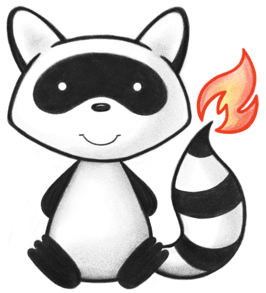
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum V3HL7UpdateMode { 037 038 /** 039 * Description:The item was (or is to be) added, having not been present 040 * immediately before. (If it is already present, this may be treated as an 041 * error condition.) 042 */ 043 A, 044 /** 045 * Description:The item was (or is to be) either added or replaced. 046 */ 047 AR, 048 /** 049 * Description:The item was (or is to be) removed (sometimes referred to as 050 * deleted). If the item is part of a collection, delete any matching items. 051 */ 052 D, 053 /** 054 * Description:This item is part of the identifying information for this object. 055 */ 056 K, 057 /** 058 * Description:There was (or is to be) no change to the item. This is primarily 059 * used when this element has not changed, but other attributes in the instance 060 * have changed. 061 */ 062 N, 063 /** 064 * Description:The item existed previously and has (or is to be) revised. (If an 065 * item does not already exist, this may be treated as an error condition.) 066 */ 067 R, 068 /** 069 * Description:This item provides enough information to allow a processing 070 * system to locate the full applicable record by identifying the object. 071 */ 072 REF, 073 /** 074 * Description:Description:</b>It is not specified whether or what kind of 075 * change has occurred to the item, or whether the item is present as a 076 * reference or identifying property. 077 */ 078 U, 079 /** 080 * These concepts apply when the element and/or message is updating a set of 081 * items. 082 */ 083 _SETUPDATEMODE, 084 /** 085 * Add the message element to the collection of items on the receiving system 086 * that correspond to the message element. 087 */ 088 ESA, 089 /** 090 * Change the item on the receiving system that corresponds to this message 091 * element; if a matching element does not exist, add a new one created with the 092 * values in the message. 093 */ 094 ESAC, 095 /** 096 * Change the item on the receiving system that corresponds to this message 097 * element; do not process if a matching element does not exist. 098 */ 099 ESC, 100 /** 101 * Delete the item on the receiving system that corresponds to this message 102 * element. 103 */ 104 ESD, 105 /** 106 * Description: AU: If this item exists, update it with these values. If it does 107 * not exist, create it with these values. If the item is part of the 108 * collection, update each item that matches this item, and if no items match, 109 * add a new item to the collection. 110 */ 111 AU, 112 /** 113 * Ignore this role, it is not relevant to the update. 114 */ 115 I, 116 /** 117 * Verify - this message element must match a value already in the receiving 118 * systems database in order to process the message. 119 */ 120 V, 121 /** 122 * added to help the parsers 123 */ 124 NULL; 125 126 public static V3HL7UpdateMode fromCode(String codeString) throws FHIRException { 127 if (codeString == null || "".equals(codeString)) 128 return null; 129 if ("A".equals(codeString)) 130 return A; 131 if ("AR".equals(codeString)) 132 return AR; 133 if ("D".equals(codeString)) 134 return D; 135 if ("K".equals(codeString)) 136 return K; 137 if ("N".equals(codeString)) 138 return N; 139 if ("R".equals(codeString)) 140 return R; 141 if ("REF".equals(codeString)) 142 return REF; 143 if ("U".equals(codeString)) 144 return U; 145 if ("_SetUpdateMode".equals(codeString)) 146 return _SETUPDATEMODE; 147 if ("ESA".equals(codeString)) 148 return ESA; 149 if ("ESAC".equals(codeString)) 150 return ESAC; 151 if ("ESC".equals(codeString)) 152 return ESC; 153 if ("ESD".equals(codeString)) 154 return ESD; 155 if ("AU".equals(codeString)) 156 return AU; 157 if ("I".equals(codeString)) 158 return I; 159 if ("V".equals(codeString)) 160 return V; 161 throw new FHIRException("Unknown V3HL7UpdateMode code '" + codeString + "'"); 162 } 163 164 public String toCode() { 165 switch (this) { 166 case A: 167 return "A"; 168 case AR: 169 return "AR"; 170 case D: 