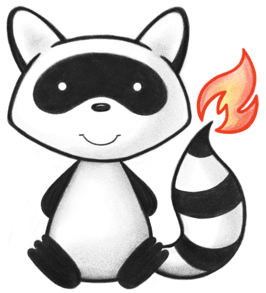
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum V3EntityNameUseR2 { 037 038 /** 039 * Description:A name that a person has assumed or has been assumed to them. 040 */ 041 ASSUMED, 042 /** 043 * Description:A name used in a Professional or Business context . Examples: 044 * Continuing to use a maiden name in a professional context, or using a stage 045 * performing name (some of these names are also pseudonyms) 046 */ 047 A, 048 /** 049 * Description:Anonymous assigned name (used to protect a persons identity for 050 * privacy reasons) 051 */ 052 ANON, 053 /** 054 * Description:e.g . Chief Red Cloud 055 */ 056 I, 057 /** 058 * Description:A non-official name by which the person is sometimes known. (This 059 * may also be used to record informal names such as a nickname) 060 */ 061 P, 062 /** 063 * Description:A name assumed as part of a religious vocation . e.g . Sister 064 * Mary Francis, Brother John 065 */ 066 R, 067 /** 068 * Description:Known as/conventional/the one you normally use 069 */ 070 C, 071 /** 072 * Description:A name used prior to marriage.Note that marriage naming customs 073 * vary greatly around the world. This name use is for use by applications that 074 * collect and store maiden names. Though the concept of maiden name is often 075 * gender specific, the use of this term is not gender specific. The use of this 076 * term does not imply any particular history for a person's name, nor should 077 * the maiden name be determined algorithmically 078 */ 079 M, 080 /** 081 * Description:Identifies the different representations of a name . The 082 * representation may affect how the name is used . (E.g . use of Ideographic 083 * for formal communications.) 084 */ 085 NAMEREPRESENTATIONUSE, 086 /** 087 * Description:Alphabetic transcription of name in latin alphabet (Japanese: 088 * romaji) 089 */ 090 ABC, 091 /** 092 * Description:Ideographic representation of name (e.g., Japanese kanji, Chinese 093 * characters) 094 */ 095 IDE, 096 /** 097 * Description:Syllabic transcription of name (e.g., Japanese kana, Korean 098 * hangul) 099 */ 100 SYL, 101 /** 102 * Description:This name is no longer in use (note: Names may also carry valid 103 * time ranges . This code is used to cover the situations where it is known 104 * that the name is no longer valid, but no particular time range for its use is 105 * known) 106 */ 107 OLD, 108 /** 109 * Description:This name should no longer be used when interacting with the 110 * person (i.e . in addition to no longer being used, the name should not be 111 * even mentioned when interacting with the person)Note: applications are not 112 * required to compare names labeled "Do Not Use" and other names in order to 113 * eliminate name parts that are common between the other name and a name 114 * labeled "Do Not Use". 115 */ 116 DN, 117 /** 118 * Description:The formal name as registered in an official (government) 119 * registry, but which name might not be commonly used . May correspond to the 120 * concept of legal name 121 */ 122 OR, 123 /** 124 * Description:The name as understood by the data enterer, i.e. a close 125 * approximation of a phonetic spelling of the name, not based on a phonetic 126 * algorithm. 127 */ 128 PHON, 129 /** 130 * Description:A name intended for use in searching or matching. This is used 131 * when the name is incomplete and contains enough details for search matching, 132 * but not enough for other uses. 133 */ 134 SRCH, 135 /** 136 * Description:A temporary name. Note that a name valid time can provide more 137 * detailed information. This may also be used for temporary names assigned at 138 * birth or in emergency situations. 139 */ 140 T, 141 /** 142 * added to help the parsers 143 */ 144 NULL; 145 146 public static V3EntityNameUseR2 fromCode(String codeString) throws FHIRException { 147 if (codeString == null || "".equals(codeString)) 148 return null; 149 if ("Assumed".equals(codeString)) 150 return ASSUMED; 151 if ("A".equals(codeString)) 152 return A; 153 if ("ANON".equals(codeString)) 154 return ANON; 155 if ("I".equals(codeString)) 156 return I; 157 if ("P".equals(codeString)) 158 return P; 159 if ("R".equals(codeString)) 160 return R; 161 if ("C".equals(codeString)) 162 return C; 163 if ("M".equals(codeString)) 164 return M; 165 if ("NameRepresentationUse".equals(codeString)) 166 return NAMEREPRESENTATIONUSE; 167 if ("ABC".equals(codeString)) 168 return ABC; 169 if ("IDE".equals(codeString)) 170 return IDE; 171 if ("SYL".equals(codeString)) 172 return SYL; 173 if ("OLD".equals(codeString)) 174 return OLD; 175 if ("DN".equals(codeString)) 176 return DN; 177 if ("OR".equals(codeString)) 178 return OR; 179 if ("PHON".equals(codeString)) 180 return PHON; 181 if ("SRCH".equals(codeString)) 182 return SRCH; 183 if ("T".equals(codeString)) 184 return T; 185 throw new FHIRException("Unknown V3EntityNameUseR2 code '" + codeString + "'"); 186 } 187 188 public String toCode() { 189 switch (this) { 190 case ASSUMED: 191 return "Assumed"; 192 case A: 193 return "A"; 194 case ANON: 195 