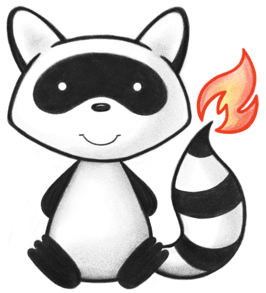
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum TestscriptOperationCodes { 037 038 /** 039 * Read the current state of the resource. 040 */ 041 READ, 042 /** 043 * Read the state of a specific version of the resource. 044 */ 045 VREAD, 046 /** 047 * Update an existing resource by its id. 048 */ 049 UPDATE, 050 /** 051 * Update an existing resource by its id (or create it if it is new). 052 */ 053 UPDATECREATE, 054 /** 055 * Patch an existing resource by its id. 056 */ 057 PATCH, 058 /** 059 * Delete a resource. 060 */ 061 DELETE, 062 /** 063 * Conditionally delete a single resource based on search parameters. 064 */ 065 DELETECONDSINGLE, 066 /** 067 * Conditionally delete one or more resources based on search parameters. 068 */ 069 DELETECONDMULTIPLE, 070 /** 071 * Retrieve the change history for a particular resource or resource type. 072 */ 073 HISTORY, 074 /** 075 * Create a new resource with a server assigned id. 076 */ 077 CREATE, 078 /** 079 * Search based on some filter criteria. 080 */ 081 SEARCH, 082 /** 083 * Update, create or delete a set of resources as independent actions. 084 */ 085 BATCH, 086 /** 087 * Update, create or delete a set of resources as a single transaction. 088 */ 089 TRANSACTION, 090 /** 091 * Get a capability statement for the system. 092 */ 093 CAPABILITIES, 094 /** 095 * Realizes an ActivityDefinition in a specific context 096 */ 097 APPLY, 098 /** 099 * Closure Table Maintenance 100 */ 101 CLOSURE, 102 /** 103 * Finding Codes based on supplied properties 104 */ 105 FINDMATCHES, 106 /** 107 * Compare two systems CapabilityStatements 108 */ 109 CONFORMS, 110 /** 111 * Aggregates and returns the parameters and data requirements for a resource 112 * and all its dependencies as a single module definition 113 */ 114 DATAREQUIREMENTS, 115 /** 116 * Generate a Document 117 */ 118 DOCUMENT, 119 /** 120 * Request clinical decision support guidance based on a specific decision 121 * support module 122 */ 123 EVALUATE, 124 /** 125 * Invoke an eMeasure and obtain the results 126 */ 127 EVALUATEMEASURE, 128 /** 129 * Return all the related information as described in the Encounter or Patient 130 */ 131 EVERYTHING, 132 /** 133 * Value Set Expansion 134 */ 135 EXPAND, 136 /** 137 * Find a functional list 138 */ 139 FIND, 140 /** 141 * Invoke a GraphQL query 142 */ 143 GRAPHQL, 144 /** 145 * Test if a server implements a client's required operations 146 */ 147 IMPLEMENTS, 148 /** 149 * Last N Observations Query 150 */ 151 LASTN, 152 /** 153 * Concept Look Up and Decomposition 154 */ 155 LOOKUP, 156 /** 157 * Find patient matches using MPI based logic 158 */ 159 MATCH, 160 /** 161 * Access a list of profiles, tags, and security labels 162 */ 163 META, 164 /** 165 * Add profiles, tags, and security labels to a resource 166 */ 167 METAADD, 168 /** 169 * Delete profiles, tags, and security labels for a resource 170 */ 171 METADELETE, 172 /** 173 * Populate Questionnaire 174 */ 175 POPULATE, 176 /** 177 * Generate HTML for Questionnaire 178 */ 179 POPULATEHTML, 180 /** 181 * Generate a link to a Questionnaire completion webpage 182 */ 183 POPULATELINK, 184 /** 185 * Process a message according to the defined event 186 */ 187 PROCESSMESSAGE, 188 /** 189 * Build Questionnaire 190 */ 191 QUESTIONNAIRE, 192 /** 193 * Observation Statistics 194 */ 195 STATS, 196 /** 197 * Fetch a subset of the CapabilityStatement resource 198 */ 199 SUBSET, 200 /** 201 * CodeSystem Subsumption Testing 202 */ 203 SUBSUMES, 204 /** 205 * Model Instance Transformation 206 */ 207 TRANSFORM, 208 /** 209 * Concept Translation 210 */ 211 TRANSLATE, 212 /** 213 * Validate a resource 214 */ 215 VALIDATE, 216 /** 217 * ValueSet based Validation 218 */ 219 VALIDATECODE, 220 /** 221 * added to help the parsers 222 */ 223 NULL; 224 225 public static TestscriptOperationCodes fromCode(String codeString) throws FHIRException { 226 if (codeString == null || "".equals(codeString)) 227 return null; 228 if ("read".equals(codeString)) 229 return READ; 230 if ("vread".equals(codeString)) 231 return VREAD; 232 if ("update".equals(codeString)) 233 return UPDATE; 234 if ("updateCreate".equals(codeString)) 235 return UPDATECREATE; 236 if ("patch".equals(codeString)) 237 return PATCH; 238 if ("delete".equals(codeString)) 239 return DELETE; 240 if ("deleteCondSingle".equals(codeString)) 241 return DELETECONDSINGLE; 242 if ("deleteCondMultiple".equals(codeString)) 243 return DELETECONDMULTIPLE; 244 if ("history".equals(codeString)) 245 return HISTORY; 246 if ("create".equals(codeString)) 247 return CREATE; 248 if ("search".equals(codeString)) 249 return SEARCH; 250 if ("batch".equals(codeString)) 251 return BATCH; 252 if ("transaction".equals(codeString)) 253 return TRANSACTION; 254 if ("capabilities".equals(codeString)) 255 return CAPABILITIES; 256 if ("apply".equals(codeString)) 257 return APPLY; 258 if ("closure".equals(codeString)) 259 return CLOSURE; 260 if ("find-matches".equals(codeString)) 261 return FINDMATCHES; 262 if ("conforms".equals(codeString)) 263 return CONFORMS; 264 if ("data-requirements".equals(codeString)) 265 return DATAREQUIREMENTS; 266 if ("document".equals(codeString)) 267 return DOCUMENT; 268 if ("evaluate".equals(codeString)) 269 return EVALUATE; 270 if ("evaluate-measure".equals(codeString)) 271 return EVALUATEMEASURE; 272 if ("everything".equals(codeString)) 273 return EVERYTHING; 274 if ("expand".equals(codeString)) 275 return EXPAND; 276 if ("find".equals(codeString)) 277 return FIND; 278 if ("graphql".equals(codeString)) 279 return GRAPHQL; 280 if ("implements".equals(codeString)) 281 return IMPLEMENTS; 282 if ("lastn".equals(codeString)) 283 return LASTN; 284 if ("lookup".equals(codeString)) 285 return LOOKUP; 286 if ("match".equals(codeString)) 287 return MATCH; 288 if ("meta".equals(codeString)) 289 return META; 290 if ("meta-add".equals(codeString)) 291 return METAADD; 292 if ("meta-delete".equals(codeString)) 293 return METADELETE; 294 if ("populate".equals(codeString)) 295 return POPULATE; 296 if ("populatehtml".equals(codeString)) 297 return POPULATEHTML; 298 if ("populatelink".equals(codeString)) 299 return POPULATELINK; 300 if ("process-message".equals(codeString)) 301 return PROCESSMESSAGE; 302 if ("questionnaire".equals(codeString)) 303 return QUESTIONNAIRE; 304 if ("stats".equals(codeString)) 305 return STATS; 306 if ("subset".equals(codeString)) 307 return SUBSET; 308 if ("subsumes".equals(codeString)) 309 return SUBSUMES; 310 if ("transform".equals(codeString)) 311 return TRANSFORM; 312 if ("translate".equals(codeString)) 313 return TRANSLATE; 314 if ("validate".equals(codeString)) 315 return VALIDATE; 316 if ("validate-code".equals(codeString)) 317 return VALIDATECODE; 318 throw new FHIRException("Unknown TestscriptOperationCodes code '" + codeString + "'"); 319 } 320 321 public String toCode() { 322 switch (this) { 323 case READ: 324 return "read"; 325 case VREAD: 326 return "vread"; 327 case UPDATE: 328 return "update"; 329 case UPDATECREATE: 330 return "updateCreate"; 331 case PATCH: 332 return "patch"; 333 case DELETE: 334 return "delete"; 335 case DELETECONDSINGLE: 336 return "deleteCondSingle"; 337 case DELETECONDMULTIPLE: 338 return "deleteCondMultiple"; 339 case HISTORY: 340 return "history"; 341 case CREATE: 342 return "create"; 343 case SEARCH: 344 return "search"; 345 case BATCH: 346 return "batch"; 347 case TRANSACTION: 348 