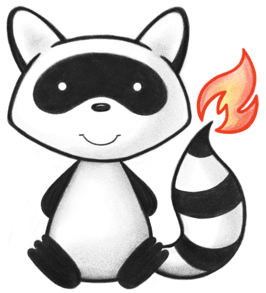
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.r4.model.EnumFactory; 035 036public class V3EntityCodeEnumFactory implements EnumFactory<V3EntityCode> { 037 038 public V3EntityCode fromCode(String codeString) throws IllegalArgumentException { 039 if (codeString == null || "".equals(codeString)) 040 return null; 041 if ("_MaterialEntityClassType".equals(codeString)) 042 return V3EntityCode._MATERIALENTITYCLASSTYPE; 043 if ("_ContainerEntityType".equals(codeString)) 044 return V3EntityCode._CONTAINERENTITYTYPE; 045 if ("PKG".equals(codeString)) 046 return V3EntityCode.PKG; 047 if ("_NonRigidContainerEntityType".equals(codeString)) 048 return V3EntityCode._NONRIGIDCONTAINERENTITYTYPE; 049 if ("BAG".equals(codeString)) 050 return V3EntityCode.BAG; 051 if ("PACKT".equals(codeString)) 052 return V3EntityCode.PACKT; 053 if ("PCH".equals(codeString)) 054 return V3EntityCode.PCH; 055 if ("SACH".equals(codeString)) 056 return V3EntityCode.SACH; 057 if ("_RigidContainerEntityType".equals(codeString)) 058 return V3EntityCode._RIGIDCONTAINERENTITYTYPE; 059 if ("_IndividualPackageEntityType".equals(codeString)) 060 return V3EntityCode._INDIVIDUALPACKAGEENTITYTYPE; 061 if ("AMP".equals(codeString)) 062 return V3EntityCode.AMP; 063 if ("MINIM".equals(codeString)) 064 return V3EntityCode.MINIM; 065 if ("NEBAMP".equals(codeString)) 066 return V3EntityCode.NEBAMP; 067 if ("OVUL".equals(codeString)) 068 return V3EntityCode.OVUL; 069 if ("_MultiUseContainerEntityType".equals(codeString)) 070 return V3EntityCode._MULTIUSECONTAINERENTITYTYPE; 071 if ("BOT".equals(codeString)) 072 return V3EntityCode.BOT; 073 if ("BOTA".equals(codeString)) 074 return V3EntityCode.BOTA; 075 if ("BOTD".equals(codeString)) 076 return V3EntityCode.BOTD; 077 if ("BOTG".equals(codeString)) 078 return V3EntityCode.BOTG; 079 if ("BOTP".equals(codeString)) 080 return V3EntityCode.BOTP; 081 if ("BOTPLY".equals(codeString)) 082 return V3EntityCode.BOTPLY; 083 if ("BOX".equals(codeString)) 084 return V3EntityCode.BOX; 085 if ("CAN".equals(codeString)) 086 return V3EntityCode.CAN; 087 if ("CART".equals(codeString)) 088 return V3EntityCode.CART; 089 if ("CNSTR".equals(codeString)) 090 return V3EntityCode.CNSTR; 091 if ("JAR".equals(codeString)) 092 return V3EntityCode.JAR; 093 if ("JUG".equals(codeString)) 094 return V3EntityCode.JUG; 095 if ("TIN".equals(codeString)) 096 return V3EntityCode.TIN; 097 if ("TUB".equals(codeString)) 098 return V3EntityCode.TUB; 099 if ("TUBE".equals(codeString)) 100 return V3EntityCode.TUBE; 101 if ("VIAL".equals(codeString)) 102 return V3EntityCode.VIAL; 103 if ("BLSTRPK".equals(codeString)) 104 return V3EntityCode.BLSTRPK; 105 if ("CARD".equals(codeString)) 106 return V3EntityCode.CARD; 107 if ("COMPPKG".equals(codeString)) 108 return V3EntityCode.COMPPKG; 109 if ("DIALPK".equals(codeString)) 110 return V3EntityCode.DIALPK; 111 if ("DISK".equals(codeString)) 112 return V3EntityCode.DISK; 113 if ("DOSET".equals(codeString)) 114 return V3EntityCode.DOSET; 115 if ("STRIP".equals(codeString)) 116 return V3EntityCode.STRIP; 117 if ("KIT".equals(codeString)) 118 return V3EntityCode.KIT; 119 if ("SYSTM".equals(codeString)) 120 return