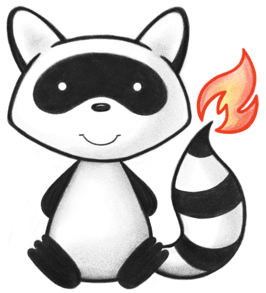
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum V3CalendarCycle { 037 038 /** 039 * CalendarCycleOneLetter 040 */ 041 _CALENDARCYCLEONELETTER, 042 /** 043 * week (continuous) 044 */ 045 CW, 046 /** 047 * year 048 */ 049 CY, 050 /** 051 * day of the month 052 */ 053 D, 054 /** 055 * day of the week (begins with Monday) 056 */ 057 DW, 058 /** 059 * hour of the day 060 */ 061 H, 062 /** 063 * month of the year 064 */ 065 M, 066 /** 067 * minute of the hour 068 */ 069 N, 070 /** 071 * second of the minute 072 */ 073 S, 074 /** 075 * CalendarCycleTwoLetter 076 */ 077 _CALENDARCYCLETWOLETTER, 078 /** 079 * day (continuous) 080 */ 081 CD, 082 /** 083 * hour (continuous) 084 */ 085 CH, 086 /** 087 * month (continuous) 088 */ 089 CM, 090 /** 091 * minute (continuous) 092 */ 093 CN, 094 /** 095 * second (continuous) 096 */ 097 CS, 098 /** 099 * day of the year 100 */ 101 DY, 102 /** 103 * week of the year 104 */ 105 WY, 106 /** 107 * The week with the month's first Thursday in it (analagous to the ISO 8601 108 * definition for week of the year). 109 */ 110 WM, 111 /** 112 * added to help the parsers 113 */ 114 NULL; 115 116 public static V3CalendarCycle fromCode(String codeString) throws FHIRException { 117 if (codeString == null || "".equals(codeString)) 118 return null; 119 if ("_CalendarCycleOneLetter".equals(codeString)) 120 return _CALENDARCYCLEONELETTER; 121 if ("CW".equals(codeString)) 122 return CW; 123 if ("CY".equals(codeString)) 124 return CY; 125 if ("D".equals(codeString)) 126 return D; 127 if ("DW".equals(codeString)) 128 return DW; 129 if ("H".equals(codeString)) 130 return H; 131 if ("M".equals(codeString)) 132 return M; 133 if ("N".equals(codeString)) 134 return N; 135 if ("S".equals(codeString)) 136 return S; 137 if ("_CalendarCycleTwoLetter".equals(codeString)) 138 return _CALENDARCYCLETWOLETTER; 139 if ("CD".equals(codeString)) 140 return CD; 141 if ("CH".equals(codeString)) 142 return CH; 143 if ("CM".equals(codeString)) 144 return CM; 145 if ("CN".equals(codeString)) 146 return CN; 147 if ("CS".equals(codeString)) 148 return CS; 149 if ("DY".equals(codeString)) 150 return DY; 151 if ("WY".equals(codeString)) 152 return WY; 153 if ("WM".equals(codeString)) 154 return WM; 155 throw new FHIRException("Unknown V3CalendarCycle code '" + codeString + "'"); 156 } 157 158 public String toCode() { 159 switch (this) { 160 case _CALENDARCYCLEONELETTER: 161 return "_CalendarCycleOneLetter"; 162 case CW: 163 return "CW"; 164 case CY: 165 return "CY"; 166 case D: 167 return "D"; 168 case DW: 169 return "DW"; 170 case H: 171 return "H"; 172 case M: 173 return "M"; 174 case N: 175 return "N"; 176 case S: 177 return "S"; 178 case _CALENDARCYCLETWOLETTER: 179 return "_CalendarCycleTwoLetter"; 180 case CD: 181 return "CD"; 182 case CH: 183 return "CH"; 184 case CM: 185 return "CM"; 186 case CN: 187 return "CN"; 188 case CS: 189 return "CS"; 190 case DY: 191 return "DY"; 192 case WY: 193 return "WY"; 194 case WM: 195 return "WM"; 196 case NULL: 197 return null; 198 default: 199 return "?"; 200 } 201 } 202 203 public String getSystem() { 204 return "http://terminology.hl7.org/CodeSystem/v3-CalendarCycle"; 205 } 206 207 public String getDefinition() { 208 switch (this) { 209 case _CALENDARCYCLEONELETTER: 210 return "CalendarCycleOneLetter"; 211 case CW: 212 return "week (continuous)"; 213 case CY: 214 return "year"; 215 case D: 216 return "day of the month"; 217 case DW: 218 return "day of the week (begins with Monday)"; 219 case H: 220 return "hour of the day"; 221 case M: 222 return "month of the year"; 223 case N: 224 return "minute of the hour"; 225 case S: 226 return "second of the minute"; 227 case _CALENDARCYCLETWOLETTER: 228 return "CalendarCycleTwoLetter"; 229 case CD: 230 return "day (continuous)"; 231 case CH: 232 return "hour (continuous)"; 233 case CM: 234 return "month (continuous)"; 235 case CN: 236 return "minute (continuous)"; 237 case CS: 238 return "second (continuous)"; 239 case DY: 240 return "day of the year"; 241 case WY: 242 return "week of the year"; 243 case WM: 244 return "The week with the month's first Thursday in it (analagous to the ISO 8601 definition for week of the year)."; 245 case NULL: 246 return null; 247 default: 248 return "?"; 249 } 250 } 251 252 public String getDisplay() { 253 switch (this) { 254 case _CALENDARCYCLEONELETTER: 255 return "CalendarCycleOneLetter"; 256 case CW: 257 return "week (continuous)"; 258 case CY: 259 return "year"; 260 case D: 261 return "day of the month"; 262 case DW: 263 return "day of the week (begins with Monday)"; 264 case H: 265 return "hour of the day"; 266 case M: 267 return "month of the year"; 268 case N: 269 return "minute of the hour"; 270 case S: 271 return "second of the minute"; 272 case _CALENDARCYCLETWOLETTER: 273 return "CalendarCycleTwoLetter"; 274 case CD: 275 return "day (continuous)"; 276 case CH: 277 return "hour (continuous)"; 278 case CM: 279 return "month (continuous)"; 280 case CN: 281 return "minute (continuous)"; 282 case CS: 283 return "second (continuous)"; 284 case DY: 285 return "day of the year"; 286 case WY: 287 return "week of the year"; 288 case WM: 289 return "week of the month"; 290 case NULL: 291 return null; 292 default: 293 return "?"; 294 } 295 } 296 297}