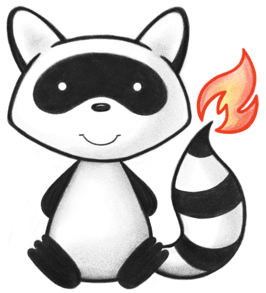
001package org.hl7.fhir.r5.conformance; 002 003import org.hl7.fhir.r5.model.CanonicalType; 004import org.hl7.fhir.r5.model.ElementDefinition; 005import org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionConstraintComponent; 006import org.hl7.fhir.r5.model.ElementDefinition.TypeRefComponent; 007import org.hl7.fhir.r5.model.Resource; 008import org.hl7.fhir.r5.model.StructureDefinition; 009import org.hl7.fhir.utilities.MarkedToMoveToAdjunctPackage; 010import org.hl7.fhir.utilities.VersionUtilities; 011 012/** 013 * This works around known issues in structure definitions 014 * 015 * @author graha 016 * 017 */ 018@MarkedToMoveToAdjunctPackage 019public class StructureDefinitionHacker { 020 021 private String version; 022 023 public StructureDefinitionHacker(String version) { 024 super(); 025 this.version = version; 026 } 027 028 public Resource fixSD(StructureDefinition sd) { 029 if (VersionUtilities.isR4Ver(version) && "http://hl7.org/fhir/StructureDefinition/example-composition".equals(sd.getUrl())) { 030 for (ElementDefinition ed : sd.getSnapshot().getElement()) { 031 fixDocSecURL(ed); 032 } 033 for (ElementDefinition ed : sd.getDifferential().getElement()) { 034 fixDocSecURL(ed); 035 if ("ClinicalImpression.problem".equals(ed.getPath())) { 036 // work around a bidi problem 037 ed.setComment("e.g. The patient is a pregnant, has congestive heart failure, has an Adenocarcinoma, and is allergic to penicillin."); 038 } 039 } 040 } 041 if (VersionUtilities.isR4Ver(version) && "http://hl7.org/fhir/StructureDefinition/ClinicalImpression".equals(sd.getUrl())) { 042 for (ElementDefinition ed : sd.getSnapshot().getElement()) { 043 if ("ClinicalImpression.problem".equals(ed.getPath())) { 044 // work around a bidi problem 045 ed.setComment("e.g. The patient is a pregnant, has congestive heart failure, has an Adenocarcinoma, and is allergic to penicillin."); 046 } 047 } 048 for (ElementDefinition ed : sd.getDifferential().getElement()) { 049 if ("ClinicalImpression.problem".equals(ed.getPath())) { 050 // work around a bidi problem 051 ed.setComment("e.g. The patient is a pregnant, has congestive heart failure, has an Adenocarcinoma, and is allergic to penicillin."); 052 } 053 } 054 } 055 if (VersionUtilities.isR4Ver(version) && "http://hl7.org/fhir/StructureDefinition/Consent".equals(sd.getUrl())) { 056 for (ElementDefinition ed : sd.getSnapshot().getElement()) { 057 if ("Consent.identifier".equals(ed.getPath())) { 058 ed.getExampleFirstRep().getValueIdentifier().setSystem("http://acme.org/identifier/local/eCMS"); 059 } 060 } 061 for (ElementDefinition ed : sd.getDifferential().getElement()) { 062 if ("Consent.identifier".equals(ed.getPath())) { 063 ed.getExampleFirstRep().getValueIdentifier().setSystem("http://acme.org/identifier/local/eCMS"); 064 } 065 } 066 } 067 if (VersionUtilities.isR4Ver(version) && "http://hl7.org/fhir/StructureDefinition/ExplanationOfBenefit".equals(sd.getUrl())) { 068 for (ElementDefinition ed : sd.getSnapshot().getElement()) { 069 if (ed.hasBinding() && "http://terminology.hl7.org/CodeSystem/processpriority".equals(ed.getBinding().getValueSet())) { 070 ed.getBinding().setValueSet("http://hl7.org/fhir/ValueSet/process-priority"); 071 } 072 } 073 for (ElementDefinition ed : sd.getDifferential().getElement()) { 074 if (ed.hasBinding() && "http://terminology.hl7.org/CodeSystem/processpriority".equals(ed.getBinding().getValueSet())) { 075 ed.getBinding().setValueSet("http://hl7.org/fhir/ValueSet/process-priority"); 076 } 077 } 078 } 079 if (sd.getUrl().startsWith("http://hl7.org/fhir/uv/subscriptions-backport")) { 080 for (ElementDefinition ed : sd.getDifferential().getElement()) { 081 fixMarkdownR4BURLs(ed); 082 } 083 for (ElementDefinition ed : sd.getSnapshot().getElement()) { 084 fixMarkdownR4BURLs(ed); 085 } 086 } 087 if ("http://hl7.org/fhir/StructureDefinition/vitalsigns".equals(sd.getUrl()) || "http://hl7.org/fhir/StructureDefinition/vitalsigns".equals(sd.getBaseDefinition())) { 088 for (ElementDefinition ed : sd.getDifferential().getElement()) { 089 checkVSConstraint(ed); 090 } 091 for (ElementDefinition ed : sd.getSnapshot().getElement()) { 092 checkVSConstraint(ed); 093 } 094 } 095 return sd; 096 } 097 098 private void checkVSConstraint(ElementDefinition ed) { 099 for (ElementDefinitionConstraintComponent constraint : ed.getConstraint()) { 100 if ("vs-1".equals(constraint.getKey())) { 101 constraint.setExpression("$this is dateTime implies $this.toString().length() >= 10"); 102 } 103 } 104 } 105 106 private void fixMarkdownR4BURLs(ElementDefinition ed) { 107 if (ed.hasDefinition()) { 108 ed.setDefinition(ed.getDefinition().replace("http://hl7.org/fhir/R4B/", "http://hl7.org/fhir/R4/")); 109 } 110 if (ed.hasComment()) { 111 ed.setComment(ed.getComment().replace("http://hl7.org/fhir/R4B/", "http://hl7.org/fhir/R4/")); 112 } 113 if (ed.hasRequirements()) { 114 ed.setRequirements(ed.getRequirements().replace("http://hl7.org/fhir/R4B/", "http://hl7.org/fhir/R4/")); 115 } 116 } 117 118 private void fixDocSecURL(ElementDefinition ed) { 119 for (TypeRefComponent tr : ed.getType()) { 120 for (CanonicalType c : tr.getProfile()) { 121 if ("http://hl7.org/fhir/StructureDefinition/document-section-library".equals(c.getValue())) { 122 c.setValue("http://hl7.org/fhir/StructureDefinition/example-section-library"); 123 } 124 } 125 } 126 } 127 128 129}