V3EntityCode.SYSTM; 121 if ("_MedicalDevice".equals(codeString)) 122 return V3EntityCode._MEDICALDEVICE; 123 if ("_AccessMedicalDevice".equals(codeString)) 124 return V3EntityCode._ACCESSMEDICALDEVICE; 125 if ("LINE".equals(codeString)) 126 return V3EntityCode.LINE; 127 if ("IALINE".equals(codeString)) 128 return V3EntityCode.IALINE; 129 if ("IVLINE".equals(codeString)) 130 return V3EntityCode.IVLINE; 131 if ("_AdministrationMedicalDevice".equals(codeString)) 132 return V3EntityCode._ADMINISTRATIONMEDICALDEVICE; 133 if ("_InjectionMedicalDevice".equals(codeString)) 134 return V3EntityCode._INJECTIONMEDICALDEVICE; 135 if ("AINJ".equals(codeString)) 136 return V3EntityCode.AINJ; 137 if ("PEN".equals(codeString)) 138 return V3EntityCode.PEN; 139 if ("SYR".equals(codeString)) 140 return V3EntityCode.SYR; 141 if ("APLCTR".equals(codeString)) 142 return V3EntityCode.APLCTR; 143 if ("INH".equals(codeString)) 144 return V3EntityCode.INH; 145 if ("DSKS".equals(codeString)) 146 return V3EntityCode.DSKS; 147 if ("DSKUNH".equals(codeString)) 148 return V3EntityCode.DSKUNH; 149 if ("TRBINH".equals(codeString)) 150 return V3EntityCode.TRBINH; 151 if ("PMP".equals(codeString)) 152 return V3EntityCode.PMP; 153 if ("_SpecimenAdditiveEntity".equals(codeString)) 154 return V3EntityCode._SPECIMENADDITIVEENTITY; 155 if ("ACDA".equals(codeString)) 156 return V3EntityCode.ACDA; 157 if ("ACDB".equals(codeString)) 158 return V3EntityCode.ACDB; 159 if ("ACET".equals(codeString)) 160 return V3EntityCode.ACET; 161 if ("AMIES".equals(codeString)) 162 return V3EntityCode.AMIES; 163 if ("BACTM".equals(codeString)) 164 return V3EntityCode.BACTM; 165 if ("BF10".equals(codeString)) 166 return V3EntityCode.BF10; 167 if ("BOR".equals(codeString)) 168 return V3EntityCode.BOR; 169 if ("BOUIN".equals(codeString)) 170 return V3EntityCode.BOUIN; 171 if ("BSKM".equals(codeString)) 172 return V3EntityCode.BSKM; 173 if ("C32".equals(codeString)) 174 return V3EntityCode.C32; 175 if ("C38".equals(codeString)) 176 return V3EntityCode.C38; 177 if ("CARS".equals(codeString)) 178 return V3EntityCode.CARS; 179 if ("CARY".equals(codeString)) 180 return V3EntityCode.CARY; 181 if ("CHLTM".equals(codeString)) 182 return V3EntityCode.CHLTM; 183 if ("CTAD".equals(codeString)) 184 return V3EntityCode.CTAD; 185 if ("EDTK15".equals(codeString)) 186 return V3EntityCode.EDTK15; 187 if ("EDTK75".equals(codeString)) 188 return V3EntityCode.EDTK75; 189 if ("EDTN".equals(codeString)) 190 return V3EntityCode.EDTN; 191 if ("ENT".equals(codeString)) 192 return V3EntityCode.ENT; 193 if ("F10".equals(codeString)) 194 return V3EntityCode.F10; 195 if ("FDP".equals(codeString)) 196 return V3EntityCode.FDP; 197 if ("FL10".equals(codeString)) 198 return V3EntityCode.FL10; 199 if ("FL100".equals(codeString)) 200 return V3EntityCode.FL100; 201 if ("HCL6".equals(codeString)) 202 return V3EntityCode.HCL6; 203 if ("HEPA".equals(codeString)) 204 return V3EntityCode.HEPA; 205 if ("HEPL".equals(codeString)) 206 return V3EntityCode.HEPL; 207 if ("HEPN".equals(codeString)) 208 return V3EntityCode.HEPN; 209 if ("HNO3".equals(codeString)) 210 return V3EntityCode.HNO3; 211 if ("JKM".equals(codeString)) 212 return V3EntityCode.JKM; 213 if ("KARN".equals(codeString)) 214 return V3EntityCode.KARN; 215 if ("KOX".equals(codeString)) 216 return V3EntityCode.KOX; 217 if ("LIA".equals(codeString)) 218 return V3EntityCode.LIA; 219 if ("M4".equals(codeString)) 220 return V3EntityCode.M4; 221 if ("M4RT".equals(codeString)) 222 return V3EntityCode.M4RT; 223 if ("M5".equals(codeString)) 224 return V3EntityCode.M5; 225 if ("MICHTM".equals(codeString)) 226 return V3EntityCode.MICHTM; 227 if ("MMDTM".equals(codeString)) 228 return V3EntityCode.MMDTM; 229 if ("NAF".equals(codeString)) 230 return V3EntityCode.NAF; 231 if ("NONE".equals(codeString)) 232 return V3EntityCode.NONE; 233 if ("PAGE".equals(codeString)) 234 return V3EntityCode.PAGE; 235 if ("PHENOL".equals(codeString)) 236 return V3EntityCode.PHENOL; 237 if ("PVA".equals(codeString)) 238 return V3EntityCode.PVA; 239 if ("RLM".equals(codeString)) 240 return V3EntityCode.RLM; 241 if ("SILICA".equals(codeString)) 242 return V3EntityCode.SILICA; 243 if ("SPS".equals(codeString)) 244 return V3EntityCode.SPS; 245 if ("SST".equals(codeString)) 246 return V3EntityCode.SST; 247 if ("STUTM".equals(codeString)) 248 return V3EntityCode.STUTM; 249 if ("THROM".equals(codeString)) 250 return V3EntityCode.THROM; 251 if ("THYMOL".equals(codeString)) 252 return V3EntityCode.THYMOL; 253 if ("THYO".equals(codeString)) 254 return V3EntityCode.THYO; 255 if ("TOLU".equals(codeString)) 256 return V3EntityCode.TOLU; 257 if ("URETM".equals(codeString)) 258 return V3EntityCode.URETM; 259 if ("VIRTM".equals(codeString)) 260 return V3EntityCode.VIRTM; 261 if ("WEST".equals(codeString)) 262 return V3EntityCode.WEST; 263 if ("BLDPRD".equals(codeString)) 264 return V3EntityCode.BLDPRD; 265 if ("VCCNE".equals(codeString)) 266 return V3EntityCode.VCCNE; 267 if ("_DrugEntity".equals(codeString)) 268 return V3EntityCode._DRUGENTITY; 269 if ("_ClinicalDrug".equals(codeString)) 270 return V3EntityCode._CLINICALDRUG; 271 if ("_NonDrugAgentEntity".equals(codeString)) 272 return V3EntityCode._NONDRUGAGENTENTITY; 273 if ("NDA01".equals(codeString)) 274 return V3EntityCode.NDA01; 275 if ("NDA02".equals(codeString)) 276 return V3EntityCode.NDA02; 277 if ("NDA03".equals(codeString)) 278 return V3EntityCode.NDA03; 279 if ("NDA04".equals(codeString)) 280 return V3EntityCode.NDA04; 281 if ("NDA05".equals(codeString)) 282 return V3EntityCode.NDA05; 283 if ("NDA06".equals(codeString)) 284 return V3EntityCode.NDA06; 285 if ("NDA07".equals(codeString)) 286 return V3EntityCode.NDA07; 287 if ("NDA08".equals(codeString)) 288 return V3EntityCode.NDA08; 289 if ("NDA09".equals(codeString)) 290 return V3EntityCode.NDA09; 291 if ("NDA10".equals(codeString)) 292 return V3EntityCode.NDA10; 293 if ("NDA11".equals(codeString)) 294 return V3EntityCode.NDA11; 295 if ("NDA12".equals(codeString)) 296 return V3EntityCode.NDA12; 297 if ("NDA13".equals(codeString)) 298 return V3EntityCode.NDA13; 299 if ("NDA14".equals(codeString)) 300 return V3EntityCode.NDA14; 301 if ("NDA15".equals(codeString)) 302 return V3EntityCode.NDA15; 303 if ("NDA16".equals(codeString)) 304 return V3EntityCode.NDA16; 305 if ("NDA17".equals(codeString)) 306 return V3EntityCode.NDA17; 307 if ("_OrganizationEntityType".equals(codeString)) 308 return