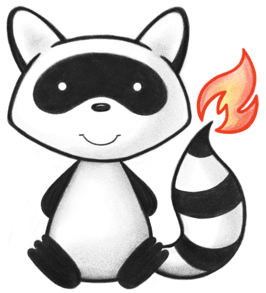
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum V3VaccineManufacturer { 037 038 /** 039 * Abbott Laboratories (includes Ross Products Division) 040 */ 041 AB, 042 /** 043 * Adams Laboratories 044 */ 045 AD, 046 /** 047 * Alpha Therapeutic Corporation 048 */ 049 ALP, 050 /** 051 * Armour [Inactive-use CEN] 052 */ 053 AR, 054 /** 055 * Aviron 056 */ 057 AVI, 058 /** 059 * Baxter Healthcare Corporation 060 */ 061 BA, 062 /** 063 * Bayer Corporation (includes Miles, Inc. and Cutter Laboratories) 064 */ 065 BAY, 066 /** 067 * Berna Products [Inactive-use BPC] 068 */ 069 BP, 070 /** 071 * Berna Products Corporation (includes Swiss Serum and Vaccine Institute Berne) 072 */ 073 BPC, 074 /** 075 * Centeon L.L.C. (includes Armour Pharmaceutical Company) 076 */ 077 CEN, 078 /** 079 * Chiron Corporation 080 */ 081 CHI, 082 /** 083 * Connaught [Inactive-use PMC] 084 */ 085 CON, 086 /** 087 * Evans Medical Limited (an affiliate of Medeva Pharmaceuticals, Inc.) 088 */ 089 EVN, 090 /** 091 * Greer Laboratories, Inc. 092 */ 093 GRE, 094 /** 095 * Immuno International AG 096 */ 097 IAG, 098 /** 099 * Merieux [Inactive-use PMC] 100 */ 101 IM, 102 /** 103 * Immuno-U.S., Inc. 104 */ 105 IUS, 106 /** 107 * The Research Foundation for Microbial Diseases of Osaka University (BIKEN) 108 */ 109 JPN, 110 /** 111 * Korea Green Cross Corporation 112 */ 113 KGC, 114 /** 115 * Lederle [Inactive-use WAL] 116 */ 117 LED, 118 /** 119 * Massachusetts Public Health Biologic Laboratories 120 */ 121 MA, 122 /** 123 * MedImmune, Inc. 124 */ 125 MED, 126 /** 127 * Miles [Inactive-use BAY] 128 */ 129 MIL, 130 /** 131 * Bioport Corporation (formerly Michigan Biologic Products Institute) 132 */ 133 MIP, 134 /** 135 * Merck & Co., Inc. 136 */ 137 MSD, 138 /** 139 * NABI (formerly North American Biologicals, Inc.) 140 */ 141 NAB, 142 /** 143 * North American Vaccine, Inc. 144 */ 145 NAV, 146 /** 147 * Novartis Pharmaceutical Corporation (includes Ciba-Geigy Limited and Sandoz 148 * Limited) 149 */ 150 NOV, 151 /** 152 * New York Blood Center 153 */ 154 NYB, 155 /** 156 * Ortho Diagnostic Systems, Inc. 157 */ 158 ORT, 159 /** 160 * Organon Teknika Corporation 161 */ 162 OTC, 163 /** 164 * Parkedale Pharmaceuticals (formerly Parke-Davis) 165 */ 166 PD, 167 /** 168 * Aventis Pasteur Inc. (formerly Pasteur Merieux Connaught; includes Connaught 169 * Laboratories and Pasteur Merieux) 170 */ 171 PMC, 172 /** 173 * Praxis Biologics [Inactive-use WAL] 174 */ 175 PRX, 176 /** 177 * Sclavo, Inc. 178 */ 179 SCL, 180 /** 181 * Swiss Serum and Vaccine Inst. [Inactive-use BPC] 182 */ 183 SI, 184 /** 185 * SmithKline Beecham 186 */ 187 SKB, 188 /** 189 * United States Army Medical Research and Materiel Command 190 */ 191 USA, 192 /** 193 * Wyeth-Ayerst [Inactive-use WAL] 194 */ 195 WA, 196 /** 197 * Wyeth-Ayerst (includes Wyeth-Lederle Vaccines and Pediatrics, Wyeth 198 * Laboratories, Lederle Laboratories, and Praxis Biologics) 199 */ 200 WAL, 201 /** 202 * added to help the parsers 203 */ 204 NULL; 205 206 public static V3VaccineManufacturer fromCode(String codeString) throws FHIRException { 207 if (codeString == null || "".equals(codeString)) 208 return null; 209 if ("AB".equals(codeString)) 210 return AB; 211 if ("AD".equals(codeString)) 212 return AD; 213 if ("ALP".equals(codeString)) 214 return ALP; 215 if ("AR".equals(codeString)) 216 return AR; 217 if ("AVI".equals(codeString)) 218 return AVI; 219 if ("BA".equals(codeString)) 220 return BA; 221 if ("BAY".equals(codeString)) 222 return BAY; 223 if ("BP".equals(codeString)) 224 return BP; 225 if ("BPC".equals(codeString)) 226 return BPC; 227 if ("CEN".equals(codeString)) 228 return CEN; 229 if ("CHI".equals(codeString)) 230 return CHI; 231 if ("CON".equals(codeString)) 232 return CON; 233 if ("EVN".equals(codeString)) 234 return EVN; 235 if ("GRE".equals(codeString)) 236 return GRE; 237 if ("IAG".equals(codeString)) 238 return IAG; 239 if ("IM".equals(codeString)) 240 return IM; 241 if ("IUS".equals(codeString)) 242 return IUS; 243 if ("JPN".equals(codeString)) 244 return JPN; 245 if ("KGC".equals(codeString)) 246 return KGC; 247 if ("LED".equals(codeString)) 248 return LED; 249 if ("MA".equals(codeString)) 250 return MA; 251 if ("MED".equals(codeString)) 252 return MED; 253 if ("MIL".equals(codeString)) 254 return MIL; 255 if ("MIP".equals(codeString)) 256 return MIP; 257 if ("MSD".equals(codeString)) 258 return MSD; 259 if ("NAB".equals(codeString)) 260 return NAB; 261 if ("NAV".equals(codeString)) 262 return NAV; 263 if ("NOV".equals(codeString)) 264 return NOV; 265 if ("NYB".equals(codeString)) 266 return NYB; 267 if ("ORT".equals(codeString)) 268 return ORT; 269 if ("OTC".equals(codeString)) 270 return OTC; 271 if ("PD".equals(codeString)) 272 return PD; 273 if ("PMC".equals(codeString)) 274 return PMC; 275 if ("PRX".equals(codeString)) 276 return PRX; 277 if ("SCL".equals(codeString)) 278 return SCL; 279 if ("SI".equals(codeString)) 280 return SI; 281 if ("SKB".equals(codeString)) 282 return SKB; 283 if ("USA".equals(codeString)) 284 return USA; 285 if ("WA".equals(codeString)) 286 return WA; 287 if ("WAL".equals(codeString)) 288 return WAL; 289 throw new FHIRException("Unknown V3VaccineManufacturer code '" + codeString + "'"); 290 } 291 292 public String toCode() { 293 switch (this) { 294 case AB: 295 return "AB"; 296 case AD: 297 return "AD"; 298 case ALP: 299 return "ALP"; 300 case AR: 301 return "AR"; 302 case AVI: 303 return "AVI"; 304 case BA: 305 return "BA"; 306 case BAY: 307 return "BAY"; 308 case BP: 309 return "BP"; 310 case BPC: 311 return "BPC"; 312 case CEN: 313 return "CEN"; 314 case CHI: 315 return "CHI"; 316 case CON: 317 return "CON"; 318 case EVN: 319 return "EVN"; 320 case GRE: 321 return "GRE"; 322 case IAG: 323 return "IAG"; 324 case IM: 325 return "IM"; 326 case IUS: 327 return "IUS"; 328 case JPN: 329 return "JPN"; 330 case KGC: 331 return "KGC"; 332 case LED: 333 return "LED"; 334 case MA: 335 return "MA"; 336 case MED: 337 return "MED"; 338 case MIL: 339 return "MIL"; 340 case MIP: 341 return "MIP"; 342 case MSD: 343 return "MSD"; 344 case NAB: 345 return "NAB"; 346 case NAV: 347 return "NAV"; 348 case NOV: 349 return "NOV"; 350 case NYB: 351 return "NYB"; 352 case ORT: 353 return "ORT"; 354 case OTC: 355 