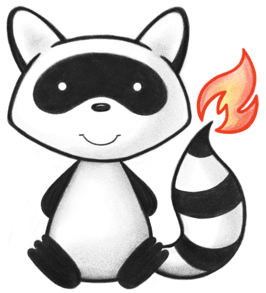
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum V3ContextControl { 037 038 /** 039 * The association adds to the existing context associated with the Act. Both 040 * this association and any associations propagated from ancestor Acts are 041 * interpreted as being related to this Act. 042 */ 043 _CONTEXTCONTROLADDITIVE, 044 /** 045 * The association adds to the existing context associated with the Act, but 046 * will not propagate to any descendant Acts reached by conducting 047 * ActRelationships (see contextControlCode). Examples: If an 'Author' 048 * Participation were marked as "Additive, Non-Propagating" it means that the 049 * author will be added to the set of author participations that have propagated 050 * from ancestor Acts for the purpose of this Act. However only the previously 051 * propagated authors will propagate to any child Acts that allow context to be 052 * propagated. 053 */ 054 AN, 055 /** 056 * The association adds to the existing context associated with the Act, and 057 * will propagate to any descendant Acts reached by conducting ActRelationships 058 * (see contextControlCode). Examples: If an 'Author' Participation were marked 059 * as "Additive, Propagating" it means that the author will be added to the set 060 * of author participations that have propagated from ancestor Acts, and will 061 * itself propagate with the other authors to any child Acts that allow context 062 * to be propagated. 063 */ 064 AP, 065 /** 066 * The association applies only to the current Act and will not propagate to any 067 * child Acts that are related via a conducting ActRelationship (refer to 068 * contextConductionInd). 069 */ 070 _CONTEXTCONTROLNONPROPAGATING, 071 /** 072 * The association is added to the existing context associated with the Act, but 073 * overrides an association with the same typeCode. However, this overriding 074 * association will not propagate to any descendant Acts reached by conducting 075 * ActRelationships (see contextControlCode). Examples: If an 'Author' 076 * Participation were marked as "Overriding, Non-Propagating" it means that the 077 * author will replace the set of author participations that have propagated 078 * from ancestor Acts. Furthermore, no author participations whatsoever will 079 * propagate to any child Acts that allow context to be propagated. 080 */ 081 ON, 082 /** 083 * The association adds to the existing context associated with the Act, but 084 * replaces associations propagated from ancestor Acts whose typeCodes are the 085 * same or more specific. 086 */ 087 _CONTEXTCONTROLOVERRIDING, 088 /** 089 * The association is added to the existing context associated with the Act, but 090 * overrides an association with the same typeCode. This overriding association 091 * will propagate to any descendant Acts reached by conducting ActRelationships 092 * (see contextControlCode). Examples: If an 'Author' Participation were marked 093 * as "Overriding, Propagating" it means that the author will replace the set of 094 * author participations that have propagated from ancestor Acts, and will 095 * itself be the only author to propagate to any child Acts that allow context 096 * to be propagated. 097 */ 098 OP, 099 /** 100 * The association propagates to any child Acts that are related via a 101 * conducting ActRelationship (refer to contextConductionInd). 102 */ 103 _CONTEXTCONTROLPROPAGATING, 104 /** 105 * added to help the parsers 106 */ 107 NULL; 108 109 public static V3ContextControl fromCode(String codeString) throws FHIRException { 110 if (codeString == null || "".equals(codeString)) 111 return null; 112 if ("_ContextControlAdditive".equals(codeString)) 113 return _CONTEXTCONTROLADDITIVE; 114 if ("AN".equals(codeString)) 115 return AN; 116 if ("AP".equals(codeString)) 117 return AP; 118 if ("_ContextControlNonPropagating".equals(codeString)) 119 return _CONTEXTCONTROLNONPROPAGATING; 120 if ("ON".equals(codeString)) 121 return ON; 122 if ("_ContextControlOverriding".equals(codeString)) 123 return _CONTEXTCONTROLOVERRIDING; 124 if ("OP".equals(codeString)) 125 return OP; 126 if ("_ContextControlPropagating".equals(codeString)) 127 return _CONTEXTCONTROLPROPAGATING; 128 throw new FHIRException("Unknown V3ContextControl code '" + codeString + "'"); 129 } 130 131 public String toCode() { 132 switch (this) { 133 case _CONTEXTCONTROLADDITIVE: 134 return "_ContextControlAdditive"; 