V3EntityCode._ORGANIZATIONENTITYTYPE; 309 if ("HHOLD".equals(codeString)) 310 return V3EntityCode.HHOLD; 311 if ("NAT".equals(codeString)) 312 return V3EntityCode.NAT; 313 if ("RELIG".equals(codeString)) 314 return V3EntityCode.RELIG; 315 if ("_PlaceEntityType".equals(codeString)) 316 return V3EntityCode._PLACEENTITYTYPE; 317 if ("BED".equals(codeString)) 318 return V3EntityCode.BED; 319 if ("BLDG".equals(codeString)) 320 return V3EntityCode.BLDG; 321 if ("FLOOR".equals(codeString)) 322 return V3EntityCode.FLOOR; 323 if ("ROOM".equals(codeString)) 324 return V3EntityCode.ROOM; 325 if ("WING".equals(codeString)) 326 return V3EntityCode.WING; 327 if ("_ResourceGroupEntityType".equals(codeString)) 328 return V3EntityCode._RESOURCEGROUPENTITYTYPE; 329 if ("PRAC".equals(codeString)) 330 return V3EntityCode.PRAC; 331 throw new IllegalArgumentException("Unknown V3EntityCode code '" + codeString + "'"); 332 } 333 334 public String toCode(V3EntityCode code) { 335 if (code == V3EntityCode.NULL) 336 return null; 337 if (code == V3EntityCode._MATERIALENTITYCLASSTYPE) 338 return "_MaterialEntityClassType"; 339 if (code == V3EntityCode._CONTAINERENTITYTYPE) 340 return "_ContainerEntityType"; 341 if (code == V3EntityCode.PKG) 342 return "PKG"; 343 if (code == V3EntityCode._NONRIGIDCONTAINERENTITYTYPE) 344 return "_NonRigidContainerEntityType"; 345 if (code == V3EntityCode.BAG) 346 return "BAG"; 347 if (code == V3EntityCode.PACKT) 348 return "PACKT"; 349 if (code == V3EntityCode.PCH) 350 return "PCH"; 351 if (code == V3EntityCode.SACH) 352 return "SACH"; 353 if (code == V3EntityCode._RIGIDCONTAINERENTITYTYPE) 354 return "_RigidContainerEntityType"; 355 if (code == V3EntityCode._INDIVIDUALPACKAGEENTITYTYPE) 356 return "_IndividualPackageEntityType"; 357 if (code == V3EntityCode.AMP) 358 return "AMP"; 359 if (code == V3EntityCode.MINIM) 360 return "MINIM"; 361 if (code == V3EntityCode.NEBAMP) 362 return "NEBAMP"; 363 if (code == V3EntityCode.OVUL) 364 return "OVUL"; 365 if (code == V3EntityCode._MULTIUSECONTAINERENTITYTYPE) 366 return "_MultiUseContainerEntityType"; 367 if (code == V3EntityCode.BOT) 368 return "BOT"; 369 if (code == V3EntityCode.BOTA) 370 return "BOTA"; 371 if (code == V3EntityCode.BOTD) 372 return "BOTD"; 373 if (code == V3EntityCode.BOTG) 374 return "BOTG"; 375 if (code == V3EntityCode.BOTP) 376 return "BOTP"; 377 if (code == V3EntityCode.BOTPLY) 378 return "BOTPLY"; 379 if (code == V3EntityCode.BOX) 380 return "BOX"; 381 if (code == V3EntityCode.CAN) 382 return "CAN"; 383 if (code == V3EntityCode.CART) 384 return "CART"; 385 if (code == V3EntityCode.CNSTR) 386 return "CNSTR"; 387 if (code == V3EntityCode.JAR) 388 return "JAR"; 389 if (code == V3EntityCode.JUG) 390 return "JUG"; 391 if (code == V3EntityCode.TIN) 392 return "TIN"; 393 if (code == V3EntityCode.TUB) 394 return "TUB"; 395 if (code == V3EntityCode.TUBE) 396 return "TUBE"; 397 if (code == V3EntityCode.VIAL) 398 return "VIAL"; 399 if (code == V3EntityCode.BLSTRPK) 400 return "BLSTRPK"; 401 if (code == V3EntityCode.CARD) 402 return "CARD"; 403 if (code == V3EntityCode.COMPPKG) 404 return "COMPPKG"; 405 if (code == V3EntityCode.DIALPK) 406 return "DIALPK"; 407 if (code == V3EntityCode.DISK) 408 