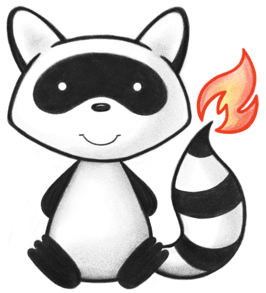
001package org.hl7.fhir.r4.utils; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032import org.hl7.fhir.r4.model.ImplementationGuide.GuideParameterCode; 033import org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDefinitionComponent; 034import org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDefinitionParameterComponent; 035 036@Deprecated 037public class IGHelper { 038 039 public static String readStringParameter(ImplementationGuideDefinitionComponent ig, GuideParameterCode name) { 040 for (ImplementationGuideDefinitionParameterComponent p : ig.getParameter()) { 041 if (name.toCode().equals(p.getCode())) { 042 return p.getValue(); 043 } 044 } 045 return null; 046 } 047 048 public static boolean getBooleanParameter(ImplementationGuideDefinitionComponent ig, GuideParameterCode name, 049 boolean defaultValue) { 050 String v = readStringParameter(ig, name); 051 return v == null ? false : Boolean.parseBoolean(v); 052 } 053 054 public static void setParameter(ImplementationGuideDefinitionComponent ig, GuideParameterCode name, String value) { 055 for (ImplementationGuideDefinitionParameterComponent p : ig.getParameter()) { 056 if (name.toCode().equals(p.getCode())) { 057 p.setValue(value); 058 return; 059 } 060 } 061 ImplementationGuideDefinitionParameterComponent p = ig.addParameter(); 062 p.setCode(name.toCode()); 063 p.setValue(value); 064 } 065 066 public static void setParameter(ImplementationGuideDefinitionComponent ig, GuideParameterCode name, boolean value) { 067 setParameter(ig, name, Boolean.toString(value)); 068 } 069 070}