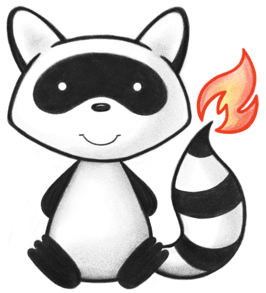
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum V3RoleLinkStatus { 037 038 /** 039 * Description: The 'typical' state. Excludes "nullified" which represents the 040 * termination state of a RoleLink instance that was created in error. 041 */ 042 NORMAL, 043 /** 044 * Description: The state indicates the RoleLink is in progress. 045 */ 046 ACTIVE, 047 /** 048 * Description: The terminal state resulting from abandoning the RoleLink prior 049 * to or after activation. 050 */ 051 CANCELLED, 052 /** 053 * Description: The terminal state representing the successful completion of the 054 * RoleLink. 055 */ 056 COMPLETED, 057 /** 058 * Description: The state indicates the RoleLink has not yet become active. 059 */ 060 PENDING, 061 /** 062 * Description: The state representing the termination of a RoleLink instance 063 * that was created in error. 064 */ 065 NULLIFIED, 066 /** 067 * added to help the parsers 068 */ 069 NULL; 070 071 public static V3RoleLinkStatus fromCode(String codeString) throws FHIRException { 072 if (codeString == null || "".equals(codeString)) 073 return null; 074 if ("NORMAL".equals(codeString)) 075 return NORMAL; 076 if ("ACTIVE".equals(codeString)) 077 return ACTIVE; 078 if ("CANCELLED".equals(codeString)) 079 return CANCELLED; 080 if ("COMPLETED".equals(codeString)) 081 return COMPLETED; 082 if ("PENDING".equals(codeString)) 083 return PENDING; 084 if ("NULLIFIED".equals(codeString)) 085 return NULLIFIED; 086 throw new FHIRException("Unknown V3RoleLinkStatus code '" + codeString + "'"); 087 } 088 089 public String toCode() { 090 switch (this) { 091 case NORMAL: 092 return "NORMAL"; 093 case ACTIVE: 094 return "ACTIVE"; 095 case CANCELLED: 096 return "CANCELLED"; 097 case COMPLETED: 098 return "COMPLETED"; 099 case PENDING: 100 return "PENDING"; 101 case NULLIFIED: 102 return "NULLIFIED"; 103 case NULL: 104 return null; 105 default: 106 return "?"; 107 } 108 } 109 110 public String getSystem() { 111 return "http://terminology.hl7.org/CodeSystem/v3-RoleLinkStatus"; 112 } 113 114 public String getDefinition() { 115 switch (this) { 116 case NORMAL: 117 return "Description: The 'typical' state. Excludes \"nullified\" which represents the termination state of a RoleLink instance that was created in error."; 118 case ACTIVE: 119 return "Description: The state indicates the RoleLink is in progress."; 120 case CANCELLED: 121 return "Description: The terminal state resulting from abandoning the RoleLink prior to or after activation."; 122 case COMPLETED: 123 return "Description: The terminal state representing the successful completion of the RoleLink."; 124 case PENDING: 125 return "Description: The state indicates the RoleLink has not yet become active."; 126 case NULLIFIED: 127 return "Description: The state representing the termination of a RoleLink instance that was created in error."; 128 case NULL: 129 return null; 130 default: 131 return "?"; 132 } 133 } 134 135 public String getDisplay() { 136 switch (this) { 137 case NORMAL: 138 return "normal"; 139 case ACTIVE: 140 return "active"; 141 case CANCELLED: 142 return "cancelled"; 143 case COMPLETED: 144 return "completed"; 145 case PENDING: 146 return "pending"; 147 case NULLIFIED: 148 return "nullified"; 149 case NULL: 150 return null; 151 default: 152 return "?"; 153 } 154 } 155 156}