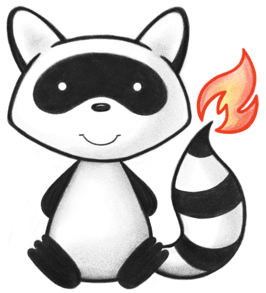
001package org.hl7.fhir.r4.utils.validation; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032import java.io.IOException; 033import java.io.InputStream; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.r4.elementmodel.Manager.FhirFormat; 038import org.hl7.fhir.r4.model.StructureDefinition; 039import org.hl7.fhir.r4.utils.ValidationProfileSet; 040import org.hl7.fhir.r4.utils.validation.constants.BestPracticeWarningLevel; 041import org.hl7.fhir.r4.utils.validation.constants.CheckDisplayOption; 042import org.hl7.fhir.r4.utils.validation.constants.IdStatus; 043import org.hl7.fhir.utilities.MarkedToMoveToAdjunctPackage; 044import org.hl7.fhir.utilities.validation.ValidationMessage; 045 046import com.google.gson.JsonObject; 047 048/** 049 * Interface to the instance validator. This takes a resource, in one of many 050 * forms, and checks whether it is valid 051 * 052 * @author Grahame Grieve 053 * 054 */ 055@MarkedToMoveToAdjunctPackage 056public interface IResourceValidator { 057 058 /** 059 * how much to check displays for coded elements 060 */ 061 CheckDisplayOption getCheckDisplay(); 062 063 void setCheckDisplay(CheckDisplayOption checkDisplay); 064 065 /** 066 * whether the resource must have an id or not (depends on context) 067 */ 068 IdStatus getResourceIdRule(); 069 070 void setResourceIdRule(IdStatus resourceIdRule); 071 072 /** 073 * whether the validator should enforce best practice guidelines as defined by 074 * various HL7 committees 075 */ 076 BestPracticeWarningLevel getBestPracticeWarningLevel(); 077 078 IResourceValidator setBestPracticeWarningLevel(BestPracticeWarningLevel value); 079 080 IValidatorResourceFetcher getFetcher(); 081 082 IResourceValidator setFetcher(IValidatorResourceFetcher value); 083 084 IValidationPolicyAdvisor getPolicyAdvisor(); 085 086 IResourceValidator setPolicyAdvisor(IValidationPolicyAdvisor advisor); 087 088 boolean isNoBindingMsgSuppressed(); 089 090 IResourceValidator setNoBindingMsgSuppressed(boolean noBindingMsgSuppressed); 091 092 boolean isNoInvariantChecks(); 093 094 IResourceValidator setNoInvariantChecks(boolean value); 095 096 boolean isNoTerminologyChecks(); 097 098 IResourceValidator setNoTerminologyChecks(boolean noTerminologyChecks); 099 100 boolean isNoExtensibleWarnings(); 101 102 IResourceValidator setNoExtensibleWarnings(boolean noExtensibleWarnings); 103 104 /** 105 * Whether being unable to resolve a profile in found in Resource.meta.profile 106 * or ElementDefinition.type.profile or targetProfile is an error or just a 107 * warning 108 */ 109 boolean isErrorForUnknownProfiles(); 110 111 void setErrorForUnknownProfiles(boolean errorForUnknownProfiles); 112 113 String getValidationLanguage(); 114 115 void setValidationLanguage(String value); 116 117 /** 118 * Validate suite 119 * 120 * you can validate one of the following representations of resources: 121 * 122 * stream - provide a format - this is the preferred choice 123 * 124 * Use one of these two if the content is known to be valid XML/JSON, and 125 * already parsed - a DOM element or Document - a Json Object 126 * 127 * In order to use these, the content must already be parsed - e.g. it must 128 * syntactically valid - a native resource - a elementmodel resource 129 * 130 * in addition, you can pass one or more profiles ti validate beyond the base 131 * standard - as structure definitions or canonical URLs 132 * 133 * @throws IOException 134 */ 135 void validate(Object Context, List<ValidationMessage> errors, org.hl7.fhir.r4.elementmodel.Element element) 136 throws FHIRException; 137 138 void validate(Object Context, List<ValidationMessage> errors, org.hl7.fhir.r4.elementmodel.Element element, 139 ValidationProfileSet profiles) throws FHIRException; 140 141 void validate(Object