: 418 return "25"; 419 case _26: 420 return "26"; 421 case _27: 422 return "27"; 423 case _28: 424 return "28"; 425 case _31: 426 return "31"; 427 case _32: 428 return "32"; 429 case _33: 430 return "33"; 431 case _34: 432 return "34"; 433 case _35: 434 return "35"; 435 case _36: 436 return "36"; 437 case _37: 438 return "37"; 439 case _38: 440 return "38"; 441 case _41: 442 return "41"; 443 case _42: 444 return "42"; 445 case _43: 446 return "43"; 447 case _44: 448 return "44"; 449 case _45: 450 return "45"; 451 case _46: 452 return "46"; 453 case _47: 454 return "47"; 455 case _48: 456 return "48"; 457 case NULL: 458 return null; 459 default: 460 return "?"; 461 } 462 } 463 464}