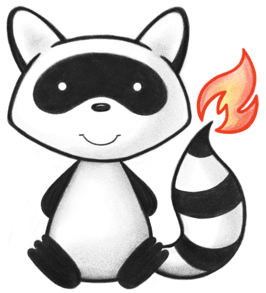
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum V3EntityNameUse { 037 038 /** 039 * Identifies the different representations of a name. The representation may 040 * affect how the name is used. (E.g. use of Ideographic for formal 041 * communications.) 042 */ 043 _NAMEREPRESENTATIONUSE, 044 /** 045 * Alphabetic transcription of name (Japanese: romaji) 046 */ 047 ABC, 048 /** 049 * Ideographic representation of name (e.g., Japanese kanji, Chinese characters) 050 */ 051 IDE, 052 /** 053 * Syllabic transcription of name (e.g., Japanese kana, Korean hangul) 054 */ 055 SYL, 056 /** 057 * A name assigned to a person. Reasons some organizations assign alternate 058 * names may include not knowing the person's name, or to maintain anonymity. 059 * Some, but not necessarily all, of the name types that people call "alias" may 060 * fit into this category. 061 */ 062 ASGN, 063 /** 064 * As recorded on a license, record, certificate, etc. (only if different from 065 * legal name) 066 */ 067 C, 068 /** 069 * e.g. Chief Red Cloud 070 */ 071 I, 072 /** 073 * Known as/conventional/the one you use 074 */ 075 L, 076 /** 077 * Definition:The formal name as registered in an official (government) 078 * registry, but which name might not be commonly used. Particularly used in 079 * countries with a law system based on Napoleonic law. 080 */ 081 OR, 082 /** 083 * A self asserted name that the person is using or has used. 084 */ 085 P, 086 /** 087 * Includes writer's pseudonym, stage name, etc 088 */ 089 A, 090 /** 091 * e.g. Sister Mary Francis, Brother John 092 */ 093 R, 094 /** 095 * A name intended for use in searching or matching. 096 */ 097 SRCH, 098 /** 099 * A name spelled phonetically. 100 * 101 * There are a variety of phonetic spelling algorithms. This code value does not 102 * distinguish between these.Discussion: 103 */ 104 PHON, 105 /** 106 * A name spelled according to the SoundEx algorithm. 107 */ 108 SNDX, 109 /** 110 * added to help the parsers 111 */ 112 NULL; 113 114 public static V3EntityNameUse fromCode(String codeString) throws FHIRException { 115 if (codeString == null || "".equals(codeString)) 116 return null; 117 if ("_NameRepresentationUse".equals(codeString)) 118 return _NAMEREPRESENTATIONUSE; 119 if ("ABC".equals(codeString)) 120 return ABC; 121 if ("IDE".equals(codeString)) 122 return IDE; 123 if ("SYL".equals(codeString)) 124 return SYL; 125 if ("ASGN".equals(codeString)) 126 return ASGN; 127 if ("C".equals(codeString)) 128 return C; 129 if ("I".equals(codeString)) 130 return I; 131 if ("L".equals(codeString)) 132 return L; 133 if ("OR".equals(codeString)) 134 return OR; 135 if ("P".equals(codeString)) 136 return P; 137 if ("A".equals(codeString)) 138 return A; 139 if ("R".equals(codeString)) 140 return R; 141 if ("SRCH".equals(codeString)) 142 return SRCH; 143 if ("PHON".equals(codeString)) 144 return PHON; 145 if ("SNDX".equals(codeString)) 146 return SNDX; 147 throw new FHIRException("Unknown V3EntityNameUse code '" + codeString + "'"); 148 } 149 150 public String toCode() { 151 switch (this) { 152 case _NAMEREPRESENTATIONUSE: 153 return "_NameRepresentationUse"; 154 case ABC: 155 return "ABC"; 156 case IDE: 157 return "IDE"; 158 case SYL: 159 return "SYL"; 160 case ASGN: 161 return "ASGN"; 162 case C: 163 return "C"; 164 case I: 165 return "I"; 166 case L: 167 return "L"; 168 case OR: 169 return "OR"; 170 case P: 171 return "P"; 172 case A: 173 return "A"; 174 case R: 175 return "R"; 176 case SRCH: 177 return "SRCH"; 178 case PHON: 179 return "PHON"; 180 case SNDX: 181 return "SNDX"; 182 case NULL: 183 return null; 184 default: 185 return "?"; 186 } 187 } 188 189 public String getSystem() { 190 return "http://terminology.hl7.org/CodeSystem/v3-EntityNameUse"; 191 } 192 193 public String getDefinition() { 194 switch (this) { 195 case _NAMEREPRESENTATIONUSE: 196 return "Identifies the different representations of a name. The representation may affect how the name is used. (E.g. use of Ideographic for formal communications.)"; 197 case ABC: 198 return "Alphabetic transcription of name (Japanese: romaji)"; 199 case IDE: 200 return "Ideographic representation of name (e.g., Japanese kanji, Chinese characters)"; 201 case SYL: 202 return "Syllabic transcription of name (e.g., Japanese kana, Korean hangul)"; 203 case ASGN: 204 return "A name assigned to a person. Reasons some organizations assign alternate names may include not knowing the person's name, or to maintain anonymity. Some, but not necessarily all, of the name types that people call \"alias\" may fit into this category."; 205 case C: 206 return "As recorded on a license, record, certificate, etc. (only if different from legal name)"; 207 case I: 208 return "e.g. Chief Red Cloud"; 209 case L: 210 return "Known as/conventional/the one you use"; 211 case OR: 212 return "Definition:The formal name as registered in an official (government) registry, but which name might not be commonly used. Particularly used in countries with a law system based on Napoleonic law."; 213 case P: 214 return "A self asserted name that the person is using or has used."; 215 case A: 216 return "Includes writer's pseudonym, stage name, etc"; 217 case R: 218 return "e.g. Sister Mary Francis, Brother John"; 219 case SRCH: 220 return "A name intended for use in searching or matching."; 221 case PHON: 222 return "A name spelled phonetically.\r\n\n There are a variety of phonetic spelling algorithms. This code value does not distinguish between these.Discussion:"; 223 case SNDX: 224 return "A name spelled according to the SoundEx algorithm."; 225 case NULL: 226 return null; 227 default: 228 return "?"; 229 } 230 } 231 232 public String getDisplay() { 233 switch (this) { 234 case _NAMEREPRESENTATIONUSE: 235 return "NameRepresentationUse"; 236 case ABC: 237 return "Alphabetic"; 238 case IDE: 239 return "Ideographic"; 240 case SYL: 241 return "Syllabic"; 242 case ASGN: 243 return "assigned"; 244 case C: 245 return "License"; 246 case I: 247 return "Indigenous/Tribal"; 248 case L: 249 return "Legal"; 250 case OR: 251 return "official registry"; 252 case P: 253 return "pseudonym"; 254 case A: 255 return "Artist/Stage"; 256 case R: 257 return "Religious"; 258 case SRCH: 259 return "search"; 260 case PHON: 261 return "phonetic"; 262 case SNDX: 263 return "Soundex"; 264 case NULL: 265 return null; 266 default: 267 return "?"; 268 } 269 } 270 271}