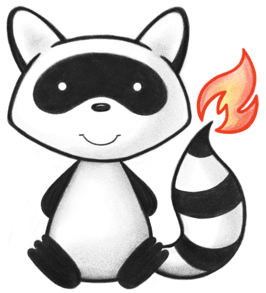
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum Tooth { 037 038 /** 039 * Oral cavity. 040 */ 041 _0, 042 /** 043 * Permanent teeth Maxillary right. 044 */ 045 _1, 046 /** 047 * Permanent teeth Maxillary left. 048 */ 049 _2, 050 /** 051 * Permanent teeth Mandibular right. 052 */ 053 _3, 054 /** 055 * Permanent teeth Mandibular left. 056 */ 057 _4, 058 /** 059 * Deciduous teeth Maxillary right. 060 */ 061 _5, 062 /** 063 * Deciduous teeth Maxillary left. 064 */ 065 _6, 066 /** 067 * Deciduous teeth Mandibular right. 068 */ 069 _7, 070 /** 071 * Deciduous teeth Mandibular left. 072 */ 073 _8, 074 /** 075 * Upper Right Tooth 1 from the central axis, permanent dentition. 076 */ 077 _11, 078 /** 079 * Upper Right Tooth 2 from the central axis, permanent dentition. 080 */ 081 _12, 082 /** 083 * Upper Right Tooth 3 from the central axis, permanent dentition. 084 */ 085 _13, 086 /** 087 * Upper Right Tooth 4 from the central axis, permanent dentition. 088 */ 089 _14, 090 /** 091 * Upper Right Tooth 5 from the central axis, permanent dentition. 092 */ 093 _15, 094 /** 095 * Upper Right Tooth 6 from the central axis, permanent dentition. 096 */ 097 _16, 098 /** 099 * Upper Right Tooth 7 from the central axis, permanent dentition. 100 */ 101 _17, 102 /** 103 * Upper Right Tooth 8 from the central axis, permanent dentition. 104 */ 105 _18, 106 /** 107 * Upper Left Tooth 1 from the central axis, permanent dentition. 108 */ 109 _21, 110 /** 111 * Upper Left Tooth 2 from the central axis, permanent dentition. 112 */ 113 _22, 114 /** 115 * Upper Left Tooth 3 from the central axis, permanent dentition. 116 */ 117 _23, 118 /** 119 * Upper Left Tooth 4 from the central axis, permanent dentition. 120 */ 121 _24, 122 /** 123 * Upper Left Tooth 5 from the central axis, permanent dentition. 124 */ 125 _25, 126 /** 127 * Upper Left Tooth 6 from the central axis, permanent dentition. 128 */ 129 _26, 130 /** 131 * Upper Left Tooth 7 from the central axis, permanent dentition. 132 */ 133 _27, 134 /** 135 * Upper Left Tooth 8 from the central axis, permanent dentition. 136 */ 137 _28, 138 /** 139 * Lower Left Tooth 1 from the central axis, permanent dentition. 140 */ 141 _31, 142 /** 143 * Lower Left Tooth 2 from the central axis, permanent dentition. 144 */ 145 _32, 146 /** 147 * Lower Left Tooth 3 from the central axis, permanent dentition. 148 */ 149 _33, 150 /** 151 * Lower Left Tooth 4 from the central axis, permanent dentition. 152 */ 153 _34, 154 /** 155 * Lower Left Tooth 5 from the central axis, permanent dentition. 156 */ 157 _35, 158 /** 159 * Lower Left Tooth 6 from the central axis, permanent dentition. 160 */ 161 _36, 162 /** 163 * Lower Left Tooth 7 from the central axis, permanent dentition. 164 */ 165 _37, 166 /** 167 * Lower Left Tooth 8 from the central axis, permanent dentition. 168 */ 169 _38, 170 /** 171 * Lower Right Tooth 1 from the central axis, permanent dentition. 172 */ 173 _41, 174 /** 175 * Lower Right Tooth 2 from the central axis, permanent dentition. 176 */ 177 _42, 178 /** 179 * Lower Right Tooth 3 from the central axis, permanent dentition. 180 */ 181 _43, 182 /** 183 * Lower Right Tooth 4 from the central axis, permanent dentition. 184 */ 185 _44, 186 /** 187 * Lower Right Tooth 5 from the central axis, permanent dentition. 188 */ 189 _45, 190 /** 191 * Lower Right Tooth 6 from the central axis, permanent dentition. 192 */ 193 _46, 194 /** 195 * Lower Right Tooth 7 from the central axis, permanent dentition. 196 */ 197 _47, 198 /** 199 * Lower Right Tooth 8 from the central axis, permanent dentition. 