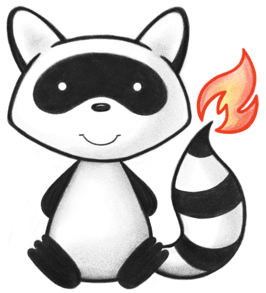
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum V3ParticipationMode { 037 038 /** 039 * Participation by non-human-languaged based electronic signal 040 */ 041 ELECTRONIC, 042 /** 043 * Participation by direct action where subject and actor are in the same 044 * location. (The participation involves more than communication.) 045 */ 046 PHYSICAL, 047 /** 048 * Participation by direct action where subject and actor are in separate 049 * locations, and the actions of the actor are transmitted by electronic or 050 * mechanical means. (The participation involves more than communication.) 051 */ 052 REMOTE, 053 /** 054 * Participation by voice communication 055 */ 056 VERBAL, 057 /** 058 * Participation by pre-recorded voice. Communication is limited to one 059 * direction (from the recorder to recipient). 060 */ 061 DICTATE, 062 /** 063 * Participation by voice communication where parties speak to each other 064 * directly. 065 */ 066 FACE, 067 /** 068 * Participation by voice communication where the voices of the communicating 069 * parties are transported over an electronic medium 070 */ 071 PHONE, 072 /** 073 * Participation by voice and visual communication where the voices and images 074 * of the communicating parties are transported over an electronic medium 075 */ 076 VIDEOCONF, 077 /** 078 * Participation by human language recorded on a physical material 079 */ 080 WRITTEN, 081 /** 082 * Participation by text or diagrams printed on paper that have been transmitted 083 * over a fax device 084 */ 085 FAXWRIT, 086 /** 087 * Participation by text or diagrams printed on paper or other recording medium 088 */ 089 HANDWRIT, 090 /** 091 * Participation by text or diagrams printed on paper transmitted physically 092 * (e.g. by courier service, postal service). 093 */ 094 MAILWRIT, 095 /** 096 * Participation by text or diagrams submitted by computer network, e.g. online 097 * survey. 098 */ 099 ONLINEWRIT, 100 /** 101 * Participation by text or diagrams transmitted over an electronic mail system. 102 */ 103 EMAILWRIT, 104 /** 105 * Participation by text or diagrams printed on paper or other recording medium 106 * where the recording was performed using a typewriter, typesetter, computer or 107 * similar mechanism. 108 */ 109 TYPEWRIT, 110 /** 111 * added to help the parsers 112 */ 113 NULL; 114 115 public static V3ParticipationMode fromCode(String codeString) throws FHIRException { 116 if (codeString == null || "".equals(codeString)) 117 return null; 118 if ("ELECTRONIC".equals(codeString)) 119 return ELECTRONIC; 120 if ("PHYSICAL".equals(codeString)) 121 return PHYSICAL; 122 if ("REMOTE".equals(codeString)) 123 return REMOTE; 124 if ("VERBAL".equals(codeString)) 125 return VERBAL; 126 if ("DICTATE".equals(codeString)) 127 return DICTATE; 128 if ("FACE".equals(codeString)) 129 return FACE; 130 if ("PHONE".equals(codeString)) 131 return PHONE; 132 if ("VIDEOCONF".equals(codeString)) 133 return VIDEOCONF; 134 if ("WRITTEN".equals(codeString)) 135 return WRITTEN; 136 if ("FAXWRIT".equals(codeString)) 137 return FAXWRIT; 138 if ("HANDWRIT".equals(codeString)) 139 return HANDWRIT; 140 if ("MAILWRIT".equals(codeString)) 141 return MAILWRIT; 142 if ("ONLINEWRIT".equals(codeString)) 143 return ONLINEWRIT; 144 if ("EMAILWRIT".equals(codeString)) 145 return EMAILWRIT; 146 if ("TYPEWRIT".equals(codeString)) 147 return TYPEWRIT; 148 throw new FHIRException("Unknown V3ParticipationMode code '" + codeString + "'"); 149 } 150 151 public String toCode() { 152 switch (this) { 153 case ELECTRONIC: 154 return "ELECTRONIC"; 155 case PHYSICAL: 156 return "PHYSICAL"; 157 case REMOTE: 158 return "REMOTE"; 159 