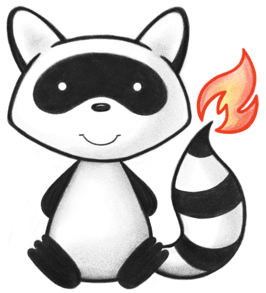
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum V3ActRelationshipSubset { 037 038 /** 039 * Used to indicate that the participation is a filtered subset of the total 040 * participations of the same type owned by the Act. 041 * 042 * Used when there is a need to limit the participations to the first, the last, 043 * the next or some other filtered subset. 044 */ 045 _PARTICIPATIONSUBSET, 046 /** 047 * An occurrence that is scheduled to occur in the future. An Act whose 048 * effective time is greater than 'now', where 'now' is the time the instance is 049 * authored. 050 */ 051 FUTURE, 052 /** 053 * Represents a 'summary' of all acts that are scheduled to occur in the future 054 * (whose effective time is greater than 'now' where is the time the instance is 055 * authored.). The effectiveTime represents the outer boundary of all 056 * occurrences, repeatNumber represents the total number of repetitions, etc. 057 */ 058 FUTSUM, 059 /** 060 * Restricted to the latest known occurrence that is scheduled to occur. The Act 061 * with the highest known effective time. 062 */ 063 LAST, 064 /** 065 * Restricted to the nearest recent known occurrence scheduled to occur in the 066 * future. The Act with the lowest effective time, still greater than 'now'. 067 * ('now' is the time the instance is authored.) 068 */ 069 NEXT, 070 /** 071 * An occurrence that occurred or was scheduled to occur in the past. An Act 072 * whose effective time is less than 'now'. ('now' is the time the instance is 073 * authored.) 074 */ 075 PAST, 076 /** 077 * Restricted to the earliest known occurrence that occurred or was scheduled to 078 * occur in the past. The Act with the lowest effective time. ('now' is the time 079 * the instance is authored.) 080 */ 081 FIRST, 082 /** 083 * Represents a 'summary' of all acts that previously occurred or were scheduled 084 * to occur. The effectiveTime represents the outer boundary of all occurrences, 085 * repeatNumber represents the total number of repetitions, etc. ('now' is the 086 * time the instance is authored.) 087 */ 088 PREVSUM, 089 /** 090 * Restricted to the most recent known occurrence that occurred or was scheduled 091 * to occur in the past. The Act with the most recent effective time, still less 092 * than 'now'. ('now' is the time the instance is authored.) 093 */ 094 RECENT, 095 /** 096 * Represents a 'summary' of all acts that have occurred or were scheduled to 097 * occur and which are scheduled to occur in the future. The effectiveTime 098 * represents the outer boundary of all occurrences, repeatNumber represents the 099 * total number of repetitions, etc. 100 */ 101 SUM, 102 /** 103 * ActRelationshipExpectedSubset 104 */ 105 ACTRELATIONSHIPEXPECTEDSUBSET, 106 /** 107 * ActRelationshipPastSubset 108 */ 109 ACTRELATIONSHIPPASTSUBSET, 110 /** 111 * The occurrence whose value attribute is greater than all other occurrences at 112 * the time the instance is created. 113 */ 114 MAX, 115 /** 116 * The occurrence whose value attribute is less than all other occurrences at 117 * the time the instance is created. 118 */ 119 MIN, 120 /** 121 * added to help the parsers 122 */ 123 NULL; 124 125 public static V3ActRelationshipSubset fromCode(String codeString) throws FHIRException { 126 if (codeString == null || "".equals(codeString)) 127 return null; 128 if ("_ParticipationSubset".equals(codeString)) 129 return _PARTICIPATIONSUBSET; 130 if ("FUTURE".equals(codeString)) 131 return FUTURE; 132 if ("FUTSUM".equals(codeString)) 133 return FUTSUM; 134 if ("LAST".equals(codeString)) 135 return LAST; 136 if ("NEXT".equals(codeString)) 137 return NEXT; 138 if ("PAST".equals(codeString)) 139 return PAST; 140 if ("FIRST".equals(codeString)) 141 return FIRST; 142 if ("PREVSUM".equals(codeString)) 143 return PREVSUM; 144 if ("RECENT".equals(codeString)) 145 return RECENT; 146 if ("SUM".equals(codeString)) 147 return SUM; 148 if ("ActRelationshipExpectedSubset".equals(codeString)) 149 return ACTRELATIONSHIPEXPECTEDSUBSET; 150 if ("ActRelationshipPastSubset".equals(codeString)) 151 return ACTRELATIONSHIPPASTSUBSET; 152 if ("MAX".equals(codeString)) 153 return MAX; 154 if ("MIN".equals(codeString)) 155 return MIN; 156 throw new FHIRException("Unknown V3ActRelationshipSubset code '" + codeString + "'"); 157 } 158 159 public String toCode() { 160 switch (this) { 161 case _PARTICIPATIONSUBSET: 162 return "_ParticipationSubset"; 163 case FUTURE: 164 return "FUTURE"; 165 