171 return "D"; 172 case K: 173 return "K"; 174 case N: 175 return "N"; 176 case R: 177 return "R"; 178 case REF: 179 return "REF"; 180 case U: 181 return "U"; 182 case _SETUPDATEMODE: 183 return "_SetUpdateMode"; 184 case ESA: 185 return "ESA"; 186 case ESAC: 187 return "ESAC"; 188 case ESC: 189 return "ESC"; 190 case ESD: 191 return "ESD"; 192 case AU: 193 return "AU"; 194 case I: 195 return "I"; 196 case V: 197 return "V"; 198 case NULL: 199 return null; 200 default: 201 return "?"; 202 } 203 } 204 205 public String getSystem() { 206 return "http://terminology.hl7.org/CodeSystem/v3-HL7UpdateMode"; 207 } 208 209 public String getDefinition() { 210 switch (this) { 211 case A: 212 return "Description:The item was (or is to be) added, having not been present immediately before. (If it is already present, this may be treated as an error condition.)"; 213 case AR: 214 return "Description:The item was (or is to be) either added or replaced."; 215 case D: 216 return "Description:The item was (or is to be) removed (sometimes referred to as deleted). If the item is part of a collection, delete any matching items."; 217 case K: 218 return "Description:This item is part of the identifying information for this object."; 219 case N: 220 return "Description:There was (or is to be) no change to the item. This is primarily used when this element has not changed, but other attributes in the instance have changed."; 221 case R: 222 return "Description:The item existed previously and has (or is to be) revised. (If an item does not already exist, this may be treated as an error condition.)"; 223 case REF: 224 return "Description:This item provides enough information to allow a processing system to locate the full applicable record by identifying the object."; 225 case U: 226 return "Description:Description:</b>It is not specified whether or what kind of change has occurred to the item, or whether the item is present as a reference or identifying property."; 227 case _SETUPDATEMODE: 228 return "These concepts apply when the element and/or message is updating a set of items."; 229 case ESA: 230 return "Add the message element to the collection of items on the receiving system that correspond to the message element."; 231 case ESAC: 232 return "Change the item on the receiving system that corresponds to this message element; if a matching element does not exist, add a new one created with the values in the message."; 233 case ESC: 234 return "Change the item on the receiving system that corresponds to this message element; do not process if a matching element does not exist."; 235 case ESD: 236 return "Delete the item on the receiving system that corresponds to this message element."; 237 case AU: 238 return "Description: AU: If this item exists, update it with these values. If it does not exist, create it with these values. If the item is part of the collection, update each item that matches this item, and if no items match, add a new item to the collection."; 239 case I: 240 return "Ignore this role, it is not relevant to the update."; 241 case V: 242 return "Verify - this message element must match a value already in the receiving systems database in order to process the message."; 243 case NULL: 244 return null; 245 default: 246 return "?"; 247 } 248 } 249 250 public String getDisplay() { 251 switch (this) { 252 case A: 253 return "Add"; 254 case AR: 255 return "Add or Replace"; 256 case D: 257 return "Remove"; 258 case K: 259 return "Key"; 260 case N: 261 return "No Change"; 262 case R: 263 return "Replace"; 264 case REF: 265 return "Reference"; 266 case U: 267 return "Unknown"; 268 case _SETUPDATEMODE: 269 return "SetUpdateMode"; 270 case ESA: 271 return "Set Add"; 272 case ESAC: 273 return "Set Add or Change"; 274 case ESC: 275 return "Set Change"; 276 case ESD: 277 return "Set Delete"; 278 case AU: 279 return "Add or Update"; 280 case I: 281 return "Ignore"; 282 case V: 283 return "Verify"; 284 case NULL: 285 return null; 286 default: 287 return "?"; 288 } 289 } 290 291}