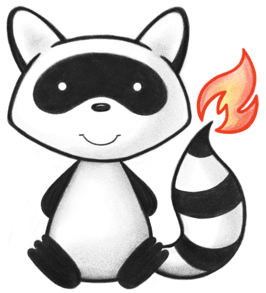
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum V3Hl7VoteResolution { 037 038 /** 039 * Description: An abstract concept grouping resolutions that can be applied to 040 * affirmative ballot comments. 041 */ 042 AFFIRMATIVERESOLUTION, 043 /** 044 * Description: The recommended change has been deferred to consideration for a 045 * future release. 046 */ 047 AFFDEF, 048 /** 049 * Description: The recommended change has been incorporated or identified issue 050 * has been answered. 051 */ 052 AFFI, 053 /** 054 * Description: The recommended change has been refused and is not expected to 055 * be incorporated. 056 */ 057 AFFR, 058 /** 059 * Description: An abstract concept grouping resolutions that can be applied to 060 * negative ballot comments. 061 */ 062 NEGATIVERESOLUTION, 063 /** 064 * Description: Responsible group has recommended that the negative vote be 065 * considered non-substantive. (Issue raised does not provide sufficiently 066 * convincing reason to make changes to the item under ballot, or otherwise 067 * impede its adoption.) 068 */ 069 NONSUBP, 070 /** 071 * Description: Ballot group has voted and declared the negative vote 072 * non-substantive. 073 */ 074 NONSUBV, 075 /** 076 * Description: Responsible group has recommended that the negative vote be 077 * considered not-related. (Issue raised is not related to the current scope of 078 * the item under ballot, or does not prevent the item under ballot for being 079 * used for its defined intent. Recommended changes may be considered as part of 080 * future versions.) (Perhaps after further reading or explanation). 081 */ 082 NOTRELP, 083 /** 084 * Description: Ballot group has voted and declared the negative vote 085 * non-related. 086 */ 087 NOTRELV, 088 /** 089 * Description: Committee identifies that the same issue has been raised as part 090 * of a previous ballot on the same element version and was found by the ballot 091 * group to be non-substantive or not related.) 092 */ 093 PREVCONS, 094 /** 095 * Description: Voter has formally withdrawn their vote or comment as having 096 * been in error. (Perhaps after further reading or explanation). 097 */ 098 RETRACT, 099 /** 100 * Description: Vote has not yet gone through resolution. 101 */ 102 UNRESOLVED, 103 /** 104 * Description: Voter has formally withdrawn their vote or comment on the basis 105 * of agreed changes or proposed future changes. 106 */ 107 WITHDRAW, 108 /** 109 * added to help the parsers 110 */ 111 NULL; 112 113 public static V3Hl7VoteResolution fromCode(String codeString) throws FHIRException { 114 if (codeString == null || "".equals(codeString)) 115 return null; 116 if ("affirmativeResolution".equals(codeString)) 117 return AFFIRMATIVERESOLUTION; 118 if ("affdef".equals(codeString)) 119 return AFFDEF; 120 if ("affi".equals(codeString)) 121 return AFFI; 122 if ("affr".equals(codeString)) 123 return AFFR; 124 if ("negativeResolution".equals(codeString)) 125 return NEGATIVERESOLUTION; 126 if ("nonsubp".equals(codeString)) 127 return NONSUBP; 128 if ("nonsubv".equals(codeString)) 129 return NONSUBV; 130 if ("notrelp".equals(codeString)) 131 return NOTRELP; 132 if ("notrelv".equals(codeString)) 133 return NOTRELV; 134 if ("prevcons".equals(codeString)) 135 return PREVCONS; 136 if ("retract".equals(codeString)) 137 return RETRACT; 138 if ("unresolved".equals(codeString)) 139 return UNRESOLVED; 140 if ("withdraw".equals(codeString)) 141 return WITHDRAW; 142 throw new FHIRException("Unknown V3Hl7VoteResolution code '" + codeString + "'"); 143 } 144 145 public String toCode() { 146 switch (this) { 147 case AFFIRMATIVERESOLUTION: 148 return "affirmativeResolution"; 149 case AFFDEF: 150 return "affdef"; 151 case AFFI: 152 return "affi"; 153 