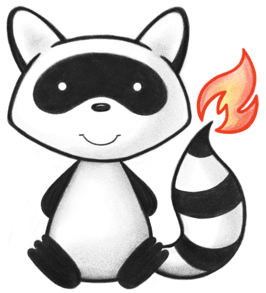
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum V3ObservationMethod { 037 038 /** 039 * Provides codes for decision methods, initially for assessing the causality of 040 * events. 041 */ 042 _DECISIONOBSERVATIONMETHOD, 043 /** 044 * Reaching a decision through the application of an algorithm designed to weigh 045 * the different factors involved. 046 */ 047 ALGM, 048 /** 049 * Reaching a decision through the use of Bayesian statistical analysis. 050 */ 051 BYCL, 052 /** 053 * Reaching a decision by consideration of the totality of factors involved in 054 * order to reach a judgement. 055 */ 056 GINT, 057 /** 058 * A code that provides additional detail about the means or technique used to 059 * ascertain the genetic analysis. Example, PCR, Micro Array 060 */ 061 _GENETICOBSERVATIONMETHOD, 062 /** 063 * Description: Polymerase Chain Reaction 064 */ 065 PCR, 066 /** 067 * Provides additional detail about the aggregation methods used to compute the 068 * aggregated values for an observation. This is an abstract code. 069 */ 070 _OBSERVATIONMETHODAGGREGATE, 071 /** 072 * Average of non-null values in the referenced set of values 073 */ 074 AVERAGE, 075 /** 076 * Count of non-null values in the referenced set of values 077 */ 078 COUNT, 079 /** 080 * Largest of all non-null values in the referenced set of values. 081 */ 082 MAX, 083 /** 084 * The median of all non-null values in the referenced set of values. 085 */ 086 MEDIAN, 087 /** 088 * Smallest of all non-null values in the referenced set of values. 089 */ 090 MIN, 091 /** 092 * The most common value of all non-null values in the referenced set of values. 093 */ 094 MODE, 095 /** 096 * Standard Deviation of the values in the referenced set of values, computed 097 * over the population. 098 */ 099 STDEV_P, 100 /** 101 * Standard Deviation of the values in the referenced set of values, computed 102 * over a sample of the population. 103 */ 104 STDEV_S, 105 /** 106 * Sum of non-null values in the referenced set of values 107 */ 108 SUM, 109 /** 110 * Variance of the values in the referenced set of values, computed over the 111 * population. 112 */ 113 VARIANCE_P, 114 /** 115 * Variance of the values in the referenced set of values, computed over a 116 * sample of the population. 117 */ 118 VARIANCE_S, 119 /** 120 * VerificationMethod 121 */ 122 _VERIFICATIONMETHOD, 123 /** 124 * Verification by means of document. 125 * 126 * 127 * Example: Fax, letter, attachment to e-mail. 128 */ 129 VDOC, 130 /** 131 * verification by means of a response to an electronic query 132 * 133 * 134 * Example: query message to a Covered Party registry application or Coverage 135 * Administrator. 136 */ 137 VREG, 138 /** 139 * Verification by means of electronic token. 140 * 141 * 142 * Example: smartcard, magnetic swipe card, RFID device. 143 */ 144 VTOKEN, 145 /** 146 * Verification by means of voice. 147 * 148 * 149 * Example: By speaking with or calling the Coverage Administrator or Covered 150 * Party 151 */ 152 VVOICE, 153 /** 154 * Complement fixation 155 */ 156 _0001, 157 /** 158 * Computed axial tomography 159 */ 160 _0002, 161 /** 162 * Susceptibility, High Level Aminoglycoside Resistance agar test 163 */ 164 _0003, 165 /** 166 * Visual, Macroscopic observation 167 */ 168 _0004, 169 /** 170 * Computed, Magnetic resonance 171 */ 172 _0005, 173 /** 174 * Computed, Morphometry 175 */ 176 _0006, 177 /** 178 * Computed, Positron emission tomography 179 */ 180 _0007, 181 /** 182 * SAMHSA drug assay confirmation 183 */ 184 _0008, 185 /** 186 * SAMHSA drug assay screening 187 */ 188 _0009, 189 /** 190 * Serum Neutralization 191 */ 192 _0010, 193 /** 194 * Titration 195 */ 196 _0011, 197 /** 198 * Ultrasound 199 */ 200 _0012, 201 /** 202 * X-ray crystallography 203 */ 204 _0013, 205 /** 206 * Agglutination 207 */ 208 _0014, 209 /** 210 * Agglutination, Buffered acidified plate 211 */ 212 _0015, 213 /** 214 * Agglutination, Card 215 */ 216 _0016, 217 /** 218 * Agglutination, Hemagglutination 219 */ 220 _0017, 221 /** 222 * Agglutination, Hemagglutination inhibition 223 */ 224 _0018, 225 /** 226 * Agglutination, Latex 227 */ 228 _0019, 229 /** 230 * Agglutination, Plate 231 */ 232 _0020, 233 /** 234 * Agglutination, Rapid Plate 235 */ 236 _0021, 237 /** 238 * Agglutination, RBC 239 */ 240 _0022, 241 /** 242 * Agglutination, Rivanol 243 */ 244 _0023, 245 /** 246 * Agglutination, Tube 247 */ 248 _0024, 249 /** 250 * Bioassay 251 */ 252 _0025, 253 /** 254 * Bioassay, Animal Inoculation 255 */ 256 _0026, 257 /** 258 * Bioassay, Cytotoxicity 259 */ 260 _0027, 261 /** 262 * Bioassay, Embryo Infective Dose 50 263 */ 264 _0028, 265 /** 266 * Bioassay, Embryo Lethal Dose 50 267 */ 268 _0029, 269 /** 270 * Bioassay, Mouse intercerebral inoculation 271 */ 272 _0030, 273 /** 274 * Bioassay, qualitative 275 */ 276 _0031, 277 /** 278 * Bioassay, quantitative 279 */ 280 _0032, 281 /** 282 * Chemical 283 */ 284 _0033, 285 /** 286 * Chemical, Differential light absorption 287 */ 288 _0034, 289 /** 290 * Chemical, Dipstick 291 */ 292 _0035, 293 /** 294 * Chemical, Dipstick colorimetric laboratory test 295 */ 296 _0036, 297 /** 298 * Chemical, Test strip 299 */ 300 _0037, 301 /** 302 * Chromatography 303 */ 304 _0038, 305 /** 306 * Chromatography, Affinity 307 */ 308 _0039, 309 /** 310 * Chromatography, Gas liquid 311 */ 312 _0040, 313 /** 314 * Chromatography, High performance liquid 315 */ 316 _0041, 317 /** 318 * Chromatography, Liquid 319 */ 320 _0042, 321 /** 322 * Chromatography, Protein A affinity 323 */ 324 _0043, 325 /** 326 * Coagulation 327 */ 328 _0044, 329 /** 330 * Coagulation, Tilt tube 331 */ 332 _0045, 333 /** 334 * Coagulation, Tilt tube reptilase induced 335 */ 336 _0046, 337 /** 338 * Count, Automated 339 */ 340 _0047, 341 /** 342 * Count, Manual 343 */ 344 _0048, 345 /** 346 * Count, Platelet, Rees-Ecker 347 */ 348 _0049, 349 /** 350 * Culture, Aerobic 351 */ 352 _0050, 353 /** 354 * Culture, Anaerobic 355 */ 356 _0051, 357 /** 358 * Culture, Chicken Embryo 359 */ 360 _0052, 361 /** 362 * Culture, Delayed secondary enrichment 363 */ 364 _0053, 365 /** 366 * Culture, Microaerophilic 367 */ 368 _0054, 369 /** 370 * Culture, Quantitative microbial, cup 371 */ 372 _0055, 373 /** 374 * Culture, Quantitative microbial, droplet 375 */ 376 _0056, 377 /** 378 * Culture, Quantitative microbial, filter paper 379 */ 380 _0057, 381 /** 382 * Culture, Quantitative microbial, pad 383 */ 384 _0058, 385 /** 386 * Culture, Quantitative microbial, pour plate 387 */ 388 _0059, 389 /** 390 * Culture, Quantitative microbial, surface streak 391 */ 392 _0060, 393 /** 394 * Culture, Somatic Cell 395 */ 396 _0061, 397 /** 398 * Diffusion, Agar 399 */ 400 _0062, 401 /** 402 * Diffusion, Agar Gel Immunodiffusion 403 */ 404 _0063, 405 /** 406 * Electrophoresis 407 */ 408 _0064, 409 /** 410 * Electrophoresis, Agaorse gel 411 */ 412 _0065, 413 /** 414 * Electrophoresis, citrate agar 415 */ 416 _0066, 417 /** 418 * Electrophoresis, Immuno 419 */ 420 _0067, 421 /** 422 * Electrophoresis, Polyacrylamide gel 423 */ 424 _0068, 425 /** 426 * Electrophoresis, Starch gel 427 */ 428 _0069, 429 /** 430 * ELISA 431 */ 432 _0070, 433 /** 434 * ELISA, antigen capture 435 */ 436 _0071, 437 /** 438 * ELISA, avidin biotin peroxidase complex 439 */ 440 _0072, 441 /** 442 * ELISA, Kinetic 443 */ 444 _0073, 445 /** 446 * ELISA, peroxidase-antiperoxidase 447 */ 448 _0074, 449 /** 450 * Identification, API 20 Strep 451 */ 452 _0075, 453 /** 454 * Identification, API 20A 455 */ 456 _0076, 457 /** 458 * Identification, API 20C AUX 459 */ 460 _0077, 461 /** 462 * Identification, API 20E 463 */ 464 _0078, 465 /** 466 * Identification, API 20NE 467 */ 468 _0079, 469 /** 470 * Identification, API 50 CH 471 */ 472 _0080, 473 /** 474 * Identification, API An-IDENT 475 */ 476 _0081, 477 /** 478 * Identification, API Coryne 479 */ 480 _0082, 481 /** 482 * Identification, API Rapid 20E 483 */ 484 _0083, 485 /** 486 * Identification, API Staph 487 */ 488 _0084, 489 /** 490 * Identification, API ZYM 491 */ 492 _0085, 493 /** 494 * Identification, Bacterial 495 */ 496 _0086, 497 /** 498 * Identification, mini VIDAS 499 */ 500 _0087, 501 /** 502 * Identification, Phage susceptibility typing 503 */ 504 _0088, 505 /** 506 * Identification, Quad-FERM+ 507 */ 508 _0089, 509 /** 510 * Identification, RAPIDEC Staph 511 */ 512 _0090, 513 /** 514 * Identification, Staphaurex 515 */ 516 _0091, 517 /** 518 * Identification, VIDAS 519 */ 520 _0092, 521 /** 522 * Identification, Vitek 523 */ 524 _0093, 525 /** 526 * Identification, VITEK 2 527 */ 528 _0094, 529 /** 530 * Immune stain 531 */ 532 _0095, 533 /** 534 * Immune stain, Immunofluorescent antibody, direct 535 */ 536 _0096, 537 /** 538 * Immune stain, Immunofluorescent antibody, indirect 539 */ 540 _0097, 541 /** 542 * Immune stain, Immunoperoxidase, Avidin-Biotin Complex 543 */ 544 _0098, 545 /** 546 * Immune stain, Immunoperoxidase, Peroxidase anti-peroxidase complex 547 */ 548 _0099, 549 /** 550 * Immune stain, Immunoperoxidase, Protein A-peroxidase complex 551 */ 552 _0100, 553 /** 554 * Immunoassay 555 */ 556 _0101, 557 /** 558 * Immunoassay, qualitative, multiple step 559 */ 560 _0102, 561 /** 562 * Immunoassay, qualitative, single step 563 */ 564 _0103, 565 /** 566 * Immunoassay, Radioimmunoassay 567 */ 568 _0104, 569 /** 570 * Immunoassay, semi-quantitative, multiple step 571 */ 572 _0105, 573 /** 574 * Immunoassay, semi-quantitative, single step 575 */ 576 _0106, 577 /** 578 * Microscopy 579 */ 580 _0107, 581 /** 582 * Microscopy, Darkfield 583 */ 584 _0108, 585 /** 586 * Microscopy, Electron 587 */ 588 _0109, 589 /** 590 * Microscopy, Electron microscopy tomography 591 */ 592 _0110, 593 /** 594 * Microscopy, Electron, negative stain 595 */ 596 _0111, 597 /** 598 * Microscopy, Electron, thick section transmission 599 */ 600 _0112, 601 /** 602 * Microscopy, Electron, thin section transmission 603 */ 604 _0113, 605 /** 606 * Microscopy, Light 607 */ 608 _0114, 609 /** 610 * Microscopy, Polarized light 611 */ 612 _0115, 613 /** 614 * Microscopy, Scanning electron 615 */ 616 _0116, 617 /** 618 * Microscopy, Transmission electron 619 */ 620 _0117, 621 /** 622 * Microscopy, Transparent tape direct examination 623 */ 624 _0118, 625 /** 626 * Molecular, 3 Self-Sustaining Sequence Replication 627 */ 628 _0119, 629 /** 630 * Molecular, Branched Chain DNA 631 */ 632 _0120, 633 /** 634 * Molecular, Hybridization Protection Assay 635 */ 636 _0121, 637 /** 638 * Molecular, Immune blot 639 */ 640 _0122, 641 /** 642 * Molecular, In-situ hybridization 643 */ 644 _0123, 645 /** 646 * Molecular, Ligase Chain Reaction 647 */ 648 _0124, 649 /** 650 * Molecular, Ligation Activated Transcription 651 */ 652 _0125, 653 /** 654 * Molecular, Nucleic Acid Probe 655 */ 656 _0126, 657 /** 658 * Molecular, Nucleic acid probe with amplification 659 * 660 * 661 * 662 * Rationale: Duplicate of code 0126. Use code 0126 instead. 