case FUTSUM: 166 return "FUTSUM"; 167 case LAST: 168 return "LAST"; 169 case NEXT: 170 return "NEXT"; 171 case PAST: 172 return "PAST"; 173 case FIRST: 174 return "FIRST"; 175 case PREVSUM: 176 return "PREVSUM"; 177 case RECENT: 178 return "RECENT"; 179 case SUM: 180 return "SUM"; 181 case ACTRELATIONSHIPEXPECTEDSUBSET: 182 return "ActRelationshipExpectedSubset"; 183 case ACTRELATIONSHIPPASTSUBSET: 184 return "ActRelationshipPastSubset"; 185 case MAX: 186 return "MAX"; 187 case MIN: 188 return "MIN"; 189 case NULL: 190 return null; 191 default: 192 return "?"; 193 } 194 } 195 196 public String getSystem() { 197 return "http://terminology.hl7.org/CodeSystem/v3-ActRelationshipSubset"; 198 } 199 200 public String getDefinition() { 201 switch (this) { 202 case _PARTICIPATIONSUBSET: 203 return "Used to indicate that the participation is a filtered subset of the total participations of the same type owned by the Act. \r\n\n Used when there is a need to limit the participations to the first, the last, the next or some other filtered subset."; 204 case FUTURE: 205 return "An occurrence that is scheduled to occur in the future. An Act whose effective time is greater than 'now', where 'now' is the time the instance is authored."; 206 case FUTSUM: 207 return "Represents a 'summary' of all acts that are scheduled to occur in the future (whose effective time is greater than 'now' where is the time the instance is authored.). The effectiveTime represents the outer boundary of all occurrences, repeatNumber represents the total number of repetitions, etc."; 208 case LAST: 209 return "Restricted to the latest known occurrence that is scheduled to occur. The Act with the highest known effective time."; 210 case NEXT: 211 return "Restricted to the nearest recent known occurrence scheduled to occur in the future. The Act with the lowest effective time, still greater than 'now'. ('now' is the time the instance is authored.)"; 212 case PAST: 213 return "An occurrence that occurred or was scheduled to occur in the past. An Act whose effective time is less than 'now'. ('now' is the time the instance is authored.)"; 214 case FIRST: 215 return "Restricted to the earliest known occurrence that occurred or was scheduled to occur in the past. The Act with the lowest effective time. ('now' is the time the instance is authored.)"; 216 case PREVSUM: 217 return "Represents a 'summary' of all acts that previously occurred or were scheduled to occur. The effectiveTime represents the outer boundary of all occurrences, repeatNumber represents the total number of repetitions, etc. ('now' is the time the instance is authored.)"; 218 case RECENT: 219 return "Restricted to the most recent known occurrence that occurred or was scheduled to occur in the past. The Act with the most recent effective time, still less than 'now'. ('now' is the time the instance is authored.)"; 220 case SUM: 221 return "Represents a 'summary' of all acts that have occurred or were scheduled to occur and which are scheduled to occur in the future. The effectiveTime represents the outer boundary of all occurrences, repeatNumber represents the total number of repetitions, etc."; 222 case ACTRELATIONSHIPEXPECTEDSUBSET: 223 return "ActRelationshipExpectedSubset"; 224 case ACTRELATIONSHIPPASTSUBSET: 225 return "ActRelationshipPastSubset"; 226 case MAX: 227 return "The occurrence whose value attribute is greater than all other occurrences at the time the instance is created."; 228 case MIN: 229 return "The occurrence whose value attribute is less than all other occurrences at the time the instance is created."; 230 case NULL: 231 return null; 232 default: 233 return "?"; 234 } 235 } 236 237 public String getDisplay() { 238 switch (this) { 239 case _PARTICIPATIONSUBSET: 240 return "ParticipationSubset"; 241 case FUTURE: 242 return "expected future"; 243 case FUTSUM: 244 return "future summary"; 245 case LAST: 246 return "expected last"; 247 case NEXT: 248 return "expected next"; 249 case PAST: 250 return "previous"; 251 case FIRST: 252 return "first known"; 253 case PREVSUM: 254 return "previous summary"; 255 case RECENT: 256 return "most recent"; 257 case SUM: 258 return "summary"; 259 case ACTRELATIONSHIPEXPECTEDSUBSET: 260 return "ActRelationshipExpectedSubset"; 261 case ACTRELATIONSHIPPASTSUBSET: 262 return "ActRelationshipPastSubset"; 263 case MAX: 264 return "maximum"; 265 case MIN: 266 return "minimum"; 267 case NULL: 268 return null; 269 default: 270 return "?"; 271 } 272 } 273 274}