return "DISK"; 409 if (code == V3EntityCode.DOSET) 410 return "DOSET"; 411 if (code == V3EntityCode.STRIP) 412 return "STRIP"; 413 if (code == V3EntityCode.KIT) 414 return "KIT"; 415 if (code == V3EntityCode.SYSTM) 416 return "SYSTM"; 417 if (code == V3EntityCode._MEDICALDEVICE) 418 return "_MedicalDevice"; 419 if (code == V3EntityCode._ACCESSMEDICALDEVICE) 420 return "_AccessMedicalDevice"; 421 if (code == V3EntityCode.LINE) 422 return "LINE"; 423 if (code == V3EntityCode.IALINE) 424 return "IALINE"; 425 if (code == V3EntityCode.IVLINE) 426 return "IVLINE"; 427 if (code == V3EntityCode._ADMINISTRATIONMEDICALDEVICE) 428 return "_AdministrationMedicalDevice"; 429 if (code == V3EntityCode._INJECTIONMEDICALDEVICE) 430 return "_InjectionMedicalDevice"; 431 if (code == V3EntityCode.AINJ) 432 return "AINJ"; 433 if (code == V3EntityCode.PEN) 434 return "PEN"; 435 if (code == V3EntityCode.SYR) 436 return "SYR"; 437 if (code == V3EntityCode.APLCTR) 438 return "APLCTR"; 439 if (code == V3EntityCode.INH) 440 return "INH"; 441 if (code == V3EntityCode.DSKS) 442 return "DSKS"; 443 if (code == V3EntityCode.DSKUNH) 444 return "DSKUNH"; 445 if (code == V3EntityCode.TRBINH) 446 return "TRBINH"; 447 if (code == V3EntityCode.PMP) 448 return "PMP"; 449 if (code == V3EntityCode._SPECIMENADDITIVEENTITY) 450 return "_SpecimenAdditiveEntity"; 451 if (code == V3EntityCode.ACDA) 452 return "ACDA"; 453 if (code == V3EntityCode.ACDB) 454 return "ACDB"; 455 if (code == V3EntityCode.ACET) 456 return "ACET"; 457 if (code == V3EntityCode.AMIES) 458 return "AMIES"; 459 if (code == V3EntityCode.BACTM) 460 return "BACTM"; 461 if (code == V3EntityCode.BF10) 462 return "BF10"; 463 if (code == V3EntityCode.BOR) 464 return "BOR"; 465 if (code == V3EntityCode.BOUIN) 466 return "BOUIN"; 467 if (code == V3EntityCode.BSKM) 468 return "BSKM"; 469 if (code == V3EntityCode.C32) 470 return "C32"; 471 if (code == V3EntityCode.C38) 472 return "C38"; 473 if (code == V3EntityCode.CARS) 474 return "CARS"; 475 if (code == V3EntityCode.CARY) 476 return "CARY"; 477 if (code == V3EntityCode.CHLTM) 478 return "CHLTM"; 479 if (code == V3EntityCode.CTAD) 480 return "CTAD"; 481 if (code == V3EntityCode.EDTK15) 482 return "EDTK15"; 483 if (code == V3EntityCode.EDTK75) 484 return "EDTK75"; 485 if (code == V3EntityCode.EDTN) 486 return "EDTN"; 487 if (code == V3EntityCode.ENT) 488 return "ENT"; 489 if (code == V3EntityCode.F10) 490 return "F10"; 491 if (code == V3EntityCode.FDP) 492 return "FDP"; 493 if (code == V3EntityCode.FL10) 494 return "FL10"; 495 if (code == V3EntityCode.FL100) 496 return "FL100"; 497 if (code == V3EntityCode.HCL6) 498 return "HCL6"; 499 if (code == V3EntityCode.HEPA) 500 return "HEPA"; 501 if (code == V3EntityCode.HEPL) 502 return "HEPL"; 503 if (code == V3EntityCode.HEPN) 504 return "HEPN"; 505 if (code == V3EntityCode.HNO3) 506 return "HNO3"; 507 if (code == V3EntityCode.JKM) 508 return "JKM"; 509 if (code == V3EntityCode.KARN) 510 return "KARN"; 511 if (code == V3EntityCode.KOX) 512 return "KOX"; 513 if (code == V3EntityCode.LIA) 514 return "LIA"; 515 if (code == V3EntityCode.M4) 516 return "M4"; 517 if (code == V3EntityCode.M4RT) 518 return "M4RT"; 519 if (code == V3EntityCode.M5) 520 