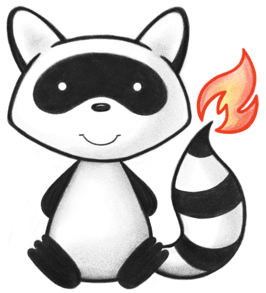
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum V3EntityDeterminer { 037 038 /** 039 * Description:A determiner that specifies that the Entity object represents a 040 * particular physical thing (as opposed to a universal, kind, or class of 041 * physical thing). 042 * 043 * 044 * Discussion: It does not matter whether an INSTANCE still exists as a whole at 045 * the point in time (or process) when we mention it, for example, a drug 046 * product lot is an INSTANCE even though it has been portioned out for retail 047 * purpose. 048 */ 049 INSTANCE, 050 /** 051 * A determiner that specifies that the Entity object represents a particular 052 * collection of physical things (as opposed to a universal, kind, or class of 053 * physical thing). While the collection may resolve to having only a single 054 * individual (or even no individuals), the potential should exist for it to 055 * cover multiple individuals. 056 */ 057 GROUP, 058 /** 059 * Description:A determiner that specifies that the Entity object represents a 060 * universal, kind or class of physical thing (as opposed to a particular 061 * thing). 062 */ 063 KIND, 064 /** 065 * A determiner that specifies that the Entity object represents a universal, 066 * kind or class of collections physical things. While the collection may 067 * resolve to having only a single individual (or even no individuals), the 068 * potential should exist for it to cover multiple individuals. 069 */ 070 GROUPKIND, 071 /** 072 * The described quantified determiner indicates that the given Entity is taken 073 * as a general description of a specific amount of a thing. For example, 074 * QUANTIFIED_KIND of syringe (quantity = 3,) stands for exactly three syringes. 075 */ 076 QUANTIFIEDKIND, 077 /** 078 * added to help the parsers 079 */ 080 NULL; 081 082 public static V3EntityDeterminer fromCode(String codeString) throws FHIRException { 083 if (codeString == null || "".equals(codeString)) 084 return null; 085 if ("INSTANCE".equals(codeString)) 086 return INSTANCE; 087 if ("GROUP".equals(codeString)) 088 return GROUP; 089 if ("KIND".equals(codeString)) 090 return KIND; 091 if ("GROUPKIND".equals(codeString)) 092 return GROUPKIND; 093 if ("QUANTIFIED_KIND".equals(codeString)) 094 return QUANTIFIEDKIND; 095 throw new FHIRException("Unknown V3EntityDeterminer code '" + codeString + "'"); 096 } 097 098 public String toCode() { 099 switch (this) { 100 case INSTANCE: 101 return "INSTANCE"; 102 case GROUP: 103 return "GROUP"; 104 case KIND: 105 return "KIND"; 106 case GROUPKIND: 107 return "GROUPKIND"; 108 case QUANTIFIEDKIND: 109 return "QUANTIFIED_KIND"; 110 case NULL: 111 return null; 112 default: 113 return "?"; 114 } 115 } 116 117 public String getSystem() { 118 return "http://terminology.hl7.org/CodeSystem/v3-EntityDeterminer"; 119 } 120 121 public String getDefinition() { 122 switch (this) { 123 case INSTANCE: 124 return "Description:A determiner that specifies that the Entity object represents a particular physical thing (as opposed to a universal, kind, or class of physical thing).\r\n\n \n Discussion: It does not matter whether an INSTANCE still exists as a whole at the point in time (or process) when we mention it, for example, a drug product lot is an INSTANCE even though it has been portioned out for retail purpose."; 125 case GROUP: 126 return "A determiner that specifies that the Entity object represents a particular collection of physical things (as opposed to a universal, kind, or class of physical thing). While the collection may resolve to having only a single individual (or even no individuals), the potential should exist for it to cover multiple individuals."; 127 case KIND: 128 return "Description:A determiner that specifies that the Entity object represents a universal, kind or class of physical thing (as opposed to a particular thing)."; 129 case GROUPKIND: 130 return "A determiner that specifies that the Entity object represents a universal, kind or class of collections physical things. While the collection may resolve to having only a single individual (or even no individuals), the potential should exist for it to cover multiple individuals."; 131 case QUANTIFIEDKIND: 132 return "The described quantified determiner indicates that the given Entity is taken as a general description of a specific amount of a thing. For example, QUANTIFIED_KIND of syringe (quantity = 3,) stands for exactly three syringes."; 133 case NULL: 134 return null; 135 default: 136 return "?"; 137 } 138 } 139 140 public String getDisplay() { 141 switch (this) { 142 case INSTANCE: 143 return "specific"; 144 case GROUP: 145 return "specific group"; 146 case KIND: 147 return "described"; 148 case GROUPKIND: 149 return "described group"; 150 case QUANTIFIEDKIND: 151 return "described quantified"; 152 case NULL: 153 return null; 154 default: 155 return "?"; 156 } 157 } 158 159}