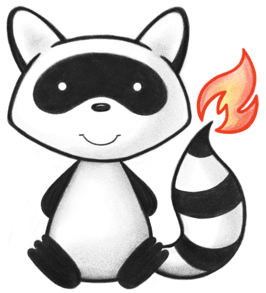
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum V3Hl7CMETAttribution { 037 038 /** 039 * Description: Provides sufficient information to allow the object identified 040 * to be contacted. This is likely to have the content of identified and 041 * confirmable plus telephone number. 042 */ 043 CONTACT, 044 /** 045 * Description: This variant is a proper subset of universal and is intended to 046 * provide sufficient information to identify the object(s) modeled by the CMET. 047 * This variant is only suitable for use within TIGHTLY COUPLED SYSTEMS ONLY. 048 * This variant provides ONLY the ID (and code where applicable) and Name. Other 049 * variants may not be substituted at runtime. 050 */ 051 IDENTIFIED, 052 /** 053 * Description: This extends the identified variant by adding just sufficient 054 * additional information to allow the identity of object modeled to be 055 * confirmed by a number of corroborating items of data; for instance a 056 * patient's date of birth and current address. However, specific contact 057 * information, such as telephone number, are not viewed as confirming 058 * information. 059 */ 060 IDENTIFIEDCONFIRMABLE, 061 /** 062 * Description: Generally the same information content as "contactable" but 063 * using new "informational" CMETs as dependant CMETs. This flavor allows 064 * expression of the CMET when non-focal class information is not known. 065 */ 066 IDENTIFIEDINFORMATIONAL, 067 /** 068 * Description: Generally the same information content as "contactable", but 069 * with required (not mandatory) ids on entry point class. This flavor allows 070 * expression of the CMET even when mandatory information is not known. 071 */ 072 INFORMATIONAL, 073 /** 074 * Description: Provides more than identified, but not as much as universal. 075 * There are not expected to be many of these. 076 */ 077 MINIMAL, 078 /** 079 * Description: This variant includes all attributes and associations present in 080 * the R-MIM. Any of non-mandatory and non-required attributes and/or 081 * associations may be present or absent, as permitted in the cardinality 082 * constraints. 083 */ 084 UNIVERSAL, 085 /** 086 * added to help the parsers 087 */ 088 NULL; 089 090 public static V3Hl7CMETAttribution fromCode(String codeString) throws FHIRException { 091 if (codeString == null || "".equals(codeString)) 092 return null; 093 if ("contact".equals(codeString)) 094 return CONTACT; 095 if ("identified".equals(codeString)) 096 return IDENTIFIED; 097 if ("identified-confirmable".equals(codeString)) 098 return IDENTIFIEDCONFIRMABLE; 099 if ("identified-informational".equals(codeString)) 100 return IDENTIFIEDINFORMATIONAL; 101 if ("informational".equals(codeString)) 102 return INFORMATIONAL; 103 if ("minimal".equals(codeString)) 104 return MINIMAL; 105 if ("universal".equals(codeString)) 106 return UNIVERSAL; 107 throw new FHIRException("Unknown V3Hl7CMETAttribution code '" + codeString + "'"); 108 } 109 110 public String toCode() { 111 switch (this) { 112 case CONTACT: 113 return "contact"; 114 case IDENTIFIED: 115 return "identified"; 116 case IDENTIFIEDCONFIRMABLE: 117 return "identified-confirmable"; 118 case IDENTIFIEDINFORMATIONAL: 119 return "identified-informational"; 120 case INFORMATIONAL: 121 return "informational"; 122 case MINIMAL: 123 return "minimal"; 124 case UNIVERSAL: 125 return "universal"; 126 case NULL: 127 return null; 128 default: 129 return "?"; 130 } 131 } 132 133 public String getSystem() { 134 return "http://terminology.hl7.org/CodeSystem/v3-hl7CMETAttribution"; 135 } 136 137 public String getDefinition() { 138 switch (this) { 139 case CONTACT: 140 return "Description: Provides sufficient information to allow the object identified to be contacted. This is likely to have the content of identified and confirmable plus telephone number."; 141 case IDENTIFIED: 142 return "Description: This variant is a proper subset of universal and is intended to provide sufficient information to identify the object(s) modeled by the CMET. This variant is only suitable for use within TIGHTLY COUPLED SYSTEMS ONLY. This variant provides ONLY the ID (and code where applicable) and Name. Other variants may not be substituted at runtime."; 143 case IDENTIFIEDCONFIRMABLE: 144 return "Description: This extends the identified variant by adding just sufficient additional information to allow the identity of object modeled to be confirmed by a number of corroborating items of data; for instance a patient's date of birth and current address. However, specific contact information, such as telephone number, are not viewed as confirming information."; 145 case IDENTIFIEDINFORMATIONAL: 146 return "Description: Generally the same information content as \"contactable\" but using new \"informational\" CMETs as dependant CMETs. This flavor allows expression of the CMET when non-focal class information is not known."; 147 case INFORMATIONAL: 148 return "Description: Generally the same information content as \"contactable\", but with required (not mandatory) ids on entry point class. This flavor allows expression of the CMET even when mandatory information is not known."; 149 case MINIMAL: 150 return "Description: Provides more than identified, but not as much as universal. There are not expected to be many of these."; 151 case UNIVERSAL: 152 return "Description: This variant includes all attributes and associations present in the R-MIM. Any of non-mandatory and non-required attributes and/or associations may be present or absent, as permitted in the cardinality constraints."; 153 case NULL: 154 return null; 155 default: 156 return "?"; 157 } 158 } 159 160 public String getDisplay() { 161 switch (this) { 162 case CONTACT: 163 return "contact"; 164 case IDENTIFIED: 165 return "identified"; 166 case IDENTIFIEDCONFIRMABLE: 167 return "identified-confirmable"; 168 case IDENTIFIEDINFORMATIONAL: 169 return "identified-informational"; 170 case INFORMATIONAL: 171 return "informational"; 172 case MINIMAL: 173 return "minimal"; 174 case UNIVERSAL: 175 return "universal"; 176 case NULL: 177 return null; 178 default: 179 return "?"; 180 } 181 } 182 183}