return "transaction"; 349 case CAPABILITIES: 350 return "capabilities"; 351 case APPLY: 352 return "apply"; 353 case CLOSURE: 354 return "closure"; 355 case FINDMATCHES: 356 return "find-matches"; 357 case CONFORMS: 358 return "conforms"; 359 case DATAREQUIREMENTS: 360 return "data-requirements"; 361 case DOCUMENT: 362 return "document"; 363 case EVALUATE: 364 return "evaluate"; 365 case EVALUATEMEASURE: 366 return "evaluate-measure"; 367 case EVERYTHING: 368 return "everything"; 369 case EXPAND: 370 return "expand"; 371 case FIND: 372 return "find"; 373 case GRAPHQL: 374 return "graphql"; 375 case IMPLEMENTS: 376 return "implements"; 377 case LASTN: 378 return "lastn"; 379 case LOOKUP: 380 return "lookup"; 381 case MATCH: 382 return "match"; 383 case META: 384 return "meta"; 385 case METAADD: 386 return "meta-add"; 387 case METADELETE: 388 return "meta-delete"; 389 case POPULATE: 390 return "populate"; 391 case POPULATEHTML: 392 return "populatehtml"; 393 case POPULATELINK: 394 return "populatelink"; 395 case PROCESSMESSAGE: 396 return "process-message"; 397 case QUESTIONNAIRE: 398 return "questionnaire"; 399 case STATS: 400 return "stats"; 401 case SUBSET: 402 return "subset"; 403 case SUBSUMES: 404 return "subsumes"; 405 case TRANSFORM: 406 return "transform"; 407 case TRANSLATE: 408 return "translate"; 409 case VALIDATE: 410 return "validate"; 411 case VALIDATECODE: 412 return "validate-code"; 413 case NULL: 414 return null; 415 default: 416 return "?"; 417 } 418 } 419 420 public String getSystem() { 421 return "http://terminology.hl7.org/CodeSystem/testscript-operation-codes"; 422 } 423 424 public String getDefinition() { 425 switch (this) { 426 case READ: 427 return "Read the current state of the resource."; 428 case VREAD: 429 return "Read the state of a specific version of the resource."; 430 case UPDATE: 431 return "Update an existing resource by its id."; 432 case UPDATECREATE: 433 return "Update an existing resource by its id (or create it if it is new)."; 434 case PATCH: 435 return "Patch an existing resource by its id."; 436 case DELETE: 437 return "Delete a resource."; 438 case DELETECONDSINGLE: 439 return "Conditionally delete a single resource based on search parameters."; 440 case DELETECONDMULTIPLE: 441 return "Conditionally delete one or more resources based on search parameters."; 442 case HISTORY: 443 return "Retrieve the change history for a particular resource or resource type."; 444 case CREATE: 445 return "Create a new resource with a server assigned id."; 446 case SEARCH: 447 return "Search based on some filter criteria."; 448 case BATCH: 449 return "Update, create or delete a set of resources as independent actions."; 450 case TRANSACTION: 451 return "Update, create or delete a set of resources as a single transaction."; 452 case CAPABILITIES: 453 return "Get a capability statement for the system."; 454 case APPLY: 455 return "Realizes an ActivityDefinition in a specific context"; 456 case CLOSURE: 457 return "Closure Table Maintenance"; 458 case FINDMATCHES: 459 return "Finding Codes based on supplied properties"; 460 case CONFORMS: 461 return "Compare two systems CapabilityStatements"; 462 case DATAREQUIREMENTS: 463 return "Aggregates and returns the parameters and data requirements for a resource and all its dependencies as a single module definition"; 464 case DOCUMENT: 465 return "Generate a Document"; 466 case EVALUATE: 467 return "Request clinical decision support guidance based on a specific decision support module"; 468 case EVALUATEMEASURE: 469 return "Invoke an eMeasure and obtain the results"; 470 case EVERYTHING: 471 return "Return all the related information as described in the Encounter or Patient"; 472 case