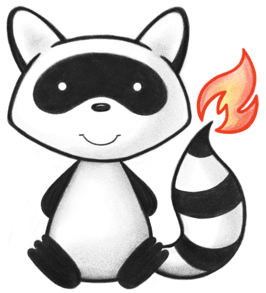
001package org.hl7.fhir.r5.context; 002 003import java.io.IOException; 004import java.io.InputStream; 005import java.util.List; 006 007import org.hl7.fhir.exceptions.FHIRException; 008import org.hl7.fhir.r5.model.Bundle; 009import org.hl7.fhir.r5.model.CodeSystem; 010import org.hl7.fhir.r5.model.Resource; 011import org.hl7.fhir.r5.terminologies.client.TerminologyClientManager.ITerminologyClientFactory; 012import org.hl7.fhir.utilities.npm.NpmPackage; 013import org.hl7.fhir.utilities.npm.NpmPackage.PackageResourceInformation; 014 015import com.google.gson.JsonSyntaxException; 016 017public interface IContextResourceLoader { 018 /** 019 * @return List of the resource types that should be loaded 020 */ 021 List<String> getTypes(); 022 023 /** 024 * Request to actually load the resources and do whatever is required 025 * 026 * @param stream 027 * @param isJson 028 * @return A bundle because some single resources become multiple resources after loading 029 * @throws FHIRException 030 * @throws IOException 031 */ 032 Bundle loadBundle(InputStream stream, boolean isJson) throws FHIRException, IOException; 033 034 /** 035 * Load a single resources (lazy load) 036 * 037 * @param stream 038 * @param isJson 039 * @return 040 * @throws FHIRException - throw this if you a single resource can't be returned - can't lazy load in this circumstance 041 * @throws IOException 042 */ 043 Resource loadResource(InputStream stream, boolean isJson) throws FHIRException, IOException; 044 045 /** 046 * get the path for references to this resource. 047 * @param resource 048 * @return null if not tracking paths 049 */ 050 String getResourcePath(Resource resource); 051 052 /** 053 * called when a new package is being loaded 054 * 055 * this is called by loadPackageAndDependencies when a new package is loaded 056 * @param npm 057 * @return 058 * @throws IOException 059 * @throws JsonSyntaxException 060 */ 061 IContextResourceLoader getNewLoader(NpmPackage npm) throws JsonSyntaxException, IOException; 062 063 /** 064 * called when processing R2 for implicit code systems in ValueSets 065 * 066 * @return 067 */ 068 List<CodeSystem> getCodeSystems(); 069 070 /** 071 * if this is true, then the loader will patch canonical URLs and cross-links 072 * to add /X.X/ into the URL so that different versions can be loaded safely 073 * 074 * default is false 075 */ 076 void setPatchUrls(boolean value); 077 078 /** 079 * patch the URL if necessary 080 * 081 * @param url 082 * @return 083 */ 084 String patchUrl(String url, String resourceType); 085 086 /** 087 * set this to false (default is true) if you don't want profiles loaded 088 * @param value 089 * @return 090 */ 091 IContextResourceLoader setLoadProfiles(boolean value); 092 093 /** 094 * @return the terminology client factory 095 */ 096 ITerminologyClientFactory txFactory(); 097 098 /** 099 * Called during the loading process - the loader can decide which resources to load. 100 * At this point, only the .index.json is being read 101 * 102 * @param pi 103 * @param pri 104 * @return 105 */ 106 boolean wantLoad(NpmPackage pi, PackageResourceInformation pri); 107}