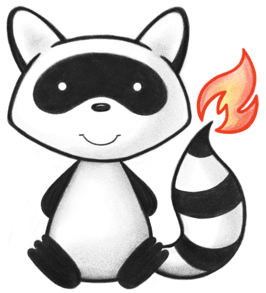
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.r4.model.EnumFactory; 035 036public class V3RaceEnumFactory implements EnumFactory<V3Race> { 037 038 public V3Race fromCode(String codeString) throws IllegalArgumentException { 039 if (codeString == null || "".equals(codeString)) 040 return null; 041 if ("1002-5".equals(codeString)) 042 return V3Race._10025; 043 if ("1004-1".equals(codeString)) 044 return V3Race._10041; 045 if ("1006-6".equals(codeString)) 046 return V3Race._10066; 047 if ("1008-2".equals(codeString)) 048 return V3Race._10082; 049 if ("1010-8".equals(codeString)) 050 return V3Race._10108; 051 if ("1011-6".equals(codeString)) 052 return V3Race._10116; 053 if ("1012-4".equals(codeString)) 054 return V3Race._10124; 055 if ("1013-2".equals(codeString)) 056 return V3Race._10132; 057 if ("1014-0".equals(codeString)) 058 return V3Race._10140; 059 if ("1015-7".equals(codeString)) 060 return V3Race._10157; 061 if ("1016-5".equals(codeString)) 062 return V3Race._10165; 063 if ("1017-3".equals(codeString)) 064 return V3Race._10173; 065 if ("1018-1".equals(codeString)) 066 return V3Race._10181; 067 if ("1019-9".equals(codeString)) 068 return V3Race._10199; 069 if ("1021-5".equals(codeString)) 070 return V3Race._10215; 071 if ("1022-3".equals(codeString)) 072 return V3Race._10223; 073 if ("1023-1".equals(codeString)) 074 return V3Race._10231; 075 if ("1024-9".equals(codeString)) 076 return V3Race._10249; 077 if ("1026-4".equals(codeString)) 078 return V3Race._10264; 079 if ("1028-0".equals(codeString)) 080 return V3Race._10280; 081 if ("1030-6".equals(codeString)) 082 return V3Race._10306; 083 if ("1031-4".equals(codeString)) 084 return V3Race._10314; 085 if ("1033-0".equals(codeString)) 086 return V3Race._10330; 087 if ("1035-5".equals(codeString)) 088 return V3Race._10355; 089 if ("1037-1".equals(codeString)) 090 return V3Race._10371; 091 if ("1039-7".equals(codeString)) 092 return V3Race._10397; 093 if ("1041-3".equals(codeString)) 094 return V3Race._10413; 095 if ("1042-1".equals(codeString)) 096 return V3Race._10421; 097 if ("1044-7".equals(codeString)) 098 return V3Race._10447; 099 if ("1045-4".equals(codeString)) 100 return V3Race._10454; 101 if ("1046-2".equals(codeString)) 102 return V3Race._10462; 103 if ("1047-0".equals(codeString)) 104 return V3Race._10470; 105 if ("1048-8".equals(codeString)) 106 return V3Race._10488; 107 if ("1049-6".equals(codeString)) 108 return V3Race._10496; 109 if ("1050-4".equals(codeString)) 110 return V3Race._10504; 111 if ("1051-2".equals(codeString)) 112 return V3Race._10512; 113 if ("1053-8".equals(codeString)) 114 return V3Race._10538; 115 if ("1054-6".equals(codeString)) 116 return V3Race._10546; 117 if ("1055-3".equals(codeString)) 118 return V3Race._10553; 119 if ("1056-1".equals(codeString)) 120 return V3Race._10561; 121 if ("1057-9".equals(codeString)) 122 return V3Race._10579; 123 if ("1058-7".equals(codeString)) 124 return V3Race._10587; 125 if ("1059-5".equals(codeString)) 126 return V3Race._10595; 127 if ("1060-3".equals(codeString)) 128 return V3Race._10603; 129 if ("1061-1".equals(codeString)) 130 return V3Race._10611; 131 if ("1062-9".equals(codeString)) 132 return V3Race._10629; 133 if ("1063-7".equals(codeString)) 134 return V3Race._10637; 135 if ("1064-5".equals(codeString)) 136 return V3Race._10645; 137 if ("1065-2".equals(codeString)) 138 return V3Race._10652; 139 if ("1066-0".equals(codeString)) 140 return V3Race._10660; 141 if ("1068-6".equals(codeString)) 142 return V3Race._10686; 143 if ("1069-4".equals(codeString)) 144 return V3Race._10694; 145 if ("1070-2".equals(codeString)) 146 return V3Race._10702; 147 if ("1071-0".equals(codeString)) 148 return V3Race._10710; 149 if ("1072-8".equals(codeString)) 150 return V3Race._10728; 151 if ("1073-6".equals(codeString)) 152 return V3Race._10736; 153 if ("1074-4".equals(codeString)) 154 return V3Race._10744; 155 if ("1076-9".equals(codeString)) 156 return V3Race._10769; 157 if ("1741-8".equals(codeString)) 158 return V3Race._17418; 159 if ("1742-6".equals(codeString)) 160 return V3Race._17426; 161 if ("1743-4".equals(codeString)) 162 return V3Race._17434; 163 if ("1744-2".equals(codeString)) 164 return V3Race._17442; 165 if ("1745-9".equals(codeString)) 166 return V3Race._17459; 167 if ("1746-7".equals(codeString)) 168 return V3Race._17467; 169 if ("1747-5".equals(codeString)) 170 return V3Race._17475; 171 if ("1748-3".equals(codeString)) 172 return V3Race._17483; 173 if ("1749-1".equals(codeString)) 174 return V3Race._17491; 175 if ("1750-9".equals(codeString)) 176 return V3Race._17509; 177 if ("1751-7".equals(codeString)) 178 return V3Race._17517; 179 if ("1752-5".equals(codeString)) 180 return V3Race._17525; 181 if ("1753-3".equals(codeString)) 182 return V3Race._17533; 183 if ("1754-1".equals(codeString)) 184 return V3Race._17541; 185 if ("1755-8".equals(codeString)) 186 return V3Race._17558; 187 if ("1756-6".equals(codeString)) 188 return V3Race._17566; 189 if ("1757-4".equals(codeString)) 190 return V3Race._17574; 191 if ("1758-2".equals(codeString)) 192 return V3Race._17582; 193 if ("1759-0".equals(codeString)) 194 return V3Race._17590; 195 if ("1760-8".equals(codeString)) 196 return V3Race._17608; 197 if ("1761-6".equals(codeString)) 198 return V3Race._17616; 199 if ("1762-4".equals(codeString)) 200 return V3Race._17624; 201 if ("1763-2".equals(codeString)) 202 return V3Race._17632; 203 if ("1764-0".equals(codeString)) 204 return V3Race._17640; 205 if ("1765-7".equals(codeString)) 206 return V3Race._17657; 207 if ("1766-5".equals(codeString)) 208 return V3Race._17665; 209 if ("1767-3".equals(codeString)) 210 return V3Race._17673; 211 if ("1768-1".equals(codeString)) 212 return V3Race._17681; 213 if ("1769-9".equals(codeString)) 214 return V3Race._17699; 215 if ("1770-7".equals(codeString)) 216 return V3Race._17707; 217 if ("1771-5".equals(codeString)) 218 return V3Race._17715; 219 if ("1772-3".equals(codeString)) 220 return V3Race._17723; 221 if ("1773-1".equals(codeString)) 222 return V3Race._17731; 223 if ("1774-9".equals(codeString)) 224 return V3Race._17749; 225 if ("1775-6".equals(codeString)) 226 return V3Race._17756; 227 if ("1776-4".equals(codeString)) 228 return V3Race._17764; 229 if ("1777-2".equals(codeString)) 230 return V3Race._17772; 231 if ("1778-0".equals(codeString)) 232 return V3Race._17780; 233 if ("1779-8".equals(codeString)) 234 return V3Race._17798; 235 if ("1780-6".equals(codeString)) 236 return V3Race._17806; 237 if ("1781-4".equals(codeString)) 238 return V3Race._17814; 239 if ("1782-2".equals(codeString)) 240 return V3Race._17822; 241 if ("1783-0".equals(codeString)) 242 return V3Race._17830; 243 if ("1784-8".equals(codeString)) 244 return V3Race._17848; 245 if ("1785-5".equals(codeString)) 246 return V3Race._17855; 247 if ("1786-3".equals(codeString)) 248 return V3Race._17863; 249 if ("1787-1".equals(codeString)) 250 return V3Race._17871; 251 if ("1788-9".equals(codeString)) 252 return V3Race._17889; 253 if ("1789-7".equals(codeString)) 254 return V3Race._17897; 255 if ("1790-5".equals(codeString)) 256 return V3Race._17905; 257 if ("1791-3".equals(codeString)) 258 return V3Race._17913; 259 if ("1792-1".equals(codeString)) 260 return V3Race._17921; 261 if ("1793-9".equals(codeString)) 262 return V3Race._17939; 263 if ("1794-7".equals(codeString)) 264 return V3Race._17947; 265 if ("1795-4".equals(codeString)) 266 return V3Race._17954; 267 if ("1796-2".equals(codeString)) 268 return V3Race._17962; 269 if ("1797-0".equals(codeString)) 270 return V3Race._17970; 271 if ("1798-8".equals(codeString)) 272 return V3Race._17988; 273 if ("1799-6".equals(codeString)) 274 return V3Race._17996; 275 if ("1800-2".equals(codeString)) 276 return V3Race._18002; 277 if ("1801-0".equals(codeString)) 278 return V3Race._18010; 279 if ("1802-8".equals(codeString)) 280 return V3Race._18028; 281 if ("1803-6".equals(codeString)) 282 return V3Race._18036; 283 if ("1804-4".equals(codeString)) 284 return V3Race._18044; 285 if ("1805-1".equals(codeString)) 286 return V3Race._18051; 287 if ("1806-9".equals(codeString)) 288 return V3Race._18069; 289 if ("1807-7".equals(codeString)) 290 return V3Race._18077; 291 if ("1808-5".equals(codeString)) 292 return V3Race._18085; 293 if ("1809-3".equals(codeString)) 294 return V3Race._18093; 295 if ("1078-5".equals(codeString)) 296 return V3Race._10785; 297 if ("1080-1".equals(codeString)) 298 return V3Race._10801; 299 if ("1082-7".equals(codeString)) 300 return V3Race._10827; 301 if ("1083-5".equals(codeString)) 302 return V3Race._10835; 303 if ("1084-3".equals(codeString)) 304 return V3Race._10843; 305 if ("1086-8".equals(codeString)) 306 return V3Race._10868; 307 if ("1088-4".equals(codeString)) 308 return V3Race._10884; 309 if ("1089-2".equals(codeString)) 310 return V3Race._10892; 311 if ("1090-0".equals(codeString)) 312 return V3Race._10900; 313 if ("1091-8".equals(codeString)) 314 return V3Race._10918; 315 if ("1092-6".equals(codeString)) 316 return V3Race._10926; 317 if ("1093-4".equals(codeString)) 318 return V3Race._10934; 319 if ("1094-2".equals(codeString)) 320 return V3Race._10942; 321 if ("1095-9".equals(codeString)) 322 return V3Race._10959; 323 if ("1096-7".equals(codeString)) 324 return V3Race._10967; 325 if ("1097-5".equals(codeString)) 326 return V3Race._10975; 327 if ("1098-3".equals(codeString)) 328 return V3Race._10983; 329 if ("1100-7".equals(codeString)) 330 return V3Race._11007; 331 if ("1102-3".equals(codeString)) 332 return V3Race._11023; 333 if ("1103-1".equals(codeString)) 334 return V3Race._11031; 335 if ("1104-9".equals(codeString)) 336 return V3Race._11049; 337 if ("1106-4".equals(codeString)) 338 return V3Race._11064; 339 if ("1108-0".equals(codeString)) 340 return V3Race._11080; 341 if ("1109-8".equals(codeString)) 342 return V3Race._11098; 343 if ("1110-6".equals(codeString)) 344 return V3Race._11106; 345 if ("1112-2".equals(codeString)) 346 return V3Race._11122; 347 if ("1114-8".equals(codeString)) 348 return V3Race._11148; 349 if ("1115-5".equals(codeString)) 350 return V3Race._11155; 351 if ("1116-3".equals(codeString)) 352 return V3Race._11163; 353 if ("1117-1".equals(codeString)) 354 return V3Race._11171; 355 if ("1118-9".equals(codeString)) 356 return V3Race._11189; 357 if ("1119-7".equals(codeString)) 358 return V3Race._11197; 359 if ("1120-5".equals(codeString)) 360 return V3Race._11205; 361 if ("1121-3".equals(codeString)) 362 return V3Race._11213; 363 if ("1123-9".equals(codeString)) 364 return V3Race._11239; 365 if ("1124-7".equals(codeString)) 366 return V3Race._11247; 367 if ("1125-4".equals(codeString)) 368 return V3Race._11254; 369 if ("1126-2".equals(codeString)) 370 return V3Race._11262; 371 if ("1127-0".equals(codeString)) 372 return V3Race._11270; 373 if ("1128-8".equals(codeString)) 374 return V3Race._11288; 375 if ("1129-6".equals(codeString)) 376 return V3Race._11296; 377 if ("1130-4".equals(codeString)) 378 return V3Race._11304; 379 if ("1131-2".equals(codeString)) 380 return V3Race._11312; 381 if ("1132-0".equals(codeString)) 382 return V3Race._11320; 383 if ("1133-8".equals(codeString)) 384 return V3Race._11338; 385 if ("1134-6".equals(codeString)) 386 return V3Race._11346; 387 if ("1135-3".equals(codeString)) 388 return V3Race._11353; 389 if ("1136-1".equals(codeString)) 390 return V3Race._11361; 391 if ("1137-9".equals(codeString)) 392 return V3Race._11379; 393 if ("1138-7".equals(codeString)) 394 return V3Race._11387; 395 if ("1139-5".equals(codeString)) 396 return V3Race._11395; 397 if ("1140-3".equals(codeString)) 398 return V3Race._11403; 399 if ("1141-1".equals(codeString)) 400 return V3Race._11411; 401 if ("1142-9".equals(codeString)) 402 return V3Race._11429; 403 if ("1143-7".equals(codeString)) 404 return V3Race._11437; 405 if ("1144-5".equals(codeString)) 406 return V3Race._11445; 407 if ("1145-2".equals(codeString)) 408 return V3Race._11452; 409 if ("1146-0".equals(codeString)) 410 return V3Race._11460; 411 if ("1147-8".equals(codeString)) 412 return V3Race._11478; 413 if ("1148-6".equals(codeString)) 414 return V3Race._11486; 415 if ("1150-2".equals(codeString)) 416 return V3Race._11502; 417 if ("1151-0".equals(codeString)) 418 return V3Race._11510; 419 if ("1153-6".equals(codeString)) 420 return V3Race._11536; 421 if ("1155-1".equals(codeString)) 422 return V3Race._11551; 423 if ("1156-9".equals(codeString)) 424 return V3Race._11569; 425 if ("1157-7".equals(codeString)) 426 return V3Race._11577; 427 if ("1158-5".equals(codeString)) 428 return V3Race._11585; 429 if ("1159-3".equals(codeString)) 430 return V3Race._11593; 431 if ("1160-1".equals(codeString)) 432 return V3Race._11601; 433 if ("1162-7".equals(codeString)) 434 return V3Race._11627; 435 if ("1163-5".equals(codeString)) 436 return V3Race._11635; 437 if ("1165-0".equals(codeString)) 438 return V3Race._11650; 439 if ("1167-6".equals(codeString)) 440 return V3Race._11676; 441 if ("1169-2".equals(codeString)) 442 return V3Race._11692; 443 if ("1171-8".equals(codeString)) 444 return V3Race._11718; 445 if ("1173-4".equals(codeString)) 446 return V3Race._11734; 447 if ("1175-9".equals(codeString)) 448 return V3Race._11759; 449 if ("1176-7".equals(codeString)) 450 return V3Race._11767; 451 if ("1178-3".equals(codeString)) 452 return V3Race._11783; 453 if ("1180-9".equals(codeString)) 454 return V3Race._11809; 455 if ("1182-5".equals(codeString)) 456 return V3Race._11825; 457 if ("1184-1".equals(codeString)) 458 return V3Race._11841; 459 if ("1186-6".equals(codeString)) 460 return V3Race._11866; 461 if ("1187-4".equals(codeString)) 462 return V3Race._11874; 463 if ("1189-0".equals(codeString)) 