135 case AN: 136 return "AN"; 137 case AP: 138 return "AP"; 139 case _CONTEXTCONTROLNONPROPAGATING: 140 return "_ContextControlNonPropagating"; 141 case ON: 142 return "ON"; 143 case _CONTEXTCONTROLOVERRIDING: 144 return "_ContextControlOverriding"; 145 case OP: 146 return "OP"; 147 case _CONTEXTCONTROLPROPAGATING: 148 return "_ContextControlPropagating"; 149 case NULL: 150 return null; 151 default: 152 return "?"; 153 } 154 } 155 156 public String getSystem() { 157 return "http://terminology.hl7.org/CodeSystem/v3-ContextControl"; 158 } 159 160 public String getDefinition() { 161 switch (this) { 162 case _CONTEXTCONTROLADDITIVE: 163 return "The association adds to the existing context associated with the Act. Both this association and any associations propagated from ancestor Acts are interpreted as being related to this Act."; 164 case AN: 165 return "The association adds to the existing context associated with the Act, but will not propagate to any descendant Acts reached by conducting ActRelationships (see contextControlCode). Examples: If an 'Author' Participation were marked as \"Additive, Non-Propagating\" it means that the author will be added to the set of author participations that have propagated from ancestor Acts for the purpose of this Act. However only the previously propagated authors will propagate to any child Acts that allow context to be propagated."; 166 case AP: 167 return "The association adds to the existing context associated with the Act, and will propagate to any descendant Acts reached by conducting ActRelationships (see contextControlCode). Examples: If an 'Author' Participation were marked as \"Additive, Propagating\" it means that the author will be added to the set of author participations that have propagated from ancestor Acts, and will itself propagate with the other authors to any child Acts that allow context to be propagated."; 168 case _CONTEXTCONTROLNONPROPAGATING: 169 return "The association applies only to the current Act and will not propagate to any child Acts that are related via a conducting ActRelationship (refer to contextConductionInd)."; 170 case ON: 171 return "The association is added to the existing context associated with the Act, but overrides an association with the same typeCode. However, this overriding association will not propagate to any descendant Acts reached by conducting ActRelationships (see contextControlCode). Examples: If an 'Author' Participation were marked as \"Overriding, Non-Propagating\" it means that the author will replace the set of author participations that have propagated from ancestor Acts. Furthermore, no author participations whatsoever will propagate to any child Acts that allow context to be propagated."; 172 case _CONTEXTCONTROLOVERRIDING: 173 return "The association adds to the existing context associated with the Act, but replaces associations propagated from ancestor Acts whose typeCodes are the same or more specific."; 174 case OP: 175 return "The association is added to the existing context associated with the Act, but overrides an association with the same typeCode. This overriding association will propagate to any descendant Acts reached by conducting ActRelationships (see contextControlCode). Examples: If an 'Author' Participation were marked as \"Overriding, Propagating\" it means that the author will replace the set of author participations that have propagated from ancestor Acts, and will itself be the only author to propagate to any child Acts that allow context to be propagated."; 176 case _CONTEXTCONTROLPROPAGATING: 177 return "The association propagates to any child Acts that are related via a conducting ActRelationship (refer to contextConductionInd)."; 178 case NULL: 179 return null; 180 default: 181 return "?"; 182 } 183 } 184 185 public String getDisplay() { 186 switch (this) { 187 case _CONTEXTCONTROLADDITIVE: 188 return "ContextControlAdditive"; 189 case AN: 190 return "additive, non-propagating"; 191 case AP: 192 return "additive, propagating"; 193 case _CONTEXTCONTROLNONPROPAGATING: 194 return "ContextControlNonPropagating"; 195 case ON: 196 return "overriding, non-propagating"; 197 case _CONTEXTCONTROLOVERRIDING: 198 return "ContextControlOverriding"; 199 case OP: 200 return "overriding, propagating"; 201 case _CONTEXTCONTROLPROPAGATING: 202 return "ContextControlPropagating"; 203 case NULL: 204 return null; 205 default: 206 return "?"; 207 } 208 } 209 210}