EXPAND: 473 return "Value Set Expansion"; 474 case FIND: 475 return "Find a functional list"; 476 case GRAPHQL: 477 return "Invoke a GraphQL query"; 478 case IMPLEMENTS: 479 return "Test if a server implements a client's required operations"; 480 case LASTN: 481 return "Last N Observations Query"; 482 case LOOKUP: 483 return "Concept Look Up and Decomposition"; 484 case MATCH: 485 return "Find patient matches using MPI based logic"; 486 case META: 487 return "Access a list of profiles, tags, and security labels"; 488 case METAADD: 489 return "Add profiles, tags, and security labels to a resource"; 490 case METADELETE: 491 return "Delete profiles, tags, and security labels for a resource"; 492 case POPULATE: 493 return "Populate Questionnaire"; 494 case POPULATEHTML: 495 return "Generate HTML for Questionnaire"; 496 case POPULATELINK: 497 return "Generate a link to a Questionnaire completion webpage"; 498 case PROCESSMESSAGE: 499 return "Process a message according to the defined event"; 500 case QUESTIONNAIRE: 501 return "Build Questionnaire"; 502 case STATS: 503 return "Observation Statistics"; 504 case SUBSET: 505 return "Fetch a subset of the CapabilityStatement resource"; 506 case SUBSUMES: 507 return "CodeSystem Subsumption Testing"; 508 case TRANSFORM: 509 return "Model Instance Transformation"; 510 case TRANSLATE: 511 return "Concept Translation"; 512 case VALIDATE: 513 return "Validate a resource"; 514 case VALIDATECODE: 515 return "ValueSet based Validation"; 516 case NULL: 517 return null; 518 default: 519 return "?"; 520 } 521 } 522 523 public String getDisplay() { 524 switch (this) { 525 case READ: 526 return "Read"; 527 case VREAD: 528 return "Version Read"; 529 case UPDATE: 530 return "Update"; 531 case UPDATECREATE: 532 return "Create using Update"; 533 case PATCH: 534 return "Patch"; 535 case DELETE: 536 return "Delete"; 537 case DELETECONDSINGLE: 538 return "Conditional Delete Single"; 539 case DELETECONDMULTIPLE: 540 return "Conditional Delete Multiple"; 541 case HISTORY: 542 return "History"; 543 case CREATE: 544 return "Create"; 545 case SEARCH: 546 return "Search"; 547 case BATCH: 548 return "Batch"; 549 case TRANSACTION: 550 return "Transaction"; 551 case CAPABILITIES: 552 return "Capabilities"; 553 case APPLY: 554 return "$apply"; 555 case CLOSURE: 556 return "$closure"; 557 case FINDMATCHES: 558 return "$find-matches"; 559 case CONFORMS: 560 return "$conforms"; 561 case DATAREQUIREMENTS: 562 return "$data-requirements"; 563 case DOCUMENT: 564 return "$document"; 565 case EVALUATE: 566 return "$evaluate"; 567 case EVALUATEMEASURE: 568 return "$evaluate-measure"; 569 case EVERYTHING: 570 return "$everything"; 571 case EXPAND: 572 return "$expand"; 573 case FIND: 574 return "$find"; 575 case GRAPHQL: 576 return "$graphql"; 577 case IMPLEMENTS: 578 return "$implements"; 579 case LASTN: 580 return "$lastn"; 581 case LOOKUP: 582 return "$lookup"; 583 case MATCH: 584 return "$match"; 585 case META: 586 return "$meta"; 587 case METAADD: 588 return "$meta-add"; 589 case METADELETE: 590 return "$meta-delete"; 591 case POPULATE: 592 return "$populate"; 593 case POPULATEHTML: 594 return "$populatehtml"; 595 case POPULATELINK: 596 return "$populatelink"; 597 case PROCESSMESSAGE: 598 return "$process-message"; 599 case QUESTIONNAIRE: 600 return "$questionnaire"; 601 case STATS: 602 return "$stats"; 603 case SUBSET: 604 return "$subset"; 605 case SUBSUMES: 606 return "$subsumes"; 607 case TRANSFORM: 608 return "$transform"; 609 case TRANSLATE: 610 return "$translate"; 611 case VALIDATE: 612 return "$validate"; 613 case VALIDATECODE: 614 return "$validate-code"; 615 case NULL: 616 return null; 617 default: 618 return "?"; 619 } 620 } 621 622}