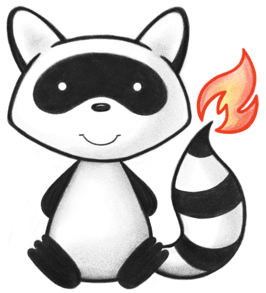
Package org.hl7.fhir.r5.profilemodel
Class PEBuilder
java.lang.Object
org.hl7.fhir.r5.profilemodel.PEBuilder
Factory class for the ProfiledElement sub-system
*** NOTE: This sub-system is still under development ***
This subsystem takes a profile and creates a view of the profile that stitches
all the parts together, and presents it as a seamless tree. There's two views:
- definition: A logical view of the contents of the profile
- instance: a logical view of a resource that conforms to the profile
The tree of elements in the profile model is different to the the base resource:
- some elements are removed (max = 0)
- extensions are turned into named elements
- slices are turned into named elements
- element properties - doco, cardinality, binding etc is updated for what the profile says
Definition
----------
This presents a single view of the contents of a resource as specified by
the profile. It's suitable for use in any kind of tree view.
Each node has a unique name amongst it's siblings, but this name may not be
the name in the instance, since slicing splits up a single named element into
different definitions.
Each node has:
- name (unique amongst siblings)
- schema name (the actual name in the instance)
- min cardinality
- max cardinality
- short documentation (for the tree view)
- full documentation (markdown source)
- profile definition - the full definition in the profile
- base definition - the full definition at the resource level
- types() - a list of possible types
- children(type) - a list of child nodes for the provided type
- expansion - if there's a binding, the codes in the expansion based on the binding
Note that the tree may not have leaves; the trees recurse indefinitely because
extensions have extensions etc. So you can't do a depth-first search of the tree
without some kind of decision to stop at a given point.
Instance
--------
todo
- Author:
- grahamegrieve
-
Nested Class Summary
Nested Classes -
Constructor Summary
ConstructorsConstructorDescriptionPEBuilder
(IWorkerContext context, PEBuilder.PEElementPropertiesPolicy elementProps, boolean fixedPropsDefault) -
Method Summary
Modifier and TypeMethodDescriptionbuildPEDefinition
(String url) Given a profile, return a tree of the elements defined in the profile model.buildPEDefinition
(String url, String version) Given a profile, return a tree of the elements defined in the profile model.buildPEDefinition
(StructureDefinition profile) Given a profile, return a tree of the elements defined in the profile model.buildPEInstance
(String url, String version, Resource resource) Given a resource and a profile, return a tree of instance data as defined by the profile model using the nominated version of the profile The tree is a facade to the underlying resource - all actual data is stored against the resource, and retrieved on the fly from the resource, so that applications can work at either level, as convenient.buildPEInstance
(String url, Resource resource) Given a resource and a profile, return a tree of instance data as defined by the profile model using the latest version of the profile The tree is a facade to the underlying resource - all actual data is stored against the resource, and retrieved on the fly from the resource, so that applications can work at either level, as convenient.buildPEInstance
(StructureDefinition profile, Resource resource) Given a resource and a profile, return a tree of instance data as defined by the profile model using the provided version of the profile The tree is a facade to the underlying resource - all actual data is stored against the resource, and retrieved on the fly from the resource, so that applications can work at either level, as convenient.createResource
(String url, boolean meta) For the current version of a profile, construct a resource and fill out any fixed or required elements Note that fixed values are filled out irrespective of the value of fixedProps when the builder is createdcreateResource
(String url, String version, boolean meta) For the current version of a profile, construct a resource and fill out any fixed or required elements Note that fixed values are filled out irrespective of the value of fixedProps when the builder is createdcreateResource
(StructureDefinition profile, boolean meta) For the provided version of a profile, construct a resource and fill out any fixed or required elements Note that fixed values are filled out irrespective of the value of fixedProps when the builder is createdprotected List
<ElementDefinition> getChildren
(StructureDefinition profileStructure, ElementDefinition definition) boolean
isResource
(String name) protected List
<PEDefinition> listChildren
(boolean allFixed, PEDefinition parent, StructureDefinition profileStructure, ElementDefinition definition, String url, String... omitList) protected List
<PEDefinition> listSlices
(StructureDefinition profileStructure, ElementDefinition definition, PEDefinition parent) protected PEDefinition
makeChild
(PEDefinition parent, StructureDefinition profileStructure, ElementDefinition definition) makeSliceExpression
(StructureDefinition profile, ElementDefinition.ElementDefinitionSlicingComponent slicing, ElementDefinition definition) protected PEType
protected PEType
protected PEType
protected PEType
protected void
populateByProfile
(Base base, PEDefinition definition)
-
Constructor Details
-
PEBuilder
public PEBuilder(IWorkerContext context, PEBuilder.PEElementPropertiesPolicy elementProps, boolean fixedPropsDefault) - Parameters:
context
- - must be loaded with R5 definitionselementProps
- - whether to include Element.id and Element.extension in the tree. Recommended choice: Extension
-
-
Method Details
-
buildPEDefinition