return "OTC"; 356 case PD: 357 return "PD"; 358 case PMC: 359 return "PMC"; 360 case PRX: 361 return "PRX"; 362 case SCL: 363 return "SCL"; 364 case SI: 365 return "SI"; 366 case SKB: 367 return "SKB"; 368 case USA: 369 return "USA"; 370 case WA: 371 return "WA"; 372 case WAL: 373 return "WAL"; 374 case NULL: 375 return null; 376 default: 377 return "?"; 378 } 379 } 380 381 public String getSystem() { 382 return "http://terminology.hl7.org/CodeSystem/v3-VaccineManufacturer"; 383 } 384 385 public String getDefinition() { 386 switch (this) { 387 case AB: 388 return "Abbott Laboratories (includes Ross Products Division)"; 389 case AD: 390 return "Adams Laboratories"; 391 case ALP: 392 return "Alpha Therapeutic Corporation"; 393 case AR: 394 return "Armour [Inactive-use CEN]"; 395 case AVI: 396 return "Aviron"; 397 case BA: 398 return "Baxter Healthcare Corporation"; 399 case BAY: 400 return "Bayer Corporation (includes Miles, Inc. and Cutter Laboratories)"; 401 case BP: 402 return "Berna Products [Inactive-use BPC]"; 403 case BPC: 404 return "Berna Products Corporation (includes Swiss Serum and Vaccine Institute Berne)"; 405 case CEN: 406 return "Centeon L.L.C. (includes Armour Pharmaceutical Company)"; 407 case CHI: 408 return "Chiron Corporation"; 409 case CON: 410 return "Connaught [Inactive-use PMC]"; 411 case EVN: 412 return "Evans Medical Limited (an affiliate of Medeva Pharmaceuticals, Inc.)"; 413 case GRE: 414 return "Greer Laboratories, Inc."; 415 case IAG: 416 return "Immuno International AG"; 417 case IM: 418 return "Merieux [Inactive-use PMC]"; 419 case IUS: 420 return "Immuno-U.S., Inc."; 421 case JPN: 422 return "The Research Foundation for Microbial Diseases of Osaka University (BIKEN)"; 423 case KGC: 424 return "Korea Green Cross Corporation"; 425 case LED: 426 return "Lederle [Inactive-use WAL]"; 427 case MA: 428 return "Massachusetts Public Health Biologic Laboratories"; 429 case MED: 430 return "MedImmune, Inc."; 431 case MIL: 432 return "Miles [Inactive-use BAY]"; 433 case MIP: 434 return "Bioport Corporation (formerly Michigan Biologic Products Institute)"; 435 case MSD: 436 return "Merck & Co., Inc."; 437 case NAB: 438 return "NABI (formerly North American Biologicals, Inc.)"; 439 case NAV: 440 return "North American Vaccine, Inc."; 441 case NOV: 442 return "Novartis Pharmaceutical Corporation (includes Ciba-Geigy Limited and Sandoz Limited)"; 443 case NYB: 444 return "New York Blood Center"; 445 case ORT: 446 return "Ortho Diagnostic Systems, Inc."; 447 case OTC: 448 return "Organon Teknika Corporation"; 449 case PD: 450 return "Parkedale Pharmaceuticals (formerly Parke-Davis)"; 451 case PMC: 452 return "Aventis Pasteur Inc. (formerly Pasteur Merieux Connaught; includes Connaught Laboratories and Pasteur Merieux)"; 453 case PRX: 454 return "Praxis Biologics [Inactive-use WAL]"; 455 case SCL: 456 return "Sclavo, Inc."; 457 case SI: 458 return "Swiss Serum and Vaccine Inst. [Inactive-use BPC]"; 459 case SKB: 460 return "SmithKline Beecham"; 461 case USA: 462 return "United States Army Medical Research and Materiel Command"; 463 case WA: 464 return "Wyeth-Ayerst [Inactive-use WAL]"; 