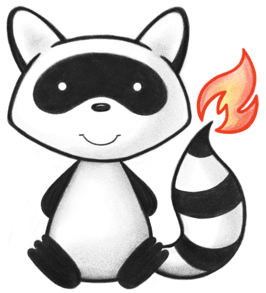
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum V3Hl7ApprovalStatus { 037 038 /** 039 * Description: Content that is being presented to an international affiliate 040 * for consideration as a realm-specific draft standard for trial use. 041 */ 042 AFFD, 043 /** 044 * Description: Content that is being presented to an international affiliate 045 * for consideration as a realm-specific informative standard. 046 */ 047 AFFI, 048 /** 049 * Description: Content that is being presented to an international affiliate 050 * for consideration as a realm-specific normative standard. 051 */ 052 AFFN, 053 /** 054 * Description: Content that has passed ballot as a realm-specific draft 055 * standard for trial use. 056 */ 057 APPAD, 058 /** 059 * Description: Content that has passed ballot as a realm-specific informative 060 * standard. 061 */ 062 APPAI, 063 /** 064 * Description: Content that has passed ballot as a realm-specific normative 065 * standard 066 */ 067 APPAN, 068 /** 069 * Description: Content that has passed ballot as a draft standard for trial 070 * use. 071 */ 072 APPD, 073 /** 074 * Description: Content that has passed ballot as a normative standard. 075 */ 076 APPI, 077 /** 078 * Description: Content that has passed ballot as a normative standard. 079 */ 080 APPN, 081 /** 082 * Description: Content prepared by a committee and submitted for internal 083 * consideration as an informative standard. 084 * 085 * 086 * 087 * Deprecation Comment No longer supported as ballot statuses within the HL7 088 * Governance and Operations Manual. Use normative or informative variants 089 * instead. 090 */ 091 COMI, 092 /** 093 * Description: Content prepared by a committee and submitted for internal 094 * consideration as an informative standard. 095 * 096 * 097 * 098 * Deprecation Comment No longer supported as ballot statuses within the HL7 099 * Governance and Operations Manual. Use normative or informative variants 100 * instead. 101 */ 102 COMN, 103 /** 104 * Description: Content that is under development and is not intended to be 105 * used. 106 */ 107 DRAFT, 108 /** 109 * Description: Content that represents an adaption of a implementable balloted 110 * material to represent the needs or capabilities of a particular installation. 111 */ 112 LOC, 113 /** 114 * Description: Content prepared by a committee and submitted for membership 115 * consideration as a draft standard for trial use. 116 */ 117 MEMD, 118 /** 119 * Description: Content prepared by a committee and submitted for membership 120 * consideration as an informative standard. 121 */ 122 MEMI, 123 /** 124 * Description: Content prepared by a committee and submitted for membership 125 * consideration as a normative standard. 126 */ 127 MEMN, 128 /** 129 * Description: Content developed independently by an organization or individual 130 * that is declared to be 'usable' but for which there is no present intention 131 * to submit through the standards submission and review process. 132 */ 133 NS, 134 /** 135 * Description: Content submitted to a committee for consideration for future 136 * inclusion in the standard. 137 */ 138 PROP, 139 /** 140 * Description: Content intended to support other content that is subject to 141 * approval, but which is not itself subject to formal approval. 142 */ 143 REF, 144 /** 145 * Description: Content that represents an item that was at one point a 146 * normative or informative standard, but was subsequently withdrawn. 147 */ 148 WD, 149 /** 150 * added to help the parsers 151 */ 152 NULL; 153 154 public static V3Hl7ApprovalStatus fromCode(String codeString) throws FHIRException { 155 if (codeString == null || "".equals(codeString)) 156 return null; 157 if ("affd".equals(codeString)) 158 return AFFD; 159 if ("affi".equals(codeString)) 160 return AFFI; 161 if ("affn".equals(codeString)) 162 return AFFN; 163 if ("appad".equals(codeString)) 164 return APPAD; 165 if ("appai".equals(codeString)) 166 return APPAI; 167 if ("appan".equals(codeString)) 168 return APPAN; 169 if ("appd".equals(codeString)) 170 return APPD; 171 if ("appi".equals(codeString)) 172 return APPI; 173 if ("appn".equals(codeString)) 174 return APPN; 175 if ("comi".equals(codeString)) 176 return COMI; 177 if ("comn".equals(codeString)) 178 return COMN; 179 if ("draft".equals(codeString)) 180 return DRAFT; 181 if ("loc".equals(codeString)) 182 return LOC; 183 if ("memd".equals(codeString)) 184 return MEMD; 185 if ("memi".equals(codeString)) 186 return MEMI; 187 if ("memn".equals(codeString)) 188 return MEMN; 189 if ("ns".equals(codeString)) 190 return NS; 191 if ("prop".equals(codeString)) 192 return PROP; 193 if ("ref".equals(codeString)) 194 return REF; 195 if ("wd".equals(codeString)) 196 return WD; 197 throw new FHIRException("Unknown V3Hl7ApprovalStatus code '" + codeString + "'"); 198 } 199 200 public String toCode() { 201 switch (this) { 202 case AFFD: 203 return "affd"; 204 case AFFI: 205 return "affi"; 206 case AFFN: 207 return "affn"; 208 case APPAD: 209 return "appad"; 210 case APPAI: 211 return "appai"; 212 case APPAN: 213 return "appan"; 214 case APPD: 