Given a profile, return a tree of the elements defined in the profile model. This builds the profile model for the provided version of the nominated profile The tree of elements in the profile model is different to those defined in the base resource: - some elements are removed (max = 0) - extensions are turned into named elements - slices are turned into named elements - element properties - doco, cardinality, binding etc is updated for what the profile says Warning: profiles and resources are recursive; you can't iterate this tree until it you get to the leaves because there are nodes that don't terminate (extensions have extensions) -
buildPEDefinition
Given a profile, return a tree of the elements defined in the profile model. This builds the profile model for the latest version of the nominated profile The tree of elements in the profile model is different to those defined in the base resource: - some elements are removed (max = 0) - extensions are turned into named elements - slices are turned into named elements - element properties - doco, cardinality, binding etc is updated for what the profile says Warning: profiles and resources are recursive; you can't iterate this tree until it you get to the leaves because there are nodes that don't terminate (extensions have extensions) -
buildPEDefinition
Given a profile, return a tree of the elements defined in the profile model. This builds the profile model for the nominated version of the nominated profile The tree of elements in the profile model is different to the the base resource: - some elements are removed (max = 0) - extensions are turned into named elements - slices are turned into named elements - element properties - doco, cardinality, binding etc is updated for what the profile says Warning: profiles and resources can be recursive; you can't iterate this tree until it you get to the leaves because you will never get to a child that doesn't have children -
buildPEInstance
Given a resource and a profile, return a tree of instance data as defined by the profile model using the latest version of the profile The tree is a facade to the underlying resource - all actual data is stored against the resource, and retrieved on the fly from the resource, so that applications can work at either level, as convenient. Note that there's a risk that deleting something through the resource while holding a handle to a PEInstance that is a facade on what is deleted leaves an orphan facade that will continue to function, but is making changes to resource content that is no longer part of the resource -
buildPEInstance
Given a resource and a profile, return a tree of instance data as defined by the profile model using the provided version of the profile The tree is a facade to the underlying resource - all actual data is stored against the resource, and retrieved on the fly from the resource, so that applications can work at either level, as convenient. Note that there's a risk that deleting something through the resource while holding a handle to a PEInstance that is a facade on what is deleted leaves an orphan facade that will continue to function, but is making changes to resource content that is no longer part of the resource -
buildPEInstance
Given a resource and a profile, return a tree of instance data as defined by the profile model using the nominated version of the profile The tree is a facade to the underlying resource - all actual data is stored against the resource, and retrieved on the fly from the resource, so that applications can work at either level, as convenient. Note that there's a risk that deleting something through the resource while holding a handle to a PEInstance that is a facade on what is deleted leaves an orphan facade that will continue to function, but is making changes to resource content that is no longer part of the resource -
createResource
For the current version of a profile, construct a resource and fill out any fixed or required elements Note that fixed values are filled out irrespective of the value of fixedProps when the builder is created- Parameters:
url
- identifies the profileversion
- identifies the version of the profilemeta
- whether to mark the profile in Resource.meta.profile- Returns:
- constructed resource
-
createResource
For the provided version of a profile, construct a resource and fill out any fixed or required elements Note that fixed values are filled out irrespective of the value of fixedProps when the builder is created- Parameters:
profile
- the profilemeta
- whether to mark the profile in Resource.meta.profile- Returns:
- constructed resource
-
createResource
For the current version of a profile, construct a resource and fill out any fixed or required elements Note that fixed values are filled out irrespective of the value of fixedProps when the builder is created- Parameters:
url
- identifies the profilemeta
- whether to mark the profile in Resource.meta.profile- Returns:
- constructed resource
-
listChildren
protected List<PEDefinition> listChildren(boolean allFixed, PEDefinition parent, StructureDefinition profileStructure, ElementDefinition definition, String url, String... omitList) -
makeChild
protected PEDefinition makeChild(PEDefinition parent, StructureDefinition profileStructure, ElementDefinition definition) -
listSlices
protected List<PEDefinition> listSlices(StructureDefinition profileStructure, ElementDefinition definition, PEDefinition parent) -
makeType
-
makeType
-
makeType
-
makeType
-
getChildren
protected List<ElementDefinition> getChildren(StructureDefinition profileStructure, ElementDefinition definition) -
getContext
-
populateByProfile
-
makeSliceExpression
public String makeSliceExpression(StructureDefinition profile, ElementDefinition.ElementDefinitionSlicingComponent slicing, ElementDefinition definition) -
exec
-
isResource
-
getContextUtilities
-