663 */ 664 _0128, 665 /** 666 * Molecular, Nucleic acid probe with target amplification 667 */ 668 _0129, 669 /** 670 * Molecular, Nucleic acid reverse transcription 671 */ 672 _0130, 673 /** 674 * Molecular, Nucleic Acid Sequence Based Analysis 675 */ 676 _0131, 677 /** 678 * Molecular, Polymerase chain reaction 679 */ 680 _0132, 681 /** 682 * Molecular, Q-Beta Replicase or probe amplification category method 683 */ 684 _0133, 685 /** 686 * Molecular, Restriction Fragment Length Polymorphism 687 */ 688 _0134, 689 /** 690 * Molecular, Southern Blot 691 */ 692 _0135, 693 /** 694 * Molecular, Strand Displacement Amplification 695 */ 696 _0136, 697 /** 698 * Molecular, Transcription Mediated Amplification 699 */ 700 _0137, 701 /** 702 * Molecular, Western Blot 703 */ 704 _0138, 705 /** 706 * Precipitation, Flocculation 707 */ 708 _0139, 709 /** 710 * Precipitation, Immune precipitation 711 */ 712 _0140, 713 /** 714 * Precipitation, Milk ring test 715 */ 716 _0141, 717 /** 718 * Precipitation, Precipitin 719 */ 720 _0142, 721 /** 722 * Stain, Acid fast 723 */ 724 _0143, 725 /** 726 * Stain, Acid fast, fluorochrome 727 */ 728 _0144, 729 /** 730 * Stain, Acid fast, Kinyoun's cold carbolfuchsin 731 */ 732 _0145, 733 /** 734 * Stain, Acid fast, Ziehl-Neelsen 735 */ 736 _0146, 737 /** 738 * Stain, Acid phosphatase 739 */ 740 _0147, 741 /** 742 * Stain, Acridine orange 743 */ 744 _0148, 745 /** 746 * Stain, Active brilliant orange KH 747 */ 748 _0149, 749 /** 750 * Stain, Alazarin red S 751 */ 752 _0150, 753 /** 754 * Stain, Alcian blue 755 */ 756 _0151, 757 /** 758 * Stain, Alcian blue with Periodic acid Schiff 759 */ 760 _0152, 761 /** 762 * Stain, Argentaffin 763 */ 764 _0153, 765 /** 766 * Stain, Argentaffin silver 767 */ 768 _0154, 769 /** 770 * Stain, Azure-eosin 771 */ 772 _0155, 773 /** 774 * Stain, Basic Fuschin 775 */ 776 _0156, 777 /** 778 * Stain, Bennhold 779 */ 780 _0157, 781 /** 782 * Stain, Bennhold's Congo red 783 */ 784 _0158, 785 /** 786 * Stain, Bielschowsky 787 */ 788 _0159, 789 /** 790 * Stain, Bielschowsky's silver 791 */ 792 _0160, 793 /** 794 * Stain, Bleach 795 */ 796 _0161, 797 /** 798 * Stain, Bodian 799 */ 800 _0162, 801 /** 802 * Stain, Brown-Brenn 803 */ 804 _0163, 805 /** 806 * Stain, Butyrate-esterase 807 */ 808 _0164, 809 /** 810 * Stain, Calcofluor white fluorescent 811 */ 812 _0165, 813 /** 814 * Stain, Carbol-fuchsin 815 */ 816 _0166, 817 /** 818 * Stain, Carmine 819 */ 820 _0167, 821 /** 822 * Stain, Churukian-Schenk 823 */ 824 _0168, 825 /** 826 * Stain, Congo red 827 */ 828 _0169, 829 /** 830 * Stain, Cresyl echt violet 831 */ 832 _0170, 833 /** 834 * Stain, Crystal violet 835 */ 836 _0171, 837 /** 838 * Stain, De Galantha 839 */ 840 _0172, 841 /** 842 * Stain, Dieterle silver impregnation 843 */ 844 _0173, 845 /** 846 * Stain, Fite-Farco 847 */ 848 _0174, 849 /** 850 * Stain, Fontana-Masson silver 851 */ 852 _0175, 853 /** 854 * Stain, Fouchet 855 */ 856 _0176, 857 /** 858 * Stain, Gomori 859 */ 860 _0177, 861 /** 862 * Stain, Gomori methenamine silver 863 */ 864 _0178, 865 /** 866 * Stain, Gomori-Wheatly trichrome 867 */ 868 _0179, 869 /** 870 * Stain, Gridley 871 */ 872 _0180, 873 /** 874 * Stain, Grimelius silver 875 */ 876 _0181, 877 /** 878 * Stain, Grocott 879 */ 880 _0182, 881 /** 882 * Stain, Grocott methenamine silver 883 */ 884 _0183, 885 /** 886 * Stain, Hale's colloidal ferric oxide 887 */ 888 _0184, 889 /** 890 * Stain, Hale's colloidal iron 891 */ 892 _0185, 893 /** 894 * Stain, Hansel 895 */ 896 _0186, 897 /** 898 * Stain, Harris regressive hematoxylin and eosin 899 */ 900 _0187, 901 /** 902 * Stain, Hematoxylin and eosin 903 */ 904 _0188, 905 /** 906 * Stain, Highman 907 */ 908 _0189, 909 /** 910 * Stain, Holzer 911 */ 912 _0190, 913 /** 914 * Stain, Iron hematoxylin 915 */ 916 _0191, 917 /** 918 * Stain, Jones 919 */ 920 _0192, 921 /** 922 * Stain, Jones methenamine silver 923 */ 924 _0193, 925 /** 926 * Stain, Kossa 927 */ 928 _0194, 929 /** 930 * Stain, Lawson-Van Gieson 931 */ 932 _0195, 933 /** 934 * Stain, Loeffler methylene blue 935 */ 936 _0196, 937 /** 938 * Stain, Luxol fast blue with cresyl violet 939 */ 940 _0197, 941 /** 942 * Stain, Luxol fast blue with Periodic acid-Schiff 943 */ 944 _0198, 945 /** 946 * Stain, MacNeal's tetrachrome blood 947 */ 948 _0199, 949 /** 950 * Stain, Mallory-Heidenhain 951 */ 952 _0200, 953 /** 954 * Stain, Masson trichrome 955 */ 956 _0201, 957 /** 958 * Stain, Mayer mucicarmine 959 */ 960 _0202, 961 /** 962 * Stain, Mayers progressive hematoxylin and eosin 963 */ 964 _0203, 965 /** 966 * Stain, May-Grunwald Giemsa 967 */ 968 _0204, 969 /** 970 * Stain, Methyl green 971 */ 972 _0205, 973 /** 974 * Stain, Methyl green pyronin 975 */ 976 _0206, 977 /** 978 * Stain, Modified Gomori-Wheatly trichrome 979 */ 980 _0207, 981 /** 982 * Stain, Modified Masson trichrome 983 */ 984 _0208, 985 /** 986 * Stain, Modified trichrome 987 */ 988 _0209, 989 /** 990 * Stain, Movat pentachrome 991 */ 992 _0210, 993 /** 994 * Stain, Mucicarmine 995 */ 996 _0211, 997 /** 998 * Stain, Neutral red 999 */ 1000 _0212, 1001 /** 1002 * Stain, Night blue 1003 */ 1004 _0213, 1005 /** 1006 * Stain, Non-specific esterase 1007 */ 1008 _0214, 1009 /** 1010 * Stain, Oil red-O 1011 */ 1012 _0215, 1013 /** 1014 * Stain, Orcein 1015 */ 1016 _0216, 1017 /** 1018 * Stain, Perls' 1019 */ 1020 _0217, 1021 /** 1022 * Stain, Phosphotungstic acid-hematoxylin 1023 */ 1024 _0218, 1025 /** 1026 * Stain, Potassium ferrocyanide 1027 */ 1028 _0219, 1029 /** 1030 * Stain, Prussian blue 1031 */ 1032 _0220, 1033 /** 1034 * Stain, Putchler modified Bennhold 1035 */ 1036 _0221, 1037 /** 1038 * Stain, Quinacrine fluorescent 1039 */ 1040 _0222, 1041 /** 1042 * Stain, Reticulin 1043 */ 1044 _0223, 1045 /** 1046 * Stain, Rhodamine 1047 */ 1048 _0224, 1049 /** 1050 * Stain, Safranin 1051 */ 1052 _0225, 1053 /** 1054 * Stain, Schmorl 1055 */ 1056 _0226, 1057 /** 1058 * Stain, Seiver-Munger 1059 */ 1060 _0227, 1061 /** 1062 * Stain, Silver 1063 */ 1064 _0228, 1065 /** 1066 * Stain, Specific esterase 1067 */ 1068 _0229, 1069 /** 1070 * Stain, Steiner silver 1071 */ 1072 _0230, 1073 /** 1074 * Stain, Sudan III 1075 */ 1076 _0231, 1077 /** 1078 * Stain, Sudan IVI 1079 */ 1080 _0232, 1081 /** 1082 * Stain, Sulfated alcian blue 1083 */ 1084 _0233, 1085 /** 1086 * Stain, Supravital 1087 */ 1088 _0234, 1089 /** 1090 * Stain, Thioflavine-S 1091 */ 1092 _0235, 1093 /** 1094 * Stain, Three micron Giemsa 1095 */ 1096 _0236, 1097 /** 1098 * Stain, Vassar-Culling 1099 */ 1100 _0237, 1101 /** 1102 * Stain, Vital 1103 */ 1104 _0238, 1105 /** 1106 * Stain, von Kossa 1107 */ 1108 _0239, 1109 /** 1110 * Susceptibility, Minimum bactericidal concentration, macrodilution 1111 */ 1112 _0243, 1113 /** 1114 * Susceptibility, Minimum bactericidal concentration, microdilution 1115 */ 1116 _0244, 1117 /** 1118 * Turbidometric 1119 */ 1120 _0247, 1121 /** 1122 * Turbidometric, Refractometric 1123 */ 1124 _0248, 1125 /** 1126 * Chromatography, Thin Layer 1127 */ 1128 _0249, 1129 /** 1130 * Immunoassay, enzyme-multiplied technique (EMIT) 1131 */ 1132 _0250, 1133 /** 1134 * Flow Cytometry 1135 */ 1136 _0251, 1137 /** 1138 * Radial Immunodiffusion 1139 */ 1140 _0252, 1141 /** 1142 * Immunoassay, Fluorescence Polarization 1143 */ 1144 _0253, 1145 /** 1146 * Electrophoresis, Immunofixation 1147 */ 1148 _0254, 1149 /** 1150 * Dialysis, Direct Equilibrium 1151 */ 1152 _0255, 1153 /** 1154 * Acid Elution, Kleihauer-Betke Method 1155 */ 1156 _0256, 1157 /** 1158 * Immunofluorescence, Anti-Complement 1159 */ 1160 _0257, 1161 /** 1162 * Gas Chromatography/Mass Spectroscopy 1163 */ 1164 _0258, 1165 /** 1166 * Light Scatter, Nephelometry 1167 */ 1168 _0259, 1169 /** 1170 * Immunoassay, IgE Antibody Test 1171 */ 1172 _0260, 1173 /** 1174 * Lymphocyte Microcytotoxicity Assay 1175 */ 1176 _0261, 1177 /** 1178 * Spectrophotometry 1179 */ 1180 _0262, 1181 /** 1182 * Spectrophotometry, Atomic Absorption 1183 */ 1184 _0263, 1185 /** 1186 * Electrochemical, Ion Selective Electrode 1187 */ 1188 _0264, 1189 /** 1190 * Chromatography, Gas 1191 */ 1192 _0265, 1193 /** 1194 * Isoelectric Focusing 1195 */ 1196 _0266, 1197 /** 1198 * Immunoassay, Chemiluminescent 1199 */ 1200 _0267, 1201 /** 1202 * Immunoassay, Microparticle Enzyme 1203 */ 1204 _0268, 1205 /** 1206 * Inductively-Coupled Plasma/Mass Spectrometry 1207 */ 1208 _0269, 1209 /** 1210 * Immunoassay, Immunoradiometric Assay 1211 */ 1212 _0270, 1213 /** 1214 * Coagulation, Photo Optical Clot Detection 1215 */ 1216 _0271, 1217 /** 1218 * Test methods designed to determine a microorganismaTMs susceptibility to 1219 * being killed by an antibiotic. 1220 */ 1221 _0280, 1222 /** 1223 * Susceptibility, Antibiotic sensitivity, disk 1224 */ 1225 _0240, 1226 /** 1227 * Susceptibility, BACTEC susceptibility test 1228 */ 1229 _0241, 1230 /** 1231 * Susceptibility, Disk dilution 1232 */ 1233 _0242, 1234 /** 1235 * Testing to measure the minimum concentration of the antibacterial agent in a 1236 * given culture medium below which bacterial growth is not inhibited. 1237 */ 1238 _0272, 1239 /** 1240 * Susceptibility, Minimum Inhibitory concentration, macrodilution 1241 */ 1242 _0245, 1243 /** 1244 * Susceptibility, Minimum Inhibitory concentration, microdilution 1245 */ 1246 _0246, 1247 /** 1248 * Viral Genotype Susceptibility 1249 */ 1250 _0273, 1251 /** 1252 * Viral Phenotype Susceptibility 1253 */ 1254 _0274, 1255 /** 1256 * Gradient Strip 1257 */ 1258 _0275, 1259 /** 1260 * Minimum Lethal Concentration (MLC) 1261 */ 1262 _0275A, 1263 /** 1264 * Testing to measure the minimum concentration of the antibacterial agent in a 1265 * given culture medium below which bacterial growth is not inhibited. 