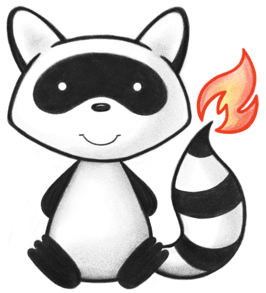
001package org.hl7.fhir.r4.terminologies; 002 003import java.io.IOException; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032 */ 033 034 035import java.net.URISyntaxException; 036import java.util.Map; 037 038import org.hl7.fhir.exceptions.FHIRException; 039import org.hl7.fhir.r4.context.HTMLClientLogger; 040import org.hl7.fhir.r4.model.CapabilityStatement; 041import org.hl7.fhir.r4.model.CodeSystem; 042import org.hl7.fhir.r4.model.Parameters; 043import org.hl7.fhir.r4.model.TerminologyCapabilities; 044import org.hl7.fhir.r4.model.ValueSet; 045import org.hl7.fhir.r4.utils.client.FHIRToolingClient; 046 047public class TerminologyClientR4 implements TerminologyClient { 048 049 private FHIRToolingClient client; 050 051 public TerminologyClientR4(String address, String userAgent) throws URISyntaxException { 052 client = new FHIRToolingClient(address, userAgent); 053 } 054 055 @Override 056 public TerminologyCapabilities getTerminologyCapabilities() { 057 return client.getTerminologyCapabilities(); 058 } 059 060 @Override 061 public String getAddress() { 062 return client.getAddress(); 063 } 064 065 @Override 066 public ValueSet expandValueset(ValueSet vs, Parameters p) { 067 return client.expandValueset(vs, p); 068 } 069 070 @Override 071 public Parameters validateCS(Parameters pin) { 072 try { 073 return client.operateType(CodeSystem.class, "validate-code", pin); 074 } catch (IOException e) { 075 throw new FHIRException(e); 076 } 077 } 078 079 @Override 080 public Parameters validateVS(Parameters pin) { 081 try { 082 return client.operateType(ValueSet.class, "validate-code", pin); 083 } catch (IOException e) { 084 throw new FHIRException(e); 085 } 086 } 087 088 @Override 089 public void setTimeout(int i) { 090 client.setTimeoutNormal(i); 091 } 092 093 @Override 094 public void setLogger(HTMLClientLogger txLog) { 095 client.setLogger(txLog); 096 } 097 098 @Override 099 public CapabilityStatement getCapabilitiesStatementQuick() { 100 return client.getCapabilitiesStatementQuick(); 101 } 102 103 @Override 104 public Parameters lookupCode(Map<String, String> params) { 105 return client.lookupCode(params); 106 } 107 108}