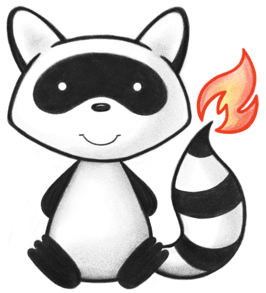
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum V3AddressUse { 037 038 /** 039 * Description: Address uses that can apply to both postal and telecommunication 040 * addresses. 041 */ 042 _GENERALADDRESSUSE, 043 /** 044 * Description: A flag indicating that the address is bad, in fact, useless. 045 */ 046 BAD, 047 /** 048 * Description: Indicates that the address is considered sensitive and should 049 * only be shared or published in accordance with organizational controls 050 * governing patient demographic information with increased sensitivity. Uses of 051 * Addresses. Lloyd to supply more complete description. 052 */ 053 CONF, 054 /** 055 * Description: A communication address at a home, attempted contacts for 056 * business purposes might intrude privacy and chances are one will contact 057 * family or other household members instead of the person one wishes to call. 058 * Typically used with urgent cases, or if no other contacts are available. 059 */ 060 H, 061 /** 062 * Description: The primary home, to reach a person after business hours. 063 */ 064 HP, 065 /** 066 * Description: A vacation home, to reach a person while on vacation. 067 */ 068 HV, 069 /** 070 * This address is no longer in use. 071 * 072 * 073 * Usage Note: Address may also carry valid time ranges. This code is used to 074 * cover the situations where it is known that the address is no longer valid, 075 * but no particular time range for its use is known. 076 */ 077 OLD, 078 /** 079 * Description: A temporary address, may be good for visit or mailing. Note that 080 * an address history can provide more detailed information. 081 */ 082 TMP, 083 /** 084 * Description: An office address. First choice for business related contacts 085 * during business hours. 086 */ 087 WP, 088 /** 089 * Description: Indicates a work place address or telecommunication address that 090 * reaches the individual or organization directly without intermediaries. For 091 * phones, often referred to as a 'private line'. 092 */ 093 DIR, 094 /** 095 * Description: Indicates a work place address or telecommunication address that 096 * is a 'standard' address which may reach a reception service, mail-room, or 097 * other intermediary prior to the target entity. 098 */ 099 PUB, 100 /** 101 * Description: Address uses that only apply to postal addresses, not 102 * telecommunication addresses. 103 */ 104 _POSTALADDRESSUSE, 105 /** 106 * Description: Used primarily to visit an address. 107 */ 108 PHYS, 109 /** 110 * Description: Used to send mail. 111 */ 112 PST, 113 /** 114 * Description: Address uses that only apply to telecommunication addresses, not 115 * postal addresses. 116 */ 117 _TELECOMMUNICATIONADDRESSUSE, 118 /** 119 * Description: An automated answering machine used for less urgent cases and if 120 * the main purpose of contact is to leave a message or access an automated 121 * announcement. 122 */ 123 AS, 124 /** 125 * Description: A contact specifically designated to be used for emergencies. 126 * This is the first choice in emergencies, independent of any other use codes. 127 */ 128 EC, 129 /** 130 * Description: A telecommunication device that moves and stays with its owner. 131 * May have characteristics of all other use codes, suitable for urgent matters, 132 * not the first choice for routine business. 133 */ 134 MC, 135 /** 136 * Description: A paging device suitable to solicit a callback or to leave a 137 * very short message. 138 */ 139 PG, 140 /** 141 * added to help the parsers 142 */ 143 NULL; 144 145 public static V3AddressUse fromCode(String codeString) throws FHIRException { 146 if (codeString == null || "".equals(codeString)) 147 return null; 148 if ("_GeneralAddressUse".equals(codeString)) 149 return _GENERALADDRESSUSE; 150 if ("BAD".equals(codeString)) 151 return BAD; 152 if ("CONF".equals(codeString)) 153 return CONF; 154 if ("H".equals(codeString)) 155 return H; 156 if ("HP".equals(codeString)) 157 return HP; 158 if ("HV".equals(codeString)) 159 return HV; 160 if ("OLD".equals(codeString)) 161 return OLD; 162 if ("TMP".equals(codeString)) 163 return TMP; 164 if ("WP".equals(codeString)) 165 return WP; 166 if ("DIR".equals(codeString)) 167 return DIR; 168 if ("PUB".equals(codeString)) 169 return PUB; 170 if ("_PostalAddressUse".equals(codeString)) 171 return _POSTALADDRESSUSE; 172 if ("PHYS".equals(codeString)) 173 return PHYS; 174 if ("PST".equals(codeString)) 175 return PST; 176 if ("_TelecommunicationAddressUse".equals(codeString)) 177 return _TELECOMMUNICATIONADDRESSUSE; 178 if ("AS".equals(codeString)) 179 return AS; 180 if ("EC".equals(codeString)) 181 return EC; 182 if ("MC".equals(codeString)) 183 return MC; 184 if ("PG".equals(codeString)) 185 return PG; 186 throw new FHIRException("Unknown V3AddressUse code '" + codeString + "'"); 187 } 188 189 public String toCode() { 190 switch (this) { 191 case _GENERALADDRESSUSE: 192 return "_GeneralAddressUse"; 193 case BAD: 194 return "BAD"; 195 case CONF: 196 return "CONF"; 197 case H: 198 return "H"; 199 case