464 return V3Race._11890; 465 if ("1191-6".equals(codeString)) 466 return V3Race._11916; 467 if ("1193-2".equals(codeString)) 468 return V3Race._11932; 469 if ("1194-0".equals(codeString)) 470 return V3Race._11940; 471 if ("1195-7".equals(codeString)) 472 return V3Race._11957; 473 if ("1196-5".equals(codeString)) 474 return V3Race._11965; 475 if ("1197-3".equals(codeString)) 476 return V3Race._11973; 477 if ("1198-1".equals(codeString)) 478 return V3Race._11981; 479 if ("1199-9".equals(codeString)) 480 return V3Race._11999; 481 if ("1200-5".equals(codeString)) 482 return V3Race._12005; 483 if ("1201-3".equals(codeString)) 484 return V3Race._12013; 485 if ("1202-1".equals(codeString)) 486 return V3Race._12021; 487 if ("1203-9".equals(codeString)) 488 return V3Race._12039; 489 if ("1204-7".equals(codeString)) 490 return V3Race._12047; 491 if ("1205-4".equals(codeString)) 492 return V3Race._12054; 493 if ("1207-0".equals(codeString)) 494 return V3Race._12070; 495 if ("1209-6".equals(codeString)) 496 return V3Race._12096; 497 if ("1211-2".equals(codeString)) 498 return V3Race._12112; 499 if ("1212-0".equals(codeString)) 500 return V3Race._12120; 501 if ("1214-6".equals(codeString)) 502 return V3Race._12146; 503 if ("1215-3".equals(codeString)) 504 return V3Race._12153; 505 if ("1216-1".equals(codeString)) 506 return V3Race._12161; 507 if ("1217-9".equals(codeString)) 508 return V3Race._12179; 509 if ("1218-7".equals(codeString)) 510 return V3Race._12187; 511 if ("1219-5".equals(codeString)) 512 return V3Race._12195; 513 if ("1220-3".equals(codeString)) 514 return V3Race._12203; 515 if ("1222-9".equals(codeString)) 516 return V3Race._12229; 517 if ("1223-7".equals(codeString)) 518 return V3Race._12237; 519 if ("1224-5".equals(codeString)) 520 return V3Race._12245; 521 if ("1225-2".equals(codeString)) 522 return V3Race._12252; 523 if ("1226-0".equals(codeString)) 524 return V3Race._12260; 525 if ("1227-8".equals(codeString)) 526 return V3Race._12278; 527 if ("1228-6".equals(codeString)) 528 return V3Race._12286; 529 if ("1229-4".equals(codeString)) 530 return V3Race._12294; 531 if ("1230-2".equals(codeString)) 532 return V3Race._12302; 533 if ("1231-0".equals(codeString)) 534 return V3Race._12310; 535 if ("1233-6".equals(codeString)) 536 return V3Race._12336; 537 if ("1234-4".equals(codeString)) 538 return V3Race._12344; 539 if ("1235-1".equals(codeString)) 540 return V3Race._12351; 541 if ("1236-9".equals(codeString)) 542 return V3Race._12369; 543 if ("1237-7".equals(codeString)) 544 return V3Race._12377; 545 if ("1238-5".equals(codeString)) 546 return V3Race._12385; 547 if ("1239-3".equals(codeString)) 548 return V3Race._12393; 549 if ("1240-1".equals(codeString)) 550 return V3Race._12401; 551 if ("1241-9".equals(codeString)) 552 return V3Race._12419; 553 if ("1242-7".equals(codeString)) 554 return V3Race._12427; 555 if ("1243-5".equals(codeString)) 556 return V3Race._12435; 557 if ("1244-3".equals(codeString)) 558 return V3Race._12443; 559 if ("1245-0".equals(codeString)) 560 return V3Race._12450; 561 if ("1246-8".equals(codeString)) 562 return V3Race._12468; 563 if ("1247-6".equals(codeString)) 564 return V3Race._12476; 565 if ("1248-4".equals(codeString)) 566 return V3Race._12484; 567 if ("1250-0".equals(codeString)) 568 return V3Race._12500; 569 if ("1252-6".equals(codeString)) 570 return V3Race._12526; 571 if ("1254-2".equals(codeString)) 572 return V3Race._12542; 573 if ("1256-7".equals(codeString)) 574 return V3Race._12567; 575 if ("1258-3".equals(codeString)) 576 return V3Race._12583; 577 if ("1260-9".equals(codeString)) 578 return V3Race._12609; 579 if ("1262-5".equals(codeString)) 580 return V3Race._12625; 581 if ("1264-1".equals(codeString)) 582 return V3Race._12641; 583 if ("1265-8".equals(codeString)) 584 return V3Race._12658; 585 if ("1267-4".equals(codeString)) 586 return V3Race._12674; 587 if ("1269-0".equals(codeString)) 588 return V3Race._12690; 589 if ("1271-6".equals(codeString)) 590 return V3Race._12716; 591 if ("1272-4".equals(codeString)) 592 return V3Race._12724; 593 if ("1273-2".equals(codeString)) 594 return V3Race._12732; 595 if ("1275-7".equals(codeString)) 596 return V3Race._12757; 597 if ("1277-3".equals(codeString)) 598 return V3Race._12773; 599 if ("1279-9".equals(codeString)) 600 return V3Race._12799; 601 if ("1281-5".equals(codeString)) 602 return V3Race._12815; 603 if ("1282-3".equals(codeString)) 604 return V3Race._12823; 605 if ("1283-1".equals(codeString)) 606 return V3Race._12831; 607 if ("1285-6".equals(codeString)) 608 return V3Race._12856; 609 if ("1286-4".equals(codeString)) 610 return V3Race._12864; 611 if ("1287-2".equals(codeString)) 612 return V3Race._12872; 613 if ("1288-0".equals(codeString)) 614 return V3Race._12880; 615 if ("1289-8".equals(codeString)) 616 return V3Race._12898; 617 if ("1290-6".equals(codeString)) 618 return V3Race._12906; 619 if ("1291-4".equals(codeString)) 620 return V3Race._12914; 621 if ("1292-2".equals(codeString)) 622 return V3Race._12922; 623 if ("1293-0".equals(codeString)) 624 return V3Race._12930; 625 if ("1294-8".equals(codeString)) 626 return V3Race._12948; 627 if ("1295-5".equals(codeString)) 628 return V3Race._12955; 629 if ("1297-1".equals(codeString)) 630 return V3Race._12971; 631 if ("1299-7".equals(codeString)) 632 return V3Race._12997; 633 if ("1301-1".equals(codeString)) 634 return V3Race._13011; 635 if ("1303-7".equals(codeString)) 636 return V3Race._13037; 637 if ("1305-2".equals(codeString)) 638 return V3Race._13052; 639 if ("1306-0".equals(codeString)) 640 return V3Race._13060; 641 if ("1307-8".equals(codeString)) 642 return V3Race._13078; 643 if ("1309-4".equals(codeString)) 644 return V3Race._13094; 645 if ("1310-2".equals(codeString)) 646 return V3Race._13102; 647 if ("1312-8".equals(codeString)) 648 return V3Race._13128; 649 if ("1313-6".equals(codeString)) 650 return V3Race._13136; 651 if ("1314-4".equals(codeString)) 652 return V3Race._13144; 653 if ("1315-1".equals(codeString)) 654 return V3Race._13151; 655 if ("1317-7".equals(codeString)) 656 return V3Race._13177; 657 if ("1319-3".equals(codeString)) 658 return V3Race._13193; 659 if ("1321-9".equals(codeString)) 660 return V3Race._13219; 661 if ("1323-5".equals(codeString)) 662 return V3Race._13235; 663 if ("1325-0".equals(codeString)) 664 return V3Race._13250; 665 if ("1326-8".equals(codeString)) 666 return V3Race._13268; 667 if ("1327-6".equals(codeString)) 668 return V3Race._13276; 669 if ("1328-4".equals(codeString)) 670 return V3Race._13284; 671 if ("1329-2".equals(codeString)) 672 return V3Race._13292; 673 if ("1331-8".equals(codeString)) 674 return V3Race._13318; 675 if ("1332-6".equals(codeString)) 676 return V3Race._13326; 677 if ("1333-4".equals(codeString)) 678 return V3Race._13334; 679 if ("1334-2".equals(codeString)) 680 return V3Race._13342; 681 if ("1335-9".equals(codeString)) 682 return V3Race._13359; 683 if ("1336-7".equals(codeString)) 684 return V3Race._13367; 685 if ("1337-5".equals(codeString)) 686 return V3Race._13375; 687 if ("1338-3".equals(codeString)) 688 return V3Race._13383; 689 if ("1340-9".equals(codeString)) 690 return V3Race._13409; 691 if ("1342-5".equals(codeString)) 692 return V3Race._13425; 693 if ("1344-1".equals(codeString)) 694 return V3Race._13441; 695 if ("1345-8".equals(codeString)) 696 return V3Race._13458; 697 if ("1346-6".equals(codeString)) 698 return V3Race._13466; 699 if ("1348-2".equals(codeString)) 700 return V3Race._13482; 701 if ("1350-8".equals(codeString)) 702 return V3Race._13508; 703 if ("1352-4".equals(codeString)) 704 return V3Race._13524; 705 if ("1354-0".equals(codeString)) 706 return V3Race._13540; 707 if ("1356-5".equals(codeString)) 708 return V3Race._13565; 709 if ("1358-1".equals(codeString)) 710 return V3Race._13581; 711 if ("1359-9".equals(codeString)) 712 return V3Race._13599; 713 if ("1360-7".equals(codeString)) 714 return V3Race._13607; 715 if ("1361-5".equals(codeString)) 716 return V3Race._13615; 717 if ("1363-1".equals(codeString)) 718 return V3Race._13631; 719 if ("1365-6".equals(codeString)) 720 return V3Race._13656; 721 if ("1366-4".equals(codeString)) 722 return V3Race._13664; 723 if ("1368-0".equals(codeString)) 724 return V3Race._13680; 725 if ("1370-6".equals(codeString)) 726 return V3Race._13706; 727 if ("1372-2".equals(codeString)) 728 return V3Race._13722; 729 if ("1374-8".equals(codeString)) 730 return V3Race._13748; 731 if ("1376-3".equals(codeString)) 732 return V3Race._13763; 733 if ("1378-9".equals(codeString)) 734 return V3Race._13789; 735 if ("1380-5".equals(codeString)) 736 return V3Race._13805; 737 if ("1382-1".equals(codeString)) 738 return V3Race._13821; 739 if ("1383-9".equals(codeString)) 740 return V3Race._13839; 741 if ("1384-7".equals(codeString)) 742 return V3Race._13847; 743 if ("1385-4".equals(codeString)) 744 return V3Race._13854; 745 if ("1387-0".equals(codeString)) 746 return V3Race._13870; 747 if ("1389-6".equals(codeString)) 748 return V3Race._13896; 749 if ("1391-2".equals(codeString)) 750 return V3Race._13912; 751 if ("1392-0".equals(codeString)) 752 return V3Race._13920; 753 if ("1393-8".equals(codeString)) 754 return V3Race._13938; 755 if ("1394-6".equals(codeString)) 756 return V3Race._13946; 757 if ("1395-3".equals(codeString)) 758 return V3Race._13953; 759 if ("1396-1".equals(codeString)) 760 return V3Race._13961; 761 if ("1397-9".equals(codeString)) 762 return V3Race._13979; 763 if ("1398-7".equals(codeString)) 764 return V3Race._13987; 765 if ("1399-5".equals(codeString)) 766 return V3Race._13995; 767 if ("1400-1".equals(codeString)) 768 return V3Race._14001; 769 if ("1401-9".equals(codeString)) 770 return V3Race._14019; 771 if ("1403-5".equals(codeString)) 772 return V3Race._14035; 773 if ("1405-0".equals(codeString)) 774 return V3Race._14050; 775 if ("1407-6".equals(codeString)) 776 return V3Race._14076; 777 if ("1409-2".equals(codeString)) 778 return V3Race._14092; 779 if ("1411-8".equals(codeString)) 780 return V3Race._14118; 781 if ("1412-6".equals(codeString)) 782 return V3Race._14126; 783 if ("1413-4".equals(codeString)) 784 return V3Race._14134; 785 if ("1414-2".equals(codeString)) 786 return V3Race._14142; 787 if ("1416-7".equals(codeString)) 788 return V3Race._14167; 789 if ("1417-5".equals(codeString)) 790 return V3Race._14175; 791 if ("1418-3".equals(codeString)) 792 return V3Race._14183; 793 if ("1419-1".equals(codeString)) 794 return V3Race._14191; 795 if ("1420-9".equals(codeString)) 796 return V3Race._14209; 797 if ("1421-7".equals(codeString)) 798 return V3Race._14217; 799 if ("1422-5".equals(codeString)) 800 return V3Race._14225; 801 if ("1423-3".equals(codeString)) 802 return V3Race._14233; 803 if ("1424-1".equals(codeString)) 804 return V3Race._14241; 805 if ("1425-8".equals(codeString)) 806 return V3Race._14258; 807 if ("1426-6".equals(codeString)) 808 return V3Race._14266; 809 if ("1427-4".equals(codeString)) 810 return V3Race._14274; 811 if ("1428-2".equals(codeString)) 812 return V3Race._14282; 813 if ("1429-0".equals(codeString)) 814 return V3Race._14290; 815 if ("1430-8".equals(codeString)) 816 return V3Race._14308; 817 if ("1431-6".equals(codeString)) 818 return V3Race._14316; 819 if ("1432-4".equals(codeString)) 820 return V3Race._14324; 821 if ("1433-2".equals(codeString)) 822 return V3Race._14332; 823 if ("1434-0".equals(codeString)) 824 return V3Race._14340; 825 if ("1435-7".equals(codeString)) 826 return V3Race._14357; 827 if ("1436-5".equals(codeString)) 828 return V3Race._14365; 829 if ("1437-3".equals(codeString)) 830 return V3Race._14373; 831 if ("1439-9".equals(codeString)) 832 return V3Race._14399; 833 if ("1441-5".equals(codeString)) 834 return V3Race._14415; 835 if ("1442-3".equals(codeString)) 836 return V3Race._14423; 837 if ("1443-1".equals(codeString)) 838 return V3Race._14431; 839 if ("1445-6".equals(codeString)) 840 return V3Race._14456; 841 if ("1446-4".equals(codeString)) 842 return V3Race._14464; 843 if ("1448-0".equals(codeString)) 844 return V3Race._14480; 845 if ("1450-6".equals(codeString)) 846 return V3Race._14506; 847 if ("1451-4".equals(codeString)) 848 return V3Race._14514; 849 if ("1453-0".equals(codeString)) 850 return V3Race._14530; 851 if ("1454-8".equals(codeString)) 852 return V3Race._14548; 853 if ("1456-3".equals(codeString)) 854 return V3Race._14563; 855 if ("1457-1".equals(codeString)) 856 return V3Race._14571; 857 if ("1458-9".equals(codeString)) 858 return V3Race._14589; 859 if ("1460-5".equals(codeString)) 860 return V3Race._14605; 861 if ("1462-1".equals(codeString)) 862 return V3Race._14621; 863 if ("1464-7".equals(codeString)) 864 return V3Race._14647; 865 if ("1465-4".equals(codeString)) 866 return V3Race._14654; 867 if ("1466-2".equals(codeString)) 868 return V3Race._14662; 869 if ("1467-0".equals(codeString)) 870 return V3Race._14670; 871 if ("1468-8".equals(codeString)) 872 return V3Race._14688; 873 if ("1469-6".equals(codeString)) 874 return V3Race._14696; 875 if ("1470-4".equals(codeString)) 876 return V3Race._14704; 877 if ("1471-2".equals(codeString)) 878 return V3Race._14712; 879 if ("1472-0".equals(codeString)) 880 return V3Race._14720; 881 if ("1474-6".equals(codeString)) 882 return V3Race._14746; 883 if ("1475-3".equals(codeString)) 884 return V3Race._14753; 885 if ("1476-1".equals(codeString)) 886 return V3Race._14761; 887 if ("1478-7".equals(codeString)) 888 return V3Race._14787; 889 if ("1479-5".equals(codeString)) 890 return V3Race._14795; 891 if ("1480-3".equals(codeString)) 892 return V3Race._14803; 893 if ("1481-1".equals(codeString)) 894 return V3Race._14811; 895 if ("1482-9".equals(codeString)) 896 return V3Race._14829; 897 if ("1483-7".equals(codeString)) 898 return V3Race._14837; 899 if ("1484-5".equals(codeString)) 900 return V3Race._14845; 901 if ("1485-2".equals(codeString)) 902 return V3Race._14852; 903 if ("1487-8".equals(codeString)) 904 return V3Race._14878; 905 if ("1489-4".equals(codeString)) 906 return V3Race._14894; 907 if ("1490-2".equals(codeString)) 908 return V3Race._14902; 909 if ("1491-0".equals(codeString)) 910 return V3Race._14910; 911 if ("1492-8".equals(codeString)) 912 return V3Race._14928; 913 if ("1493-6".equals(codeString)) 914 return V3Race._14936; 915 if ("1494-4".equals(codeString)) 916 return V3Race._14944; 917 if ("1495-1".equals(codeString)) 918 return V3Race._14951; 919 if ("1496-9".equals(codeString)) 920 return V3Race._14969; 921 if ("1497-7".equals(codeString)) 922 return V3Race._14977; 923 if ("1498-5".equals(codeString)) 924 return V3Race._14985; 925 if ("1499-3".equals(codeString)) 926 return V3Race._14993; 927 if ("1500-8".equals(codeString)) 928 return V3Race._15008; 929 if ("1501-6".equals(codeString)) 930 return V3Race._15016; 931 if ("1502-4".equals(codeString)) 932 return V3Race._15024; 933 if ("1503-2".equals(codeString)) 934 return V3Race._15032; 935 if ("1504-0".equals(codeString)) 936 return V3Race._15040; 937 if ("1505-7".equals(codeString)) 938 return V3Race._15057; 939 if ("1506-5".equals(codeString)) 940 return V3Race._15065; 941 if ("1507-3".equals(codeString)) 942 return V3Race._15073; 943 if ("1508-1".equals(codeString)) 944 return V3Race._15081; 945 if ("1509-9".equals(codeString)) 946 return V3Race._15099; 947 if ("1510-7".equals(codeString)) 948 return V3Race._15107; 949 if ("1511-5".equals(codeString)) 950 return V3Race._15115; 951 if ("1512-3".equals(codeString)) 952 return V3Race._15123; 953 if ("1513-1".equals(codeString)) 954 return V3Race._15131; 955 if ("1514-9".equals(codeString)) 956 return V3Race._15149; 957 if ("1515-6".equals(codeString)) 958 return V3Race._15156; 959 if ("1516-4".equals(codeString)) 960 return V3Race._15164; 961 if ("1518-0".equals(codeString)) 962 return V3Race._15180; 963 if ("1519-8".equals(codeString)) 964 return V3Race._15198; 965 if ("1520-6".equals(codeString)) 966 return V3Race._15206; 967 if ("1521-4".equals(codeString)) 968 return V3Race._15214; 969 if ("1522-2".equals(codeString)) 970 return V3Race._15222; 971 if ("1523-0".equals(codeString)) 972 return V3Race._15230; 973 if ("1524-8".equals(codeString)) 974 return V3Race._15248; 975 if ("1525-5".equals(codeString)) 976 return V3Race._15255; 977 if ("1526-3".equals(codeString)) 978 return V3Race._15263; 979 if ("1527-1".equals(codeString)) 980 return V3Race._15271; 981 if ("1528-9".equals(codeString)) 982 return V3Race._15289; 983 if ("1529-7".equals(codeString)) 984 return V3Race._15297; 985 if ("1530-5".equals(codeString)) 986 return V3Race._15305; 987 if ("1531-3".equals(codeString)) 988 return V3Race._15313; 989 if ("1532-1".equals(codeString)) 990 return V3Race._15321; 991 if ("1533-9".equals(codeString)) 992 return V3Race._15339; 993 if ("1534-7".equals(codeString)) 994 return V3Race._15347; 995 if ("1535-4".equals(codeString)) 996 return V3Race._15354; 997 if ("1536-2".equals(codeString)) 998 return V3Race._15362; 999 if ("1537-0".equals(codeString)) 1000 return V3Race._15370; 1001 if ("1538-8".equals(codeString)) 1002 return V3Race._15388; 1003 if ("1539-6".equals(codeString)) 1004 return V3Race._15396; 1005 if ("1541-2".equals(codeString)) 1006 return V3Race._15412; 1007 if ("1543-8".equals(codeString)) 1008 return V3Race._15438; 1009 if ("1545-3".equals(codeString)) 1010 return V3Race._15453; 1011 if ("1547-9".equals(codeString)) 1012 return V3Race._15479; 1013 if ("1549-5".equals(codeString)) 1014 return V3Race._15495; 1015 if ("1551-1".equals(codeString)) 1016 return V3Race._15511; 1017 if ("1552-9".equals(codeString)) 1018 return V3Race._15529; 1019 if ("1553-7".equals(codeString)) 1020 return V3Race._15537; 1021 if ("1554-5".equals(codeString)) 1022 return V3Race._15545; 1023 if ("1556-0".equals(codeString)) 1024 return V3Race._15560; 1025 if ("1558-6".equals(codeString)) 1026 return V3Race._15586; 1027 if ("1560-2".equals(codeString)) 1028 return V3Race._15602; 1029 if ("1562-8".equals(codeString)) 1030 return V3Race._15628; 1031 if ("1564-4".equals(codeString)) 1032 return V3Race._15644; 1033 if ("1566-9".equals(codeString)) 1034 return V3Race._15669; 1035 if ("1567-7".equals(codeString)) 1036 return V3Race._15677; 1037 if ("1568-5".equals(codeString)) 1038 return V3Race._15685; 1039 if ("1569-3".equals(codeString)) 1040 return V3Race._15693; 1041 if ("1570-1".equals(codeString)) 1042 return V3Race._15701; 1043 if ("1571-9".equals(codeString)) 1044 return V3Race._15719; 1045 if ("1573-5".equals(codeString)) 1046 return V3Race._15735; 1047 if ("1574-3".equals(codeString)) 1048 return V3Race._15743; 1049 if ("1576-8".equals(codeString)) 1050 return V3Race._15768; 1051 if ("1578-4".equals(codeString)) 1052 return V3Race._15784; 1053 if ("1579-2".equals(codeString)) 1054 return V3Race._15792; 1055 if ("1580-0".equals(codeString)) 1056 return V3Race._15800; 1057 if ("1582-6".equals(codeString)) 1058 return V3Race._15826; 1059 if ("1584-2".equals(codeString)) 1060 return V3Race._15842; 1061 if ("1586-7".equals(codeString)) 1062 return V3Race._15867; 1063 if ("1587-5".equals(codeString)) 1064 return V3Race._15875; 1065 if ("1588-3".equals(codeString)) 1066 return V3Race._15883; 1067 if ("1589-1".equals(codeString)) 1068 return V3Race._15891; 1069 if ("1590-9".equals(codeString)) 1070 return V3Race._15909; 1071 if ("1591-7".equals(codeString)) 1072 return V3Race._15917; 1073 if ("1592-5".equals(codeString)) 1074 return V3Race._15925; 1075 if ("1593-3".equals(codeString)) 1076 return V3Race._15933; 1077 if ("1594-1".equals(codeString)) 1078 return V3Race._15941; 1079 if ("1595-8".equals(codeString)) 1080 return V3Race._15958; 1081 if ("1596-6".equals(codeString)) 1082 return V3Race._15966; 1083 if ("1597-4".equals(codeString)) 1084 return V3Race._15974; 1085 if ("1598-2".equals(codeString)) 1086 return V3Race._15982; 1087 if ("1599-0".equals(codeString)) 1088 return V3Race._15990; 1089 if ("1600-6".equals(codeString)) 1090 return V3Race._16006; 1091 if ("1602-2".equals(codeString)) 1092 return V3Race._16022; 1093 if ("1603-0".equals(codeString)) 1094 return V3Race._16030; 1095 if ("1604-8".equals(codeString)) 1096 return V3Race._16048; 1097 if ("1605-5".equals(codeString)) 1098 return V3Race._16055; 1099 if ("1607-1".equals(codeString)) 1100 return V3Race._16071; 1101 if ("1609-7".equals(codeString)) 1102 return V3Race._16097; 1103 if ("1610-5".equals(codeString)) 1104 return V3Race._16105; 1105 if ("1611-3".equals(codeString)) 1106 return V3Race._16113; 1107 if ("1612-1".equals(codeString)) 1108 return V3Race._16121; 1109 if ("1613-9".equals(codeString)) 1110 return V3Race._16139; 1111 if ("1614-7".equals(codeString)) 1112 return V3Race._16147; 1113 if ("1615-4".equals(codeString)) 1114 return V3Race._16154; 1115 if ("1616-2".equals(codeString)) 1116 return V3Race._16162; 1117 if ("1617-0".equals(codeString)) 1118 return V3Race._16170; 1119 if ("1618-8".equals(codeString)) 1120 return V3Race._16188; 1121 if ("1619-6".equals(codeString)) 1122 return V3Race._16196; 1123 if ("1620-4".equals(codeString)) 1124 return V3Race._16204; 1125 if ("1621-2".equals(codeString)) 1126 return V3Race._16212; 1127 if ("1622-0".equals(codeString)) 1128 return V3Race._16220; 1129 if ("1623-8".equals(codeString)) 1130 return V3Race._16238; 1131 if ("1624-6".equals(codeString)) 1132 return V3Race._16246; 1133 if ("1625-3".equals(codeString)) 1134 return V3Race._16253; 1135 if ("1626-1".equals(codeString)) 1136 return V3Race._16261; 1137 if ("1627-9".equals(codeString)) 1138 return V3Race._16279; 1139 if ("1628-7".equals(codeString)) 1140 return V3Race._16287; 1141 if ("1629-5".equals(codeString)) 1142 return V3Race._16295; 1143 if ("1630-3".equals(codeString)) 1144 return V3Race._16303; 1145 if ("1631-1".equals(codeString)) 1146 return V3Race._16311; 1147 if ("1632-9".equals(codeString)) 1148 return V3Race._16329; 1149 if ("1633-7".equals(codeString)) 1150 return V3Race._16337; 1151 if ("1634-5".equals(codeString)) 1152 return V3Race._16345; 1153 if ("1635-2".equals(codeString)) 1154 return V3Race._16352; 1155 if ("1636-0".equals(codeString)) 1156 return V3Race._16360; 1157 if ("1637-8".equals(codeString)) 1158 return V3Race._16378; 1159 if ("1638-6".equals(codeString)) 1160 return V3Race._16386; 1161 if ("1639-4".equals(codeString)) 1162 return V3Race._16394; 1163 if ("1640-2".equals(codeString)) 1164 return V3Race._16402; 1165 if ("1641-0".equals(codeString)) 1166 return V3Race._16410; 1167 if ("1643-6".equals(codeString)) 1168 return V3Race._16436; 1169 if ("1645-1".equals(codeString)) 1170 return V3Race._16451; 1171 if ("1647-7".equals(codeString)) 1172 return V3Race._16477; 1173 if ("1649-3".equals(codeString)) 1174 return V3Race._16493; 1175 if ("1651-9".equals(codeString)) 1176 return V3Race._16519; 1177 if ("1653-5".equals(codeString)) 1178 return V3Race._16535; 1179 if ("1654-3".equals(codeString)) 1180 return V3Race._16543; 1181 if ("1655-0".equals(codeString)) 1182 return V3Race._16550; 1183 if ("1656-8".equals(codeString)) 1184 return V3Race._16568; 1185 if ("1657-6".equals(codeString)) 1186 return V3Race._16576; 1187 if ("1659-2".equals(codeString)) 1188 return V3Race._16592; 1189 if ("1661-8".equals(codeString)) 1190 return V3Race._16618; 1191 if ("1663-4".equals(codeString)) 1192 return V3Race._16634; 1193 if ("1665-9".equals(codeString)) 1194 return V3Race._16659; 1195 if ("1667-5".equals(codeString)) 1196 return V3Race._16675; 1197 if ("1668-3".equals(codeString)) 1198 return V3Race._16683; 1199 if ("1670-9".equals(codeString)) 1200 return V3Race._16709; 1201 if ("1671-7".equals(codeString)) 1202 return V3Race._16717; 1203 if ("1672-5".equals(codeString)) 1204 return V3Race._16725; 1205 if ("1673-3".equals(codeString)) 1206 return V3Race._16733; 1207 if ("1675-8".equals(codeString)) 1208 return V3Race._16758; 1209 if ("1677-4".equals(codeString)) 1210 return V3Race._16774; 1211 if ("1679-0".equals(codeString)) 1212 return V3Race._16790; 1213 if ("1680-8".equals(codeString)) 1214 return V3Race._16808; 1215 if ("1681-6".equals(codeString)) 1216 return V3Race._16816; 1217 if ("1683-2".equals(codeString)) 1218 return V3Race._16832; 1219 if ("1685-7".equals(codeString)) 1220 return V3Race._16857; 1221 if ("1687-3".equals(codeString)) 1222 return V3Race._16873; 1223 if ("1688-1".equals(codeString)) 1224 return V3Race._16881; 1225 if ("1689-9".equals(codeString)) 1226 return V3Race._16899; 1227 if ("1690-7".equals(codeString)) 1228 return V3Race._16907; 1229 if ("1692-3".equals(codeString)) 1230 return V3Race._16923; 1231 if ("1694-9".equals(codeString)) 1232 return V3Race._16949; 1233 if ("1696-4".equals(codeString)) 1234 return V3Race._16964; 1235 if ("1697-2".equals(codeString)) 1236 return V3Race._16972; 1237 if ("1698-0".equals(codeString)) 1238 return V3Race._16980; 1239 if ("1700-4".equals(codeString)) 1240 return V3Race._17004; 1241 if ("1702-0".equals(codeString)) 1242 return V3Race._17020; 1243 if ("1704-6".equals(codeString)) 1244 return V3Race._17046; 1245 if ("1705-3".equals(codeString)) 1246 return V3Race._17053; 1247 if ("1707-9".equals(codeString)) 1248 return V3Race._17079; 1249 if ("1709-5".equals(codeString)) 1250 return V3Race._17095; 1251 if ("1711-1".equals(codeString)) 1252 return V3Race._17111; 1253 if ("1712-9".equals(codeString)) 1254 return V3Race._17129; 1255 if ("1713-7".equals(codeString)) 1256 return V3Race._17137; 1257 if ("1715-2".equals(codeString)) 1258 return V3Race._17152; 1259 if ("1717-8".equals(codeString)) 1260 return V3Race._17178; 1261 if ("1718-6".equals(codeString)) 1262 return V3Race._17186; 1263 if ("1719-4".equals(codeString)) 1264 return V3Race._17194; 1265 if ("1720-2".equals(codeString)) 1266 return V3Race._17202; 1267 if ("1722-8".equals(codeString)) 1268 return V3Race._17228; 1269 if ("1724-4".equals(codeString)) 1270 return V3Race._17244; 1271 if ("1725-1".equals(codeString)) 1272 return V3Race._17251; 1273 if ("1726-9".equals(codeString)) 1274 return V3Race._17269; 1275 if ("1727-7".equals(codeString)) 1276 return V3Race._17277; 1277 if ("1728-5".equals(codeString)) 1278 return V3Race._17285; 1279 if ("1729-3".equals(codeString)) 1280 return V3Race._17293; 1281 if ("1730-1".equals(codeString)) 1282 return V3Race._17301; 1283 if ("1731-9".equals(codeString)) 1284 return V3Race._17319; 1285 if ("1732-7".equals(codeString)) 1286 return V3Race._17327; 1287 if ("1733-5".equals(codeString)) 1288 return V3Race._17335; 1289 if ("1735-0".equals(codeString)) 1290 return V3Race._17350; 1291 if ("1737-6".equals(codeString)) 1292 return V3Race._17376; 1293 if ("1739-2".equals(codeString)) 1294 return V3Race._17392; 1295 if ("1740-0".equals(codeString)) 1296 return V3Race._17400; 1297 if ("1811-9".equals(codeString)) 1298 return V3Race._18119; 1299 if ("1813-5".equals(codeString)) 1300 return V3Race._18135; 1301 if ("1814-3".equals(codeString)) 1302 return V3Race._18143; 1303 if ("1815-0".equals(codeString)) 1304 return V3Race._18150; 1305 if ("1816-8".equals(codeString)) 1306 return V3Race._18168; 1307 if ("1817-6".equals(codeString)) 1308 return V3Race._18176; 1309 if ("1818-4".equals(codeString)) 1310 return V3Race._18184; 1311 if ("1819-2".equals(codeString)) 1312 return V3Race._18192; 1313 if ("1820-0".equals(codeString)) 1314 return V3Race._18200; 1315 if ("1821-8".equals(codeString)) 1316 return V3Race._18218; 1317 if ("1822-6".equals(codeString)) 1318 return V3Race._18226; 1319 if ("1823-4".equals(codeString)) 1320 return V3Race._18234; 