return "ANON"; 196 case I: 197 return "I"; 198 case P: 199 return "P"; 200 case R: 201 return "R"; 202 case C: 203 return "C"; 204 case M: 205 return "M"; 206 case NAMEREPRESENTATIONUSE: 207 return "NameRepresentationUse"; 208 case ABC: 209 return "ABC"; 210 case IDE: 211 return "IDE"; 212 case SYL: 213 return "SYL"; 214 case OLD: 215 return "OLD"; 216 case DN: 217 return "DN"; 218 case OR: 219 return "OR"; 220 case PHON: 221 return "PHON"; 222 case SRCH: 223 return "SRCH"; 224 case T: 225 return "T"; 226 case NULL: 227 return null; 228 default: 229 return "?"; 230 } 231 } 232 233 public String getSystem() { 234 return "http://terminology.hl7.org/CodeSystem/v3-EntityNameUseR2"; 235 } 236 237 public String getDefinition() { 238 switch (this) { 239 case ASSUMED: 240 return "Description:A name that a person has assumed or has been assumed to them."; 241 case A: 242 return "Description:A name used in a Professional or Business context . Examples: Continuing to use a maiden name in a professional context, or using a stage performing name (some of these names are also pseudonyms)"; 243 case ANON: 244 return "Description:Anonymous assigned name (used to protect a persons identity for privacy reasons)"; 245 case I: 246 return "Description:e.g . Chief Red Cloud"; 247 case P: 248 return "Description:A non-official name by which the person is sometimes known. (This may also be used to record informal names such as a nickname)"; 249 case R: 250 return "Description:A name assumed as part of a religious vocation . e.g . Sister Mary Francis, Brother John"; 251 case C: 252 return "Description:Known as/conventional/the one you normally use"; 253 case M: 254 return "Description:A name used prior to marriage.Note that marriage naming customs vary greatly around the world. This name use is for use by applications that collect and store maiden names. Though the concept of maiden name is often gender specific, the use of this term is not gender specific. The use of this term does not imply any particular history for a person's name, nor should the maiden name be determined algorithmically"; 255 case NAMEREPRESENTATIONUSE: 256 return "Description:Identifies the different representations of a name . The representation may affect how the name is used . (E.g . use of Ideographic for formal communications.)"; 257 case ABC: 258 return "Description:Alphabetic transcription of name in latin alphabet (Japanese: romaji)"; 259 case IDE: 260 return "Description:Ideographic representation of name (e.g., Japanese kanji, Chinese characters)"; 261 case SYL: 262 return "Description:Syllabic transcription of name (e.g., Japanese kana, Korean hangul)"; 263 case OLD: 264 return "Description:This name is no longer in use (note: Names may also carry valid time ranges . This code is used to cover the situations where it is known that the name is no longer valid, but no particular time range for its use is known)"; 265 case DN: 266 return "Description:This name should no longer be used when interacting with the person (i.e . in addition to no longer being used, the name should not be even mentioned when interacting with the person)Note: applications are not required to compare names labeled \"Do Not Use\" and other names in order to eliminate name parts that are common between the other name and a name labeled \"Do Not Use\"."; 267 case OR: 268 return "Description:The formal name as registered in an official (government) registry, but which name might not be commonly used . May correspond to the concept of legal name"; 269 case PHON: 270 return "Description:The name as understood by the data enterer, i.e. a close approximation of a phonetic spelling of the name, not based on a phonetic algorithm."; 271 case SRCH: 272 return "Description:A name intended for use in searching or matching. This is used when the name is incomplete and contains enough details for search matching, but not enough for other uses."; 273 case T: 274 return "Description:A temporary name. Note that a name valid time can provide more detailed information. This may also be used for temporary names assigned at birth or in emergency situations."; 275 case NULL: 276 return null; 277 default: 278 return "?"; 279 } 280 } 281 282 public String getDisplay() { 283 switch (this) { 284 case ASSUMED: 285 return "Assumed"; 286 case A: 287 return "business name"; 288 case ANON: 289 return "Anonymous"; 290 case I: 291 return "Indigenous/Tribal"; 292 case P: 293 return "Other/Pseudonym/Alias"; 294 case R: 295 return "religious"; 296 case C: 297 return "customary"; 298 case M: 299 return "maiden name"; 300 case NAMEREPRESENTATIONUSE: 301 return "NameRepresentationUse"; 302 case ABC: 303 return "alphabetic"; 304 case IDE: 305 return "ideographic"; 306 case SYL: 307 return "syllabic"; 308 case OLD: 309 return "no longer in use"; 310 case DN: 311 return "do not use"; 312 case OR: 313 return "official registry name"; 314 case PHON: 315 return "phonetic"; 316 case SRCH: 317 return "search"; 318 case T: 319 return "temporary"; 320 case NULL: 321 return null; 322 default: 323 return "?"; 324 } 325 } 326 327}