215 return "appd"; 216 case APPI: 217 return "appi"; 218 case APPN: 219 return "appn"; 220 case COMI: 221 return "comi"; 222 case COMN: 223 return "comn"; 224 case DRAFT: 225 return "draft"; 226 case LOC: 227 return "loc"; 228 case MEMD: 229 return "memd"; 230 case MEMI: 231 return "memi"; 232 case MEMN: 233 return "memn"; 234 case NS: 235 return "ns"; 236 case PROP: 237 return "prop"; 238 case REF: 239 return "ref"; 240 case WD: 241 return "wd"; 242 case NULL: 243 return null; 244 default: 245 return "?"; 246 } 247 } 248 249 public String getSystem() { 250 return "http://terminology.hl7.org/CodeSystem/v3-hl7ApprovalStatus"; 251 } 252 253 public String getDefinition() { 254 switch (this) { 255 case AFFD: 256 return "Description: Content that is being presented to an international affiliate for consideration as a realm-specific draft standard for trial use."; 257 case AFFI: 258 return "Description: Content that is being presented to an international affiliate for consideration as a realm-specific informative standard."; 259 case AFFN: 260 return "Description: Content that is being presented to an international affiliate for consideration as a realm-specific normative standard."; 261 case APPAD: 262 return "Description: Content that has passed ballot as a realm-specific draft standard for trial use."; 263 case APPAI: 264 return "Description: Content that has passed ballot as a realm-specific informative standard."; 265 case APPAN: 266 return "Description: Content that has passed ballot as a realm-specific normative standard"; 267 case APPD: 268 return "Description: Content that has passed ballot as a draft standard for trial use."; 269 case APPI: 270 return "Description: Content that has passed ballot as a normative standard."; 271 case APPN: 272 return "Description: Content that has passed ballot as a normative standard."; 273 case COMI: 274 return "Description: Content prepared by a committee and submitted for internal consideration as an informative standard.\r\n\n \n \n Deprecation Comment\n No longer supported as ballot statuses within the HL7 Governance and Operations Manual. Use normative or informative variants instead."; 275 case COMN: 276 return "Description: Content prepared by a committee and submitted for internal consideration as an informative standard.\r\n\n \n \n Deprecation Comment\n No longer supported as ballot statuses within the HL7 Governance and Operations Manual. Use normative or informative variants instead."; 277 case DRAFT: 278 return "Description: Content that is under development and is not intended to be used."; 279 case LOC: 280 return "Description: Content that represents an adaption of a implementable balloted material to represent the needs or capabilities of a particular installation."; 281 case MEMD: 282 return "Description: Content prepared by a committee and submitted for membership consideration as a draft standard for trial use."; 283 case MEMI: 284 return "Description: Content prepared by a committee and submitted for membership consideration as an informative standard."; 285 case MEMN: 286 return "Description: Content prepared by a committee and submitted for membership consideration as a normative standard."; 287 case NS: 288 return "Description: Content developed independently by an organization or individual that is declared to be 'usable' but for which there is no present intention to submit through the standards submission and review process."; 289 case PROP: 290 return "Description: Content submitted to a committee for consideration for future inclusion in the standard."; 291 case REF: 292 return "Description: Content intended to support other content that is subject to approval, but which is not itself subject to formal approval."; 293 case WD: 294 return "Description: Content that represents an item that was at one point a normative or informative standard, but was subsequently withdrawn."; 295 case NULL: 296 return null; 297 default: 298 return "?"; 299 } 300 } 301 302 public String getDisplay() { 303 switch (this) { 304 case AFFD: 305 return "affiliate ballot - DSTU"; 306 case AFFI: 307 return "affiliate ballot - informative"; 308 case AFFN: 309 return "affiliate ballot - normative"; 310 case APPAD: 311 return "approved affiliate DSTU"; 312 case APPAI: 313 return "approved affiliate informative"; 314 case APPAN: 315 return "approved affiliate normative"; 316 case APPD: 317 return "approved DSTU"; 318 case APPI: 319 return "approved informative"; 320 case APPN: 321 return "approved normative"; 322 case COMI: 323 return "committee ballot - informative"; 324 case COMN: 325 return "committee ballot - normative"; 326 case DRAFT: 327 return "draft"; 328 case LOC: 329 return "localized adaptation"; 330 case MEMD: 331 return "membership ballot - DSTU"; 332 case MEMI: 333 return "membership ballot - informative"; 334 case MEMN: 335 return "membership ballot - normative"; 336 case NS: 337 return "non-standard - available for use"; 338 case PROP: 339 return "proposal"; 340 case REF: 341 return "reference"; 342 case WD: 343 return "withdrawn"; 344 case NULL: 345 return null; 346 default: 347 return "?"; 348 } 349 } 350 351}