465 case WAL: 466 return "Wyeth-Ayerst (includes Wyeth-Lederle Vaccines and Pediatrics, Wyeth Laboratories, Lederle Laboratories, and Praxis Biologics)"; 467 case NULL: 468 return null; 469 default: 470 return "?"; 471 } 472 } 473 474 public String getDisplay() { 475 switch (this) { 476 case AB: 477 return "Abbott Laboratories (includes Ross Products Division)"; 478 case AD: 479 return "Adams Laboratories"; 480 case ALP: 481 return "Alpha Therapeutic Corporation"; 482 case AR: 483 return "Armour [Inactive - use CEN]"; 484 case AVI: 485 return "Aviron"; 486 case BA: 487 return "Baxter Healthcare Corporation"; 488 case BAY: 489 return "Bayer Corporation (includes Miles, Inc. and Cutter Laboratories)"; 490 case BP: 491 return "Berna Products [Inactive - use BPC]"; 492 case BPC: 493 return "Berna Products Corporation (includes Swiss Serum and Vaccine Institute Berne)"; 494 case CEN: 495 return "Centeon L.L.C. (includes Armour Pharmaceutical Company)"; 496 case CHI: 497 return "Chiron Corporation"; 498 case CON: 499 return "Connaught [Inactive - use PMC]"; 500 case EVN: 501 return "Evans Medical Limited (an affiliate of Medeva Pharmaceuticals, Inc.)"; 502 case GRE: 503 return "Greer Laboratories, Inc."; 504 case IAG: 505 return "Immuno International AG"; 506 case IM: 507 return "Merieux [Inactive - use PMC]"; 508 case IUS: 509 return "Immuno-U.S., Inc."; 510 case JPN: 511 return "The Research Foundation for Microbial Diseases of Osaka University (BIKEN)"; 512 case KGC: 513 return "Korea Green Cross Corporation"; 514 case LED: 515 return "Lederle [Inactive - use WAL]"; 516 case MA: 517 return "Massachusetts Public Health Biologic Laboratories"; 518 case MED: 519 return "MedImmune, Inc."; 520 case MIL: 521 return "Miles [Inactive - use BAY]"; 522 case MIP: 523 return "Bioport Corporation (formerly Michigan Biologic Products Institute)"; 524 case MSD: 525 return "Merck and Co., Inc."; 526 case NAB: 527 return "NABI (formerly North American Biologicals, Inc.)"; 528 case NAV: 529 return "North American Vaccine, Inc."; 530 case NOV: 531 return "Novartis Pharmaceutical Corporation (includes Ciba-Geigy Limited and Sandoz Limited)"; 532 case NYB: 533 return "New York Blood Center"; 534 case ORT: 535 return "Ortho Diagnostic Systems, Inc."; 536 case OTC: 537 return "Organon Teknika Corporation"; 538 case PD: 539 return "Parkedale Pharmaceuticals (formerly Parke-Davis)"; 540 case PMC: 541 return "Aventis Pasteur Inc. (formerly Pasteur Merieux Connaught; includes Connaught Laboratories and Pasteur Merieux)"; 542 case PRX: 543 return "Praxis Biologics [Inactive - use WAL]"; 544 case SCL: 545 return "Sclavo, Inc."; 546 case SI: 547 return "Swiss Serum and Vaccine Inst. [Inactive - use BPC]"; 548 case SKB: 549 return "SmithKline Beecham"; 550 case USA: 551 return "United States Army Medical Research and Materiel Command"; 552 case WA: 553 return "Wyeth-Ayerst [Inactive - use WAL]"; 554 case WAL: 555 return "Wyeth-Ayerst (includes Wyeth-Lederle Vaccines and Pediatrics, Wyeth Laboratories, Lederle Laboratories, and Praxis Biologics)"; 556 case NULL: 557 return null; 558 default: 559 return "?"; 560 } 561 } 562 563}