1266 */ 1267 _0276, 1268 /** 1269 * Serum bactericidal titer 1270 */ 1271 _0277, 1272 /** 1273 * Agar screen 1274 */ 1275 _0278, 1276 /** 1277 * Disk induction 1278 */ 1279 _0279, 1280 /** 1281 * Molecular, Nucleic acid probe 1282 */ 1283 _0127, 1284 /** 1285 * added to help the parsers 1286 */ 1287 NULL; 1288 1289 public static V3ObservationMethod fromCode(String codeString) throws FHIRException { 1290 if (codeString == null || "".equals(codeString)) 1291 return null; 1292 if ("_DecisionObservationMethod".equals(codeString)) 1293 return _DECISIONOBSERVATIONMETHOD; 1294 if ("ALGM".equals(codeString)) 1295 return ALGM; 1296 if ("BYCL".equals(codeString)) 1297 return BYCL; 1298 if ("GINT".equals(codeString)) 1299 return GINT; 1300 if ("_GeneticObservationMethod".equals(codeString)) 1301 return _GENETICOBSERVATIONMETHOD; 1302 if ("PCR".equals(codeString)) 1303 return PCR; 1304 if ("_ObservationMethodAggregate".equals(codeString)) 1305 return _OBSERVATIONMETHODAGGREGATE; 1306 if ("AVERAGE".equals(codeString)) 1307 return AVERAGE; 1308 if ("COUNT".equals(codeString)) 1309 return COUNT; 1310 if ("MAX".equals(codeString)) 1311 return MAX; 1312 if ("MEDIAN".equals(codeString)) 1313 return MEDIAN; 1314 if ("MIN".equals(codeString)) 1315 return MIN; 1316 if ("MODE".equals(codeString)) 1317 return MODE; 1318 if ("STDEV.P".equals(codeString)) 1319 return STDEV_P; 1320 if ("STDEV.S".equals(codeString)) 1321 return STDEV_S; 1322 if ("SUM".equals(codeString)) 1323 return SUM; 1324 if ("VARIANCE.P".equals(codeString)) 1325 return VARIANCE_P; 1326 if ("VARIANCE.S".equals(codeString)) 1327 return VARIANCE_S; 1328 if ("_VerificationMethod".equals(codeString)) 1329 return _VERIFICATIONMETHOD; 1330 if ("VDOC".equals(codeString)) 1331 return VDOC; 1332 if ("VREG".equals(codeString)) 1333 return VREG; 1334 if ("VTOKEN".equals(codeString)) 1335 return VTOKEN; 1336 if ("VVOICE".equals(codeString)) 1337 return VVOICE; 1338 if ("0001".equals(codeString)) 1339 return _0001; 1340 if ("0002".equals(codeString)) 1341 return _0002; 1342 if ("0003".equals(codeString)) 1343 return _0003; 1344 if ("0004".equals(codeString)) 1345 return _0004; 1346 if ("0005".equals(codeString)) 1347 return _0005; 1348 if ("0006".equals(codeString)) 1349 return _0006; 1350 if ("0007".equals(codeString)) 1351 return _0007; 1352 if ("0008".equals(codeString)) 1353 return _0008; 1354 if ("0009".equals(codeString)) 1355 return _0009; 1356 if ("0010".equals(codeString)) 1357 return _0010; 1358 if ("0011".equals(codeString)) 1359 return _0011; 1360 if ("0012".equals(codeString)) 1361 return _0012; 1362 if ("0013".equals(codeString)) 1363 return _0013; 1364 if ("0014".equals(codeString)) 1365 return _0014; 1366 if ("0015".equals(codeString)) 1367 return _0015; 1368 if ("0016".equals(codeString)) 1369 return _0016; 1370 if ("0017".equals(codeString)) 1371 return _0017; 1372 if ("0018".equals(codeString)) 1373 return _0018; 1374 if ("0019".equals(codeString)) 1375 return _0019; 1376 if ("0020".equals(codeString)) 1377 return _0020; 1378 if ("0021".equals(codeString)) 1379 return _0021; 1380 if ("0022".equals(codeString)) 1381 return _0022; 1382 if ("0023".equals(codeString)) 1383 return _0023; 1384 if ("0024".equals(codeString)) 1385 return _0024; 1386 if ("0025".equals(codeString)) 1387 return _0025; 1388 if ("0026".equals(codeString)) 1389 return _0026; 1390 if ("0027".equals(codeString)) 1391 return _0027; 1392 if ("0028".equals(codeString)) 1393 return _0028; 1394 if ("0029".equals(codeString)) 1395 return _0029; 1396 if ("0030".equals(codeString)) 1397 return _0030; 1398 if ("0031".equals(codeString)) 1399 return _0031; 1400 if ("0032".equals(codeString)) 1401 return _0032; 1402 if ("0033".equals(codeString)) 1403 return _0033; 1404 if ("0034".equals(codeString)) 1405 return _0034; 1406 if ("0035".equals(codeString)) 1407 return _0035; 1408 if ("0036".equals(codeString)) 1409 return _0036; 1410 if ("0037".equals(codeString)) 1411 return _0037; 1412 if ("0038".equals(codeString)) 1413 return _0038; 1414 if ("0039".equals(codeString)) 1415 return _0039; 1416 if ("0040".equals(codeString)) 1417 return _0040; 1418 if ("0041".equals(codeString)) 1419 return _0041; 1420 if ("0042".equals(codeString)) 1421 return _0042; 1422 if ("0043".equals(codeString)) 1423 return _0043; 1424 if ("0044".equals(codeString)) 1425 return _0044; 1426 if ("0045".equals(codeString)) 1427 return _0045; 1428 if ("0046".equals(codeString)) 1429 return _0046; 1430 if ("0047".equals(codeString)) 1431 return _0047; 1432 if ("0048".equals(codeString)) 1433 return _0048; 1434 if ("0049".equals(codeString)) 1435 return _0049; 1436 if ("0050".equals(codeString)) 1437 return _0050; 1438 if ("0051".equals(codeString)) 1439 return _0051; 1440 if ("0052".equals(codeString)) 1441 return _0052; 1442 if ("0053".equals(codeString)) 1443 return _0053; 1444 if ("0054".equals(codeString)) 1445 return _0054; 1446 if ("0055".equals(codeString)) 1447 return _0055; 1448 if ("0056".equals(codeString)) 1449 return _0056; 1450 if ("0057".equals(codeString)) 1451 return _0057; 1452 if ("0058".equals(codeString)) 1453 return _0058; 1454 if ("0059".equals(codeString)) 1455 return _0059; 1456 if ("0060".equals(codeString)) 1457 return _0060; 1458 if ("0061".equals(codeString)) 1459 return _0061; 1460 if ("0062".equals(codeString)) 1461 return _0062; 1462 if ("0063".equals(codeString)) 1463 return _0063; 1464 if ("0064".equals(codeString)) 1465 return _0064; 1466 if ("0065".equals(codeString)) 1467 return _0065; 1468 if ("0066".equals(codeString)) 1469 return _0066; 1470 if ("0067".equals(codeString)) 1471 return _0067; 1472 if ("0068".equals(codeString)) 1473 return _0068; 1474 if ("0069".equals(codeString)) 1475 return _0069; 1476 if ("0070".equals(codeString)) 1477 return _0070; 1478 if ("0071".equals(codeString)) 1479 return _0071; 1480 if ("0072".equals(codeString)) 1481 return _0072; 1482 if ("0073".equals(codeString)) 1483 return _0073; 1484 if ("0074".equals(codeString)) 1485 return _0074; 1486 if ("0075".equals(codeString)) 1487 return _0075; 1488 if ("0076".equals(codeString)) 1489 return _0076; 1490 if ("0077".equals(codeString)) 1491 return _0077; 1492 if ("0078".equals(codeString)) 1493 return _0078; 1494 if ("0079".equals(codeString)) 1495 return _0079; 1496 if ("0080".equals(codeString)) 1497 return _0080; 1498 if ("0081".equals(codeString)) 1499 return _0081; 1500 if ("0082".equals(codeString)) 1501 return _0082; 1502 if ("0083".equals(codeString)) 1503 return _0083; 1504 if ("0084".equals(codeString)) 1505 return _0084; 1506 if ("0085".equals(codeString)) 1507 return _0085; 1508 if ("0086".equals(codeString)) 1509 return _0086; 1510 if ("0087".equals(codeString)) 1511 return _0087; 1512 if ("0088".equals(codeString)) 1513 return _0088; 1514 if ("0089".equals(codeString)) 1515 return _0089; 1516 if ("0090".equals(codeString)) 1517 return _0090; 1518 if ("0091".equals(codeString)) 1519 return _0091; 1520 if ("0092".equals(codeString)) 1521 return _0092; 1522 if ("0093".equals(codeString)) 1523 return _0093; 1524 if ("0094".equals(codeString)) 1525 return _0094; 1526 if ("0095".equals(codeString)) 1527 return _0095; 1528 if ("0096".equals(codeString)) 1529 return _0096; 1530 if ("0097".equals(codeString)) 1531 return _0097; 1532 if ("0098".equals(codeString)) 1533 return _0098; 1534 if ("0099".equals(codeString)) 1535 return _0099; 1536 if ("0100".equals(codeString)) 1537 return _0100; 1538 if ("0101".equals(codeString)) 1539 return _0101; 1540 if ("0102".equals(codeString)) 1541 return _0102; 1542 if ("0103".equals(codeString)) 1543 return _0103; 1544 if ("0104".equals(codeString)) 1545 return _0104; 1546 if ("0105".equals(codeString)) 1547 return _0105; 1548 if ("0106".equals(codeString)) 1549 return _0106; 1550 if ("0107".equals(codeString)) 1551 return _0107; 1552 if ("0108".equals(codeString)) 1553 return _0108; 1554 if ("0109".equals(codeString)) 1555 return _0109; 1556 if ("0110".equals(codeString)) 1557 return _0110; 1558 if ("0111".equals(codeString)) 1559 return _0111; 1560 if ("0112".equals(codeString)) 1561 return _0112; 1562 if ("0113".equals(codeString)) 1563 return _0113; 1564 if ("0114".equals(codeString)) 1565 return _0114; 1566 if ("0115".equals(codeString)) 1567 return _0115; 1568 if ("0116".equals(codeString)) 1569 return _0116; 1570 if ("0117".equals(codeString)) 1571 return _0117; 1572 if ("0118".equals(codeString)) 1573 return _0118; 1574 if ("0119".equals(codeString)) 1575 return _0119; 1576 if ("0120".equals(codeString)) 1577 return _0120; 1578 if ("0121".equals(codeString)) 1579 return _0121; 1580 if ("0122".equals(codeString)) 1581 return _0122; 1582 if ("0123".equals(codeString)) 1583 return _0123; 1584 if ("0124".equals(codeString)) 1585 return _0124; 1586 if ("0125".equals(codeString)) 1587 return _0125; 1588 if ("0126".equals(codeString)) 1589 return _0126; 1590 if ("0128".equals(codeString)) 1591 return _0128; 1592 if ("0129".equals(codeString)) 1593 return _0129; 1594 if ("0130".equals(codeString)) 1595 return _0130; 1596 if ("0131".equals(codeString)) 1597 return _0131; 1598 if ("0132".equals(codeString)) 1599 return _0132; 1600 if ("0133".equals(codeString)) 1601 return _0133; 1602 if ("0134".equals(codeString)) 1603 return _0134; 1604 if ("0135".equals(codeString)) 1605 return _0135; 1606 if ("0136".equals(codeString)) 1607 return _0136; 1608 if ("0137".equals(codeString)) 1609 return _0137; 1610 if ("0138".equals(codeString)) 1611 return _0138; 1612 if ("0139".equals(codeString)) 1613 return _0139; 1614 if ("0140".equals(codeString)) 1615 return _0140; 1616 if ("0141".equals(codeString)) 1617 return _0141; 1618 if ("0142".equals(codeString)) 1619 return _0142; 1620 if ("0143".equals(codeString)) 1621 return _0143; 1622 if ("0144".equals(codeString)) 1623 return _0144; 1624 if ("0145".equals(codeString)) 1625 return _0145; 1626 if ("0146".equals(codeString)) 1627 return _0146; 1628 if ("0147".equals(codeString)) 1629 return _0147; 1630 if ("0148".equals(codeString)) 1631 return _0148; 1632 if ("0149".equals(codeString)) 1633 return _0149; 1634 if ("0150".equals(codeString)) 1635 return _0150; 1636 if ("0151".equals(codeString)) 1637 return _0151; 1638 if ("0152".equals(codeString)) 1639 return _0152; 1640 if ("0153".equals(codeString)) 1641 return _0153; 1642 if ("0154".equals(codeString)) 1643 return _0154; 1644 if ("0155".equals(codeString)) 1645 return _0155; 1646 if ("0156".equals(codeString)) 1647 return _0156; 1648 if ("0157".equals(codeString)) 1649 return _0157; 1650 if ("0158".equals(codeString)) 1651 return _0158; 1652 if ("0159".equals(codeString)) 1653 return _0159; 1654 if ("0160".equals(codeString)) 1655 return _0160; 1656 if ("0161".equals(codeString)) 1657 return _0161; 1658 if ("0162".equals(codeString)) 1659 return _0162; 1660 if ("0163".equals(codeString)) 1661 return _0163; 1662 if ("0164".equals(codeString)) 1663 return _0164; 1664 if ("0165".equals(codeString)) 1665 return _0165; 1666 if ("0166".equals(codeString)) 1667 return _0166; 1668 if ("0167".equals(codeString)) 1669 return _0167; 1670 if ("0168".equals(codeString)) 1671 return _0168; 1672 if ("0169".equals(codeString)) 1673 return _0169; 1674 if ("0170".equals(codeString)) 1675 return _0170; 1676 if ("0171".equals(codeString)) 1677 return _0171; 1678 if ("0172".equals(codeString)) 1679 return _0172; 1680 if ("0173".equals(codeString)) 1681 return _0173; 1682 if ("0174".equals(codeString)) 1683 return _0174; 1684 if ("0175".equals(codeString)) 1685 return _0175; 1686 if ("0176".equals(codeString)) 1687 return _0176; 1688 if ("0177".equals(codeString)) 1689 return _0177; 1690 if ("0178".equals(codeString)) 1691 return _0178; 1692 if ("0179".equals(codeString)) 1693 return _0179; 1694 if ("0180".equals(codeString)) 1695 return _0180; 1696 if ("0181".equals(codeString)) 1697 return _0181; 1698 if ("0182".equals(codeString)) 1699 return _0182; 1700 if ("0183".equals(codeString)) 1701 return _0183; 1702 if ("0184".equals(codeString)) 1703 return _0184; 1704 if ("0185".equals(codeString)) 1705 return _0185; 1706 if ("0186".equals(codeString)) 1707 return _0186; 1708 if ("0187".equals(codeString)) 1709 return _0187; 1710 if ("0188".equals(codeString)) 1711 return _0188; 1712 if ("0189".equals(codeString)) 1713 return _0189; 1714 if ("0190".equals(codeString)) 1715 return _0190; 1716 if ("0191".equals(codeString)) 1717 return _0191; 1718 if ("0192".equals(codeString)) 1719 return _0192; 1720 if ("0193".equals(codeString)) 1721 return _0193; 1722 if ("0194".equals(codeString)) 1723 return _0194; 1724 if ("0195".equals(codeString)) 1725 return _0195; 1726 if ("0196".equals(codeString)) 1727 return _0196; 1728 if ("0197".equals(codeString)) 1729 return _0197; 1730 if ("0198".equals(codeString)) 1731 return _0198; 1732 if ("0199".equals(codeString)) 1733 return _0199; 1734 if ("0200".equals(codeString)) 1735 return _0200; 1736 if ("0201".equals(codeString)) 1737 return _0201; 1738 if ("0202".equals(codeString)) 1739 return _0202; 1740 if ("0203".equals(codeString)) 1741 return _0203; 1742 if ("0204".equals(codeString)) 1743 return _0204; 1744 if ("0205".equals(codeString)) 1745 return _0205; 1746 if ("0206".equals(codeString)) 1747 return _0206; 1748 if ("0207".equals(codeString)) 1749 return _0207; 1750 if ("0208".equals(codeString)) 1751 return _0208; 1752 if ("0209".equals(codeString)) 1753 return _0209; 1754 if ("0210".equals(codeString)) 1755 return _0210; 1756 if ("0211".equals(codeString)) 1757 return _0211; 1758 if ("0212".equals(codeString)) 1759 return _0212; 1760 if ("0213".equals(codeString)) 1761 return _0213; 1762 if ("0214".equals(codeString)) 1763 return _0214; 1764 if ("0215".equals(codeString)) 1765 return _0215; 1766 if ("0216".equals(codeString)) 1767 return _0216; 1768 if ("0217".equals(codeString)) 1769 return _0217; 1770 if ("0218".equals(codeString)) 1771 return _0218; 1772 if ("0219".equals(codeString)) 1773 return _0219; 1774 if ("0220".equals(codeString)) 1775 return _0220; 1776 if ("0221".equals(codeString)) 1777 return _0221; 1778 if ("0222".equals(codeString)) 1779 return _0222; 1780 if ("0223".equals(codeString)) 1781 return _0223; 1782 if ("0224".equals(codeString)) 1783 return _0224; 1784 if ("0225".equals(codeString)) 1785 return _0225; 1786 if ("0226".equals(codeString)) 1787 return _0226; 1788 if ("0227".equals(codeString)) 1789 return _0227; 1790 if ("0228".equals(codeString)) 1791 return _0228; 1792 if ("0229".equals(codeString)) 1793 return _0229; 1794 if ("0230".equals(codeString)) 1795 return _0230; 1796 if ("0231".equals(codeString)) 1797 return _0231; 1798 if ("0232".equals(codeString)) 1799 return _0232; 1800 if ("0233".equals(codeString)) 1801 return _0233; 1802 if ("0234".equals(codeString)) 1803 return _0234; 1804 if ("0235".equals(codeString)) 1805 return _0235; 1806 if ("0236".equals(codeString)) 1807 return _0236; 1808 if ("0237".equals(codeString)) 1809 return _0237; 1810 if ("0238".equals(codeString)) 1811 return _0238; 1812 if ("0239".equals(codeString)) 1813 return _0239; 1814 if ("0243".equals(codeString)) 1815 return _0243; 1816 if ("0244".equals(codeString)) 1817 return _0244; 1818 if ("0247".equals(codeString)) 1819 return _0247; 1820 if ("0248".equals(codeString)) 1821 return _0248; 1822 if ("0249".equals(codeString)) 1823 return _0249; 1824 if ("0250".equals(codeString)) 1825 return _0250; 1826 if ("0251".equals(codeString)) 1827 return _0251; 1828 if ("0252".equals(codeString)) 1829 return _0252; 1830 if ("0253".equals(codeString)) 1831 return _0253; 1832 if ("0254".equals(codeString)) 1833 return _0254; 1834 if ("0255".equals(codeString)) 1835 return _0255; 1836 if ("0256".equals(codeString)) 1837 return _0256; 1838 if ("0257".equals(codeString)) 1839 return _0257; 1840 if ("0258".equals(codeString)) 1841 return _0258; 1842 if ("0259".equals(codeString)) 1843 return _0259; 1844 if ("0260".equals(codeString)) 1845 return _0260; 1846 if ("0261".equals(codeString)) 1847 return _0261; 1848 if ("0262".equals(codeString)) 1849 return _0262; 1850 if ("0263".equals(codeString)) 1851 return _0263; 1852 if ("0264".equals(codeString)) 1853 return _0264; 1854 if ("0265".equals(codeString)) 1855 return _0265; 1856 if ("0266".equals(codeString)) 1857 return _0266; 1858 if ("0267".equals(codeString)) 1859 return _0267; 1860 if ("0268".equals(codeString)) 1861 return _0268; 1862 if ("0269".equals(codeString)) 1863 return _0269; 1864 if ("0270".equals(codeString)) 1865 return _0270; 1866 if ("0271".equals(codeString)) 1867 return _0271; 1868 if ("0280".equals(codeString)) 1869 return _0280; 1870 if ("0240".equals(codeString)) 1871 return _0240; 1872 if ("0241".equals(codeString)) 1873 return _0241; 1874 if ("0242".equals(codeString)) 1875 return _0242; 1876 if ("0272".equals(codeString)) 1877 return _0272; 1878 if ("0245".equals(codeString)) 1879 return _0245; 1880 if ("0246".equals(codeString)) 1881 return _0246; 1882 if ("0273".equals(codeString)) 1883 return _0273; 1884 if ("0274".equals(codeString)) 1885 return _0274; 1886 if ("0275".equals(codeString)) 1887 return _0275; 1888 if ("0275a".equals(codeString)) 1889 return _0275A; 1890 if ("0276".equals(codeString)) 1891 return _0276; 1892 if ("0277".equals(codeString)) 1893 return _0277; 1894 if ("0278".equals(codeString)) 1895 return _0278; 1896 if ("0279".equals(codeString)) 1897 return _0279; 1898 if ("0127".equals(codeString)) 1899 return _0127; 1900 throw new FHIRException("Unknown V3ObservationMethod code '" + codeString + "'"); 1901 } 1902 1903 public String toCode() { 1904 switch (this) { 1905 case _DECISIONOBSERVATIONMETHOD: 1906 return "_DecisionObservationMethod"; 1907 case ALGM: 1908 return "ALGM"; 1909 case BYCL: 1910 return "BYCL"; 1911 case GINT: 1912 return "GINT"; 1913 case _GENETICOBSERVATIONMETHOD: 1914 return "_GeneticObservationMethod"; 1915 case PCR: 1916 return "PCR"; 1917 case _OBSERVATIONMETHODAGGREGATE: 1918 return "_ObservationMethodAggregate"; 1919 case AVERAGE: 1920 return "AVERAGE"; 1921 case COUNT: 1922 return "COUNT"; 1923 case MAX: 1924 return "MAX"; 1925 case MEDIAN: 1926 return "MEDIAN"; 1927 case MIN: 1928 return "MIN"; 1929 case MODE: 1930 return "MODE"; 1931 case STDEV_P: 1932 return "STDEV.P"; 1933 case STDEV_S: 1934 return "STDEV.S"; 1935 case SUM: 1936 return "SUM"; 1937 case VARIANCE_P: 1938 return "VARIANCE.P"; 1939 case VARIANCE_S: 1940 return "VARIANCE.S"; 1941 case _VERIFICATIONMETHOD: 1942 return "_VerificationMethod"; 1943 case VDOC: 1944 return "VDOC"; 1945 case VREG: 1946 return "VREG"; 1947 case VTOKEN: 1948 return "VTOKEN"; 1949 case VVOICE: 1950 return "VVOICE"; 1951 case _0001: 1952 return "0001"; 1953 case _0002: 1954 return "0002"; 1955 case _0003: 1956 return "0003"; 1957 case _0004: 1958 return "0004"; 1959 case _0005: 1960 return "0005"; 1961 case _0006: 1962 return "0006"; 1963 case _0007: 1964 return "0007"; 1965 case _0008: 1966 return "0008"; 1967 case _0009: 1968 return "0009"; 1969 case _0010: 1970 return "0010"; 1971 case _0011: 1972 return "0011"; 1973 case _0012: 1974 return "0012"; 1975 case _0013: 1976 return "0013"; 1977 case _0014: 1978 return "0014"; 1979 case _0015: 1980 return "0015"; 1981 case _0016: 1982 return "0016"; 1983 case _0017: 1984 return "0017"; 1985 case _0018: 1986 return "0018"; 1987 case _0019: 1988 return "0019"; 1989 case _0020: 1990 return "0020"; 1991 case _0021: 1992 return "0021"; 1993 case _0022: 1994 return "0022"; 1995 case _0023: 1996 return "0023"; 1997 case _0024: 1998 return "0024"; 1999 case _0025: 2000 return "0025"; 2001 case _0026: 2002 return "0026"; 2003 case _0027: 2004 return "0027"; 2005 case _0028: 2006 return "0028"; 2007 case _0029: 2008 return "0029"; 2009 case _0030: 2010 return "0030"; 2011 case _0031: 2012 return "0031"; 2013 case _0032: 2014 return "0032"; 2015 case _0033: 2016 return "0033"; 2017 case _0034: 2018 return "0034"; 2019 case _0035: 2020 return "0035"; 2021 case _0036: 2022 return "0036"; 2023 case _0037: 2024 return "0037"; 2025 case _0038: 2026 return "0038"; 2027 case _0039: 2028 return "0039"; 2029 case _0040: 2030 return "0040"; 2031 case _0041: 2032 return "0041"; 2033 case _0042: 2034 return "0042"; 2035 case _0043: 2036 return "0043"; 2037 case _0044: 2038 return "0044"; 2039 case _0045: 2040 return "0045"; 2041 case _0046: 2042 return "0046"; 2043 case _0047: 2044 return "0047"; 2045 case _0048: 2046 return "0048"; 2047 case _0049: 2048 return "0049"; 2049 case _0050: 2050 return "0050"; 2051 case _0051: 2052 return "0051"; 2053 case _0052: 2054 return "0052"; 2055 case _0053: 2056 return "0053"; 2057 case _0054: 2058 return "0054"; 2059 case _0055: 2060 return "0055"; 2061 case _0056: 2062 return "0056"; 2063 case _0057: 2064 return "0057"; 2065 case _0058: 2066 return "0058"; 2067 case _0059: 2068 return "0059"; 2069 case _0060: 2070 return "0060"; 2071 case _0061: 2072 return "0061"; 2073 case _0062: 2074 return "0062"; 2075 case _0063: 2076 return "0063"; 2077 case _0064: 2078 return "0064"; 2079 case _0065: 2080 return "0065"; 2081 case _0066: 2082 return "0066"; 2083 case _0067: 2084 return "0067"; 2085 case _0068: 2086 return "0068"; 2087 case _0069: 2088 return "0069"; 2089 case _0070: 2090 return "0070"; 2091 case _0071: 2092 return "0071"; 2093 case _0072: 2094 return "0072"; 2095 case _0073: 2096 return "0073"; 2097 case _0074: 2098 return "0074"; 2099 case _0075: 2100 return "0075"; 2101 case _0076: 2102 return "0076"; 2103 case _0077: 2104 return "0077"; 2105 case _0078: 2106 return "0078"; 2107 case _0079: 2108 return "0079"; 2109 case _0080: 2110 return "0080"; 2111 case _0081: 2112 return "0081"; 2113 case _0082: 2114 return "0082"; 2115 case _0083: 2116 return "0083"; 2117 case _0084: 2118 return "0084"; 2119 case _0085: 2120 return "0085"; 2121 case _0086: 2122 return "0086"; 2123 case _0087: 2124 return "0087"; 2125 case _0088: 2126 return "0088"; 2127 case _0089: 2128 return "0089"; 2129 case _0090: 2130 return "0090"; 2131 case _0091: 2132 return "0091"; 2133 case _0092: 2134 return "0092"; 2135 case _0093: 2136 return "0093"; 2137 case _0094: 2138 return "0094"; 2139 case _0095: 2140 return "0095"; 2141 case _0096: 2142 return "0096"; 2143 case _0097: 2144 return "0097"; 2145 case _0098: 2146 return "0098"; 2147 case _0099: 2148 return "0099"; 2149 case _0100: 2150 return "0100"; 2151 case _0101: 2152 return "0101"; 2153 case _0102: 2154 return "0102"; 2155 case _0103: 2156 return "0103"; 2157 case _0104: 2158 return "0104"; 2159 case _0105: 2160 return "0105"; 2161 case _0106: 2162 return "0106"; 2163 case _0107: 2164 return "0107"; 2165 case _0108: 2166 return "0108"; 2167 case _0109: 2168 return "0109"; 2169 case _0110: 2170 return "0110"; 2171 case _0111: 2172 return "0111"; 2173 case _0112: 2174 return "0112"; 2175 case _0113: 2176 return "0113"; 2177 case _0114: 2178 return "0114"; 2179 case _0115: 2180 return "0115"; 2181 case _0116: 2182 return "0116"; 2183 case _0117: 2184 return "0117"; 2185 case _0118: 2186 return "0118"; 2187 case _0119: 2188 return "0119"; 2189 case _0120: 2190 return "0120"; 2191 case _0121: 2192 return "0121"; 2193 case _0122: 2194 return "0122"; 2195 case _0123: 2196 