return "M5"; 521 if (code == V3EntityCode.MICHTM) 522 return "MICHTM"; 523 if (code == V3EntityCode.MMDTM) 524 return "MMDTM"; 525 if (code == V3EntityCode.NAF) 526 return "NAF"; 527 if (code == V3EntityCode.NONE) 528 return "NONE"; 529 if (code == V3EntityCode.PAGE) 530 return "PAGE"; 531 if (code == V3EntityCode.PHENOL) 532 return "PHENOL"; 533 if (code == V3EntityCode.PVA) 534 return "PVA"; 535 if (code == V3EntityCode.RLM) 536 return "RLM"; 537 if (code == V3EntityCode.SILICA) 538 return "SILICA"; 539 if (code == V3EntityCode.SPS) 540 return "SPS"; 541 if (code == V3EntityCode.SST) 542 return "SST"; 543 if (code == V3EntityCode.STUTM) 544 return "STUTM"; 545 if (code == V3EntityCode.THROM) 546 return "THROM"; 547 if (code == V3EntityCode.THYMOL) 548 return "THYMOL"; 549 if (code == V3EntityCode.THYO) 550 return "THYO"; 551 if (code == V3EntityCode.TOLU) 552 return "TOLU"; 553 if (code == V3EntityCode.URETM) 554 return "URETM"; 555 if (code == V3EntityCode.VIRTM) 556 return "VIRTM"; 557 if (code == V3EntityCode.WEST) 558 return "WEST"; 559 if (code == V3EntityCode.BLDPRD) 560 return "BLDPRD"; 561 if (code == V3EntityCode.VCCNE) 562 return "VCCNE"; 563 if (code == V3EntityCode._DRUGENTITY) 564 return "_DrugEntity"; 565 if (code == V3EntityCode._CLINICALDRUG) 566 return "_ClinicalDrug"; 567 if (code == V3EntityCode._NONDRUGAGENTENTITY) 568 return "_NonDrugAgentEntity"; 569 if (code == V3EntityCode.NDA01) 570 return "NDA01"; 571 if (code == V3EntityCode.NDA02) 572 return "NDA02"; 573 if (code == V3EntityCode.NDA03) 574 return "NDA03"; 575 if (code == V3EntityCode.NDA04) 576 return "NDA04"; 577 if (code == V3EntityCode.NDA05) 578 return "NDA05"; 579 if (code == V3EntityCode.NDA06) 580 return "NDA06"; 581 if (code == V3EntityCode.NDA07) 582 return "NDA07"; 583 if (code == V3EntityCode.NDA08) 584 return "NDA08"; 585 if (code == V3EntityCode.NDA09) 586 return "NDA09"; 587 if (code == V3EntityCode.NDA10) 588 return "NDA10"; 589 if (code == V3EntityCode.NDA11) 590 return "NDA11"; 591 if (code == V3EntityCode.NDA12) 592 return "NDA12"; 593 if (code == V3EntityCode.NDA13) 594 return "NDA13"; 595 if (code == V3EntityCode.NDA14) 596 return "NDA14"; 597 if (code == V3EntityCode.NDA15) 598 return "NDA15"; 599 if (code == V3EntityCode.NDA16) 600 return "NDA16"; 601 if (code == V3EntityCode.NDA17) 602 return "NDA17"; 603 if (code == V3EntityCode._ORGANIZATIONENTITYTYPE) 604 return "_OrganizationEntityType"; 605 if (code == V3EntityCode.HHOLD) 606 return "HHOLD"; 607 if (code == V3EntityCode.NAT) 608 return "NAT"; 609 if (code == V3EntityCode.RELIG) 610 return "RELIG"; 611 if (code == V3EntityCode._PLACEENTITYTYPE) 612 return "_PlaceEntityType"; 613 if (code == V3EntityCode.BED) 614 return "BED"; 615 if (code == V3EntityCode.BLDG) 616 return "BLDG"; 617 if (code == V3EntityCode.FLOOR) 618 return "FLOOR"; 619 if (code == V3EntityCode.ROOM) 620 return "ROOM"; 621 if (code == V3EntityCode.WING) 622 return "WING"; 623 if (code == V3EntityCode._RESOURCEGROUPENTITYTYPE) 624 return "_ResourceGroupEntityType"; 625 if (code == V3EntityCode.PRAC) 626 return "PRAC"; 627 return "?"; 628 } 629 630 public String toSystem(V3EntityCode code) { 631 return code.getSystem(); 632 } 633 634}