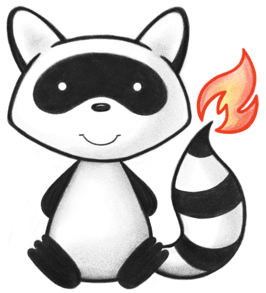
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum V3EntityHandling { 037 038 /** 039 * Keep at ambient temperature, 22 +/- 2C 040 */ 041 AMB, 042 /** 043 * Critical to keep at body temperature 36-38C 044 */ 045 C37, 046 /** 047 * Critical ambient - must not be refrigerated or frozen. 048 */ 049 CAMB, 050 /** 051 * Critical. Do not expose to atmosphere. Do not uncap. 052 */ 053 CATM, 054 /** 055 * Critical frozen. Specimen must not be allowed to thaw until immediately prior 056 * to testing. 057 */ 058 CFRZ, 059 /** 060 * Critical refrigerated - must not be allowed to freeze or warm until 061 * imediately prior to testing. 062 */ 063 CREF, 064 /** 065 * Deep Frozen -16 to -20C. 066 */ 067 DFRZ, 068 /** 069 * Keep in a dry environment 070 */ 071 DRY, 072 /** 073 * Keep frozen below 0 ?C 074 */ 075 FRZ, 076 /** 077 * Container is free of heavy metals, including lead. 078 */ 079 MTLF, 080 /** 081 * Keep in liquid nitrogen 082 */ 083 NTR, 084 /** 085 * Protect from light (eg. Wrap in aluminum foil). 086 */ 087 PRTL, 088 /** 089 * Do not shake 090 */ 091 PSA, 092 /** 093 * Protect against shock 094 */ 095 PSO, 096 /** 097 * Keep at refrigerated temperature:4-8C Accidental warming or freezing is of 098 * little consequence. 099 */ 100 REF, 101 /** 102 * Shake thoroughly before using 103 */ 104 SBU, 105 /** 106 * Ultra cold frozen -75 to -85C. Ultra cold freezer is typically at temperature 107 * of dry ice. 108 */ 109 UFRZ, 110 /** 111 * Keep upright, do not turn upside down 112 */ 113 UPR, 114 /** 115 * added to help the parsers 116 */ 117 NULL; 118 119 public static V3EntityHandling fromCode(String codeString) throws FHIRException { 120 if (codeString == null || "".equals(codeString)) 121 return null; 122 if ("AMB".equals(codeString)) 123 return AMB; 124 if ("C37".equals(codeString)) 125 return C37; 126 if ("CAMB".equals(codeString)) 127 return CAMB; 128 if ("CATM".equals(codeString)) 129 return CATM; 130 if ("CFRZ".equals(codeString)) 131 return CFRZ; 132 if ("CREF".equals(codeString)) 133 return CREF; 134 if ("DFRZ".equals(codeString)) 135 return DFRZ; 136 if ("DRY".equals(codeString)) 137 return DRY; 138 if ("FRZ".equals(codeString)) 139 return FRZ; 140 if ("MTLF".equals(codeString)) 141 return MTLF; 142 if ("NTR".equals(codeString)) 143 return NTR; 144 if ("PRTL".equals(codeString)) 145 return PRTL; 146 if ("PSA".equals(codeString)) 147 return PSA; 148 if ("PSO".equals(codeString)) 149 return PSO; 150 if ("REF".equals(codeString)) 151 return REF; 152 if ("SBU".equals(codeString)) 153 return SBU; 154 if ("UFRZ".equals(codeString)) 155 return UFRZ; 156 if ("UPR".equals(codeString)) 157 return UPR; 158 throw new FHIRException("Unknown V3EntityHandling code '" + codeString + "'"); 159 } 160 161 public String toCode() { 162 switch (this) { 163 case AMB: 164 return "AMB"; 165 case C37: 166 return "C37"; 167 case CAMB: 168 return "CAMB"; 169 case CATM: 170 return "CATM"; 171 case CFRZ: 172 return "CFRZ"; 173 case CREF: 174 return "CREF"; 175 case DFRZ: 176 return "DFRZ"; 177 case DRY: 178 return "DRY"; 179 case FRZ: 180 return "FRZ"; 181 case MTLF: 182 return "MTLF"; 183 case NTR: 184 return "NTR"; 185 case PRTL: 186 return "PRTL"; 187 case PSA: 188 return "PSA"; 189 case PSO: 190 return "PSO"; 191 case REF: 192 return "REF"; 193 case SBU: 194 return "SBU"; 195 case UFRZ: 196 return "UFRZ"; 197 case UPR: 198 return "UPR"; 199 case NULL: 200 return null; 201 default: 202 return "?"; 203 } 204 } 205 206 public String getSystem() { 207 return "http://terminology.hl7.org/CodeSystem/v3-EntityHandling"; 208 } 209 210 public String getDefinition() { 211 switch (this) { 212 case AMB: 213 return "Keep at ambient temperature, 22 +/- 2C"; 214 case C37: 215 return "Critical to keep at body temperature 36-38C"; 216 case CAMB: 217 return "Critical ambient - must not be refrigerated or frozen."; 218 case CATM: 219 return "Critical. Do not expose to atmosphere. Do not uncap."; 220 case CFRZ: 221 return "Critical frozen. Specimen must not be allowed to thaw until immediately prior to testing."; 222 case CREF: 223 return "Critical refrigerated - must not be allowed to freeze or warm until imediately prior to testing."; 224 case DFRZ: 225 return "Deep Frozen -16 to -20C."; 226 case DRY: 227 return "Keep in a dry environment"; 228 case FRZ: 229 return "Keep frozen below 0 ?C"; 230 case MTLF: 231 return "Container is free of heavy metals, including lead."; 232 case NTR: 233 return "Keep in liquid nitrogen"; 234 case PRTL: 235 return "Protect from light (eg. Wrap in aluminum foil)."; 236 case PSA: 237 return "Do not shake"; 238 case PSO: 239 return "Protect against shock"; 240 case REF: 241 return "Keep at refrigerated temperature:4-8C Accidental warming or freezing is of little consequence."; 242 case SBU: 243 return "Shake thoroughly before using"; 244 case UFRZ: 245 return "Ultra cold frozen -75 to -85C. Ultra cold freezer is typically at temperature of dry ice."; 246 case UPR: 247 return "Keep upright, do not turn upside down"; 248 case NULL: 249 return null; 250 default: 251 return "?"; 252 } 253 } 254 255 public String getDisplay() { 256 switch (this) { 257 case AMB: 258 return "Ambient Temperature"; 259 case C37: 260 return "Body Temperature"; 261 case CAMB: 262 return "Critical Ambient temperature"; 263 case CATM: 264 return "Protect from Air"; 265 case CFRZ: 266 return "Critical frozen"; 267 case CREF: 268 return "Critical refrigerated temperature"; 269 case DFRZ: 270 return "Deep Frozen"; 271 case DRY: 272 return "dry"; 273 case FRZ: 274 return "frozen"; 275 case MTLF: 276 return "Metal Free"; 277 case NTR: 278 return "nitrogen"; 279 case PRTL: 280 return "Protect from Light"; 281 case PSA: 282 return "do not shake"; 283 case PSO: 284 return "no shock"; 285 case REF: 286 return "Refrigerated temperature"; 287 case SBU: 288 return "Shake before use"; 289 case UFRZ: 290 return "Ultra frozen"; 291 case UPR: 292 return "upright"; 293 case NULL: 294 return null; 295 default: 296 return "?"; 297 } 298 } 299 300}