return "0123"; 2197 case _0124: 2198 return "0124"; 2199 case _0125: 2200 return "0125"; 2201 case _0126: 2202 return "0126"; 2203 case _0128: 2204 return "0128"; 2205 case _0129: 2206 return "0129"; 2207 case _0130: 2208 return "0130"; 2209 case _0131: 2210 return "0131"; 2211 case _0132: 2212 return "0132"; 2213 case _0133: 2214 return "0133"; 2215 case _0134: 2216 return "0134"; 2217 case _0135: 2218 return "0135"; 2219 case _0136: 2220 return "0136"; 2221 case _0137: 2222 return "0137"; 2223 case _0138: 2224 return "0138"; 2225 case _0139: 2226 return "0139"; 2227 case _0140: 2228 return "0140"; 2229 case _0141: 2230 return "0141"; 2231 case _0142: 2232 return "0142"; 2233 case _0143: 2234 return "0143"; 2235 case _0144: 2236 return "0144"; 2237 case _0145: 2238 return "0145"; 2239 case _0146: 2240 return "0146"; 2241 case _0147: 2242 return "0147"; 2243 case _0148: 2244 return "0148"; 2245 case _0149: 2246 return "0149"; 2247 case _0150: 2248 return "0150"; 2249 case _0151: 2250 return "0151"; 2251 case _0152: 2252 return "0152"; 2253 case _0153: 2254 return "0153"; 2255 case _0154: 2256 return "0154"; 2257 case _0155: 2258 return "0155"; 2259 case _0156: 2260 return "0156"; 2261 case _0157: 2262 return "0157"; 2263 case _0158: 2264 return "0158"; 2265 case _0159: 2266 return "0159"; 2267 case _0160: 2268 return "0160"; 2269 case _0161: 2270 return "0161"; 2271 case _0162: 2272 return "0162"; 2273 case _0163: 2274 return "0163"; 2275 case _0164: 2276 return "0164"; 2277 case _0165: 2278 return "0165"; 2279 case _0166: 2280 return "0166"; 2281 case _0167: 2282 return "0167"; 2283 case _0168: 2284 return "0168"; 2285 case _0169: 2286 return "0169"; 2287 case _0170: 2288 return "0170"; 2289 case _0171: 2290 return "0171"; 2291 case _0172: 2292 return "0172"; 2293 case _0173: 2294 return "0173"; 2295 case _0174: 2296 return "0174"; 2297 case _0175: 2298 return "0175"; 2299 case _0176: 2300 return "0176"; 2301 case _0177: 2302 return "0177"; 2303 case _0178: 2304 return "0178"; 2305 case _0179: 2306 return "0179"; 2307 case _0180: 2308 return "0180"; 2309 case _0181: 2310 return "0181"; 2311 case _0182: 2312 return "0182"; 2313 case _0183: 2314 return "0183"; 2315 case _0184: 2316 return "0184"; 2317 case _0185: 2318 return "0185"; 2319 case _0186: 2320 return "0186"; 2321 case _0187: 2322 return "0187"; 2323 case _0188: 2324 return "0188"; 2325 case _0189: 2326 return "0189"; 2327 case _0190: 2328 return "0190"; 2329 case _0191: 2330 return "0191"; 2331 case _0192: 2332 return "0192"; 2333 case _0193: 2334 return "0193"; 2335 case _0194: 2336 return "0194"; 2337 case _0195: 2338 return "0195"; 2339 case _0196: 2340 return "0196"; 2341 case _0197: 2342 return "0197"; 2343 case _0198: 2344 return "0198"; 2345 case _0199: 2346 return "0199"; 2347 case _0200: 2348 return "0200"; 2349 case _0201: 2350 return "0201"; 2351 case _0202: 2352 return "0202"; 2353 case _0203: 2354 return "0203"; 2355 case _0204: 2356 return "0204"; 2357 case _0205: 2358 return "0205"; 2359 case _0206: 2360 return "0206"; 2361 case _0207: 2362 return "0207"; 2363 case _0208: 2364 return "0208"; 2365 case _0209: 2366 return "0209"; 2367 case _0210: 2368 return "0210"; 2369 case _0211: 2370 return "0211"; 2371 case _0212: 2372 return "0212"; 2373 case _0213: 2374 return "0213"; 2375 case _0214: 2376 return "0214"; 2377 case _0215: 2378 return "0215"; 2379 case _0216: 2380 return "0216"; 2381 case _0217: 2382 return "0217"; 2383 case _0218: 2384 return "0218"; 2385 case _0219: 2386 return "0219"; 2387 case _0220: 2388 return "0220"; 2389 case _0221: 2390 return "0221"; 2391 case _0222: 2392 return "0222"; 2393 case _0223: 2394 return "0223"; 2395 case _0224: 2396 return "0224"; 2397 case _0225: 2398 return "0225"; 2399 case _0226: 2400 return "0226"; 2401 case _0227: 2402 return "0227"; 2403 case _0228: 2404 return "0228"; 2405 case _0229: 2406 return "0229"; 2407 case _0230: 2408 return "0230"; 2409 case _0231: 2410 return "0231"; 2411 case _0232: 2412 return "0232"; 2413 case _0233: 2414 return "0233"; 2415 case _0234: 2416 return "0234"; 2417 case _0235: 2418 return "0235"; 2419 case _0236: 2420 return "0236"; 2421 case _0237: 2422 return "0237"; 2423 case _0238: 2424 return "0238"; 2425 case _0239: 2426 return "0239"; 2427 case _0243: 2428 return "0243"; 2429 case _0244: 2430 return "0244"; 2431 case _0247: 2432 return "0247"; 2433 case _0248: 2434 return "0248"; 2435 case _0249: 2436 return "0249"; 2437 case _0250: 2438 return "0250"; 2439 case _0251: 2440 return "0251"; 2441 case _0252: 2442 return "0252"; 2443 case _0253: 2444 return "0253"; 2445 case _0254: 2446 return "0254"; 2447 case _0255: 2448 return "0255"; 2449 case _0256: 2450 return "0256"; 2451 case _0257: 2452 return "0257"; 2453 case _0258: 2454 return "0258"; 2455 case _0259: 2456 return "0259"; 2457 case _0260: 2458 return "0260"; 2459 case _0261: 2460 return "0261"; 2461 case _0262: 2462 return "0262"; 2463 case _0263: 2464 return "0263"; 2465 case _0264: 2466 return "0264"; 2467 case _0265: 2468 return "0265"; 2469 case _0266: 2470 return "0266"; 2471 case _0267: 2472 return "0267"; 2473 case _0268: 2474 return "0268"; 2475 case _0269: 2476 return "0269"; 2477 case _0270: 2478 return "0270"; 2479 case _0271: 2480 return "0271"; 2481 case _0280: 2482 return "0280"; 2483 case _0240: 2484 return "0240"; 2485 case _0241: 2486 return "0241"; 2487 case _0242: 2488 return "0242"; 2489 case _0272: 2490 return "0272"; 2491 case _0245: 2492 return "0245"; 2493 case _0246: 2494 return "0246"; 2495 case _0273: 2496 return "0273"; 2497 case _0274: 2498 return "0274"; 2499 case _0275: 2500 return "0275"; 2501 case _0275A: 2502 return "0275a"; 2503 case _0276: 2504 return "0276"; 2505 case _0277: 2506 return "0277"; 2507 case _0278: 2508 return "0278"; 2509 case _0279: 2510 return "0279"; 2511 case _0127: 2512 return "0127"; 2513 case NULL: 2514 return null; 2515 default: 2516 return "?"; 2517 } 2518 } 2519 2520 public String getSystem() { 2521 return "http://terminology.hl7.org/CodeSystem/v3-ObservationMethod"; 2522 } 2523 2524 public String getDefinition() { 2525 switch (this) { 2526 case _DECISIONOBSERVATIONMETHOD: 2527 return "Provides codes for decision methods, initially for assessing the causality of events."; 2528 case ALGM: 2529 return "Reaching a decision through the application of an algorithm designed to weigh the different factors involved."; 2530 case BYCL: 2531 return "Reaching a decision through the use of Bayesian statistical analysis."; 2532 case GINT: 2533 return "Reaching a decision by consideration of the totality of factors involved in order to reach a judgement."; 2534 case _GENETICOBSERVATIONMETHOD: 2535 return "A code that provides additional detail about the means or technique used to ascertain the genetic analysis. Example, PCR, Micro Array"; 2536 case PCR: 2537 return "Description: Polymerase Chain Reaction"; 2538 case _OBSERVATIONMETHODAGGREGATE: 2539 return "Provides additional detail about the aggregation methods used to compute the aggregated values for an observation. This is an abstract code."; 2540 case AVERAGE: 2541 return "Average of non-null values in the referenced set of values"; 2542 case COUNT: 2543 return "Count of non-null values in the referenced set of values"; 2544 case MAX: 2545 return "Largest of all non-null values in the referenced set of values."; 2546 case MEDIAN: 2547 return "The median of all non-null values in the referenced set of values."; 2548 case MIN: 2549 return "Smallest of all non-null values in the referenced set of values."; 2550 case MODE: 2551 return "The most common value of all non-null values in the referenced set of values."; 2552 case STDEV_P: 2553 return "Standard Deviation of the values in the referenced set of values, computed over the population."; 2554 case STDEV_S: 2555 return "Standard Deviation of the values in the referenced set of values, computed over a sample of the population."; 2556 case SUM: 2557 return "Sum of non-null values in the referenced set of values"; 2558 case VARIANCE_P: 2559 return "Variance of the values in the referenced set of values, computed over the population."; 2560 case VARIANCE_S: 2561 return "Variance of the values in the referenced set of values, computed over a sample of the population."; 2562 case _VERIFICATIONMETHOD: 2563 return "VerificationMethod"; 2564 case VDOC: 2565 return "Verification by means of document.\r\n\n \n Example: Fax, letter, attachment to e-mail."; 2566 case VREG: 2567 return "verification by means of a response to an electronic query\r\n\n \n Example: query message to a Covered Party registry application or Coverage Administrator."; 2568 case VTOKEN: 2569 return "Verification by means of electronic token.\r\n\n \n Example: smartcard, magnetic swipe card, RFID device."; 2570 case VVOICE: 2571 return "Verification by means of voice.\r\n\n \n Example: By speaking with or calling the Coverage Administrator or Covered Party"; 2572 case _0001: 2573 return "Complement fixation"; 2574 case _0002: 2575 return "Computed axial tomography"; 2576 case _0003: 2577 return "Susceptibility, High Level Aminoglycoside Resistance agar test"; 2578 case _0004: 2579 return "Visual, Macroscopic observation"; 2580 case _0005: 2581 return "Computed, Magnetic resonance"; 2582 case _0006: 2583 return "Computed, Morphometry"; 2584 case _0007: 2585 return "Computed, Positron emission tomography"; 2586 case _0008: 2587 return "SAMHSA drug assay confirmation"; 2588 case _0009: 2589 return "SAMHSA drug assay screening"; 2590 case _0010: 2591 return "Serum Neutralization"; 2592 case _0011: 2593 return "Titration"; 2594 case _0012: 2595 return "Ultrasound"; 2596 case _0013: 2597 return "X-ray crystallography"; 2598 case _0014: 2599 return "Agglutination"; 2600 case _0015: 2601 return "Agglutination, Buffered acidified plate"; 2602 case _0016: 2603 return "Agglutination, Card"; 2604 case _0017: 2605 return "Agglutination, Hemagglutination"; 2606 case _0018: 2607 return "Agglutination, Hemagglutination inhibition"; 2608 case _0019: 2609 return "Agglutination, Latex"; 2610 case _0020: 2611 return "Agglutination, Plate"; 2612 case _0021: 2613 return "Agglutination, Rapid Plate"; 2614 case _0022: 2615 return "Agglutination, RBC"; 2616 case _0023: 2617 return "Agglutination, Rivanol"; 2618 case _0024: 2619 return "Agglutination, Tube"; 2620 case _0025: 2621 return "Bioassay"; 2622 case _0026: 2623 return "Bioassay, Animal Inoculation"; 2624 case _0027: 2625 return "Bioassay, Cytotoxicity"; 2626 case _0028: 2627 return "Bioassay, Embryo Infective Dose 50"; 2628 case _0029: 2629 return "Bioassay, Embryo Lethal Dose 50"; 2630 case _0030: 2631 return "Bioassay, Mouse intercerebral inoculation"; 2632 case _0031: 2633 return "Bioassay, qualitative"; 2634 case _0032: 2635 return "Bioassay, quantitative"; 2636 case _0033: 2637 return "Chemical"; 2638 case _0034: 2639 return "Chemical, Differential light absorption"; 2640 case _0035: 2641 return "Chemical, Dipstick"; 2642 case _0036: 2643 return "Chemical, Dipstick colorimetric laboratory test"; 2644 case _0037: 2645 return "Chemical, Test strip"; 2646 case _0038: 2647 return "Chromatography"; 2648 case _0039: 2649 return "Chromatography, Affinity"; 2650 case _0040: 2651 return "Chromatography, Gas liquid"; 2652 case _0041: 2653 return "Chromatography, High performance liquid"; 2654 case _0042: 2655 return "Chromatography, Liquid"; 2656 case _0043: 2657 return "Chromatography, Protein A affinity"; 2658 case _0044: 2659 return "Coagulation"; 2660 case _0045: 2661 return "Coagulation, Tilt tube"; 2662 case _0046: 2663 return "Coagulation, Tilt tube reptilase induced"; 2664 case _0047: 2665 return "Count, Automated"; 2666 case _0048: 2667 return "Count, Manual"; 2668 case _0049: 2669 return "Count, Platelet, Rees-Ecker"; 2670 case _0050: 2671 return "Culture, Aerobic"; 2672 case _0051: 2673 return "Culture, Anaerobic"; 2674 case _0052: 2675 return "Culture, Chicken Embryo"; 2676 case _0053: 2677 return "Culture, Delayed secondary enrichment"; 2678 case _0054: 2679 return "Culture, Microaerophilic"; 2680 case _0055: 2681 return "Culture, Quantitative microbial, cup"; 2682 case _0056: 2683 return "Culture, Quantitative microbial, droplet"; 2684 case _0057: 2685 return "Culture, Quantitative microbial, filter paper"; 2686 case _0058: 2687 return "Culture, Quantitative microbial, pad"; 2688 case _0059: 2689 return "Culture, Quantitative microbial, pour plate"; 2690 case _0060: 2691 return "Culture, Quantitative microbial, surface streak"; 2692 case _0061: 2693 return "Culture, Somatic Cell"; 2694 case _0062: 2695 return "Diffusion, Agar"; 2696 case _0063: 2697 return "Diffusion, Agar Gel Immunodiffusion"; 2698 case _0064: 2699 return "Electrophoresis"; 2700 case _0065: 2701 return "Electrophoresis, Agaorse gel"; 2702 case _0066: 2703 return "Electrophoresis, citrate agar"; 2704 case _0067: 2705 return "Electrophoresis, Immuno"; 2706 case _0068: 2707 return "Electrophoresis, Polyacrylamide gel"; 2708 case _0069: 2709 return "Electrophoresis, Starch gel"; 2710 case _0070: 2711 return "ELISA"; 2712 case _0071: 2713 return "ELISA, antigen capture"; 2714 case _0072: 2715 return "ELISA, avidin biotin peroxidase complex"; 2716 case _0073: 2717 return "ELISA, Kinetic"; 2718 case _0074: 2719 return "ELISA, peroxidase-antiperoxidase"; 2720 case _0075: 2721 return "Identification, API 20 Strep"; 2722 case _0076: 2723 return "Identification, API 20A"; 2724 case _0077: 2725 return "Identification, API 20C AUX"; 2726 case _0078: 2727 return "Identification, API 20E"; 2728 case _0079: 2729 return "Identification, API 20NE"; 2730 case _0080: 2731 return "Identification, API 50 CH"; 2732 case _0081: 2733 return "Identification, API An-IDENT"; 2734 case _0082: 2735 return "Identification, API Coryne"; 2736 case _0083: 2737 return "Identification, API Rapid 20E"; 2738 case _0084: 2739 return "Identification, API Staph"; 2740 case _0085: 2741 return "Identification, API ZYM"; 2742 case _0086: 2743 return "Identification, Bacterial"; 2744 case _0087: 2745 return "Identification, mini VIDAS"; 2746 case _0088: 2747 return "Identification, Phage susceptibility typing"; 2748 case _0089: 2749 return "Identification, Quad-FERM+"; 2750 case _0090: 2751 return "Identification, RAPIDEC Staph"; 2752 case _0091: 2753 return "Identification, Staphaurex"; 2754 case _0092: 2755 return "Identification, VIDAS"; 2756 case _0093: 2757 return "Identification, Vitek"; 2758 case _0094: 2759 return "Identification, VITEK 2"; 2760 case _0095: 2761 return "Immune stain"; 2762 case _0096: 2763 return "Immune stain, Immunofluorescent antibody, direct"; 2764 case _0097: 2765 return "Immune stain, Immunofluorescent antibody, indirect"; 2766 case _0098: 2767 return "Immune stain, Immunoperoxidase, Avidin-Biotin Complex"; 2768 case _0099: 2769 return "Immune stain, Immunoperoxidase, Peroxidase anti-peroxidase complex"; 2770 case _0100: 2771 return "Immune stain, Immunoperoxidase, Protein A-peroxidase complex"; 2772 case _0101: 2773 return "Immunoassay"; 2774 case _0102: 2775 return "Immunoassay, qualitative, multiple step"; 2776 case _0103: 2777 return "Immunoassay, qualitative, single step"; 2778 case _0104: 2779 return "Immunoassay, Radioimmunoassay"; 2780 case _0105: 2781 return "Immunoassay, semi-quantitative, multiple step"; 2782 case _0106: 2783 return "Immunoassay, semi-quantitative, single step"; 2784 case _0107: 2785 return "Microscopy"; 2786 case _0108: 2787 return "Microscopy, Darkfield"; 2788 case _0109: 2789 return "Microscopy, Electron"; 2790 case _0110: 2791 return "Microscopy, Electron microscopy tomography"; 2792 case _0111: 2793 return "Microscopy, Electron, negative stain"; 2794 case _0112: 2795 return "Microscopy, Electron, thick section transmission"; 2796 case _0113: 2797 return "Microscopy, Electron, thin section transmission"; 2798 case _0114: 2799 return "Microscopy, Light"; 2800 case _0115: 2801 return "Microscopy, Polarized light"; 2802 case _0116: 2803 return "Microscopy, Scanning electron"; 2804 case _0117: 2805 return "Microscopy, Transmission electron"; 2806 case _0118: 2807 return "Microscopy, Transparent tape direct examination"; 2808 case _0119: 2809 return "Molecular, 3 Self-Sustaining Sequence Replication"; 2810 case _0120: 2811 return "Molecular, Branched Chain DNA"; 2812 case _0121: 2813 return "Molecular, Hybridization Protection Assay"; 2814 case _0122: 2815 return "Molecular, Immune blot"; 2816 case _0123: 2817 return "Molecular, In-situ hybridization"; 2818 case _0124: 2819 return "Molecular, Ligase Chain Reaction"; 2820 case _0125: 2821 return "Molecular, Ligation Activated Transcription"; 2822 case _0126: 2823 return "Molecular, Nucleic Acid Probe"; 2824 case _0128: 2825 return "Molecular, Nucleic acid probe with amplification\r\n\n \r\n\n Rationale: Duplicate of code 0126. Use code 0126 instead."; 2826 case _0129: 2827 return "Molecular, Nucleic acid probe with target amplification"; 2828 case _0130: 2829 return "Molecular, Nucleic acid reverse transcription"; 2830 case _0131: 2831 return "Molecular, Nucleic Acid Sequence Based Analysis"; 2832 case _0132: 2833 return "Molecular, Polymerase chain reaction"; 2834 case _0133: 2835 return "Molecular, Q-Beta Replicase or probe amplification category method"; 2836 case _0134: 2837 return "Molecular, Restriction Fragment Length Polymorphism"; 2838 case _0135: 2839 return "Molecular, Southern Blot"; 2840 case _0136: 2841 return "Molecular, Strand Displacement Amplification"; 2842 case _0137: 2843 return "Molecular, Transcription Mediated Amplification"; 2844 case _0138: 2845 return "Molecular, Western Blot"; 2846 case _0139: 2847 return "Precipitation, Flocculation"; 2848 case _0140: 2849 return "Precipitation, Immune precipitation"; 2850 case _0141: 2851 return "Precipitation, Milk ring test"; 2852 case _0142: 2853 return "Precipitation, Precipitin"; 2854 case _0143: 2855 return "Stain, Acid fast"; 2856 case _0144: 2857 return "Stain, Acid fast, fluorochrome"; 2858 case _0145: 2859 return "Stain, Acid fast, Kinyoun's cold carbolfuchsin"; 2860 case _0146: 2861 return "Stain, Acid fast, Ziehl-Neelsen"; 2862 case _0147: 2863 return "Stain, Acid phosphatase"; 2864 case _0148: 2865 return "Stain, Acridine orange"; 2866 case _0149: 2867 return "Stain, Active brilliant orange KH"; 2868 case _0150: 2869 return "Stain, Alazarin red S"; 2870 case _0151: 2871 return "Stain, Alcian blue"; 2872 case _0152: 2873 return "Stain, Alcian blue with Periodic acid Schiff"; 2874 case _0153: 2875 return "Stain, Argentaffin"; 2876 case _0154: 2877 return "Stain, Argentaffin silver"; 2878 case _0155: 2879 return "Stain, Azure-eosin"; 2880 case _0156: 2881 return "Stain, Basic Fuschin"; 2882 case _0157: 2883 return "Stain, Bennhold"; 2884 case _0158: 2885 return "Stain, Bennhold's Congo red"; 2886 case _0159: 2887 return "Stain, Bielschowsky"; 2888 case _0160: 2889 return "Stain, Bielschowsky's silver"; 2890 case _0161: 2891 return "Stain, Bleach"; 2892 case _0162: 2893 return "Stain, Bodian"; 2894 case _0163: 2895 return "Stain, Brown-Brenn"; 2896 case _0164: 2897 return "Stain, Butyrate-esterase"; 2898 case _0165: 2899 return "Stain, Calcofluor white fluorescent"; 2900 case _0166: 2901 return "Stain, Carbol-fuchsin"; 2902 case _0167: 2903 return "Stain, Carmine"; 2904 case _0168: 2905 return "Stain, Churukian-Schenk"; 2906 case _0169: 2907 return "Stain, Congo red"; 2908 case _0170: 2909 return "Stain, Cresyl echt violet"; 2910 case _0171: 2911 return "Stain, Crystal violet"; 2912 case _0172: 2913 return "Stain, De Galantha"; 2914 case _0173: 2915 return "Stain, Dieterle silver impregnation"; 2916 case _0174: 2917 return "Stain, Fite-Farco"; 2918 case _0175: 2919 return "Stain, Fontana-Masson silver"; 2920 case _0176: 2921 return "Stain, Fouchet"; 2922 case _0177: 2923 return "Stain, Gomori"; 2924 case _0178: 2925 return "Stain, Gomori methenamine silver"; 2926 case _0179: 2927 return "Stain, Gomori-Wheatly trichrome"; 2928 case _0180: 2929 return "Stain, Gridley"; 2930 case _0181: 2931 return "Stain, Grimelius silver"; 2932 case _0182: 2933 return "Stain, Grocott"; 2934 case _0183: 2935 return "Stain, Grocott methenamine silver"; 2936 case _0184: 2937 return "Stain, Hale's colloidal ferric oxide"; 2938 case _0185: 2939 return "Stain, Hale's colloidal iron"; 2940 case _0186: 2941 return "Stain, Hansel"; 2942 case _0187: 2943 return "Stain, Harris regressive hematoxylin and eosin"; 2944 case _0188: 2945 return "Stain, Hematoxylin and eosin"; 2946 case _0189: 2947 return "Stain, Highman"; 2948 case _0190: 2949 return "Stain, Holzer"; 2950 case _0191: 2951 return "Stain, Iron hematoxylin"; 2952 case _0192: 2953 return "Stain, Jones"; 2954 case _0193: 2955 return "Stain, Jones methenamine silver"; 2956 case _0194: 2957 return "Stain, Kossa"; 2958 case _0195: 2959 return "Stain, Lawson-Van Gieson"; 2960 case _0196: 2961 return "Stain, Loeffler methylene blue"; 2962 case _0197: 2963 return "Stain, Luxol fast blue with cresyl violet"; 2964 case _0198: 2965 return "Stain, Luxol fast blue with Periodic acid-Schiff"; 2966 case _0199: 2967 return "Stain, MacNeal's tetrachrome blood"; 2968 case _0200: 2969 return "Stain, Mallory-Heidenhain"; 2970 case _0201: 2971 return "Stain, Masson trichrome"; 2972 case _0202: 2973 return "Stain, Mayer mucicarmine"; 2974 case _0203: 2975 return "Stain, Mayers progressive hematoxylin and eosin"; 2976 case _0204: 2977 return "Stain, May-Grunwald Giemsa"; 2978 case _0205: 2979 return "Stain, Methyl green"; 2980 case _0206: 2981 return "Stain, Methyl green pyronin"; 2982 case _0207: 2983 return "Stain, Modified Gomori-Wheatly trichrome"; 2984 case _0208: 2985 return "Stain, Modified Masson trichrome"; 2986 case _0209: 2987 return "Stain, Modified trichrome"; 2988 case _0210: 2989 return "Stain, Movat pentachrome"; 2990 case _0211: 2991 return "Stain, Mucicarmine"; 2992 case _0212: 2993 return "Stain, Neutral red"; 2994 case _0213: 2995 return "Stain, Night blue"; 2996 case _0214: 2997 return "Stain, Non-specific esterase"; 2998 case _0215: 2999 return "Stain, Oil red-O"; 3000 case _0216: 3001 return "Stain, Orcein"; 3002 case _0217: 3003 return "Stain, Perls'"; 3004 case _0218: 3005 return "Stain, Phosphotungstic acid-hematoxylin"; 3006 case _0219: 3007 return "Stain, Potassium ferrocyanide"; 3008 case _0220: 3009 return "Stain, Prussian blue"; 3010 case _0221: 3011 return "Stain, Putchler modified Bennhold"; 3012 case _0222: 3013 return "Stain, Quinacrine fluorescent"; 3014 case _0223: 3015 return "Stain, Reticulin"; 3016 case _0224: 3017 return "Stain, Rhodamine"; 3018 case _0225: 3019 return "Stain, Safranin"; 3020 case _0226: 3021 return "Stain, Schmorl"; 3022 case _0227: 3023 return "Stain, Seiver-Munger"; 3024 case _0228: 3025 return "Stain, Silver"; 3026 case _0229: 3027 return "Stain, Specific esterase"; 3028 case _0230: 3029 return "Stain, Steiner silver"; 3030 case _0231: 3031 return "Stain, Sudan III"; 3032 case _0232: 3033 return "Stain, Sudan IVI"; 3034 case _0233: 3035 return "Stain, Sulfated alcian blue"; 3036 case _0234: 3037 return "Stain, Supravital"; 3038 case _0235: 3039 return "Stain, Thioflavine-S"; 3040 case _0236: 3041 return "Stain, Three micron Giemsa"; 3042 case _0237: 3043 return "Stain, Vassar-Culling"; 3044 case _0238: 3045 return "Stain, Vital"; 3046 case _0239: 3047 return "Stain, von Kossa"; 3048 case _0243: 3049 return "Susceptibility, Minimum bactericidal concentration, macrodilution"; 3050 case _0244: 3051 return "Susceptibility, Minimum bactericidal concentration, microdilution"; 3052 case _0247: 3053 return "Turbidometric"; 3054 case _0248: 3055 return "Turbidometric, Refractometric"; 3056 case _0249: 3057 return "Chromatography, Thin Layer"; 3058 case _0250: 3059 return "Immunoassay, enzyme-multiplied technique (EMIT)"; 3060 case _0251: 3061 return "Flow Cytometry"; 3062 case _0252: 3063 return "Radial Immunodiffusion"; 3064 case _0253: 3065 return "Immunoassay, Fluorescence Polarization"; 3066 case _0254: 3067 return "Electrophoresis, Immunofixation"; 3068 case _0255: 3069 return "Dialysis, Direct Equilibrium"; 3070 case _0256: 3071 return "Acid Elution, Kleihauer-Betke Method"; 3072 case _0257: 3073 return "Immunofluorescence, Anti-Complement"; 3074 case _0258: 3075 return "Gas Chromatography/Mass Spectroscopy"; 3076 case _0259: 3077 return "Light Scatter, Nephelometry"; 3078 case _0260: 3079 return "Immunoassay, IgE Antibody Test"; 3080 case _0261: 3081 return "Lymphocyte Microcytotoxicity Assay"; 3082 case _0262: 3083 return "Spectrophotometry"; 3084 case _0263: 3085 return "Spectrophotometry, Atomic Absorption"; 3086 case _0264: 3087 return "Electrochemical, Ion Selective Electrode"; 3088 case _0265: 3089 return "Chromatography, Gas"; 3090 case _0266: 3091 return "Isoelectric Focusing"; 3092 case _0267: 3093 return "Immunoassay, Chemiluminescent"; 3094 case _0268: 3095 return "Immunoassay, Microparticle Enzyme"; 3096 case _0269: 3097 return "Inductively-Coupled Plasma/Mass Spectrometry"; 3098 case _0270: 3099 return "Immunoassay, Immunoradiometric Assay"; 3100 case _0271: 3101 return "Coagulation, Photo Optical Clot Detection"; 3102 case _0280: 3103 return "Test methods designed to determine a microorganismaTMs susceptibility to being killed by an antibiotic."; 3104 case _0240: 3105 return "Susceptibility, Antibiotic sensitivity, disk"; 3106 case _0241: 3107 return "Susceptibility, BACTEC susceptibility test"; 3108 case _0242: 3109 return "Susceptibility, Disk dilution"; 3110 case _0272: 3111 return "Testing to measure the minimum concentration of the antibacterial agent in a given culture medium below which bacterial growth is not inhibited."; 3112 case _0245: 3113 return "Susceptibility, Minimum Inhibitory concentration, macrodilution"; 3114 case _0246: 3115 return "Susceptibility, Minimum Inhibitory concentration, microdilution"; 3116 case _0273: 3117 return "Viral Genotype Susceptibility"; 3118 case _0274: 3119 return "Viral Phenotype Susceptibility"; 3120 case _0275: 3121 return "Gradient Strip"; 3122 case _0275A: 3123 return "Minimum Lethal Concentration (MLC)"; 3124 case _0276: 3125 return "Testing to measure the minimum concentration of the antibacterial agent in a given culture medium below which bacterial growth is not inhibited."; 3126 case _0277: 3127 return "Serum bactericidal titer"; 3128 case _0278: 3129 return "Agar screen"; 3130 case _0279: 3131 return "Disk induction"; 3132 case _0127: 3133 return "Molecular, Nucleic acid probe"; 3134 case NULL: 3135 return null; 3136 default: 3137 return "?"; 3138 } 3139 } 3140 3141 public String getDisplay() { 3142 switch (this) { 3143 case _DECISIONOBSERVATIONMETHOD: 3144 return "DecisionObservationMethod"; 3145 case ALGM: 3146 return "algorithm"; 3147 case BYCL: 3148 return "bayesian calculation"; 3149 case GINT: 3150 return "global introspection"; 3151 case _GENETICOBSERVATIONMETHOD: 3152 return "GeneticObservationMethod"; 3153 case PCR: 3154 return "PCR"; 3155 case _OBSERVATIONMETHODAGGREGATE: 3156 return "observation method aggregate"; 3157 case AVERAGE: 3158 return "average"; 3159 case COUNT: 3160 return "count"; 3161 case MAX: 3162 return "maxima"; 3163 case MEDIAN: 3164 return "median"; 3165 case MIN: 3166 return "minima"; 3167 case MODE: 3168 return "mode"; 3169 case STDEV_P: 3170 return "population standard deviation"; 3171 case STDEV_S: 3172 return "sample standard deviation"; 3173 case SUM: 3174 return "sum"; 3175 case VARIANCE_P: 3176 return "population variance"; 3177 case VARIANCE_S: 3178 return "sample variance"; 3179 case _VERIFICATIONMETHOD: 3180 return "VerificationMethod"; 3181 case VDOC: 3182 return "document verification"; 3183 case VREG: 3184 return "registry verification"; 3185 case VTOKEN: 3186 return "electronic token verification"; 3187 case VVOICE: 3188 return "voice-based verification"; 3189 case _0001: 3190 return "Complement fixation"; 3191 case _0002: 3192 return "Computed axial tomography"; 3193 case _0003: 3194 return "HLAR agar test"; 3195 case _0004: 3196 return "Macroscopic observation"; 3197 case _0005: 3198 return "Magnetic resonance"; 3199 case _0006: 3200 return "Morphometry"; 3201 case _0007: 3202 return "Positron emission tomography"; 3203 case _0008: 3204 return "SAMHSA confirmation"; 3205 case _0009: 3206 return "SAMHSA screening"; 3207 case _0010: 3208 return "Serum Neutralization"; 3209 case _0011: 3210 return "Titration"; 3211 case _0012: 3212 return "Ultrasound"; 3213 case _0013: 3214 return "X-ray crystallography"; 3215 case _0014: 3216 return "Agglutination"; 3217 case _0015: 3218 return "Buffered acidified plate agglutination"; 3219 case _0016: 3220 return "Card agglutination"; 3221 case _0017: 3222 return "Hemagglutination"; 3223 case _0018: 3224 return "Hemagglutination inhibition"; 3225 case _0019: 3226 return "Latex agglutination"; 3227 case _0020: 3228 return "Plate agglutination"; 3229 case _0021: 3230 return "Rapid agglutination"; 3231 case _0022: 3232 return "RBC agglutination"; 3233 case _0023: 3234 return "Rivanol agglutination"; 3235 case _0024: 3236 return "Tube agglutination"; 3237 case _0025: 3238 return "Bioassay"; 3239 case _0026: 3240 return "Animal Inoculation"; 3241 case _0027: 3242 return "Cytotoxicity"; 3243 case _0028: 3244 return "Embryo infective dose 50"; 3245 case _0029: 3246 return "Embryo lethal dose 50"; 3247 case _0030: 3248 return "Mouse intercerebral inoculation"; 3249 case _0031: 3250 return "Bioassay, qualitative"; 3251 case _0032: 3252 return "Bioassay, quantitative"; 3253 case _0033: 3254 return "Chemical method"; 3255 case _0034: 3256 return "Differential light absorption chemical test"; 3257 case _0035: 3258 return "Dipstick"; 3259 case _0036: 3260 return "Dipstick colorimetric laboratory test"; 3261 case _0037: 3262 return "Test strip"; 3263 case _0038: 3264 return "Chromatography"; 3265 case _0039: 3266 return "Affinity chromatography"; 3267 case _0040: 3268 return "Gas liquid chromatography"; 3269 case _0041: 3270 return "High performance liquid chromatography"; 3271 case _0042: 3272 return "Liquid Chromatography"; 3273 case _0043: 3274 return "Protein A affinity chromatography"; 3275 case _0044: 3276 return "Coagulation"; 3277 case _0045: 3278 return "Tilt tube coagulation time"; 3279 case _0046: 3280 return "Tilt tube reptilase induced coagulation"; 3281 case _0047: 3282 return "Automated count"; 3283 case _0048: 3284 return "Manual cell count"; 3285 case _0049: 3286 return "Platelet count, Rees-Ecker"; 3287 case _0050: 3288 return "Aerobic Culture"; 3289 case _0051: 3290 return "Anaerobic Culture"; 3291 case _0052: 3292 return "Chicken embryo culture"; 3293 case _0053: 3294 return "Delayed secondary enrichment"; 3295 case _0054: 3296 return "Microaerophilic Culture"; 3297 case _0055: 3298 return "Quantitative microbial culture, cup"; 3299 case _0056: 3300 return "Quantitative microbial culture, droplet"; 3301 case _0057: 3302 return "Quantitative microbial culture, filter paper"; 3303 case _0058: 3304 return "Quantitative microbial culture, pad culture"; 3305 case _0059: 3306 return "Quantitative microbial culture, pour plate"; 3307 case _0060: 3308 return "Quantitative microbial culture, surface streak"; 3309 case _0061: 3310 return "Somatic Cell culture"; 3311 case _0062: 3312 return "Agar diffusion"; 3313 case _0063: 3314 return "Agar Gel Immunodiffusion"; 3315 case _0064: 3316 return "Electrophoresis"; 3317 case _0065: 3318 return "Agaorse gel electrophoresis"; 3319 case _0066: 3320 return "Electrophoresis, citrate agar"; 3321 case _0067: 3322 return "Immunoelectrophoresis"; 3323 case _0068: 3324 return "Polyacrylamide gel electrophoresis"; 3325 case _0069: 3326 return "Starch gel electrophoresis"; 3327 case _0070: 3328 return "ELISA"; 3329 case _0071: 3330 return "ELISA, antigen capture"; 3331 case _0072: 3332 return "ELISA, avidin biotin peroxidase complex"; 3333 case _0073: 3334 return "Kinetic ELISA"; 3335 case _0074: 3336 return "ELISA, peroxidase-antiperoxidase"; 3337 case _0075: 3338 return "API 20 Strep"; 3339 case _0076: 3340 return "API 20A"; 3341 case _0077: 3342 return "API 20C AUX"; 3343 case _0078: 3344 return "API 20E"; 3345 case _0079: 3346 return "API 20NE"; 3347 case _0080: 3348 return "API 50 CH"; 3349 case _0081: 3350 return "API An-IDENT"; 3351 case _0082: 3352 return "API Coryne"; 3353 case _0083: 3354 return "API Rapid 20E"; 3355 case _0084: 3356 return "API Staph"; 3357 case _0085: 3358 return "API ZYM"; 3359 case _0086: 3360 return "Bacterial identification"; 3361 case _0087: 3362 return "mini VIDAS"; 3363 case _0088: 3364 return "Phage susceptibility typing"; 3365 case _0089: 3366 return "Quad-FERM+"; 3367 case _0090: 3368 return "RAPIDEC Staph"; 3369 case _0091: 3370 return "Staphaurex"; 3371 case _0092: 3372 return "VIDAS"; 3373 case _0093: 3374 return "Vitek"; 3375 case _0094: 3376 