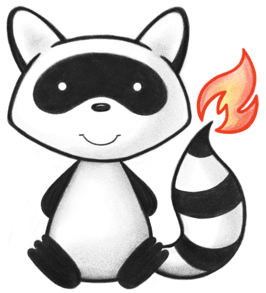
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum V3EducationLevel { 037 038 /** 039 * Associate's or technical degree complete 040 */ 041 ASSOC, 042 /** 043 * College or baccalaureate degree complete 044 */ 045 BD, 046 /** 047 * Elementary School 048 */ 049 ELEM, 050 /** 051 * Graduate or professional Degree complete 052 */ 053 GD, 054 /** 055 * High School or secondary school degree complete 056 */ 057 HS, 058 /** 059 * Some post-baccalaureate education 060 */ 061 PB, 062 /** 063 * Doctoral or post graduate education 064 */ 065 POSTG, 066 /** 067 * Some College education 068 */ 069 SCOL, 070 /** 071 * Some secondary or high school education 072 */ 073 SEC, 074 /** 075 * added to help the parsers 076 */ 077 NULL; 078 079 public static V3EducationLevel fromCode(String codeString) throws FHIRException { 080 if (codeString == null || "".equals(codeString)) 081 return null; 082 if ("ASSOC".equals(codeString)) 083 return ASSOC; 084 if ("BD".equals(codeString)) 085 return BD; 086 if ("ELEM".equals(codeString)) 087 return ELEM; 088 if ("GD".equals(codeString)) 089 return GD; 090 if ("HS".equals(codeString)) 091 return HS; 092 if ("PB".equals(codeString)) 093 return PB; 094 if ("POSTG".equals(codeString)) 095 return POSTG; 096 if ("SCOL".equals(codeString)) 097 return SCOL; 098 if ("SEC".equals(codeString)) 099 return SEC; 100 throw new FHIRException("Unknown V3EducationLevel code '" + codeString + "'"); 101 } 102 103 public String toCode() { 104 switch (this) { 105 case ASSOC: 106 return "ASSOC"; 107 case BD: 108 return "BD"; 109 case ELEM: 110 return "ELEM"; 111 case GD: 112 return "GD"; 113 case HS: 114 return "HS"; 115 case PB: 116 return "PB"; 117 case POSTG: 118 return "POSTG"; 119 case SCOL: 120 return "SCOL"; 121 case SEC: 122 return "SEC"; 123 case NULL: 124 return null; 125 default: 126 return "?"; 127 } 128 } 129 130 public String getSystem() { 131 return "http://terminology.hl7.org/CodeSystem/v3-EducationLevel"; 132 } 133 134 public String getDefinition() { 135 switch (this) { 136 case ASSOC: 137 return "Associate's or technical degree complete"; 138 case BD: 139 return "College or baccalaureate degree complete"; 140 case ELEM: 141 return "Elementary School"; 142 case GD: 143 return "Graduate or professional Degree complete"; 144 case HS: 145 return "High School or secondary school degree complete"; 146 case PB: 147 return "Some post-baccalaureate education"; 148 case POSTG: 149 return "Doctoral or post graduate education"; 150 case SCOL: 151 return "Some College education"; 152 case SEC: 153 return "Some secondary or high school education"; 154 case NULL: 155 return null; 156 default: 157 return "?"; 158 } 159 } 160 161 public String getDisplay() { 162 switch (this) { 163 case ASSOC: 164 return "Associate's or technical degree complete"; 165 case BD: 166 return "College or baccalaureate degree complete"; 167 case ELEM: 168 return "Elementary School"; 169 case GD: 170 return "Graduate or professional Degree complete"; 171 case HS: 172 return "High School or secondary school degree complete"; 173 case PB: 174 return "Some post-baccalaureate education"; 175 case POSTG: 176 return "Doctoral or post graduate education"; 177 case SCOL: 178 return "Some College education"; 179 case SEC: 180 return "Some secondary or high school education"; 181 case NULL: 182 return null; 183 default: 184 return "?"; 185 } 186 } 187 188}