case VERBAL: 160 return "VERBAL"; 161 case DICTATE: 162 return "DICTATE"; 163 case FACE: 164 return "FACE"; 165 case PHONE: 166 return "PHONE"; 167 case VIDEOCONF: 168 return "VIDEOCONF"; 169 case WRITTEN: 170 return "WRITTEN"; 171 case FAXWRIT: 172 return "FAXWRIT"; 173 case HANDWRIT: 174 return "HANDWRIT"; 175 case MAILWRIT: 176 return "MAILWRIT"; 177 case ONLINEWRIT: 178 return "ONLINEWRIT"; 179 case EMAILWRIT: 180 return "EMAILWRIT"; 181 case TYPEWRIT: 182 return "TYPEWRIT"; 183 case NULL: 184 return null; 185 default: 186 return "?"; 187 } 188 } 189 190 public String getSystem() { 191 return "http://terminology.hl7.org/CodeSystem/v3-ParticipationMode"; 192 } 193 194 public String getDefinition() { 195 switch (this) { 196 case ELECTRONIC: 197 return "Participation by non-human-languaged based electronic signal"; 198 case PHYSICAL: 199 return "Participation by direct action where subject and actor are in the same location. (The participation involves more than communication.)"; 200 case REMOTE: 201 return "Participation by direct action where subject and actor are in separate locations, and the actions of the actor are transmitted by electronic or mechanical means. (The participation involves more than communication.)"; 202 case VERBAL: 203 return "Participation by voice communication"; 204 case DICTATE: 205 return "Participation by pre-recorded voice. Communication is limited to one direction (from the recorder to recipient)."; 206 case FACE: 207 return "Participation by voice communication where parties speak to each other directly."; 208 case PHONE: 209 return "Participation by voice communication where the voices of the communicating parties are transported over an electronic medium"; 210 case VIDEOCONF: 211 return "Participation by voice and visual communication where the voices and images of the communicating parties are transported over an electronic medium"; 212 case WRITTEN: 213 return "Participation by human language recorded on a physical material"; 214 case FAXWRIT: 215 return "Participation by text or diagrams printed on paper that have been transmitted over a fax device"; 216 case HANDWRIT: 217 return "Participation by text or diagrams printed on paper or other recording medium"; 218 case MAILWRIT: 219 return "Participation by text or diagrams printed on paper transmitted physically (e.g. by courier service, postal service)."; 220 case ONLINEWRIT: 221 return "Participation by text or diagrams submitted by computer network, e.g. online survey."; 222 case EMAILWRIT: 223 return "Participation by text or diagrams transmitted over an electronic mail system."; 224 case TYPEWRIT: 225 return "Participation by text or diagrams printed on paper or other recording medium where the recording was performed using a typewriter, typesetter, computer or similar mechanism."; 226 case NULL: 227 return null; 228 default: 229 return "?"; 230 } 231 } 232 233 public String getDisplay() { 234 switch (this) { 235 case ELECTRONIC: 236 return "electronic data"; 237 case PHYSICAL: 238 return "physical presence"; 239 case REMOTE: 240 return "remote presence"; 241 case VERBAL: 242 return "verbal"; 243 case DICTATE: 244 return "dictated"; 245 case FACE: 246 return "face-to-face"; 247 case PHONE: 248 return "telephone"; 249 case VIDEOCONF: 250 return "videoconferencing"; 251 case WRITTEN: 252 return "written"; 253 case FAXWRIT: 254 return "telefax"; 255 case HANDWRIT: 256 return "handwritten"; 257 case MAILWRIT: 258 return "mail"; 259 case ONLINEWRIT: 260 return "online written"; 261 case EMAILWRIT: 262 return "email"; 263 case TYPEWRIT: 264 return "typewritten"; 265 case NULL: 266 return null; 267 default: 268 return "?"; 269 } 270 } 271 272}