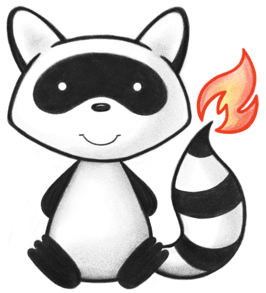
001package org.hl7.fhir.r5.comparison; 002 003import java.util.ArrayList; 004import java.util.HashMap; 005import java.util.List; 006import java.util.Map; 007 008import org.hl7.fhir.r5.comparison.CanonicalResourceComparer.CanonicalResourceComparison; 009import org.hl7.fhir.r5.model.Base; 010import org.hl7.fhir.r5.model.CanonicalResource; 011import org.hl7.fhir.r5.utils.UserDataNames; 012import org.hl7.fhir.utilities.MarkedToMoveToAdjunctPackage; 013 014@MarkedToMoveToAdjunctPackage 015public class VersionComparisonAnnotation { 016 017 public enum AnotationType { 018 NoChange, Added, Changed, Deleted; 019 } 020 021 022 private AnotationType type; 023 private String original; 024 private Map<String, List<Base>> deletedChildren; 025 private CanonicalResourceComparison<? extends CanonicalResource> comp; 026 027 public VersionComparisonAnnotation(AnotationType type) { 028 super(); 029 this.type = type; 030 } 031 032 public void added() { 033 type = AnotationType.Added; 034 } 035 036 public void changed(Base orig) { 037 assert type == AnotationType.NoChange; 038 type = AnotationType.Changed; 039 if (orig != null && orig.isPrimitive() && orig.primitiveValue().length() < 120) { // arbitrary, but we don't a whack of markdown 040 this.original = orig.primitiveValue(); 041 } 042 } 043 044 public void deleted() { 045 assert type == AnotationType.NoChange; 046 type = AnotationType.Deleted; 047 048 049 } 050 051 public void deleted(String name, Base other) { 052 if (deletedChildren == null) { 053 deletedChildren = new HashMap<>(); 054 } 055 if (!deletedChildren.containsKey(name)) { 056 deletedChildren.put(name, new ArrayList<>()); 057 } 058 deletedChildren.get(name).add(other); 059 } 060 061 public void comp(CanonicalResourceComparison<? extends CanonicalResource> comp) { 062 assert this.comp == null; 063 // TODO Auto-generated method stub 064 if (!comp.noUpdates()) { 065 type = AnotationType.Changed; 066 } 067 this.comp = comp; 068 } 069 070 public AnotationType getType() { 071 return type; 072 } 073 074 public String getOriginal() { 075 return original; 076 } 077 078 public Map<String, List<Base>> getDeletedChildren() { 079 return deletedChildren; 080 } 081 082 public CanonicalResourceComparison<? extends CanonicalResource> getComp() { 083 return comp; 084 } 085 086 087 public static boolean hasDeleted(Base base, String... names) { 088 boolean result = false; 089 if (base.hasUserData(UserDataNames.COMP_VERSION_ANNOTATION)) { 090 VersionComparisonAnnotation self = (VersionComparisonAnnotation) base.getUserData(UserDataNames.COMP_VERSION_ANNOTATION); 091 for (String name : names) { 092 if (self.deletedChildren != null && self.deletedChildren.containsKey(name)) { 093 result = true; 094 } 095 } 096 } 097 return result; 098 } 099 100 public static List<Base> getDeleted(Base base, String... names) { 101 List<Base> result = new ArrayList<>(); 102 if (base.hasUserData(UserDataNames.COMP_VERSION_ANNOTATION)) { 103 VersionComparisonAnnotation self = (VersionComparisonAnnotation) base.getUserData(UserDataNames.COMP_VERSION_ANNOTATION); 104 for (String name : names) { 105 if (self.deletedChildren != null && self.deletedChildren.containsKey(name)) { 106 result.addAll(self.deletedChildren.get(name)); 107 } 108 } 109 } 110 return result; 111 } 112 113 public static Base getDeletedItem(Base base, String name) { 114 List<Base> result = new ArrayList<>(); 115 if (base.hasUserData(UserDataNames.COMP_VERSION_ANNOTATION)) { 116 VersionComparisonAnnotation self = (VersionComparisonAnnotation) base.getUserData(UserDataNames.COMP_VERSION_ANNOTATION); 117 if (self.deletedChildren != null && self.deletedChildren.containsKey(name)) { 118 result.addAll(self.deletedChildren.get(name)); 119 } 120 } 121 return result.isEmpty() ? null : result.get(0); 122 } 123 124 125 126 127 public static CanonicalResourceComparison<? extends CanonicalResource> artifactComparison(Base base) { 128 if (base.hasUserData(UserDataNames.COMP_VERSION_ANNOTATION)) { 129 VersionComparisonAnnotation self = (VersionComparisonAnnotation) base.getUserData(UserDataNames.COMP_VERSION_ANNOTATION); 130 return self.comp; 131 } else { 132 return null; 133 } 134 } 135 136 137}