HP: 200 return "HP"; 201 case HV: 202 return "HV"; 203 case OLD: 204 return "OLD"; 205 case TMP: 206 return "TMP"; 207 case WP: 208 return "WP"; 209 case DIR: 210 return "DIR"; 211 case PUB: 212 return "PUB"; 213 case _POSTALADDRESSUSE: 214 return "_PostalAddressUse"; 215 case PHYS: 216 return "PHYS"; 217 case PST: 218 return "PST"; 219 case _TELECOMMUNICATIONADDRESSUSE: 220 return "_TelecommunicationAddressUse"; 221 case AS: 222 return "AS"; 223 case EC: 224 return "EC"; 225 case MC: 226 return "MC"; 227 case PG: 228 return "PG"; 229 case NULL: 230 return null; 231 default: 232 return "?"; 233 } 234 } 235 236 public String getSystem() { 237 return "http://terminology.hl7.org/CodeSystem/v3-AddressUse"; 238 } 239 240 public String getDefinition() { 241 switch (this) { 242 case _GENERALADDRESSUSE: 243 return "Description: Address uses that can apply to both postal and telecommunication addresses."; 244 case BAD: 245 return "Description: A flag indicating that the address is bad, in fact, useless."; 246 case CONF: 247 return "Description: Indicates that the address is considered sensitive and should only be shared or published in accordance with organizational controls governing patient demographic information with increased sensitivity. Uses of Addresses. Lloyd to supply more complete description."; 248 case H: 249 return "Description: A communication address at a home, attempted contacts for business purposes might intrude privacy and chances are one will contact family or other household members instead of the person one wishes to call. Typically used with urgent cases, or if no other contacts are available."; 250 case HP: 251 return "Description: The primary home, to reach a person after business hours."; 252 case HV: 253 return "Description: A vacation home, to reach a person while on vacation."; 254 case OLD: 255 return "This address is no longer in use.\r\n\n \n Usage Note: Address may also carry valid time ranges. This code is used to cover the situations where it is known that the address is no longer valid, but no particular time range for its use is known."; 256 case TMP: 257 return "Description: A temporary address, may be good for visit or mailing. Note that an address history can provide more detailed information."; 258 case WP: 259 return "Description: An office address. First choice for business related contacts during business hours."; 260 case DIR: 261 return "Description: Indicates a work place address or telecommunication address that reaches the individual or organization directly without intermediaries. For phones, often referred to as a 'private line'."; 262 case PUB: 263 return "Description: Indicates a work place address or telecommunication address that is a 'standard' address which may reach a reception service, mail-room, or other intermediary prior to the target entity."; 264 case _POSTALADDRESSUSE: 265 return "Description: Address uses that only apply to postal addresses, not telecommunication addresses."; 266 case PHYS: 267 return "Description: Used primarily to visit an address."; 268 case PST: 269 return "Description: Used to send mail."; 270 case _TELECOMMUNICATIONADDRESSUSE: 271 return "Description: Address uses that only apply to telecommunication addresses, not postal addresses."; 272 case AS: 273 return "Description: An automated answering machine used for less urgent cases and if the main purpose of contact is to leave a message or access an automated announcement."; 274 case EC: 275 return "Description: A contact specifically designated to be used for emergencies. This is the first choice in emergencies, independent of any other use codes."; 276 case MC: 277 return "Description: A telecommunication device that moves and stays with its owner. May have characteristics of all other use codes, suitable for urgent matters, not the first choice for routine business."; 278 case PG: 279 return "Description: A paging device suitable to solicit a callback or to leave a very short message."; 280 case NULL: 281 return null; 282 default: 283 return "?"; 284 } 285 } 286 287 public String getDisplay() { 288 switch (this) { 289 case _GENERALADDRESSUSE: 290 return "_GeneralAddressUse"; 291 case BAD: 292 return "bad address"; 293 case CONF: 294 return "confidential address"; 295 case H: 296 return "home address"; 297 case HP: 298 return "primary home"; 299 case HV: 300 return "vacation home"; 301 case OLD: 302 return "no longer in use"; 303 case TMP: 304 return "temporary address"; 305 case WP: 306 return "work place"; 307 case DIR: 308 return "direct"; 309 case PUB: 310 return "public"; 311 case _POSTALADDRESSUSE: 312 return "_PostalAddressUse"; 313 case PHYS: 314 return "physical visit address"; 315 case PST: 316 return "postal address"; 317 case _TELECOMMUNICATIONADDRESSUSE: 318 return "_TelecommunicationAddressUse"; 319 case AS: 320 return "answering service"; 321 case EC: 322 return "emergency contact"; 323 case MC: 324 return "mobile contact)"; 325 case PG: 326 return "pager"; 327 case NULL: 328 return null; 329 default: 330 return "?"; 331 } 332 } 333 334}