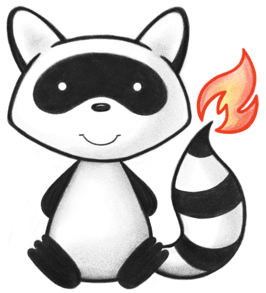
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum V3PaymentTerms { 037 038 /** 039 * Payment in full for products and/or services is required as soon as the 040 * service is performed or goods delivered. 041 */ 042 COD, 043 /** 044 * Payment in full for products and/or services is required 30 days from the 045 * time the service is performed or goods delivered. 046 */ 047 N30, 048 /** 049 * Payment in full for products and/or services is required 60 days from the 050 * time the service is performed or goods delivered. 051 */ 052 N60, 053 /** 054 * Payment in full for products and/or services is required 90 days from the 055 * time the service is performed or goods delivered. 056 */ 057 N90, 058 /** 059 * added to help the parsers 060 */ 061 NULL; 062 063 public static V3PaymentTerms fromCode(String codeString) throws FHIRException { 064 if (codeString == null || "".equals(codeString)) 065 return null; 066 if ("COD".equals(codeString)) 067 return COD; 068 if ("N30".equals(codeString)) 069 return N30; 070 if ("N60".equals(codeString)) 071 return N60; 072 if ("N90".equals(codeString)) 073 return N90; 074 throw new FHIRException("Unknown V3PaymentTerms code '" + codeString + "'"); 075 } 076 077 public String toCode() { 078 switch (this) { 079 case COD: 080 return "COD"; 081 case N30: 082 return "N30"; 083 case N60: 084 return "N60"; 085 case N90: 086 return "N90"; 087 case NULL: 088 return null; 089 default: 090 return "?"; 091 } 092 } 093 094 public String getSystem() { 095 return "http://terminology.hl7.org/CodeSystem/v3-PaymentTerms"; 096 } 097 098 public String getDefinition() { 099 switch (this) { 100 case COD: 101 return "Payment in full for products and/or services is required as soon as the service is performed or goods delivered."; 102 case N30: 103 return "Payment in full for products and/or services is required 30 days from the time the service is performed or goods delivered."; 104 case N60: 105 return "Payment in full for products and/or services is required 60 days from the time the service is performed or goods delivered."; 106 case N90: 107 return "Payment in full for products and/or services is required 90 days from the time the service is performed or goods delivered."; 108 case NULL: 109 return null; 110 default: 111 return "?"; 112 } 113 } 114 115 public String getDisplay() { 116 switch (this) { 117 case COD: 118 return "Cash on Delivery"; 119 case N30: 120 return "Net 30 days"; 121 case N60: 122 return "Net 60 days"; 123 case N90: 124 return "Net 90 days"; 125 case NULL: 126 return null; 127 default: 128 return "?"; 129 } 130 } 131 132}