case AFFR: 154 return "affr"; 155 case NEGATIVERESOLUTION: 156 return "negativeResolution"; 157 case NONSUBP: 158 return "nonsubp"; 159 case NONSUBV: 160 return "nonsubv"; 161 case NOTRELP: 162 return "notrelp"; 163 case NOTRELV: 164 return "notrelv"; 165 case PREVCONS: 166 return "prevcons"; 167 case RETRACT: 168 return "retract"; 169 case UNRESOLVED: 170 return "unresolved"; 171 case WITHDRAW: 172 return "withdraw"; 173 case NULL: 174 return null; 175 default: 176 return "?"; 177 } 178 } 179 180 public String getSystem() { 181 return "http://terminology.hl7.org/CodeSystem/v3-hl7VoteResolution"; 182 } 183 184 public String getDefinition() { 185 switch (this) { 186 case AFFIRMATIVERESOLUTION: 187 return "Description: An abstract concept grouping resolutions that can be applied to affirmative ballot comments."; 188 case AFFDEF: 189 return "Description: The recommended change has been deferred to consideration for a future release."; 190 case AFFI: 191 return "Description: The recommended change has been incorporated or identified issue has been answered."; 192 case AFFR: 193 return "Description: The recommended change has been refused and is not expected to be incorporated."; 194 case NEGATIVERESOLUTION: 195 return "Description: An abstract concept grouping resolutions that can be applied to negative ballot comments."; 196 case NONSUBP: 197 return "Description: Responsible group has recommended that the negative vote be considered non-substantive. (Issue raised does not provide sufficiently convincing reason to make changes to the item under ballot, or otherwise impede its adoption.)"; 198 case NONSUBV: 199 return "Description: Ballot group has voted and declared the negative vote non-substantive."; 200 case NOTRELP: 201 return "Description: Responsible group has recommended that the negative vote be considered not-related. (Issue raised is not related to the current scope of the item under ballot, or does not prevent the item under ballot for being used for its defined intent. Recommended changes may be considered as part of future versions.) (Perhaps after further reading or explanation)."; 202 case NOTRELV: 203 return "Description: Ballot group has voted and declared the negative vote non-related."; 204 case PREVCONS: 205 return "Description: Committee identifies that the same issue has been raised as part of a previous ballot on the same element version and was found by the ballot group to be non-substantive or not related.)"; 206 case RETRACT: 207 return "Description: Voter has formally withdrawn their vote or comment as having been in error. (Perhaps after further reading or explanation)."; 208 case UNRESOLVED: 209 return "Description: Vote has not yet gone through resolution."; 210 case WITHDRAW: 211 return "Description: Voter has formally withdrawn their vote or comment on the basis of agreed changes or proposed future changes."; 212 case NULL: 213 return null; 214 default: 215 return "?"; 216 } 217 } 218 219 public String getDisplay() { 220 switch (this) { 221 case AFFIRMATIVERESOLUTION: 222 return "affirmative resolution"; 223 case AFFDEF: 224 return "affirmative-deferred"; 225 case AFFI: 226 return "affirmative-incorporated"; 227 case AFFR: 228 return "affirmative-rejected"; 229 case NEGATIVERESOLUTION: 230 return "negative resolution"; 231 case NONSUBP: 232 return "non-substantive proposed"; 233 case NONSUBV: 234 return "non-substantive voted"; 235 case NOTRELP: 236 return "not related proposed"; 237 case NOTRELV: 238 return "not related voted"; 239 case PREVCONS: 240 return "previously considered"; 241 case RETRACT: 242 return "retracted"; 243 case UNRESOLVED: 244 return "unresolved"; 245 case WITHDRAW: 246 return "withdrawn"; 247 case NULL: 248 return null; 249 default: 250 return "?"; 251 } 252 } 253 254}