1321 if ("1824-2".equals(codeString)) 1322 return V3Race._18242; 1323 if ("1825-9".equals(codeString)) 1324 return V3Race._18259; 1325 if ("1826-7".equals(codeString)) 1326 return V3Race._18267; 1327 if ("1827-5".equals(codeString)) 1328 return V3Race._18275; 1329 if ("1828-3".equals(codeString)) 1330 return V3Race._18283; 1331 if ("1829-1".equals(codeString)) 1332 return V3Race._18291; 1333 if ("1830-9".equals(codeString)) 1334 return V3Race._18309; 1335 if ("1831-7".equals(codeString)) 1336 return V3Race._18317; 1337 if ("1832-5".equals(codeString)) 1338 return V3Race._18325; 1339 if ("1833-3".equals(codeString)) 1340 return V3Race._18333; 1341 if ("1834-1".equals(codeString)) 1342 return V3Race._18341; 1343 if ("1835-8".equals(codeString)) 1344 return V3Race._18358; 1345 if ("1837-4".equals(codeString)) 1346 return V3Race._18374; 1347 if ("1838-2".equals(codeString)) 1348 return V3Race._18382; 1349 if ("1840-8".equals(codeString)) 1350 return V3Race._18408; 1351 if ("1842-4".equals(codeString)) 1352 return V3Race._18424; 1353 if ("1844-0".equals(codeString)) 1354 return V3Race._18440; 1355 if ("1845-7".equals(codeString)) 1356 return V3Race._18457; 1357 if ("1846-5".equals(codeString)) 1358 return V3Race._18465; 1359 if ("1847-3".equals(codeString)) 1360 return V3Race._18473; 1361 if ("1848-1".equals(codeString)) 1362 return V3Race._18481; 1363 if ("1849-9".equals(codeString)) 1364 return V3Race._18499; 1365 if ("1850-7".equals(codeString)) 1366 return V3Race._18507; 1367 if ("1851-5".equals(codeString)) 1368 return V3Race._18515; 1369 if ("1852-3".equals(codeString)) 1370 return V3Race._18523; 1371 if ("1853-1".equals(codeString)) 1372 return V3Race._18531; 1373 if ("1854-9".equals(codeString)) 1374 return V3Race._18549; 1375 if ("1855-6".equals(codeString)) 1376 return V3Race._18556; 1377 if ("1856-4".equals(codeString)) 1378 return V3Race._18564; 1379 if ("1857-2".equals(codeString)) 1380 return V3Race._18572; 1381 if ("1858-0".equals(codeString)) 1382 return V3Race._18580; 1383 if ("1859-8".equals(codeString)) 1384 return V3Race._18598; 1385 if ("1860-6".equals(codeString)) 1386 return V3Race._18606; 1387 if ("1861-4".equals(codeString)) 1388 return V3Race._18614; 1389 if ("1862-2".equals(codeString)) 1390 return V3Race._18622; 1391 if ("1863-0".equals(codeString)) 1392 return V3Race._18630; 1393 if ("1864-8".equals(codeString)) 1394 return V3Race._18648; 1395 if ("1865-5".equals(codeString)) 1396 return V3Race._18655; 1397 if ("1866-3".equals(codeString)) 1398 return V3Race._18663; 1399 if ("1867-1".equals(codeString)) 1400 return V3Race._18671; 1401 if ("1868-9".equals(codeString)) 1402 return V3Race._18689; 1403 if ("1869-7".equals(codeString)) 1404 return V3Race._18697; 1405 if ("1870-5".equals(codeString)) 1406 return V3Race._18705; 1407 if ("1871-3".equals(codeString)) 1408 return V3Race._18713; 1409 if ("1872-1".equals(codeString)) 1410 return V3Race._18721; 1411 if ("1873-9".equals(codeString)) 1412 return V3Race._18739; 1413 if ("1874-7".equals(codeString)) 1414 return V3Race._18747; 1415 if ("1875-4".equals(codeString)) 1416 return V3Race._18754; 1417 if ("1876-2".equals(codeString)) 1418 return V3Race._18762; 1419 if ("1877-0".equals(codeString)) 1420 return V3Race._18770; 1421 if ("1878-8".equals(codeString)) 1422 return V3Race._18788; 1423 if ("1879-6".equals(codeString)) 1424 return V3Race._18796; 1425 if ("1880-4".equals(codeString)) 1426 return V3Race._18804; 1427 if ("1881-2".equals(codeString)) 1428 return V3Race._18812; 1429 if ("1882-0".equals(codeString)) 1430 return V3Race._18820; 1431 if ("1883-8".equals(codeString)) 1432 return V3Race._18838; 1433 if ("1884-6".equals(codeString)) 1434 return V3Race._18846; 1435 if ("1885-3".equals(codeString)) 1436 return V3Race._18853; 1437 if ("1886-1".equals(codeString)) 1438 return V3Race._18861; 1439 if ("1887-9".equals(codeString)) 1440 return V3Race._18879; 1441 if ("1888-7".equals(codeString)) 1442 return V3Race._18887; 1443 if ("1889-5".equals(codeString)) 1444 return V3Race._18895; 1445 if ("1891-1".equals(codeString)) 1446 return V3Race._18911; 1447 if ("1892-9".equals(codeString)) 1448 return V3Race._18929; 1449 if ("1893-7".equals(codeString)) 1450 return V3Race._18937; 1451 if ("1894-5".equals(codeString)) 1452 return V3Race._18945; 1453 if ("1896-0".equals(codeString)) 1454 return V3Race._18960; 1455 if ("1897-8".equals(codeString)) 1456 return V3Race._18978; 1457 if ("1898-6".equals(codeString)) 1458 return V3Race._18986; 1459 if ("1899-4".equals(codeString)) 1460 return V3Race._18994; 1461 if ("1900-0".equals(codeString)) 1462 return V3Race._19000; 1463 if ("1901-8".equals(codeString)) 1464 return V3Race._19018; 1465 if ("1902-6".equals(codeString)) 1466 return V3Race._19026; 1467 if ("1903-4".equals(codeString)) 1468 return V3Race._19034; 1469 if ("1904-2".equals(codeString)) 1470 return V3Race._19042; 1471 if ("1905-9".equals(codeString)) 1472 return V3Race._19059; 1473 if ("1906-7".equals(codeString)) 1474 return V3Race._19067; 1475 if ("1907-5".equals(codeString)) 1476 return V3Race._19075; 1477 if ("1908-3".equals(codeString)) 1478 return V3Race._19083; 1479 if ("1909-1".equals(codeString)) 1480 return V3Race._19091; 1481 if ("1910-9".equals(codeString)) 1482 return V3Race._19109; 1483 if ("1911-7".equals(codeString)) 1484 return V3Race._19117; 1485 if ("1912-5".equals(codeString)) 1486 return V3Race._19125; 1487 if ("1913-3".equals(codeString)) 1488 return V3Race._19133; 1489 if ("1914-1".equals(codeString)) 1490 return V3Race._19141; 1491 if ("1915-8".equals(codeString)) 1492 return V3Race._19158; 1493 if ("1916-6".equals(codeString)) 1494 return V3Race._19166; 1495 if ("1917-4".equals(codeString)) 1496 return V3Race._19174; 1497 if ("1918-2".equals(codeString)) 1498 return V3Race._19182; 1499 if ("1919-0".equals(codeString)) 1500 return V3Race._19190; 1501 if ("1920-8".equals(codeString)) 1502 return V3Race._19208; 1503 if ("1921-6".equals(codeString)) 1504 return V3Race._19216; 1505 if ("1922-4".equals(codeString)) 1506 return V3Race._19224; 1507 if ("1923-2".equals(codeString)) 1508 return V3Race._19232; 1509 if ("1924-0".equals(codeString)) 1510 return V3Race._19240; 1511 if ("1925-7".equals(codeString)) 1512 return V3Race._19257; 1513 if ("1926-5".equals(codeString)) 1514 return V3Race._19265; 1515 if ("1927-3".equals(codeString)) 1516 return V3Race._19273; 1517 if ("1928-1".equals(codeString)) 1518 return V3Race._19281; 1519 if ("1929-9".equals(codeString)) 1520 return V3Race._19299; 1521 if ("1930-7".equals(codeString)) 1522 return V3Race._19307; 1523 if ("1931-5".equals(codeString)) 1524 return V3Race._19315; 1525 if ("1932-3".equals(codeString)) 1526 return V3Race._19323; 1527 if ("1933-1".equals(codeString)) 1528 return V3Race._19331; 1529 if ("1934-9".equals(codeString)) 1530 return V3Race._19349; 1531 if ("1935-6".equals(codeString)) 1532 return V3Race._19356; 1533 if ("1936-4".equals(codeString)) 1534 return V3Race._19364; 1535 if ("1937-2".equals(codeString)) 1536 return V3Race._19372; 1537 if ("1938-0".equals(codeString)) 1538 return V3Race._19380; 1539 if ("1939-8".equals(codeString)) 1540 return V3Race._19398; 1541 if ("1940-6".equals(codeString)) 1542 return V3Race._19406; 1543 if ("1941-4".equals(codeString)) 1544 return V3Race._19414; 1545 if ("1942-2".equals(codeString)) 1546 return V3Race._19422; 1547 if ("1943-0".equals(codeString)) 1548 return V3Race._19430; 1549 if ("1944-8".equals(codeString)) 1550 return V3Race._19448; 1551 if ("1945-5".equals(codeString)) 1552 return V3Race._19455; 1553 if ("1946-3".equals(codeString)) 1554 return V3Race._19463; 1555 if ("1947-1".equals(codeString)) 1556 return V3Race._19471; 1557 if ("1948-9".equals(codeString)) 1558 return V3Race._19489; 1559 if ("1949-7".equals(codeString)) 1560 return V3Race._19497; 1561 if ("1950-5".equals(codeString)) 1562 return V3Race._19505; 1563 if ("1951-3".equals(codeString)) 1564 return V3Race._19513; 1565 if ("1952-1".equals(codeString)) 1566 return V3Race._19521; 1567 if ("1953-9".equals(codeString)) 1568 return V3Race._19539; 1569 if ("1954-7".equals(codeString)) 1570 return V3Race._19547; 1571 if ("1955-4".equals(codeString)) 1572 return V3Race._19554; 1573 if ("1956-2".equals(codeString)) 1574 return V3Race._19562; 1575 if ("1957-0".equals(codeString)) 1576 return V3Race._19570; 1577 if ("1958-8".equals(codeString)) 1578 return V3Race._19588; 1579 if ("1959-6".equals(codeString)) 1580 return V3Race._19596; 1581 if ("1960-4".equals(codeString)) 1582 return V3Race._19604; 1583 if ("1961-2".equals(codeString)) 1584 return V3Race._19612; 1585 if ("1962-0".equals(codeString)) 1586 return V3Race._19620; 1587 if ("1963-8".equals(codeString)) 1588 return V3Race._19638; 1589 if ("1964-6".equals(codeString)) 1590 return V3Race._19646; 1591 if ("1966-1".equals(codeString)) 1592 return V3Race._19661; 1593 if ("1968-7".equals(codeString)) 1594 return V3Race._19687; 1595 if ("1969-5".equals(codeString)) 1596 return V3Race._19695; 1597 if ("1970-3".equals(codeString)) 1598 return V3Race._19703; 1599 if ("1972-9".equals(codeString)) 1600 return V3Race._19729; 1601 if ("1973-7".equals(codeString)) 1602 return V3Race._19737; 1603 if ("1974-5".equals(codeString)) 1604 return V3Race._19745; 1605 if ("1975-2".equals(codeString)) 1606 return V3Race._19752; 1607 if ("1976-0".equals(codeString)) 1608 return V3Race._19760; 1609 if ("1977-8".equals(codeString)) 1610 return V3Race._19778; 1611 if ("1978-6".equals(codeString)) 1612 return V3Race._19786; 1613 if ("1979-4".equals(codeString)) 1614 return V3Race._19794; 1615 if ("1980-2".equals(codeString)) 1616 return V3Race._19802; 1617 if ("1981-0".equals(codeString)) 1618 return V3Race._19810; 1619 if ("1982-8".equals(codeString)) 1620 return V3Race._19828; 1621 if ("1984-4".equals(codeString)) 1622 return V3Race._19844; 1623 if ("1985-1".equals(codeString)) 1624 return V3Race._19851; 1625 if ("1986-9".equals(codeString)) 1626 return V3Race._19869; 1627 if ("1987-7".equals(codeString)) 1628 return V3Race._19877; 1629 if ("1988-5".equals(codeString)) 1630 return V3Race._19885; 1631 if ("1990-1".equals(codeString)) 1632 return V3Race._19901; 1633 if ("1992-7".equals(codeString)) 1634 return V3Race._19927; 1635 if ("1993-5".equals(codeString)) 1636 return V3Race._19935; 1637 if ("1994-3".equals(codeString)) 1638 return V3Race._19943; 1639 if ("1995-0".equals(codeString)) 1640 return V3Race._19950; 1641 if ("1996-8".equals(codeString)) 1642 return V3Race._19968; 1643 if ("1997-6".equals(codeString)) 1644 return V3Race._19976; 1645 if ("1998-4".equals(codeString)) 1646 return V3Race._19984; 1647 if ("1999-2".equals(codeString)) 1648 return V3Race._19992; 1649 if ("2000-8".equals(codeString)) 1650 return V3Race._20008; 1651 if ("2002-4".equals(codeString)) 1652 return V3Race._20024; 1653 if ("2004-0".equals(codeString)) 1654 return V3Race._20040; 1655 if ("2006-5".equals(codeString)) 1656 return V3Race._20065; 1657 if ("2007-3".equals(codeString)) 1658 return V3Race._20073; 1659 if ("2008-1".equals(codeString)) 1660 return V3Race._20081; 1661 if ("2009-9".equals(codeString)) 1662 return V3Race._20099; 1663 if ("2010-7".equals(codeString)) 1664 return V3Race._20107; 1665 if ("2011-5".equals(codeString)) 1666 return V3Race._20115; 1667 if ("2012-3".equals(codeString)) 1668 return V3Race._20123; 1669 if ("2013-1".equals(codeString)) 1670 return V3Race._20131; 1671 if ("2014-9".equals(codeString)) 1672 return V3Race._20149; 1673 if ("2015-6".equals(codeString)) 1674 return V3Race._20156; 1675 if ("2016-4".equals(codeString)) 1676 return V3Race._20164; 1677 if ("2017-2".equals(codeString)) 1678 return V3Race._20172; 1679 if ("2018-0".equals(codeString)) 1680 return V3Race._20180; 1681 if ("2019-8".equals(codeString)) 1682 return V3Race._20198; 1683 if ("2020-6".equals(codeString)) 1684 return V3Race._20206; 1685 if ("2021-4".equals(codeString)) 1686 return V3Race._20214; 1687 if ("2022-2".equals(codeString)) 1688 return V3Race._20222; 1689 if ("2023-0".equals(codeString)) 1690 return V3Race._20230; 1691 if ("2024-8".equals(codeString)) 1692 return V3Race._20248; 1693 if ("2025-5".equals(codeString)) 1694 return V3Race._20255; 1695 if ("2026-3".equals(codeString)) 1696 return V3Race._20263; 1697 if ("2028-9".equals(codeString)) 1698 return V3Race._20289; 1699 if ("2029-7".equals(codeString)) 1700 return V3Race._20297; 1701 if ("2030-5".equals(codeString)) 1702 return V3Race._20305; 1703 if ("2031-3".equals(codeString)) 1704 return V3Race._20313; 1705 if ("2032-1".equals(codeString)) 1706 return V3Race._20321; 1707 if ("2033-9".equals(codeString)) 1708 return V3Race._20339; 1709 if ("2034-7".equals(codeString)) 1710 return V3Race._20347; 1711 if ("2035-4".equals(codeString)) 1712 return V3Race._20354; 1713 if ("2036-2".equals(codeString)) 1714 return V3Race._20362; 1715 if ("2037-0".equals(codeString)) 1716 return V3Race._20370; 1717 if ("2038-8".equals(codeString)) 1718 return V3Race._20388; 1719 if ("2039-6".equals(codeString)) 1720 return V3Race._20396; 1721 if ("2040-4".equals(codeString)) 1722 return V3Race._20404; 1723 if ("2041-2".equals(codeString)) 1724 return V3Race._20412; 1725 if ("2042-0".equals(codeString)) 1726 return V3Race._20420; 1727 if ("2043-8".equals(codeString)) 1728 return V3Race._20438; 1729 if ("2044-6".equals(codeString)) 1730 return V3Race._20446; 1731 if ("2045-3".equals(codeString)) 1732 return V3Race._20453; 1733 if ("2046-1".equals(codeString)) 1734 return V3Race._20461; 1735 if ("2047-9".equals(codeString)) 1736 return V3Race._20479; 1737 if ("2048-7".equals(codeString)) 1738 return V3Race._20487; 1739 if ("2049-5".equals(codeString)) 1740 return V3Race._20495; 1741 if ("2050-3".equals(codeString)) 1742 return V3Race._20503; 1743 if ("2051-1".equals(codeString)) 1744 return V3Race._20511; 1745 if ("2052-9".equals(codeString)) 1746 return V3Race._20529; 1747 if ("2054-5".equals(codeString)) 1748 return V3Race._20545; 1749 if ("2056-0".equals(codeString)) 1750 return V3Race._20560; 1751 if ("2058-6".equals(codeString)) 1752 return V3Race._20586; 1753 if ("2060-2".equals(codeString)) 1754 return V3Race._20602; 1755 if ("2061-0".equals(codeString)) 1756 return V3Race._20610; 1757 if ("2062-8".equals(codeString)) 1758 return V3Race._20628; 1759 if ("2063-6".equals(codeString)) 1760 return V3Race._20636; 1761 if ("2064-4".equals(codeString)) 1762 return V3Race._20644; 1763 if ("2065-1".equals(codeString)) 1764 return V3Race._20651; 1765 if ("2066-9".equals(codeString)) 1766 return V3Race._20669; 1767 if ("2067-7".equals(codeString)) 1768 return V3Race._20677; 1769 if ("2068-5".equals(codeString)) 1770 return V3Race._20685; 1771 if ("2069-3".equals(codeString)) 1772 return V3Race._20693; 1773 if ("2070-1".equals(codeString)) 1774 return V3Race._20701; 1775 if ("2071-9".equals(codeString)) 1776 return V3Race._20719; 1777 if ("2072-7".equals(codeString)) 1778 return V3Race._20727; 1779 if ("2073-5".equals(codeString)) 1780 return V3Race._20735; 1781 if ("2074-3".equals(codeString)) 1782 return V3Race._20743; 1783 if ("2075-0".equals(codeString)) 1784 return V3Race._20750; 1785 if ("2076-8".equals(codeString)) 1786 return V3Race._20768; 1787 if ("2078-4".equals(codeString)) 1788 return V3Race._20784; 1789 if ("2079-2".equals(codeString)) 1790 return V3Race._20792; 1791 if ("2080-0".equals(codeString)) 1792 return V3Race._20800; 1793 if ("2081-8".equals(codeString)) 1794 return V3Race._20818; 1795 if ("2082-6".equals(codeString)) 1796 return V3Race._20826; 1797 if ("2083-4".equals(codeString)) 1798 return V3Race._20834; 1799 if ("2085-9".equals(codeString)) 1800 return V3Race._20859; 1801 if ("2086-7".equals(codeString)) 1802 return V3Race._20867; 1803 if ("2087-5".equals(codeString)) 1804 return V3Race._20875; 1805 if ("2088-3".equals(codeString)) 1806 return V3Race._20883; 1807 if ("2089-1".equals(codeString)) 1808 return V3Race._20891; 1809 if ("2090-9".equals(codeString)) 1810 return V3Race._20909; 1811 if ("2091-7".equals(codeString)) 1812 return V3Race._20917; 1813 if ("2092-5".equals(codeString)) 1814 return V3Race._20925; 1815 if ("2093-3".equals(codeString)) 1816 return V3Race._20933; 1817 if ("2094-1".equals(codeString)) 1818 return V3Race._20941; 1819 if ("2095-8".equals(codeString)) 1820 return V3Race._20958; 1821 if ("2096-6".equals(codeString)) 1822 return V3Race._20966; 1823 if ("2097-4".equals(codeString)) 1824 return V3Race._20974; 1825 if ("2098-2".equals(codeString)) 1826 return V3Race._20982; 1827 if ("2100-6".equals(codeString)) 1828 return V3Race._21006; 1829 if ("2101-4".equals(codeString)) 1830 return V3Race._21014; 1831 if ("2102-2".equals(codeString)) 1832 return V3Race._21022; 1833 if ("2103-0".equals(codeString)) 1834 return V3Race._21030; 1835 if ("2104-8".equals(codeString)) 1836 return V3Race._21048; 1837 if ("2500-7".equals(codeString)) 1838 return V3Race._25007; 1839 if ("2106-3".equals(codeString)) 1840 return V3Race._21063; 1841 if ("2108-9".equals(codeString)) 1842 return V3Race._21089; 1843 if ("2109-7".equals(codeString)) 1844 return V3Race._21097; 1845 if ("2110-5".equals(codeString)) 1846 return V3Race._21105; 1847 if ("2111-3".equals(codeString)) 1848 return V3Race._21113; 1849 if ("2112-1".equals(codeString)) 1850 return V3Race._21121; 1851 if ("2113-9".equals(codeString)) 1852 return V3Race._21139; 1853 if ("2114-7".equals(codeString)) 1854 return V3Race._21147; 1855 if ("2115-4".equals(codeString)) 1856 return V3Race._21154; 1857 if ("2116-2".equals(codeString)) 1858 return V3Race._21162; 1859 if ("2118-8".equals(codeString)) 1860 return V3Race._21188; 1861 if ("2119-6".equals(codeString)) 1862 return V3Race._21196; 1863 if ("2120-4".equals(codeString)) 1864 return V3Race._21204; 1865 if ("2121-2".equals(codeString)) 1866 return V3Race._21212; 1867 if ("2122-0".equals(codeString)) 1868 return V3Race._21220; 1869 if ("2123-8".equals(codeString)) 1870 return V3Race._21238; 1871 if ("2124-6".equals(codeString)) 1872 return V3Race._21246; 1873 if ("2125-3".equals(codeString)) 1874 return V3Race._21253; 1875 if ("2126-1".equals(codeString)) 1876 return V3Race._21261; 1877 if ("2127-9".equals(codeString)) 1878 return V3Race._21279; 1879 if ("2129-5".equals(codeString)) 1880 return V3Race._21295; 1881 if ("2131-1".equals(codeString)) 1882 return V3Race._21311; 1883 throw new IllegalArgumentException("Unknown V3Race code '" + codeString + "'"); 1884 } 1885 1886 public String toCode(V3Race code) { 1887 if (code == V3Race.NULL) 1888 return null; 1889 if (code == V3Race._10025) 1890 return "1002-5"; 1891 if (code == V3Race._10041) 1892 return "1004-1"; 1893 if (code == V3Race._10066) 1894 return "1006-6"; 1895 if (code == V3Race._10082) 1896 return "1008-2"; 1897 if (code == V3Race._10108) 1898 return "1010-8"; 1899 if (code == V3Race._10116) 1900 return "1011-6"; 1901 if (code == V3Race._10124) 1902 return "1012-4"; 1903 if (code == V3Race._10132) 1904 return "1013-2"; 1905 if (code == V3Race._10140) 1906 return "1014-0"; 1907 if (code == V3Race._10157) 1908 return "1015-7"; 1909 if (code == V3Race._10165) 1910 return "1016-5"; 1911 if (code == V3Race._10173) 1912 return "1017-3"; 1913 if (code == V3Race._10181) 1914 return "1018-1"; 1915 if (code == V3Race._10199) 1916 return "1019-9"; 1917 if (code == V3Race._10215) 1918 return "1021-5"; 1919 if (code == V3Race._10223) 1920 return "1022-3"; 1921 if (code == V3Race._10231) 1922 return "1023-1"; 1923 if (code == V3Race._10249) 1924 return "1024-9"; 1925 if (code == V3Race._10264) 1926 return "1026-4"; 1927 if (code == V3Race._10280) 1928 return "1028-0"; 1929 if (code == V3Race._10306) 1930 return "1030-6"; 1931 if (code == V3Race._10314) 1932 return "1031-4"; 1933 if (code == V3Race._10330) 1934 return "1033-0"; 1935 if (code == V3Race._10355) 1936 return "1035-5"; 1937 if (code == V3Race._10371) 1938 return "1037-1"; 1939 if (code == V3Race._10397) 1940 return "1039-7"; 1941 if (code == V3Race._10413) 1942 return "1041-3"; 1943 if (code == V3Race._10421) 1944 return "1042-1"; 1945 if (code == V3Race._10447) 1946 return "1044-7"; 1947 if (code == V3Race._10454) 1948 return "1045-4"; 1949 if (code == V3Race._10462) 1950 return "1046-2"; 1951 if (code == V3Race._10470) 1952 return "1047-0"; 1953 if (code == V3Race._10488) 1954 return "1048-8"; 1955 if (code == V3Race._10496) 1956 return "1049-6"; 1957 if (code == V3Race._10504) 1958 return "1050-4"; 1959 if (code == V3Race._10512) 1960 return "1051-2"; 1961 if (code == V3Race._10538) 1962 return "1053-8"; 1963 if (code == V3Race._10546) 1964 return "1054-6"; 1965 if (code == V3Race._10553) 1966 return "1055-3"; 1967 if (code == V3Race._10561) 1968 return "1056-1"; 1969 if (code == V3Race._10579) 1970 return "1057-9"; 1971 if (code == V3Race._10587) 1972 return "1058-7"; 1973 if (code == V3Race._10595) 1974 return "1059-5"; 1975 if (code == V3Race._10603) 1976 return "1060-3"; 1977 if (code == V3Race._10611) 1978 return "1061-1"; 1979 if (code == V3Race._10629) 1980 return "1062-9"; 1981 if (code == V3Race._10637) 1982 return "1063-7"; 1983 if (code == V3Race._10645) 1984 return "1064-5"; 1985 if (code == V3Race._10652) 1986 return "1065-2"; 1987 if (code == V3Race._10660) 1988 return "1066-0"; 1989 if (code == V3Race._10686) 1990 return "1068-6"; 1991 if (code == V3Race._10694) 1992 return "1069-4"; 1993 if (code == V3Race._10702) 1994 return "1070-2"; 1995 if (code == V3Race._10710) 1996 return "1071-0"; 1997 if (code == V3Race._10728) 1998 return "1072-8"; 1999 if (code == V3Race._10736) 2000 return "1073-6"; 2001 if (code == V3Race._10744) 2002 return "1074-4"; 2003 if (code == V3Race._10769) 2004 return "1076-9"; 2005 if (code == V3Race._17418) 2006 return "1741-8"; 2007 if (code == V3Race._17426) 2008 return "1742-6"; 2009 if (code == V3Race._17434) 2010 return "1743-4"; 2011 if (code == V3Race._17442) 2012 return "1744-2"; 2013 if (code == V3Race._17459) 2014 return "1745-9"; 2015 if (code == V3Race._17467) 2016 return "1746-7"; 2017 if (code == V3Race._17475) 2018 return "1747-5"; 2019 if (code == V3Race._17483) 2020 return "1748-3"; 2021 if (code == V3Race._17491) 2022 return "1749-1"; 2023 if (code == V3Race._17509) 2024 return "1750-9"; 2025 if (code == V3Race._17517) 2026 return "1751-7"; 2027 if (code == V3Race._17525) 2028 return "1752-5"; 2029 if (code == V3Race._17533) 2030 return "1753-3"; 2031 if (code == V3Race._17541) 2032 return "1754-1"; 2033 if (code == V3Race._17558) 2034 return "1755-8"; 2035 if (code == V3Race._17566) 2036 return "1756-6"; 2037 if (code == V3Race._17574) 2038 return "1757-4"; 2039 if (code == V3Race._17582) 2040 return "1758-2"; 2041 if (code == V3Race._17590) 2042 return "1759-0"; 2043 if (code == V3Race._17608) 2044 return "1760-8"; 2045 if (code == V3Race._17616) 2046 return "1761-6"; 2047 if (code == V3Race._17624) 2048 return "1762-4"; 2049 if (code == V3Race._17632) 2050 return "1763-2"; 2051 if (code == V3Race._17640) 2052 return "1764-0"; 2053 if (code == V3Race._17657) 2054 return "1765-7"; 2055 if (code == V3Race._17665) 2056 return "1766-5"; 2057 if (code == V3Race._17673) 2058 return "1767-3"; 2059 if (code == V3Race._17681) 2060 return "1768-1"; 2061 if (code == V3Race._17699) 2062 return "1769-9"; 2063 if (code == V3Race._17707) 2064 return "1770-7"; 2065 if (code == V3Race._17715) 2066 return "1771-5"; 2067 if (code == V3Race._17723) 2068 return "1772-3"; 2069 if (code == V3Race._17731) 2070 return "1773-1"; 2071 if (code == V3Race._17749) 2072 return "1774-9"; 2073 if (code == V3Race._17756) 2074 return "1775-6"; 2075 if (code == V3Race._17764) 2076 return "1776-4"; 2077 if (code == V3Race._17772) 2078 return "1777-2"; 2079 if (code == V3Race._17780) 2080 return "1778-0"; 2081 if (code == V3Race._17798) 2082 return "1779-8"; 2083 if (code == V3Race._17806) 2084 return "1780-6"; 2085 if (code == V3Race._17814) 2086 return "1781-4"; 2087 if (code == V3Race._17822) 2088 return "1782-2"; 2089 if (code == V3Race._17830) 2090 return "1783-0"; 2091 if (code == V3Race._17848) 2092 return "1784-8"; 2093 if (code == V3Race._17855) 2094 return "1785-5"; 2095 if (code == V3Race._17863) 2096 return "1786-3"; 2097 if (code == V3Race._17871) 2098 return "1787-1"; 2099 if (code == V3Race._17889) 2100 return "1788-9"; 2101 if (code == V3Race._17897) 2102 return "1789-7"; 2103 if (code == V3Race._17905) 2104 return "1790-5"; 2105 if (code == V3Race._17913) 2106 return "1791-3"; 2107 if (code == V3Race._17921) 2108 return "1792-1"; 2109 if (code == V3Race._17939) 2110 return "1793-9"; 2111 if (code == V3Race._17947) 2112 return "1794-7"; 2113 if (code == V3Race._17954) 2114 return "1795-4"; 2115 if (code == V3Race._17962) 2116 return "1796-2"; 2117 if (code == V3Race._17970) 2118 return "1797-0"; 2119 if (code == V3Race._17988) 2120 return "1798-8"; 2121 if (code == V3Race._17996) 2122 return "1799-6"; 2123 if (code == V3Race._18002) 2124 return "1800-2"; 2125 if (code == V3Race._18010) 2126 return "1801-0"; 2127 if (code == V3Race._18028) 2128 return "1802-8"; 2129 if (code == V3Race._18036) 2130 return "1803-6"; 2131 if (code == V3Race._18044) 2132 return "1804-4"; 2133 if (code == V3Race._18051) 2134 return "1805-1"; 2135 if (code == V3Race._18069) 2136 return "1806-9"; 2137 if (code == V3Race._18077) 2138 return "1807-7"; 2139 if (code == V3Race._18085) 2140 return "1808-5"; 2141 if (code == V3Race._18093) 2142 return "1809-3"; 2143 if (code == V3Race._10785) 2144 return "1078-5"; 2145 if (code == V3Race._10801) 2146 return "1080-1"; 2147 if (code == V3Race._10827) 2148 return "1082-7"; 2149 if (code == V3Race._10835) 2150 return "1083-5"; 2151 if (code == V3Race._10843) 2152 return "1084-3"; 2153 if (code == V3Race._10868) 2154 return "1086-8"; 2155 if (code == V3Race._10884) 2156 return "1088-4"; 2157 if (code == V3Race._10892) 2158 return "1089-2"; 2159 if (code == V3Race._10900) 2160 return "1090-0"; 2161 if (code == V3Race._10918) 2162 return "1091-8"; 2163 if (code == V3Race._10926) 2164 return "1092-6"; 2165 if (code == V3Race._10934) 2166 return "1093-4"; 2167 if (code == V3Race._10942) 2168 return "1094-2"; 2169 if (code == V3Race._10959) 2170 return "1095-9"; 2171 if (code == V3Race._10967) 2172 return "1096-7"; 2173 if (code == V3Race._10975) 2174 return "1097-5"; 2175 if (code == V3Race._10983) 2176 return "1098-3"; 2177 if (code == V3Race._11007) 2178 return "1100-7"; 2179 if (code == V3Race._11023) 2180 return "1102-3"; 2181 if (code == V3Race._11031) 2182 return "1103-1"; 2183 if (code == V3Race._11049) 2184 return "1104-9"; 2185 if (code == V3Race._11064) 2186 return "1106-4"; 2187 if (code == V3Race._11080) 2188 return "1108-0"; 2189 if (code == V3Race._11098) 2190 return "1109-8"; 2191 if (code == V3Race._11106) 2192 return "1110-6"; 2193 if (code == V3Race._11122) 2194 return "1112-2"; 2195 if (code == V3Race._11148) 2196 return "1114-8"; 2197 if (code == V3Race._11155) 2198 return "1115-5"; 2199 if (code == V3Race._11163) 2200 return "1116-3"; 2201 if (code == V3Race._11171) 2202 return "1117-1"; 2203 if (code == V3Race._11189) 2204 return "1118-9"; 2205 if (code == V3Race._11197) 2206 return "1119-7"; 2207 if (code == V3Race._11205) 2208 return "1120-5"; 2209 if (code == V3Race._11213) 2210 return "1121-3"; 2211 if (code == V3Race._11239) 2212 return "1123-9"; 2213 if (code == V3Race._11247) 2214 return "1124-7"; 2215 if (code == V3Race._11254) 2216 return "1125-4"; 2217 if (code == V3Race._11262) 2218 return "1126-2"; 2219 if (code == V3Race._11270) 2220 return "1127-0"; 2221 if (code == V3Race._11288) 2222 return "1128-8"; 2223 if (code == V3Race._11296) 2224 return "1129-6"; 2225 if (code == V3Race._11304) 2226 return "1130-4"; 2227 if (code == V3Race._11312) 2228 return "1131-2"; 2229 if (code == V3Race._11320) 2230 return "1132-0"; 2231 if (code == V3Race._11338) 2232 return "1133-8"; 2233 if (code == V3Race._11346) 2234 return "1134-6"; 2235 if (code == V3Race._11353) 2236 return "1135-3"; 2237 if (code == V3Race._11361) 2238 return "1136-1"; 2239 if (code == V3Race._11379) 2240 return "1137-9"; 2241 if (code == V3Race._11387) 2242 return "1138-7"; 2243 if (code == V3Race._11395) 2244 return "1139-5"; 2245 if (code == V3Race._11403) 2246 return "1140-3"; 2247 if (code == V3Race._11411) 2248 return "1141-1"; 2249 if (code == V3Race._11429) 2250 return "1142-9"; 2251 if (code == V3Race._11437) 2252 return "1143-7"; 2253 if (code == V3Race._11445) 2254 return "1144-5"; 2255 if (code == V3Race._11452) 2256 return "1145-2"; 2257 if (code == V3Race._11460) 2258 return "1146-0"; 2259 if (code == V3Race._11478) 2260 return "1147-8"; 2261 if (code == V3Race._11486) 2262 return "1148-6"; 2263 if (code == V3Race._11502) 2264 return "1150-2"; 2265 if (code == V3Race._11510) 2266 return "1151-0"; 2267 if (code == V3Race._11536) 2268 return "1153-6"; 2269 if (code == V3Race._11551) 2270 return "1155-1"; 2271 if (code == V3Race._11569) 2272 return "1156-9"; 2273 if (code == V3Race._11577) 2274 return "1157-7"; 2275 if (code == V3Race._11585) 2276 return "1158-5"; 2277 if (code == V3Race._11593) 2278 return "1159-3"; 2279 if (code == V3Race._11601) 2280 return "1160-1"; 2281 if (code == V3Race._11627) 2282 return "1162-7"; 2283 if (code == V3Race._11635) 2284 return "1163-5"; 2285 if (code == V3Race._11650) 2286 return "1165-0"; 2287 if (code == V3Race._11676) 2288 return "1167-6"; 2289 if (code == V3Race._11692) 2290 return "1169-2"; 2291 if (code == V3Race._11718) 2292 return "1171-8"; 2293 if (code == V3Race._11734) 2294 return "1173-4"; 2295 if (code == V3Race._11759) 2296 return "1175-9"; 2297 if (code == V3Race._11767) 2298 return "1176-7"; 2299 if (code == V3Race._11783) 2300 return "1178-3"; 2301 if (code == V3Race._11809) 2302 return "1180-9"; 2303 if (code == V3Race._11825) 2304 return "1182-5"; 2305 if (code == V3Race._11841) 2306 return "1184-1"; 2307 if (code == V3Race._11866) 2308 return "1186-6"; 2309 if (code == V3Race._11874) 2310 return "1187-4"; 2311 if (code == V3Race._11890) 2312 return "1189-0"; 2313 if (code == V3Race._11916) 2314 return "1191-6"; 2315 if (code == V3Race._11932) 2316 return "1193-2"; 2317 if (code == V3Race._11940) 2318 return "1194-0"; 2319 if (code == V3Race._11957) 2320 return "1195-7"; 2321 if (code == V3Race._11965) 2322 return "1196-5"; 2323 if (code == V3Race._11973) 2324 return "1197-3"; 2325 if (code == V3Race._11981) 2326 return "1198-1"; 2327 if (code == V3Race._11999) 2328 return "1199-9"; 2329 if (code == V3Race._12005) 2330 return "1200-5"; 2331 if (code == V3Race._12013) 2332 return "1201-3"; 2333 if (code == V3Race._12021) 2334 return "1202-1"; 2335 if (code == V3Race._12039) 2336 return "1203-9"; 2337 if (code == V3Race._12047) 2338 return "1204-7"; 2339 if (code == V3Race._12054) 2340 return "1205-4"; 2341 if (code == V3Race._12070) 2342 return "1207-0"; 2343 if (code == V3Race._12096) 2344 return "1209-6"; 2345 if (code == V3Race._12112) 2346 return "1211-2"; 2347 if (code == V3Race._12120) 2348 return "1212-0"; 2349 if (code == V3Race._12146) 2350 return "1214-6"; 2351 if (code == V3Race._12153) 2352 return "1215-3"; 2353 if (code == V3Race._12161) 2354 return "1216-1"; 2355 if (code == V3Race._12179) 2356 return "1217-9"; 2357 if (code == V3Race._12187) 2358 return "1218-7"; 2359 if (code == V3Race._12195) 2360 return "1219-5"; 2361 if (code == V3Race._12203) 2362 return "1220-3"; 2363 if (code == V3Race._12229) 2364 return "1222-9"; 2365 if (code == V3Race._12237) 2366 return "1223-7"; 2367 if (code == V3Race._12245) 2368 return "1224-5"; 2369 if (code == V3Race._12252) 2370 return "1225-2"; 2371 if (code == V3Race._12260) 2372 return "1226-0"; 2373 if (code == V3Race._12278) 2374 return "1227-8"; 2375 if (code == V3Race._12286) 2376 return "1228-6"; 2377 if (code == V3Race._12294) 2378 return "1229-4"; 2379 if (code == V3Race._12302) 2380 return "1230-2"; 2381 if (code == V3Race._12310) 2382 return "1231-0"; 2383 if (code == V3Race._12336) 2384 return "1233-6"; 2385 if (code == V3Race._12344) 2386 return "1234-4"; 2387 if (code == V3Race._12351) 2388 return "1235-1"; 2389 if (code == V3Race._12369) 2390 return "1236-9"; 2391 if (code == V3Race._12377) 2392 return "1237-7"; 2393 if (code == V3Race._12385) 2394 return "1238-5"; 2395 if (code == V3Race._12393) 2396 return "1239-3"; 2397 if (code == V3Race._12401) 2398 return "1240-1"; 2399 if (code == V3Race._12419) 2400 return "1241-9"; 2401 if (code == V3Race._12427) 2402 return "1242-7"; 2403 if (code == V3Race._12435) 2404 return "1243-5"; 2405 if (code == V3Race._12443) 2406 return "1244-3"; 2407 if (code == V3Race._12450) 2408 return "1245-0"; 2409 if (code == V3Race._12468) 2410 return "1246-8"; 2411 if (code == V3Race._12476) 2412 return "1247-6"; 2413 if (code == V3Race._12484) 2414 return "1248-4"; 2415 if (code == V3Race._12500) 2416 return "1250-0"; 2417 if (code == V3Race._12526) 2418 return "1252-6"; 2419 if (code == V3Race._12542) 2420 return "1254-2"; 2421 if (code == V3Race._12567) 2422 return "1256-7"; 2423 if (code == V3Race._12583) 2424 return "1258-3"; 2425 if (code == V3Race._12609) 2426 return "1260-9"; 2427 if (code == V3Race._12625) 2428 return "1262-5"; 2429 if (code == V3Race._12641) 2430 return "1264-1"; 2431 if (code == V3Race._12658) 2432 return "1265-8"; 2433 if (code == V3Race._12674) 2434 return "1267-4"; 2435 if (code == V3Race._12690) 2436 return "1269-0"; 2437 if (code == V3Race._12716) 2438 return "1271-6"; 2439 if (code == V3Race._12724) 2440 return "1272-4"; 2441 if (code == V3Race._12732) 2442 return "1273-2"; 2443 if (code == V3Race._12757) 2444 return "1275-7"; 2445 if (code == V3Race._12773) 2446 return "1277-3"; 2447 if (code == V3Race._12799) 2448 return "1279-9"; 2449 if (code == V3Race._12815) 2450 return "1281-5"; 2451 if (code == V3Race._12823) 2452 return "1282-3"; 2453 if (code == V3Race._12831) 2454 return "1283-1"; 2455 if (code == V3Race._12856) 2456 return "1285-6"; 2457 if (code == V3Race._12864) 2458 return "1286-4"; 2459 if (code == V3Race._12872) 2460 return "1287-2"; 2461 if (code == V3Race._12880) 2462 return "1288-0"; 2463 if (code == V3Race._12898) 2464 return "1289-8"; 2465 if (code == V3Race._12906) 2466 return "1290-6"; 2467 if (code == V3Race._12914) 2468 return "1291-4"; 2469 if (code == V3Race._12922) 2470 return "1292-2"; 2471 if (code == V3Race._12930) 2472 return "1293-0"; 2473 if (code == V3Race._12948) 2474 return "1294-8"; 2475 if (code == V3Race._12955) 2476 return "1295-5"; 2477 if (code == V3Race._12971) 2478 return "1297-1"; 2479 if (code == V3Race._12997) 2480 return "1299-7"; 2481 if (code == V3Race._13011) 2482 return "1301-1"; 2483 if (code == V3Race._13037) 2484 return "1303-7"; 2485 if (code == V3Race._13052) 2486 return "1305-2"; 2487 if (code == V3Race._13060) 2488 return "1306-0"; 2489 if (code == V3Race._13078) 2490 return "1307-8"; 2491 if (code == V3Race._13094) 2492 return "1309-4"; 2493 if (code == V3Race._13102) 2494 return "1310-2"; 2495 if (code == V3Race._13128) 2496 return "1312-8"; 2497 if (code == V3Race._13136) 2498 return "1313-6"; 2499 if (code == V3Race._13144) 2500 return "1314-4"; 2501 if (code == V3Race._13151) 2502 return "1315-1"; 2503 if (code == V3Race._13177) 2504 return "1317-7"; 2505 if (code == V3Race._13193) 2506 return "1319-3"; 2507 if (code == V3Race._13219) 2508 return "1321-9"; 2509 if (code == V3Race._13235) 2510 return "1323-5"; 2511 if (code == V3Race._13250) 2512 return "1325-0"; 2513 if (code == V3Race._13268) 2514 return "1326-8"; 2515 if (code == V3Race._13276) 2516 return "1327-6"; 2517 if (code == V3Race._13284) 2518 return "1328-4"; 2519 if (code == V3Race._13292) 2520 return "1329-2"; 2521 if (code == V3Race._13318) 2522 return "1331-8"; 2523 if (code == V3Race._13326) 2524 return "1332-6"; 2525 if (code == V3Race._13334) 2526 return "1333-4"; 2527 if (code == V3Race._13342) 2528 return "1334-2"; 2529 if (code == V3Race._13359) 2530 return "1335-9"; 2531 if (code == V3Race._13367) 2532 return "1336-7"; 2533 if (code == V3Race._13375) 2534 return "1337-5"; 2535 if (code == V3Race._13383) 2536 return "1338-3"; 2537 if (code == V3Race._13409) 2538 return "1340-9"; 2539 if (code == V3Race._13425) 2540 return "1342-5"; 2541 if (code == V3Race._13441) 2542 return "1344-1"; 2543 if (code == V3Race._13458) 2544 return "1345-8"; 2545 if (code == V3Race._13466) 2546 return "1346-6"; 2547 if (code == V3Race._13482) 2548 return "1348-2"; 2549 if (code == V3Race._13508) 2550 return "1350-8"; 2551 if (code == V3Race._13524) 2552 return "1352-4"; 2553 if (code == V3Race._13540) 2554 return "1354-0"; 2555 if (code == V3Race._13565) 2556 return "1356-5"; 2557 if (code == V3Race._13581) 2558 return "1358-1"; 2559 if (code == V3Race._13599) 2560 return "1359-9"; 2561 if (code == V3Race._13607) 2562 return "1360-7"; 2563 if (code == V3Race._13615) 2564 return "1361-5"; 2565 if (code == V3Race._13631) 2566 return "1363-1"; 2567 if (code == V3Race._13656) 2568 return "1365-6"; 2569 if (code == V3Race._13664) 2570 return "1366-4"; 2571 if (code == V3Race._13680) 2572 return "1368-0"; 2573 if (code == V3Race._13706) 2574 return "1370-6"; 2575 if (code == V3Race._13722) 2576 return "1372-2"; 2577 if (code == V3Race._13748) 2578 return "1374-8"; 2579 if (code == V3Race._13763) 2580 return "1376-3"; 2581 if (code == V3Race._13789) 2582 return "1378-9"; 2583 if (code == V3Race._13805) 2584 return "1380-5"; 2585 if (code == V3Race._13821) 2586 return "1382-1"; 2587 if (code == V3Race._13839) 2588 return "1383-9"; 2589 if (code == V3Race._13847) 2590 return "1384-7"; 2591 if (code == V3Race._13854) 2592 return "1385-4"; 2593 if (code == V3Race._13870) 2594 return "1387-0"; 2595 if (code == V3Race._13896) 2596 return "1389-6"; 2597 if (code == V3Race._13912) 2598 return "1391-2"; 2599 if (code == V3Race._13920) 2600 return "1392-0"; 2601 if (code == V3Race._13938) 2602 return "1393-8"; 2603 if (code == V3Race._13946) 2604 return "1394-6"; 2605 if (code == V3Race._13953) 2606 return "1395-3"; 2607 if (code == V3Race._13961) 2608 return "1396-1"; 2609 if (code == V3Race._13979) 2610 return "1397-9"; 2611 if (code == V3Race._13987) 2612 return "1398-7"; 2613 if (code == V3Race._13995) 2614 return "1399-5"; 2615 if (code == V3Race._14001) 2616 return "1400-1"; 2617 if (code == V3Race._14019) 2618 return "1401-9"; 2619 if (code == V3Race._14035) 2620 return "1403-5"; 2621 if (code == V3Race._14050) 2622 return "1405-0"; 2623 if (code == V3Race._14076) 2624 return "1407-6"; 2625 if (code == V3Race._14092) 2626 return "1409-2"; 2627 if (code == V3Race._14118) 2628 return "1411-8"; 2629 if (code == V3Race._14126) 2630 return "1412-6"; 2631 if (code == V3Race._14134) 2632 return "1413-4"; 2633 if (code == V3Race._14142) 2634 return "1414-2"; 2635 if (code == V3Race._14167) 2636 return "1416-7"; 2637 if (code == V3Race._14175) 2638 return "1417-5"; 2639 if (code == V3Race._14183) 2640 return "1418-3"; 2641 if (code == V3Race._14191) 2642 return "1419-1"; 2643 if (code == V3Race._14209) 2644 return "1420-9"; 2645 if (code == V3Race._14217) 2646 return "1421-7"; 2647 if (code == V3Race._14225) 2648 return "1422-5"; 2649 if (code == V3Race._14233) 2650 return "1423-3"; 2651 if (code == V3Race._14241) 2652 return "1424-1"; 2653 if (code == V3Race._14258) 2654 return "1425-8"; 2655 if (code == V3Race._14266) 2656 return "1426-6"; 2657 if (code == V3Race._14274) 2658 return "1427-4"; 2659 if (code == V3Race._14282) 2660 return "1428-2"; 2661 if (code == V3Race._14290) 2662 return "1429-0"; 2663 if (code == V3Race._14308) 2664 return "1430-8"; 2665 if (code == V3Race._14316) 2666 return "1431-6"; 2667 if (code == V3Race._14324) 2668 return "1432-4"; 2669 if (code == V3Race._14332) 2670 return "1433-2"; 2671 if (code == V3Race._14340) 2672 return "1434-0"; 2673 if (code == V3Race._14357) 2674 return "1435-7"; 2675 if (code == V3Race._14365) 2676 return "1436-5"; 2677 if (code == V3Race._14373) 2678 return "1437-3"; 2679 if (code == V3Race._14399) 2680 return "1439-9"; 2681 if (code == V3Race._14415) 2682 return "1441-5"; 2683 if (code == V3Race._14423) 2684 return "1442-3"; 2685 if (code == V3Race._14431) 2686 return "1443-1"; 2687 if (code == V3Race._14456) 2688 return "1445-6"; 2689 if (code == V3Race._14464) 2690 return "1446-4"; 2691 if (code == V3Race._14480) 2692 return "1448-0"; 2693 if (code == V3Race._14506) 2694 return "1450-6"; 2695 if (code == V3Race._14514) 2696 return "1451-4"; 2697 if (code == V3Race._14530) 2698 return "1453-0"; 2699 if (code == V3Race._14548) 2700 return "1454-8"; 2701 if (code == V3Race._14563) 2702 return "1456-3"; 2703 if (code == V3Race._14571) 2704 return "1457-1"; 2705 if (code == V3Race._14589) 2706 return "1458-9"; 2707 if (code == V3Race._14605) 2708 return "1460-5"; 2709 if (code == V3Race._14621) 2710 return "1462-1"; 2711 if (code == V3Race._14647) 2712 return "1464-7"; 2713 if (code == V3Race._14654) 2714 return "1465-4"; 2715 if (code == V3Race._14662) 2716 return "1466-2"; 2717 if (code == V3Race._14670) 2718 return "1467-0"; 2719 if (code == V3Race._14688) 2720 return "1468-8"; 2721 if (code == V3Race._14696) 2722 return "1469-6"; 2723 if (code == V3Race._14704) 2724 return "1470-4"; 2725 if (code == V3Race._14712) 2726 return "1471-2"; 2727 if (code == V3Race._14720) 2728 return "1472-0"; 2729 if (code == V3Race._14746) 2730 return "1474-6"; 2731 if (code == V3Race._14753) 2732 return "1475-3"; 2733 if (code == V3Race._14761) 2734 return "1476-1"; 2735 if (code == V3Race._14787) 2736 return "1478-7"; 2737 if (code == V3Race._14795) 2738 return "1479-5"; 2739 if (code == V3Race._14803) 2740 return "1480-3"; 2741 if (code == V3Race._14811) 2742 return "1481-1"; 2743 if (code == V3Race._14829) 2744 return "1482-9"; 2745 if (code == V3Race._14837) 2746 return "1483-7"; 2747 if (code == V3Race._14845) 2748 return "1484-5"; 2749 if (code == V3Race._14852) 2750 return "1485-2"; 2751 if (code == V3Race._14878) 2752 return "1487-8"; 2753 if (code == V3Race._14894) 2754 return "1489-4"; 2755 if (code == V3Race._14902) 2756 return "1490-2"; 2757 if (code == V3Race._14910) 2758 return "1491-0"; 2759 if (code == V3Race._14928) 2760 return "1492-8"; 2761 if (code == V3Race._14936) 2762 return "1493-6"; 2763 if (code == V3Race._14944) 2764 return "1494-4"; 2765 if (code == V3Race._14951) 2766 return "1495-1"; 2767 if (code == V3Race._14969) 2768 return "1496-9"; 2769 if (code == V3Race._14977) 2770 return "1497-7"; 2771 if (code == V3Race._14985) 2772 return "1498-5"; 2773 if (code == V3Race._14993) 2774 return "1499-3"; 2775 if (code == V3Race._15008) 2776 return "1500-8"; 2777 if (code == V3Race._15016) 2778 return "1501-6"; 2779 if (code == V3Race._15024) 2780 return "1502-4"; 2781 if (code == V3Race._15032) 2782 return "1503-2"; 2783 if (code == V3Race._15040) 2784 return "1504-0"; 2785 if (code == V3Race._15057) 2786 return "1505-7"; 2787 if (code == V3Race._15065) 2788 return "1506-5"; 2789 if (code == V3Race._15073) 2790 return "1507-3"; 2791 if (code == V3Race._15081) 2792 return "1508-1"; 2793 if (code == V3Race._15099) 2794 return "1509-9"; 2795 if (code == V3Race._15107) 2796 return "1510-7"; 2797 if (code == V3Race._15115) 2798 return "1511-5"; 2799 if (code == V3Race._15123) 2800 return "1512-3"; 2801 if (code == V3Race._15131) 2802 return "1513-1"; 2803 if (code == V3Race._15149) 2804 return "1514-9"; 2805 if (code == V3Race._15156) 2806 return "1515-6"; 2807 if (code == V3Race._15164) 2808 return "1516-4"; 2809 if (code == V3Race._15180) 2810 return "1518-0"; 2811 if (code == V3Race._15198) 2812 return "1519-8"; 2813 if (code == V3Race._15206) 2814 return "1520-6"; 2815 if (code == V3Race._15214) 2816 return "1521-4"; 2817 if (code == V3Race._15222) 2818 return "1522-2"; 2819 if (code == V3Race._15230) 2820 return "1523-0"; 2821 if (code == V3Race._15248) 2822 return "1524-8"; 2823 if (code == V3Race._15255) 2824 return "1525-5"; 2825 if (code == V3Race._15263) 2826 return "1526-3"; 2827 if (code == V3Race._15271) 2828 return "1527-1"; 2829 if (code == V3Race._15289) 2830 return "1528-9"; 2831 if (code == V3Race._15297) 2832 return "1529-7"; 2833 if (code == V3Race._15305) 2834 return "1530-5"; 2835 if (code == V3Race._15313) 2836 return "1531-3"; 2837 if (code == V3Race._15321) 2838 return "1532-1"; 2839 if (code == V3Race._15339) 2840 return "1533-9"; 2841 if (code == V3Race._15347) 2842 return "1534-7"; 2843 if (code == V3Race._15354) 2844 return "1535-4"; 2845 if (code == V3Race._15362) 2846 return "1536-2"; 2847 if (code == V3Race._15370) 2848 return "1537-0"; 2849 if (code == V3Race._15388) 2850 return "1538-8"; 2851 if (code == V3Race._15396) 2852 return "1539-6"; 2853 if (code == V3Race._15412) 2854 return "1541-2"; 2855 if (code == V3Race._15438) 2856 return "1543-8"; 2857 if (code == V3Race._15453) 2858 return "1545-3"; 2859 if (code == V3Race._15479) 2860 return "1547-9"; 2861 if (code == V3Race._15495) 2862 return "1549-5"; 2863 if (code == V3Race._15511) 2864 return "1551-1"; 2865 if (code == V3Race._15529) 2866 return "1552-9"; 2867 if (code == V3Race._15537) 2868 return "1553-7"; 2869 if (code == V3Race._15545) 2870 return "1554-5"; 2871 if (code == V3Race._15560) 2872 return "1556-0"; 2873 if (code == V3Race._15586) 2874 return "1558-6"; 2875 if (code == V3Race._15602) 2876 return "1560-2"; 2877 if (code == V3Race._15628) 2878 return "1562-8"; 2879 if (code == V3Race._15644) 2880 return "1564-4"; 2881 if (code == V3Race._15669) 2882 return "1566-9"; 2883 if (code == V3Race._15677) 2884 return "1567-7"; 2885 if (code == V3Race._15685) 2886 return "1568-5"; 2887 if (code == V3Race._15693) 2888 return "1569-3"; 2889 if (code == V3Race._15701) 2890 return "1570-1"; 2891 if (code == V3Race._15719) 2892 return "1571-9"; 2893 if (code == V3Race._15735) 2894 return "1573-5"; 2895 if (code == V3Race._15743) 2896 return "1574-3"; 2897 if (code == V3Race._15768) 2898 return "1576-8"; 2899 if (code == V3Race._15784) 2900 return "1578-4"; 2901 if (code == V3Race._15792) 2902 return "1579-2"; 2903 if (code == V3Race._15800) 2904 return "1580-0"; 2905 if (code == V3Race._15826) 2906 return "1582-6"; 2907 if (code == V3Race._15842) 2908 return "1584-2"; 2909 if (code == V3Race._15867) 2910 return "1586-7"; 2911 if (code == V3Race._15875) 2912 return "1587-5"; 2913 if (code == V3Race._15883) 2914 return "1588-3"; 2915 if (code == V3Race._15891) 2916 return "1589-1"; 2917 if (code == V3Race._15909) 2918 return "1590-9"; 2919 if (code == V3Race._15917) 2920 return "1591-7"; 2921 if (code == V3Race._15925) 2922 return "1592-5"; 2923 if (code == V3Race._15933) 2924 return "1593-3"; 2925 if (code == V3Race._15941) 2926 return "1594-1"; 2927 if (code == V3Race._15958) 2928 return "1595-8"; 2929 if (code == V3Race._15966) 2930 return "1596-6"; 2931 if (code == V3Race._15974) 2932 return "1597-4"; 2933 if (code == V3Race._15982) 2934 return "1598-2"; 2935 if (code == V3Race._15990) 2936 return "1599-0"; 2937 if (code == V3Race._16006) 2938 return "1600-6"; 2939 if (code == V3Race._16022) 2940 return "1602-2"; 2941 if (code == V3Race._16030) 2942 return "1603-0"; 2943 if (code == V3Race._16048) 2944 return "1604-8"; 2945 if (code == V3Race._16055) 2946 return "1605-5"; 2947 if (code == V3Race._16071) 2948 return "1607-1"; 2949 if (code == V3Race._16097) 2950 return "1609-7"; 2951 if (code == V3Race._16105) 2952 return "1610-5"; 2953 if (code == V3Race._16113) 2954 return "1611-3"; 2955 if (code == V3Race._16121) 2956 return "1612-1"; 2957 if (code == V3Race._16139) 2958 return "1613-9"; 2959 if (code == V3Race._16147) 2960 return "1614-7"; 2961 if (code == V3Race._16154) 2962 return "1615-4"; 2963 if (code == V3Race._16162) 2964 return "1616-2"; 2965 if (code == V3Race._16170) 2966 return "1617-0"; 2967 if (code == V3Race._16188) 2968 return "1618-8"; 2969 if (code == V3Race._16196) 2970 return "1619-6"; 2971 if (code == V3Race._16204) 2972 return "1620-4"; 2973 if (code == V3Race._16212) 2974 return "1621-2"; 2975 if (code == V3Race._16220) 2976 return "1622-0"; 2977 if (code == V3Race._16238) 2978 return "1623-8"; 2979 if (code == V3Race._16246) 2980 return "1624-6"; 2981 if (code == V3Race._16253) 2982 return "1625-3"; 2983 if (code == V3Race._16261) 2984 return "1626-1"; 2985 if (code == V3Race._16279) 2986 return "1627-9"; 2987 if (code == V3Race._16287) 2988 return "1628-7"; 2989 if (code == V3Race._16295) 2990 return "1629-5"; 2991 if (code == V3Race._16303) 2992 return "1630-3"; 2993 if (code == V3Race._16311) 2994 return "1631-1"; 2995 if (code == V3Race._16329) 2996 return "1632-9"; 2997 if (code == V3Race._16337) 2998 return "1633-7"; 2999 if (code == V3Race._16345) 3000 return "1634-5"; 3001 if (code == V3Race._16352) 3002 return "1635-2"; 3003 if (code == V3Race._16360) 3004 return "1636-0"; 3005 if (code == V3Race._16378) 3006 return "1637-8"; 3007 if (code == V3Race._16386) 3008 return "1638-6"; 3009 if (code == V3Race._16394) 3010 return "1639-4"; 3011 if (code == V3Race._16402) 3012 return "1640-2"; 3013 if (code == V3Race._16410) 3014 return "1641-0"; 3015 if (code == V3Race._16436) 3016 return "1643-6"; 3017 if (code == V3Race._16451) 3018 return "1645-1"; 3019 if (code == V3Race._16477) 3020 return "1647-7"; 3021 if (code == V3Race._16493) 3022 return "1649-3"; 3023 if (code == V3Race._16519) 3024 return "1651-9"; 3025 if (code == V3Race._16535) 3026 return "1653-5"; 3027 if (code == V3Race._16543) 3028 return "1654-3"; 3029 if (code == V3Race._16550) 3030 return "1655-0"; 3031 if (code == V3Race._16568) 3032 return "1656-8"; 3033 if (code == V3Race._16576) 3034 return "1657-6"; 3035 if (code == V3Race._16592) 3036 return "1659-2"; 3037 if (code == V3Race._16618) 3038 return "1661-8"; 3039 if (code == V3Race._16634) 3040 return "1663-4"; 3041 if (code == V3Race._16659) 3042 return "1665-9"; 3043 if (code == V3Race._16675) 3044 return "1667-5"; 3045 if (code == V3Race._16683) 3046 return "1668-3"; 3047 if (code == V3Race._16709) 3048 return "1670-9"; 3049 if (code == V3Race._16717) 3050 return "1671-7"; 3051 if (code == V3Race._16725) 3052 return "1672-5"; 3053 if (code == V3Race._16733) 3054 return "1673-3"; 3055 if (code == V3Race._16758) 3056 return "1675-8"; 3057 if (code == V3Race._16774) 3058 return "1677-4"; 3059 if (code == V3Race._16790) 3060 return "1679-0"; 3061 if (code == V3Race._16808) 3062 return "1680-8"; 3063 if (code == V3Race._16816) 3064 return "1681-6"; 3065 if (code == V3Race._16832) 3066 return "1683-2"; 3067 if (code == V3Race._16857) 3068 return "1685-7"; 3069 if (code == V3Race._16873) 3070 return "1687-3"; 3071 if (code == V3Race._16881) 3072 return "1688-1"; 3073 if (code == V3Race._16899) 3074 return "1689-9"; 3075 if (code == V3Race._16907) 3076 return "1690-7"; 3077 if (code == V3Race._16923) 3078 return "1692-3"; 3079 if (code == V3Race._16949) 3080 return "1694-9"; 3081 if (code == V3Race._16964) 3082 return "1696-4"; 3083 if (code == V3Race._16972) 3084 return "1697-2"; 3085 if (code == V3Race._16980) 3086 return "1698-0"; 3087 if (code == V3Race._17004) 3088 return "1700-4"; 3089 if (code == V3Race._17020) 3090 return "1702-0"; 3091 if (code == V3Race._17046) 3092 return "1704-6"; 3093 if (code == V3Race._17053) 3094 return "1705-3"; 3095 if (code == V3Race._17079) 3096 return "1707-9"; 3097 if (code == V3Race._17095) 3098 return "1709-5"; 3099 if (code == V3Race._17111) 3100 return "1711-1"; 3101 if (code == V3Race._17129) 3102 return "1712-9"; 3103 if (code == V3Race._17137) 3104 return "1713-7"; 3105 if (code == V3Race._17152) 3106 return "1715-2"; 3107 if (code == V3Race._17178) 3108 return "1717-8"; 3109 if (code == V3Race._17186) 3110 return "1718-6"; 3111 if (code == V3Race._17194) 3112 return "1719-4"; 3113 if (code == V3Race._17202) 3114 return "1720-2"; 3115 if (code == V3Race._17228) 3116 return "1722-8"; 3117 if (code == V3Race._17244) 3118 return "1724-4"; 3119 if (code == V3Race._17251) 3120 return "1725-1"; 3121 if (code == V3Race._17269) 3122 return "1726-9"; 3123 if (code == V3Race._17277) 3124 return "1727-7"; 3125 if (code == V3Race._17285) 3126 return "1728-5"; 3127 if (code == V3Race._17293) 3128 return "1729-3"; 3129 if (code == V3Race._17301) 3130 return "1730-1"; 3131 if (code == V3Race._17319) 3132 return "1731-9"; 3133 if (code == V3Race._17327) 3134 return "1732-7"; 3135 if (code == V3Race._17335) 3136 return "1733-5"; 3137 if (code == V3Race._17350) 3138 return "1735-0"; 3139 if (code == V3Race._17376) 3140 return "1737-6"; 3141 if (code == V3Race._17392) 3142 return "1739-2"; 3143 if (code == V3Race._17400) 3144 return "1740-0"; 3145 if (code == V3Race._18119) 3146 return "1811-9"; 3147 if (code == V3Race._18135) 3148 return "1813-5"; 3149 if (code == V3Race._18143) 3150 return "1814-3"; 3151 if (code == V3Race._18150) 3152 return "1815-0"; 3153 if (code == V3Race._18168) 3154 return "1816-8"; 3155 if (code == V3Race._18176) 3156 return "1817-6"; 3157 if (code == V3Race._18184) 3158 return "1818-4"; 3159 if (code == V3Race._18192) 3160 return "1819-2"; 3161 if (code == V3Race._18200) 3162 return "1820-0"; 3163 if (code == V3Race._18218) 3164 return "1821-8"; 3165 if (code == V3Race._18226) 3166 return "1822-6"; 3167 if (code == V3Race._18234) 3168 return "1823-4"; 3169 if (code == V3Race._18242) 3170 return "1824-2"; 3171 if (code == V3Race._18259) 3172 return "1825-9"; 3173 if (code == V3Race._18267) 3174 return "1826-7"; 3175 if (code == V3Race._18275) 3176 return "1827-5"; 3177 if (code == V3Race._18283) 3178 return "1828-3"; 3179 if (code == V3Race._18291) 3180 return "1829-1"; 3181 if (code == V3Race._18309) 3182 return "1830-9"; 3183 if (code == V3Race._18317) 3184 return "1831-7"; 3185 if (code == V3Race._18325) 3186 return "1832-5"; 3187 if (code == V3Race._18333) 3188 return "1833-3"; 3189 if (code == V3Race._18341) 3190 return "1834-1"; 3191 if (code == V3Race._18358) 3192 return "1835-8"; 3193 if (code == V3Race._18374) 3194 return "1837-4"; 3195 if (code == V3Race._18382) 3196 return "1838-2"; 3197 if (code == V3Race._18408) 3198 return "1840-8"; 3199 if (code == V3Race._18424) 3200 return "1842-4"; 3201 if (code == V3Race._18440) 3202 return "1844-0"; 3203 if (code == V3Race._18457) 3204 return "1845-7"; 3205 if (code == V3Race._18465) 3206 return "1846-5"; 3207 if (code == V3Race._18473) 3208 return "1847-3"; 3209 if (code == V3Race._18481) 3210 return "1848-1"; 3211 if (code == V3Race._18499) 3212 return "1849-9"; 3213 if (code == V3Race._18507) 3214 return "1850-7"; 3215 if (code == V3Race._18515) 3216 return "1851-5"; 3217 if (code == V3Race._18523) 3218 return "1852-3"; 3219 if (code == V3Race._18531) 3220 return "1853-1"; 3221 if (code == V3Race._18549) 3222 return "1854-9"; 3223 if (code == V3Race._18556) 3224 return "1855-6"; 3225 if (code == V3Race._18564) 3226 return "1856-4"; 3227 if (code == V3Race._18572) 3228 return "1857-2"; 3229 if (code == V3Race._18580) 3230 return "1858-0"; 3231 if (code == V3Race._18598) 3232 return "1859-8"; 3233 if (code == V3Race._18606) 3234 return "1860-6"; 3235 if (code == V3Race._18614) 3236 return "1861-4"; 3237 if (code == V3Race._18622) 3238 return "1862-2"; 3239 if (code == V3Race._18630) 3240 return "1863-0"; 3241 if (code == V3Race._18648) 3242 return "1864-8"; 3243 if (code == V3Race._18655) 3244 return "1865-5"; 3245 if (code == V3Race._18663) 3246 return "1866-3"; 3247 if (code == V3Race._18671) 3248 return "1867-1"; 3249 if (code == V3Race._18689) 3250 return "1868-9"; 3251 if (code == V3Race._18697) 3252 return "1869-7"; 3253 if (code == V3Race._18705) 3254 return "1870-5"; 3255 if (code == V3Race._18713) 3256 return "1871-3"; 3257 if (code == V3Race._18721) 3258 return "1872-1"; 3259 if (code == V3Race._18739) 3260 return "1873-9"; 3261 if (code == V3Race._18747) 3262 return "1874-7"; 3263 if (code == V3Race._18754) 3264 return "1875-4"; 3265 if (code == V3Race._18762) 3266 return "1876-2"; 3267 if (code == V3Race._18770) 3268 return "1877-0"; 3269 if (code == V3Race._18788) 3270 return "1878-8"; 3271 if (code == V3Race._18796) 3272 return "1879-6"; 3273 if (code == V3Race._18804) 3274 return "1880-4"; 3275 if (code == V3Race._18812) 3276 return "1881-2"; 3277 if (code == V3Race._18820) 3278 return "1882-0"; 3279 if (code == V3Race._18838) 3280 return "1883-8"; 3281 if (code == V3Race._18846) 3282 return "1884-6"; 3283 if (code == V3Race._18853) 3284 return "1885-3"; 3285 if (code == V3Race._18861) 3286 return "1886-1"; 3287 if (code == V3Race._18879) 3288 return "1887-9"; 3289 if (code == V3Race._18887) 3290 return "1888-7"; 3291 if (code == V3Race._18895) 3292 return "1889-5"; 3293 if (code == V3Race._18911) 3294 return "1891-1"; 3295 if (code == V3Race._18929) 3296 return "1892-9"; 3297 if (code == V3Race._18937) 3298 return "1893-7"; 3299 if (code == V3Race._18945) 3300 return "1894-5"; 3301 if (code == V3Race._18960) 3302 return "1896-0"; 3303 if (code == V3Race._18978) 3304 return "1897-8"; 3305 if (code == V3Race._18986) 3306 return "1898-6"; 3307 if (code == V3Race._18994) 3308 return "1899-4"; 3309 if (code == V3Race._19000) 3310 return "1900-0"; 3311 if (code == V3Race._19018) 3312 return "1901-8"; 3313 if (code == V3Race._19026) 3314 return "1902-6"; 3315 if (code == V3Race._19034) 3316 return "1903-4"; 3317 if (code == V3Race._19042) 3318 return "1904-2"; 3319 if (code == V3Race._19059) 3320 return "1905-9"; 3321 if (code == V3Race._19067) 3322 return "1906-7"; 3323 if (code == V3Race._19075) 3324 return "1907-5"; 3325 if (code == V3Race._19083) 3326 return "1908-3"; 3327 if (code == V3Race._19091) 3328 return "1909-1"; 3329 if (code == V3Race._19109) 3330 return "1910-9"; 3331 if (code == V3Race._19117) 3332 return "1911-7"; 3333 if (code == V3Race._19125) 3334 return "1912-5"; 3335 if (code == V3Race._19133) 3336 return "1913-3"; 3337 if (code == V3Race._19141) 3338 return "1914-1"; 3339 if (code == V3Race._19158) 3340 return "1915-8"; 3341 if (code == V3Race._19166) 3342 return "1916-6"; 3343 if (code == V3Race._19174) 3344 return "1917-4"; 3345 if (code == V3Race._19182) 3346 return "1918-2"; 3347 if (code == V3Race._19190) 3348 return "1919-0"; 3349 if (code == V3Race._19208) 3350 return "1920-8"; 3351 if (code == V3Race._19216) 3352 return "1921-6"; 3353 if (code == V3Race._19224) 3354 return "1922-4"; 3355 if (code == V3Race._19232) 3356 return "1923-2"; 3357 if (code == V3Race._19240) 3358 return "1924-0"; 3359 if (code == V3Race._19257) 3360 return "1925-7"; 3361 if (code == V3Race._19265) 3362 return "1926-5"; 3363 if (code == V3Race._19273) 3364 return "1927-3"; 3365 if (code == V3Race._19281) 3366 return "1928-1"; 3367 if (code == V3Race._19299) 3368 return "1929-9"; 3369 if (code == V3Race._19307) 3370 return "1930-7"; 3371 if (code == V3Race._19315) 3372 return "1931-5"; 3373 if (code == V3Race._19323) 3374 return "1932-3"; 3375 if (code == V3Race._19331) 3376 return "1933-1"; 3377 if (code == V3Race._19349) 3378 return "1934-9"; 3379 if (code == V3Race._19356) 3380 return "1935-6"; 3381 if (code == V3Race._19364) 3382 return "1936-4"; 3383 if (code == V3Race._19372) 3384 return "1937-2"; 3385 if (code == V3Race._19380) 3386 return "1938-0"; 3387 if (code == V3Race._19398) 3388 return "1939-8"; 3389 if (code == V3Race._19406) 3390 return "1940-6"; 3391 if (code == V3Race._19414) 3392 return "1941-4"; 3393 if (code == V3Race._19422) 3394 return "1942-2"; 3395 if (code == V3Race._19430) 3396 return "1943-0"; 3397 if (code == V3Race._19448) 3398 return "1944-8"; 3399 if (code == V3Race._19455) 3400 return "1945-5"; 3401 if (code == V3Race._19463) 3402 return "1946-3"; 3403 if (code == V3Race._19471) 3404 return "1947-1"; 3405 if (code == V3Race._19489) 3406 return "1948-9"; 3407 if (code == V3Race._19497) 3408 return "1949-7"; 3409 if (code == V3Race._19505) 3410 return "1950-5"; 3411 if (code == V3Race._19513) 3412 return "1951-3"; 3413 if (code == V3Race._19521) 3414 return "1952-1"; 3415 if (code == V3Race._19539) 3416 return "1953-9"; 3417 if (code == V3Race._19547) 3418 return "1954-7"; 3419 if (code == V3Race._19554) 3420 return "1955-4"; 3421 if (code == V3Race._19562) 3422 return "1956-2"; 3423 if (code == V3Race._19570) 3424 return "1957-0"; 3425 if (code == V3Race._19588) 3426 return "1958-8"; 3427 if (code == V3Race._19596) 3428 return "1959-6"; 3429 if (code == V3Race._19604) 3430 return "1960-4"; 3431 if (code == V3Race._19612) 3432 return "1961-2"; 3433 if (code == V3Race._19620) 3434 return "1962-0"; 3435 if (code == V3Race._19638) 3436 return "1963-8"; 3437 if (code == V3Race._19646) 3438 return "1964-6"; 3439 if (code == V3Race._19661) 3440 return "1966-1"; 3441 if (code == V3Race._19687) 3442 return "1968-7"; 3443 if (code == V3Race._19695) 3444 return "1969-5"; 3445 if (code == V3Race._19703) 3446 return "1970-3"; 3447 if (code == V3Race._19729) 3448 return "1972-9"; 3449 if (code == V3Race._19737) 3450 return "1973-7"; 3451 if (code == V3Race._19745) 3452 return "1974-5"; 3453 if (code == V3Race._19752) 3454 return "1975-2"; 3455 if (code == V3Race._19760) 3456 return "1976-0"; 3457 if (code == V3Race._19778) 3458 return "1977-8"; 3459 if (code == V3Race._19786) 3460 return "1978-6"; 3461 if (code == V3Race._19794) 3462 return "1979-4"; 3463 if (code == V3Race._19802) 3464 return "1980-2"; 3465 if (code == V3Race._19810) 3466 return "1981-0"; 3467 if (code == V3Race._19828) 3468 return "1982-8"; 3469 if (code == V3Race._19844) 3470 return "1984-4"; 3471 if (code == V3Race._19851) 3472 return "1985-1"; 3473 if (code == V3Race._19869) 3474 return "1986-9"; 3475 if (code == V3Race._19877) 3476 return "1987-7"; 3477 if (code == V3Race._19885) 3478 return "1988-5"; 3479 if (code == V3Race._19901) 3480 return "1990-1"; 3481 if (code == V3Race._19927) 3482 return "1992-7"; 3483 if (code == V3Race._19935) 3484 return "1993-5"; 3485 if (code == V3Race._19943) 3486 return "1994-3"; 3487 if (code == V3Race._19950) 3488 return "1995-0"; 3489 if (code == V3Race._19968) 3490 return "1996-8"; 3491 if (code == V3Race._19976) 3492 return "1997-6"; 3493 if (code == V3Race._19984) 3494 return "1998-4"; 3495 if (code == V3Race._19992) 3496 return "1999-2"; 3497 if (code == V3Race._20008) 3498 return "2000-8"; 3499 if (code == V3Race._20024) 3500 return "2002-4"; 3501 if (code == V3Race._20040) 3502 return "2004-0"; 3503 if (code == V3Race._20065) 3504 return "2006-5"; 3505 if (code == V3Race._20073) 3506 return "2007-3"; 3507 if (code == V3Race._20081) 3508 return "2008-1"; 3509 if (code == V3Race._20099) 3510 return "2009-9"; 3511 if (code == V3Race._20107) 3512 return "2010-7"; 3513 if (code == V3Race._20115) 3514 return "2011-5"; 3515 if (code == V3Race._20123) 3516 return "2012-3"; 3517 if (code == V3Race._20131) 3518 return "2013-1"; 3519 if (code == V3Race._20149) 3520 return "2014-9"; 3521 if (code == V3Race._20156) 3522 return "2015-6"; 3523 if (code == V3Race._20164) 3524 return "2016-4"; 3525 if (code == V3Race._20172) 3526 return "2017-2"; 3527 if (code == V3Race._20180) 3528 return "2018-0"; 3529 if (code == V3Race._20198) 3530 return "2019-8"; 3531 if (code == V3Race._20206) 3532 return "2020-6"; 3533 if (code == V3Race._20214) 3534 return "2021-4"; 3535 if (code == V3Race._20222) 3536 return "2022-2"; 3537 if (code == V3Race._20230) 3538 return "2023-0"; 3539 if (code == V3Race._20248) 3540 return "2024-8"; 3541 if (code == V3Race._20255) 3542 return "2025-5"; 3543 if (code == V3Race._20263) 3544 return "2026-3"; 3545 if (code == V3Race._20289) 3546 return "2028-9"; 3547 if (code == V3Race._20297) 3548 return "2029-7"; 3549 if (code == V3Race._20305) 3550 return "2030-5"; 3551 if (code == V3Race._20313) 3552 return "2031-3"; 3553 if (code == V3Race._20321) 3554 return "2032-1"; 3555 if (code == V3Race._20339) 3556 return "2033-9"; 3557 if (code == V3Race._20347) 3558 return "2034-7"; 3559 if (code == V3Race._20354) 3560 return "2035-4"; 3561 if (code == V3Race._20362) 3562 return "2036-2"; 3563 if (code == V3Race._20370) 3564 return "2037-0"; 3565 if (code == V3Race._20388) 3566 return "2038-8"; 3567 if (code == V3Race._20396) 3568 return "2039-6"; 3569 if (code == V3Race._20404) 3570 return "2040-4"; 3571 if (code == V3Race._20412) 3572 return "2041-2"; 3573 if (code == V3Race._20420) 3574 return "2042-0"; 3575 if (code == V3Race._20438) 3576 return "2043-8"; 3577 if (code == V3Race._20446) 3578 return "2044-6"; 3579 if (code == V3Race._20453) 3580 return "2045-3"; 3581 if (code == V3Race._20461) 3582 return "2046-1"; 3583 if (code == V3Race._20479) 3584 return "2047-9"; 3585 if (code == V3Race._20487) 3586 return "2048-7"; 3587 if (code == V3Race._20495) 3588 return "2049-5"; 3589 if (code == V3Race._20503) 3590 return "2050-3"; 3591 if (code == V3Race._20511) 3592 return "2051-1"; 3593 if (code == V3Race._20529) 3594 return "2052-9"; 3595 if (code == V3Race._20545) 3596 return "2054-5"; 3597 if (code == V3Race._20560) 3598 return "2056-0"; 3599 if (code == V3Race._20586) 3600 return "2058-6"; 3601 if (code == V3Race._20602) 3602 return "2060-2"; 3603 if (code == V3Race._20610) 3604 return "2061-0"; 3605 if (code == V3Race._20628) 3606 return "2062-8"; 3607 if (code == V3Race._20636) 3608 return "2063-6"; 3609 if (code == V3Race._20644) 3610 return "2064-4"; 3611 if (code == V3Race._20651) 3612 return "2065-1"; 3613 if (code == V3Race._20669) 3614 return "2066-9"; 3615 if (code == V3Race._20677) 3616 return "2067-7"; 3617 if (code == V3Race._20685) 3618 return "2068-5"; 3619 if (code == V3Race._20693) 3620 return "2069-3"; 3621 if (code == V3Race._20701) 3622 return "2070-1"; 3623 if (code == V3Race._20719) 3624 return "2071-9"; 3625 if (code == V3Race._20727) 3626 return "2072-7"; 3627 if (code == V3Race._20735) 3628 return "2073-5"; 3629 if (code == V3Race._20743) 3630 return "2074-3"; 3631 if (code == V3Race._20750) 3632 return "2075-0"; 3633 if (code == V3Race._20768) 3634 return "2076-8"; 3635 if (code == V3Race._20784) 3636 return "2078-4"; 3637 if (code == V3Race._20792) 3638 return "2079-2"; 3639 if (code == V3Race._20800) 3640 return "2080-0"; 3641 if (code == V3Race._20818) 3642 return "2081-8"; 3643 if (code == V3Race._20826) 3644 return "2082-6"; 3645 if (code == V3Race._20834) 3646 return "2083-4"; 3647 if (code == V3Race._20859) 3648 return "2085-9"; 3649 if (code == V3Race._20867) 3650 return "2086-7"; 3651 if (code == V3Race._20875) 3652 return "2087-5"; 3653 if (code == V3Race._20883) 3654 return "2088-3"; 3655 if (code == V3Race._20891) 3656 return "2089-1"; 3657 if (code == V3Race._20909) 3658 return "2090-9"; 3659 if (code == V3Race._20917) 3660 return "2091-7"; 3661 if (code == V3Race._20925) 3662 return "2092-5"; 3663 if (code == V3Race._20933) 3664 return "2093-3"; 3665 if (code == V3Race._20941) 3666 return "2094-1"; 3667 if (code == V3Race._20958) 3668 return "2095-8"; 3669 if (code == V3Race._20966) 3670 return "2096-6"; 3671 if (code == V3Race._20974) 3672 return "2097-4"; 3673 if (code == V3Race._20982) 3674 return "2098-2"; 3675 if (code == V3Race._21006) 3676 return "2100-6"; 3677 if (code == V3Race._21014) 3678 return "2101-4"; 3679 if (code == V3Race._21022) 3680 return "2102-2"; 3681 if (code == V3Race._21030) 3682 return "2103-0"; 3683 if (code == V3Race._21048) 3684 return "2104-8"; 3685 if (code == V3Race._25007) 3686 return "2500-7"; 3687 if (code == V3Race._21063) 3688 return "2106-3"; 3689 if (code == V3Race._21089) 3690 return "2108-9"; 3691 if (code == V3Race._21097) 3692 return "2109-7"; 3693 if (code == V3Race._21105) 3694 return "2110-5"; 3695 if (code == V3Race._21113) 3696 return "2111-3"; 3697 if (code == V3Race._21121) 3698 return "2112-1"; 3699 if (code == V3Race._21139) 3700 return "2113-9"; 3701 if (code == V3Race._21147) 3702 return "2114-7"; 3703 if (code == V3Race._21154) 3704 return "2115-4"; 3705 if (code == V3Race._21162) 3706 return "2116-2"; 3707 if (code == V3Race._21188) 3708 return "2118-8"; 3709 if (code == V3Race._21196) 3710 return "2119-6"; 3711 if (code == V3Race._21204) 3712 return "2120-4"; 3713 if (code == V3Race._21212) 3714 return "2121-2"; 3715 if (code == V3Race._21220) 3716 return "2122-0"; 3717 if (code == V3Race._21238) 3718 return "2123-8"; 3719 if (code == V3Race._21246) 3720 return "2124-6"; 3721 if (code == V3Race._21253) 3722 return "2125-3"; 3723 if (code == V3Race._21261) 3724 return "2126-1"; 3725 if (code == V3Race._21279) 3726 return "2127-9"; 3727 if (code == V3Race._21295) 3728 return "2129-5"; 3729 if (code == V3Race._21311) 3730 return "2131-1"; 3731 return "?"; 3732 } 3733 3734 public String toSystem(V3Race code) { 3735 return code.getSystem(); 3736 } 3737 3738}