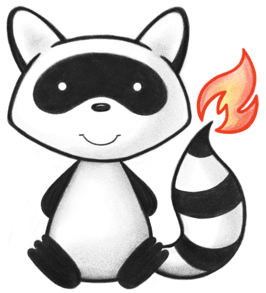
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum V3Hl7PublishingSection { 037 038 /** 039 * Description: Represents the HL7 V3 publishing section that deals with the 040 * administration and management of health care activities and organizations. 041 * 042 * 043 * UsageNote: V3 Specifications are published in a set of "domains", which 044 * contain interactions and related specifications for a single area of health 045 * care within which can be supported by a single, coherent set of 046 * interoperability specifications. 047 * 048 * For publishing purposes, these domains are aggregated into sub-sections of 049 * related health care areas and these sub-sections are further aggregated into 050 * three major sets. 051 */ 052 AM, 053 /** 054 * Description: Represents the HL7 V3 publishing section that deals with the 055 * health care provision and clinical management. 056 * 057 * 058 * UsageNote: V3 Specifications are published in a set of "domains", which 059 * contain interactions and related specifications for a single area of health 060 * care within which can be supported by a single, coherent set of 061 * interoperability specifications. 062 * 063 * For publishing purposes, these domains are aggregated into sub-sections of 064 * related health care areas and these sub-sections are further aggregated into 065 * three major sets. 066 */ 067 HM, 068 /** 069 * Description: Represents the HL7 V3 publishing section that deals with the 070 * definition and management of the computing and communication infrastructure 071 * necessary to support health care. 072 * 073 * 074 * UsageNote: V3 Specifications are published in a set of "domains", which 075 * contain interactions and related specifications for a single area of health 076 * care within which can be supported by a single, coherent set of 077 * interoperability specifications. 078 * 079 * For publishing purposes, these domains are aggregated into sub-sections of 080 * related health care areas and these sub-sections are further aggregated into 081 * three major sets. 082 */ 083 IM, 084 /** 085 * Description: Represents the HL7 V3 publishing section that holds 086 * specifications that are unassigned - that have not yet been assigned to one 087 * of the formal publishing sections. 088 * 089 * 090 * UsageNote: V3 Specifications are published in a set of "domains", which 091 * contain interactions and related specifications for a single area of health 092 * care within which can be supported by a single, coherent set of 093 * interoperability specifications. 094 * 095 * For publishing purposes, these domains are aggregated into sub-sections of 096 * related health care areas and these sub-sections are further aggregated into 097 * three major sets. 098 */ 099 UU, 100 /** 101 * added to help the parsers 102 */ 103 NULL; 104 105 public static V3Hl7PublishingSection fromCode(String codeString) throws FHIRException { 106 if (codeString == null || "".equals(codeString)) 107 return null; 108 if ("AM".equals(codeString)) 109 return AM; 110 if ("HM".equals(codeString)) 111 return HM; 112 if ("IM".equals(codeString)) 113 return IM; 114 if ("UU".equals(codeString)) 115 return UU; 116 throw new FHIRException("Unknown V3Hl7PublishingSection code '" + codeString + "'"); 117 } 118 119 public String toCode() { 120 switch (this) { 121 case AM: 122 return "AM"; 123 case HM: 124 return "HM"; 125 case IM: 126 return "IM"; 127 case UU: 128 return "UU"; 129 case NULL: 130 return null; 131 default: 132 return "?"; 133 } 134 } 135 136 public String getSystem() { 137 return "http://terminology.hl7.org/CodeSystem/v3-hl7PublishingSection"; 138 } 139 140 public String getDefinition() { 141 switch (this) { 142 case AM: 143 return "Description: Represents the HL7 V3 publishing section that deals with the administration and management of health care activities and organizations.\r\n\n \n UsageNote: V3 Specifications are published in a set of \"domains\", which contain interactions and related specifications for a single area of health care within which can be supported by a single, coherent set of interoperability specifications.\r\n\n For publishing purposes, these domains are aggregated into sub-sections of related health care areas and these sub-sections are further aggregated into three major sets."; 144 case HM: 145 return "Description: Represents the HL7 V3 publishing section that deals with the health care provision and clinical management.\r\n\n \n UsageNote: V3 Specifications are published in a set of \"domains\", which contain interactions and related specifications for a single area of health care within which can be supported by a single, coherent set of interoperability specifications.\r\n\n For publishing purposes, these domains are aggregated into sub-sections of related health care areas and these sub-sections are further aggregated into three major sets."; 146 case IM: 147 return "Description: Represents the HL7 V3 publishing section that deals with the definition and management of the computing and communication infrastructure necessary to support health care.\r\n\n \n UsageNote: V3 Specifications are published in a set of \"domains\", which contain interactions and related specifications for a single area of health care within which can be supported by a single, coherent set of interoperability specifications.\r\n\n For publishing purposes, these domains are aggregated into sub-sections of related health care areas and these sub-sections are further aggregated into three major sets."; 148 case UU: 149 return "Description: Represents the HL7 V3 publishing section that holds specifications that are unassigned - that have not yet been assigned to one of the formal publishing sections.\r\n\n \n UsageNote: V3 Specifications are published in a set of \"domains\", which contain interactions and related specifications for a single area of health care within which can be supported by a single, coherent set of interoperability specifications.\r\n\n For publishing purposes, these domains are aggregated into sub-sections of related health care areas and these sub-sections are further aggregated into three major sets."; 150 case NULL: 151 return null; 152 default: 153 return "?"; 154 } 155 } 156 157 public String getDisplay() { 158 switch (this) { 159 case AM: 160 return "administrative management"; 161 case HM: 162 return "health and clinical management"; 163 case IM: 164 return "infrastructure management"; 165 case UU: 166 return "unknown"; 167 case NULL: 168 return null; 169 default: 170 return "?"; 171 } 172 } 173 174}