200 */ 201 _48, 202 /** 203 * added to help the parsers 204 */ 205 NULL; 206 207 public static Tooth fromCode(String codeString) throws FHIRException { 208 if (codeString == null || "".equals(codeString)) 209 return null; 210 if ("0".equals(codeString)) 211 return _0; 212 if ("1".equals(codeString)) 213 return _1; 214 if ("2".equals(codeString)) 215 return _2; 216 if ("3".equals(codeString)) 217 return _3; 218 if ("4".equals(codeString)) 219 return _4; 220 if ("5".equals(codeString)) 221 return _5; 222 if ("6".equals(codeString)) 223 return _6; 224 if ("7".equals(codeString)) 225 return _7; 226 if ("8".equals(codeString)) 227 return _8; 228 if ("11".equals(codeString)) 229 return _11; 230 if ("12".equals(codeString)) 231 return _12; 232 if ("13".equals(codeString)) 233 return _13; 234 if ("14".equals(codeString)) 235 return _14; 236 if ("15".equals(codeString)) 237 return _15; 238 if ("16".equals(codeString)) 239 return _16; 240 if ("17".equals(codeString)) 241 return _17; 242 if ("18".equals(codeString)) 243 return _18; 244 if ("21".equals(codeString)) 245 return _21; 246 if ("22".equals(codeString)) 247 return _22; 248 if ("23".equals(codeString)) 249 return _23; 250 if ("24".equals(codeString)) 251 return _24; 252 if ("25".equals(codeString)) 253 return _25; 254 if ("26".equals(codeString)) 255 return _26; 256 if ("27".equals(codeString)) 257 return _27; 258 if ("28".equals(codeString)) 259 return _28; 260 if ("31".equals(codeString)) 261 return _31; 262 if ("32".equals(codeString)) 263 return _32; 264 if ("33".equals(codeString)) 265 return _33; 266 if ("34".equals(codeString)) 267 return _34; 268 if ("35".equals(codeString)) 269 return _35; 270 if ("36".equals(codeString)) 271 return _36; 272 if ("37".equals(codeString)) 273 return _37; 274 if ("38".equals(codeString)) 275 return _38; 276 if ("41".equals(codeString)) 277 return _41; 278 if ("42".equals(codeString)) 279 return _42; 280 if ("43".equals(codeString)) 281 return _43; 282 if ("44".equals(codeString)) 283 return _44; 284 if ("45".equals(codeString)) 285 return _45; 286 if ("46".equals(codeString)) 287 return _46; 288 if ("47".equals(codeString)) 289 return _47; 290 if ("48".equals(codeString)) 291 return _48; 292 throw new FHIRException("Unknown Tooth code '" + codeString + "'"); 293 } 294 295 public String toCode() { 296 switch (this) { 297 case _0: 298 return "0"; 299 case _1: 300 return "1"; 301 case _2: 302 return "2"; 303 case _3: 304 return "3"; 305 case _4: 306 return "4"; 307 case _5: 308 return "5"; 309 case _6: 310 return "6"; 311 case _7: 312 return "7"; 313 case _8: 314 return "8"; 315 case _11: 316 return "11"; 317 case _12: 318 return "12"; 319 case _13: 320 return "13"; 321 case _14: 322 return "14"; 323 case _15: 324 return "15"; 325 case _16: 326 return "16"; 327 case _17: 328 return "17"; 329 case _18: 330 return "18"; 331 case _21: 332 return "21"; 333 case _22: 334 return "22"; 335 case _23: 336 return "23"; 337 case _24: 338 return "24"; 339 case _25: 340 return "25"; 341 case _26: 342 return "26"; 343 case _27: 344 return "27"; 345 case _28: 346 return "28"; 347 case _31: 348 return "31"; 349 case _32: 350 return "32"; 351 case _33: 352 return "33"; 353 case _34: 354 return "34"; 355 case _35: 356 return "35"; 357 case _36: 358 return "36"; 359 case _37: 360 return "37"; 361 case _38: 362 return "38"; 363 case _41: 364 return "41"; 365 case _42: 366 return "42"; 367 case _43: 368 return "43"; 369 case _44: 370 return "44"; 371 case _45: 372 return "45"; 373 case _46: 374 return "46"; 375 case _47: 376 return "47"; 377 case _48: 378 return "48"; 379 case NULL: 380 return null; 381 default: 382 return "?"; 383 } 384 } 385 386 public String getSystem() { 387 return "http://terminology.hl7.org/CodeSystem/ex-tooth"; 388 } 389 390 public String getDefinition() { 391 switch (this) { 392 case _0: 393 return "Oral cavity."; 394 case _1: 395 return "Permanent teeth Maxillary right."; 396 case _2: 397 return "Permanent teeth Maxillary left."; 398 case _3: 399 return "Permanent teeth Mandibular right."; 400 case _4: 401 return "Permanent teeth Mandibular left."; 402 case _5: 403 return "Deciduous teeth Maxillary right."; 404 case _6: 405 return "Deciduous teeth Maxillary left."; 406 case _7: 407 return "Deciduous teeth Mandibular right."; 408 case _8: 409 return "Deciduous teeth Mandibular left."; 410 case _11: 411 return "Upper Right Tooth 1 from the central axis, permanent dentition."; 412 case _12: 413 return "Upper Right Tooth 2 from the central axis, permanent dentition."; 414 case _13: 415 return "Upper Right Tooth 3 from the central axis, permanent dentition."; 