Context, List<ValidationMessage> errors, org.hl7.fhir.r4.elementmodel.Element element, 142 String profile) throws FHIRException; 143 144 void validate(Object Context, List<ValidationMessage> errors, org.hl7.fhir.r4.elementmodel.Element element, 145 StructureDefinition profile) throws FHIRException; 146 147 org.hl7.fhir.r4.elementmodel.Element validate(Object Context, List<ValidationMessage> errors, InputStream stream, 148 FhirFormat format) throws FHIRException; 149 150 org.hl7.fhir.r4.elementmodel.Element validate(Object Context, List<ValidationMessage> errors, InputStream stream, 151 FhirFormat format, ValidationProfileSet profiles) throws FHIRException; 152 153 org.hl7.fhir.r4.elementmodel.Element validate(Object Context, List<ValidationMessage> errors, InputStream stream, 154 FhirFormat format, String profile) throws FHIRException; 155 156 org.hl7.fhir.r4.elementmodel.Element validate(Object Context, List<ValidationMessage> errors, InputStream stream, 157 FhirFormat format, StructureDefinition profile) throws FHIRException; 158 159 org.hl7.fhir.r4.elementmodel.Element validate(Object Context, List<ValidationMessage> errors, 160 org.hl7.fhir.r4.model.Resource resource) throws FHIRException; 161 162 org.hl7.fhir.r4.elementmodel.Element validate(Object Context, List<ValidationMessage> errors, 163 org.hl7.fhir.r4.model.Resource resource, ValidationProfileSet profiles) throws FHIRException; 164 165 org.hl7.fhir.r4.elementmodel.Element validate(Object Context, List<ValidationMessage> errors, 166 org.hl7.fhir.r4.model.Resource resource, String profile) throws FHIRException; 167 168 org.hl7.fhir.r4.elementmodel.Element validate(Object Context, List<ValidationMessage> errors, 169 org.hl7.fhir.r4.model.Resource resource, StructureDefinition profile) throws FHIRException; 170 171 org.hl7.fhir.r4.elementmodel.Element validate(Object Context, List<ValidationMessage> errors, 172 org.w3c.dom.Element element) throws FHIRException; 173 174 org.hl7.fhir.r4.elementmodel.Element validate(Object Context, List<ValidationMessage> errors, 175 org.w3c.dom.Element element, ValidationProfileSet profiles) throws FHIRException; 176 177 org.hl7.fhir.r4.elementmodel.Element validate(Object Context, List<ValidationMessage> errors, 178 org.w3c.dom.Element element, String profile) throws FHIRException; 179 180 org.hl7.fhir.r4.elementmodel.Element validate(Object Context, List<ValidationMessage> errors, 181 org.w3c.dom.Element element, StructureDefinition profile) throws FHIRException; 182 183 org.hl7.fhir.r4.elementmodel.Element validate(Object Context, List<ValidationMessage> errors, 184 org.w3c.dom.Document document) throws FHIRException; 185 186 org.hl7.fhir.r4.elementmodel.Element validate(Object Context, List<ValidationMessage> errors, 187 org.w3c.dom.Document document, ValidationProfileSet profiles) throws FHIRException; 188 189 org.hl7.fhir.r4.elementmodel.Element validate(Object Context, List<ValidationMessage> errors, 190 org.w3c.dom.Document document, String profile) throws FHIRException; 191 192 org.hl7.fhir.r4.elementmodel.Element validate(Object Context, List<ValidationMessage> errors, 193 org.w3c.dom.Document document, StructureDefinition profile) throws FHIRException; 194 195 org.hl7.fhir.r4.elementmodel.Element validate(Object Context, List<ValidationMessage> errors, JsonObject object) 196 throws FHIRException; 197 198 org.hl7.fhir.r4.elementmodel.Element validate(Object Context, List<ValidationMessage> errors, JsonObject object, 199 ValidationProfileSet profiles) throws FHIRException; 200 201 org.hl7.fhir.r4.elementmodel.Element validate(Object Context, List<ValidationMessage> errors, JsonObject object, 202 String profile) throws FHIRException; 203 204 org.hl7.fhir.r4.elementmodel.Element validate(Object Context, List<ValidationMessage> errors, JsonObject object, 205 StructureDefinition profile) throws FHIRException; 206 207}