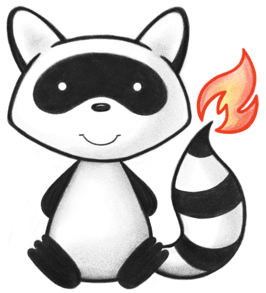
001package org.hl7.fhir.r4.utils; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032import org.hl7.fhir.r4.model.CodeableConcept; 033import org.hl7.fhir.r4.model.IntegerType; 034import org.hl7.fhir.r4.model.Narrative.NarrativeStatus; 035import org.hl7.fhir.r4.model.OperationOutcome; 036import org.hl7.fhir.r4.model.OperationOutcome.IssueSeverity; 037import org.hl7.fhir.r4.model.OperationOutcome.IssueType; 038import org.hl7.fhir.r4.model.OperationOutcome.OperationOutcomeIssueComponent; 039import org.hl7.fhir.utilities.validation.ValidationMessage; 040import org.hl7.fhir.utilities.xhtml.NodeType; 041import org.hl7.fhir.utilities.xhtml.XhtmlNode; 042 043public class OperationOutcomeUtilities { 044 045 public static OperationOutcomeIssueComponent convertToIssue(ValidationMessage message, OperationOutcome op) { 046 OperationOutcomeIssueComponent issue = new OperationOutcome.OperationOutcomeIssueComponent(); 047 issue.setCode(convert(message.getType())); 048 049 if (message.getLocation() != null) { 050 // message location has a fhirPath in it. We need to populate the expression 051 issue.addExpression(message.getLocation()); 052 } 053 // pass through line/col if they're present 054 if (message.getLine() != 0) 055 issue.addExtension().setUrl(ToolingExtensions.EXT_ISSUE_LINE).setValue(new IntegerType(message.getLine())); 056 if (message.getCol() != 0) 057 issue.addExtension().setUrl(ToolingExtensions.EXT_ISSUE_COL).setValue(new IntegerType(message.getCol())); 058 issue.setSeverity(convert(message.getLevel())); 059 CodeableConcept c = new CodeableConcept(); 060 c.setText(message.getMessage()); 061 issue.setDetails(c); 062 if (message.getSource() != null) { 063 issue.getExtension().add(ToolingExtensions.makeIssueSource(message.getSource())); 064 } 065 return issue; 066 } 067 068 private static IssueSeverity convert(org.hl7.fhir.utilities.validation.ValidationMessage.IssueSeverity level) { 069 switch (level) { 070 case FATAL: 071 return IssueSeverity.FATAL; 072 case ERROR: 073 return IssueSeverity.ERROR; 074 case WARNING: 075 return IssueSeverity.WARNING; 076 case INFORMATION: 077 return IssueSeverity.INFORMATION; 078 case NULL: 079 return IssueSeverity.NULL; 080 } 081 return IssueSeverity.NULL; 082 } 083 084 private static IssueType convert(org.hl7.fhir.utilities.validation.ValidationMessage.IssueType type) { 085 switch (type) { 086 case INVALID: 087 case STRUCTURE: 088 return IssueType.STRUCTURE; 089 case REQUIRED: 090 return IssueType.REQUIRED; 091 case VALUE: 092 return IssueType.VALUE; 093 case INVARIANT: 094 return IssueType.INVARIANT; 095 case SECURITY: 096 return IssueType.SECURITY; 097 case LOGIN: 098 return IssueType.LOGIN; 099 case UNKNOWN: 100 return IssueType.UNKNOWN; 101 case EXPIRED: 102 return IssueType.EXPIRED; 103 case FORBIDDEN: 104 return IssueType.FORBIDDEN; 105 case SUPPRESSED: 106 return IssueType.SUPPRESSED; 107 case PROCESSING: 108 return IssueType.PROCESSING; 109 case NOTSUPPORTED: 110 return IssueType.NOTSUPPORTED; 111 case DUPLICATE: 112 return IssueType.DUPLICATE; 113 case NOTFOUND: 114 return IssueType.NOTFOUND; 115 case TOOLONG: 116 return IssueType.TOOLONG; 117 case CODEINVALID: 118 return IssueType.CODEINVALID; 119 case EXTENSION: 120 return IssueType.EXTENSION; 121 case TOOCOSTLY: 122 return IssueType.TOOCOSTLY; 123 case BUSINESSRULE: 124 return IssueType.BUSINESSRULE; 125 case CONFLICT: 126 return IssueType.CONFLICT; 127 case INCOMPLETE: 128 return IssueType.INCOMPLETE; 129 case TRANSIENT: 130 return IssueType.TRANSIENT; 131 case LOCKERROR: 132 return IssueType.LOCKERROR; 133 case NOSTORE: 134 return IssueType.NOSTORE; 135 case EXCEPTION: 136 return IssueType.EXCEPTION; 137 case TIMEOUT: 138 return IssueType.TIMEOUT; 139 case THROTTLED: 140 return IssueType.THROTTLED; 141 case INFORMATIONAL: 142 return IssueType.INFORMATIONAL; 143 case NULL: 144 return IssueType.NULL; 145 } 146 return IssueType.NULL; 147 } 148 149 150 public static OperationOutcome outcomeFromTextError(String text) { 151 OperationOutcome oo = new OperationOutcome(); 152 oo.getText().setStatus(NarrativeStatus.GENERATED); 153 oo.getText().setDiv(new XhtmlNode(NodeType.Element, "div")); 154 oo.getText().getDiv().tx(text); 155 OperationOutcomeIssueComponent issue = oo.addIssue(); 156 issue.setSeverity(IssueSeverity.ERROR); 157 issue.setCode(IssueType.EXCEPTION); 158 issue.getDetails().setText(text); 159 return oo; 160 } 161 162}