416 case _14: 417 return "Upper Right Tooth 4 from the central axis, permanent dentition."; 418 case _15: 419 return "Upper Right Tooth 5 from the central axis, permanent dentition."; 420 case _16: 421 return "Upper Right Tooth 6 from the central axis, permanent dentition."; 422 case _17: 423 return "Upper Right Tooth 7 from the central axis, permanent dentition."; 424 case _18: 425 return "Upper Right Tooth 8 from the central axis, permanent dentition."; 426 case _21: 427 return "Upper Left Tooth 1 from the central axis, permanent dentition."; 428 case _22: 429 return "Upper Left Tooth 2 from the central axis, permanent dentition."; 430 case _23: 431 return "Upper Left Tooth 3 from the central axis, permanent dentition."; 432 case _24: 433 return "Upper Left Tooth 4 from the central axis, permanent dentition."; 434 case _25: 435 return "Upper Left Tooth 5 from the central axis, permanent dentition."; 436 case _26: 437 return "Upper Left Tooth 6 from the central axis, permanent dentition."; 438 case _27: 439 return "Upper Left Tooth 7 from the central axis, permanent dentition."; 440 case _28: 441 return "Upper Left Tooth 8 from the central axis, permanent dentition."; 442 case _31: 443 return "Lower Left Tooth 1 from the central axis, permanent dentition."; 444 case _32: 445 return "Lower Left Tooth 2 from the central axis, permanent dentition."; 446 case _33: 447 return "Lower Left Tooth 3 from the central axis, permanent dentition."; 448 case _34: 449 return "Lower Left Tooth 4 from the central axis, permanent dentition."; 450 case _35: 451 return "Lower Left Tooth 5 from the central axis, permanent dentition."; 452 case _36: 453 return "Lower Left Tooth 6 from the central axis, permanent dentition."; 454 case _37: 455 return "Lower Left Tooth 7 from the central axis, permanent dentition."; 456 case _38: 457 return "Lower Left Tooth 8 from the central axis, permanent dentition."; 458 case _41: 459 return "Lower Right Tooth 1 from the central axis, permanent dentition."; 460 case _42: 461 return "Lower Right Tooth 2 from the central axis, permanent dentition."; 462 case _43: 463 return "Lower Right Tooth 3 from the central axis, permanent dentition."; 464 case _44: 465 return "Lower Right Tooth 4 from the central axis, permanent dentition."; 466 case _45: 467 return "Lower Right Tooth 5 from the central axis, permanent dentition."; 468 case _46: 469 return "Lower Right Tooth 6 from the central axis, permanent dentition."; 470 case _47: 471 return "Lower Right Tooth 7 from the central axis, permanent dentition."; 472 case _48: 473 return "Lower Right Tooth 8 from the central axis, permanent dentition."; 474 case NULL: 475 return null; 476 default: 477 return "?"; 478 } 479 } 480 481 public String getDisplay() { 482 switch (this) { 483 case _0: 484 return "Oral cavity"; 485 case _1: 486 return "1"; 487 case _2: 488 return "2"; 489 case _3: 490 return "3"; 491 case _4: 492 return "4"; 493 case _5: 494 return "5"; 495 case _6: 496 return "6"; 497 case _7: 498 return "7"; 499 case _8: 500 return "8"; 501 case _11: 502 return "11"; 503 case _12: 504 return "12"; 505 case _13: 506 return "13"; 507 case _14: 508 return "14"; 509 case _15: 510 return "15"; 511 case _16: 512 return "16"; 513 case _17: 514 return "17"; 515 case _18: 516 return "18"; 517 case _21: 518 return "21"; 519 case _22: 520 return "22"; 521 case _23: 522 return "23"; 523 case _24: 524 return "24"; 525 case _25: 526 return "25"; 527 case _26: 528 return "26"; 529 case _27: 530 return "27"; 531 case _28: 532 return "28"; 533 case _31: 534 return "31"; 535 case _32: 536 return "32"; 537 case _33: 538 return "33"; 539 case _34: 540 return "34"; 541 case _35: 542 return "35"; 543 case _36: 544 return "36"; 545 case _37: 546 return "37"; 547 case _38: 548 return "38"; 549 case _41: 550 return "41"; 551 case _42: 552 return "42"; 553 case _43: 554 return "43"; 555 case _44: 556 return "44"; 557 case _45: 558 return "45"; 559 case _46: 560 return "46"; 561 case _47: 562 return "47"; 563 case _48: 564 return "48"; 565 case NULL: 566 return null; 567 default: 568 return "?"; 569 } 570 } 571 572}