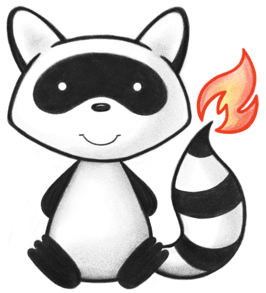
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum V3Hl7V3Conformance { 037 038 /** 039 * Description: Implementers receiving this property must not raise an error if 040 * the data is received, but will not perform any useful function with the data. 041 * This conformance level is not used in profiles or other artifacts that are 042 * specific to the "sender" or "initiator" of a communication. 043 */ 044 I, 045 /** 046 * Description: All implementers are prohibited from transmitting this content, 047 * and may raise an error if they receive it. 048 */ 049 NP, 050 /** 051 * Description: All implementers must support this property. I.e. they must be 052 * able to transmit, or to receive and usefully handle the concept. 053 */ 054 R, 055 /** 056 * Description: The element is considered "required" (i.e. must be supported) 057 * from the perspective of systems that consume instances, but is "undetermined" 058 * for systems that generate instances. Used only as part of specifications that 059 * define both initiator and consumer expectations. 060 */ 061 RC, 062 /** 063 * Description: The element is considered "required" (i.e. must be supported) 064 * from the perspective of systems that generate instances, but is 065 * "undetermined" for systems that consume instances. Used only as part of 066 * specifications that define both initiator and consumer expectations. 067 */ 068 RI, 069 /** 070 * Description: The conformance expectations for this element have not yet been 071 * determined. 072 */ 073 U, 074 /** 075 * added to help the parsers 076 */ 077 NULL; 078 079 public static V3Hl7V3Conformance fromCode(String codeString) throws FHIRException { 080 if (codeString == null || "".equals(codeString)) 081 return null; 082 if ("I".equals(codeString)) 083 return I; 084 if ("NP".equals(codeString)) 085 return NP; 086 if ("R".equals(codeString)) 087 return R; 088 if ("RC".equals(codeString)) 089 return RC; 090 if ("RI".equals(codeString)) 091 return RI; 092 if ("U".equals(codeString)) 093 return U; 094 throw new FHIRException("Unknown V3Hl7V3Conformance code '" + codeString + "'"); 095 } 096 097 public String toCode() { 098 switch (this) { 099 case I: 100 return "I"; 101 case NP: 102 return "NP"; 103 case R: 104 return "R"; 105 case RC: 106 return "RC"; 107 case RI: 108 return "RI"; 109 case U: 110 return "U"; 111 case NULL: 112 return null; 113 default: 114 return "?"; 115 } 116 } 117 118 public String getSystem() { 119 return "http://terminology.hl7.org/CodeSystem/v3-hl7V3Conformance"; 120 } 121 122 public String getDefinition() { 123 switch (this) { 124 case I: 125 return "Description: Implementers receiving this property must not raise an error if the data is received, but will not perform any useful function with the data. This conformance level is not used in profiles or other artifacts that are specific to the \"sender\" or \"initiator\" of a communication."; 126 case NP: 127 return "Description: All implementers are prohibited from transmitting this content, and may raise an error if they receive it."; 128 case R: 129 return "Description: All implementers must support this property. I.e. they must be able to transmit, or to receive and usefully handle the concept."; 130 case RC: 131 return "Description: The element is considered \"required\" (i.e. must be supported) from the perspective of systems that consume instances, but is \"undetermined\" for systems that generate instances. Used only as part of specifications that define both initiator and consumer expectations."; 132 case RI: 133 return "Description: The element is considered \"required\" (i.e. must be supported) from the perspective of systems that generate instances, but is \"undetermined\" for systems that consume instances. Used only as part of specifications that define both initiator and consumer expectations."; 134 case U: 135 return "Description: The conformance expectations for this element have not yet been determined."; 136 case NULL: 137 return null; 138 default: 139 return "?"; 140 } 141 } 142 143 public String getDisplay() { 144 switch (this) { 145 case I: 146 return "ignored"; 147 case NP: 148 return "not permitted"; 149 case R: 150 return "required"; 151 case RC: 152 return "required for consumer"; 153 case RI: 154 return "required for initiator"; 155 case U: 156 return "undetermined"; 157 case NULL: 158 return null; 159 default: 160 return "?"; 161 } 162 } 163 164}