return "VITEK 2"; 3377 case _0095: 3378 return "Immune stain"; 3379 case _0096: 3380 return "Immunofluorescent antibody, direct"; 3381 case _0097: 3382 return "Immunofluorescent antibody, indirect"; 3383 case _0098: 3384 return "Immunoperoxidase, Avidin-Biotin Complex"; 3385 case _0099: 3386 return "Immunoperoxidase, Peroxidase anti-peroxidase complex"; 3387 case _0100: 3388 return "Immunoperoxidase, Protein A-peroxidase complex"; 3389 case _0101: 3390 return "Immunoassay"; 3391 case _0102: 3392 return "Immunoassay, qualitative, multiple step"; 3393 case _0103: 3394 return "Immunoassay, qualitative, single step"; 3395 case _0104: 3396 return "Radioimmunoassay"; 3397 case _0105: 3398 return "Immunoassay, semi-quantitative, multiple step"; 3399 case _0106: 3400 return "Immunoassay, semi-quantitative, single step"; 3401 case _0107: 3402 return "Microscopy"; 3403 case _0108: 3404 return "Darkfield microscopy"; 3405 case _0109: 3406 return "Electron microscopy"; 3407 case _0110: 3408 return "Electron microscopy tomography"; 3409 case _0111: 3410 return "Electron microscopy, negative stain"; 3411 case _0112: 3412 return "Electron microscopy, thick section"; 3413 case _0113: 3414 return "Electron microscopy, thin section"; 3415 case _0114: 3416 return "Microscopy, Light"; 3417 case _0115: 3418 return "Polarizing light microscopy"; 3419 case _0116: 3420 return "Scanning electron microscopy"; 3421 case _0117: 3422 return "Transmission electron microscopy"; 3423 case _0118: 3424 return "Transparent tape direct examination"; 3425 case _0119: 3426 return "3 Self-Sustaining Sequence Replication"; 3427 case _0120: 3428 return "Branched Chain DNA"; 3429 case _0121: 3430 return "Hybridization Protection Assay"; 3431 case _0122: 3432 return "Immune blot"; 3433 case _0123: 3434 return "In-situ hybridization"; 3435 case _0124: 3436 return "Ligase Chain Reaction"; 3437 case _0125: 3438 return "Ligation Activated Transcription"; 3439 case _0126: 3440 return "Nucleic Acid Probe"; 3441 case _0128: 3442 return "Nucleic acid probe with amplification"; 3443 case _0129: 3444 return "Nucleic acid probe with target amplification"; 3445 case _0130: 3446 return "Nucleic acid reverse transcription"; 3447 case _0131: 3448 return "Nucleic Acid Sequence Based Analysis"; 3449 case _0132: 3450 return "Polymerase chain reaction"; 3451 case _0133: 3452 return "Q-Beta Replicase or probe amplification category method"; 3453 case _0134: 3454 return "Restriction Fragment Length Polymorphism"; 3455 case _0135: 3456 return "Southern Blot"; 3457 case _0136: 3458 return "Strand Displacement Amplification"; 3459 case _0137: 3460 return "Transcription Mediated Amplification"; 3461 case _0138: 3462 return "Western Blot"; 3463 case _0139: 3464 return "Flocculation"; 3465 case _0140: 3466 return "Immune precipitation"; 3467 case _0141: 3468 return "Milk ring test"; 3469 case _0142: 3470 return "Precipitin"; 3471 case _0143: 3472 return "Acid fast stain"; 3473 case _0144: 3474 return "Acid fast stain, fluorochrome"; 3475 case _0145: 3476 return "Acid fast stain, Kinyoun's cold carbolfuchsin"; 3477 case _0146: 3478 return "Acid fast stain, Ziehl-Neelsen"; 3479 case _0147: 3480 return "Acid phosphatase stain"; 3481 case _0148: 3482 return "Acridine orange stain"; 3483 case _0149: 3484 return "Active brilliant orange KH stain"; 3485 case _0150: 3486 return "Alazarin red S stain"; 3487 case _0151: 3488 return "Alcian blue stain"; 3489 case _0152: 3490 return "Alcian blue with Periodic acid Schiff stain"; 3491 case _0153: 3492 return "Argentaffin stain"; 3493 case _0154: 3494 return "Argentaffin silver stain"; 3495 case _0155: 3496 return "Azure-eosin stain"; 3497 case _0156: 3498 return "Basic Fuschin stain"; 3499 case _0157: 3500 return "Bennhold stain"; 3501 case _0158: 3502 return "Bennhold's Congo red stain"; 3503 case _0159: 3504 return "Bielschowsky stain"; 3505 case _0160: 3506 return "Bielschowsky's silver stain"; 3507 case _0161: 3508 return "Bleach stain"; 3509 case _0162: 3510 return "Bodian stain"; 3511 case _0163: 3512 return "Brown-Brenn stain"; 3513 case _0164: 3514 return "Butyrate-esterase stain"; 3515 case _0165: 3516 return "Calcofluor white fluorescent stain"; 3517 case _0166: 3518 return "Carbol-fuchsin stain"; 3519 case _0167: 3520 return "Carmine stain"; 3521 case _0168: 3522 return "Churukian-Schenk stain"; 3523 case _0169: 3524 return "Congo red stain"; 3525 case _0170: 3526 return "Cresyl echt violet stain"; 3527 case _0171: 3528 return "Crystal violet stain"; 3529 case _0172: 3530 return "De Galantha stain"; 3531 case _0173: 3532 return "Dieterle silver impregnation stain"; 3533 case _0174: 3534 return "Fite-Farco stain"; 3535 case _0175: 3536 return "Fontana-Masson silver stain"; 3537 case _0176: 3538 return "Fouchet stain"; 3539 case _0177: 3540 return "Gomori stain"; 3541 case _0178: 3542 return "Gomori methenamine silver stain"; 3543 case _0179: 3544 return "Gomori-Wheatly trichrome stain"; 3545 case _0180: 3546 return "Gridley stain"; 3547 case _0181: 3548 return "Grimelius silver stain"; 3549 case _0182: 3550 return "Grocott stain"; 3551 case _0183: 3552 return "Grocott methenamine silver stain"; 3553 case _0184: 3554 return "Hale's colloidal ferric oxide stain"; 3555 case _0185: 3556 return "Hale's colloidal iron stain"; 3557 case _0186: 3558 return "Hansel stain"; 3559 case _0187: 3560 return "Harris regressive hematoxylin and eosin stain"; 3561 case _0188: 3562 return "Hematoxylin and eosin stain"; 3563 case _0189: 3564 return "Highman stain"; 3565 case _0190: 3566 return "Holzer stain"; 3567 case _0191: 3568 return "Iron hematoxylin stain"; 3569 case _0192: 3570 return "Jones stain"; 3571 case _0193: 3572 return "Jones methenamine silver stain"; 3573 case _0194: 3574 return "Kossa stain"; 3575 case _0195: 3576 return "Lawson-Van Gieson stain"; 3577 case _0196: 3578 return "Loeffler methylene blue stain"; 3579 case _0197: 3580 return "Luxol fast blue with cresyl violet stain"; 3581 case _0198: 3582 return "Luxol fast blue with Periodic acid-Schiff stain"; 3583 case _0199: 3584 return "MacNeal's tetrachrome blood stain"; 3585 case _0200: 3586 return "Mallory-Heidenhain stain"; 3587 case _0201: 3588 return "Masson trichrome stain"; 3589 case _0202: 3590 return "Mayer mucicarmine stain"; 3591 case _0203: 3592 return "Mayers progressive hematoxylin and eosin stain"; 3593 case _0204: 3594 return "May-Grunwald Giemsa stain"; 3595 case _0205: 3596 return "Methyl green stain"; 3597 case _0206: 3598 return "Methyl green pyronin stain"; 3599 case _0207: 3600 return "Modified Gomori-Wheatly trichrome stain"; 3601 case _0208: 3602 return "Modified Masson trichrome stain"; 3603 case _0209: 3604 return "Modified trichrome stain"; 3605 case _0210: 3606 return "Movat pentachrome stain"; 3607 case _0211: 3608 return "Mucicarmine stain"; 3609 case _0212: 3610 return "Neutral red stain"; 3611 case _0213: 3612 return "Night blue stain"; 3613 case _0214: 3614 return "Non-specific esterase stain"; 3615 case _0215: 3616 return "Oil red-O stain"; 3617 case _0216: 3618 return "Orcein stain"; 3619 case _0217: 3620 return "Perls' stain"; 3621 case _0218: 3622 return "Phosphotungstic acid-hematoxylin stain"; 3623 case _0219: 3624 return "Potassium ferrocyanide stain"; 3625 case _0220: 3626 return "Prussian blue stain"; 3627 case _0221: 3628 return "Putchler modified Bennhold stain"; 3629 case _0222: 3630 return "Quinacrine fluorescent stain"; 3631 case _0223: 3632 return "Reticulin stain"; 3633 case _0224: 3634 return "Rhodamine stain"; 3635 case _0225: 3636 return "Safranin stain"; 3637 case _0226: 3638 return "Schmorl stain"; 3639 case _0227: 3640 return "Seiver-Munger stain"; 3641 case _0228: 3642 return "Silver stain"; 3643 case _0229: 3644 return "Specific esterase stain"; 3645 case _0230: 3646 return "Steiner silver stain"; 3647 case _0231: 3648 return "Sudan III stain"; 3649 case _0232: 3650 return "Sudan IVI stain"; 3651 case _0233: 3652 return "Sulfated alcian blue stain"; 3653 case _0234: 3654 return "Supravital stain"; 3655 case _0235: 3656 return "Thioflavine-S stain"; 3657 case _0236: 3658 return "Three micron Giemsa stain"; 3659 case _0237: 3660 return "Vassar-Culling stain"; 3661 case _0238: 3662 return "Vital Stain"; 3663 case _0239: 3664 return "von Kossa stain"; 3665 case _0243: 3666 return "Minimum bactericidal concentration test, macrodilution"; 3667 case _0244: 3668 return "Minimum bactericidal concentration test, microdilution"; 3669 case _0247: 3670 return "Turbidometric"; 3671 case _0248: 3672 return "Refractometric"; 3673 case _0249: 3674 return "Thin layer chromatography (TLC)"; 3675 case _0250: 3676 return "EMIT"; 3677 case _0251: 3678 return "Flow cytometry (FC)"; 3679 case _0252: 3680 return "Radial immunodiffusion (RID)"; 3681 case _0253: 3682 return "Fluorescence polarization immunoassay (FPIA)"; 3683 case _0254: 3684 return "Immunofixation electrophoresis (IFE)"; 3685 case _0255: 3686 return "Equilibrium dialysis"; 3687 case _0256: 3688 return "Kleihauer-Betke acid elution"; 3689 case _0257: 3690 return "Anti-complement immunofluorescence (ACIF)"; 3691 case _0258: 3692 return "GC/MS"; 3693 case _0259: 3694 return "Nephelometry"; 3695 case _0260: 3696 return "IgE immunoassay antibody"; 3697 case _0261: 3698 return "Lymphocyte Microcytotoxicity Assay"; 3699 case _0262: 3700 return "Spectrophotometry"; 3701 case _0263: 3702 return "Atomic absorption spectrophotometry (AAS)"; 3703 case _0264: 3704 return "Ion selective electrode (ISE)"; 3705 case _0265: 3706 return "Gas chromatography (GC)"; 3707 case _0266: 3708 return "Isoelectric focusing (IEF)"; 3709 case _0267: 3710 return "Immunochemiluminescence"; 3711 case _0268: 3712 return "Microparticle enzyme immunoassay (MEIA)"; 3713 case _0269: 3714 return "ICP/MS"; 3715 case _0270: 3716 return "Immunoradiometric assay (IRMA)"; 3717 case _0271: 3718 return "Photo optical clot detection"; 3719 case _0280: 3720 return "Susceptibility Testing"; 3721 case _0240: 3722 return "Antibiotic sensitivity, disk"; 3723 case _0241: 3724 return "BACTEC susceptibility test"; 3725 case _0242: 3726 return "Disk dilution"; 3727 case _0272: 3728 return "Minimum Inhibitory Concentration"; 3729 case _0245: 3730 return "Minimum Inhibitory Concentration, macrodilution"; 3731 case _0246: 3732 return "Minimum Inhibitory Concentration, microdilution"; 3733 case _0273: 3734 return "Viral Genotype Susceptibility"; 3735 case _0274: 3736 return "Viral Phenotype Susceptibility"; 3737 case _0275: 3738 return "Gradient Strip"; 3739 case _0275A: 3740 return "Minimum Lethal Concentration (MLC)"; 3741 case _0276: 3742 return "Slow Mycobacteria Susceptibility"; 3743 case _0277: 3744 return "Serum bactericidal titer"; 3745 case _0278: 3746 return "Agar screen"; 3747 case _0279: 3748 return "Disk induction"; 3749 case _0127: 3750 return "Nucleic acid probe"; 3751 case NULL: 3752 return null; 3753 default: 3754 return "?"; 3755 } 3756 } 3757 3758}