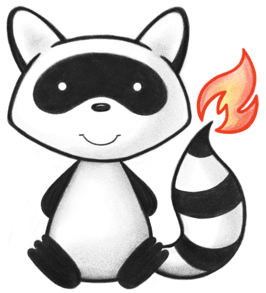
001package org.hl7.fhir.r5.elementmodel; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032 033 034import java.io.IOException; 035import java.io.InputStream; 036import java.io.OutputStream; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.DefinitionException; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.exceptions.FHIRFormatError; 042import org.hl7.fhir.r5.conformance.profile.ProfileUtilities; 043import org.hl7.fhir.r5.context.ContextUtilities; 044import org.hl7.fhir.r5.context.IWorkerContext; 045import org.hl7.fhir.r5.formats.IParser.OutputStyle; 046import org.hl7.fhir.r5.model.StructureDefinition; 047import org.hl7.fhir.utilities.MarkedToMoveToAdjunctPackage; 048 049@MarkedToMoveToAdjunctPackage 050public class Manager { 051 052 //TODO use EnumMap 053 public enum FhirFormat { XML, JSON, TURTLE, TEXT, VBAR, SHC, SHL, FML, NDJSON; 054 // SHC = smart health cards, including as text versions of QR codes 055 // SHL = smart health links, also a text version of the QR code 056 057 public String getExtension() { 058 switch (this) { 059 case JSON: 060 return "json"; 061 case TURTLE: 062 return "ttl"; 063 case XML: 064 return "xml"; 065 case TEXT: 066 return "txt"; 067 case VBAR: 068 return "hl7"; 069 case SHC: 070 return "shc"; 071 case SHL: 072 return "shl"; 073 case FML: 074 return "fml"; 075 case NDJSON: 076 return "ndjson"; 077 } 078 return null; 079 } 080 081 public static FhirFormat getFhirFormat(String code) { 082 switch (code) { 083 case "json": 084 return JSON; 085 case "ttl": 086 return TURTLE; 087 case "xml": 088 return XML; 089 case "txt": 090 return TEXT; 091 case "hl7": 092 return VBAR; 093 case "shc": 094 return SHC; 095 case "shl": 096 return SHL; 097 case "fml": 098 return FML; 099 case "ndjson": 100 return NDJSON; 101 } 102 return null; 103 } 104 public static FhirFormat readFromMimeType(String mt) { 105 if (mt == null) { 106 return null; 107 } 108 if (mt.contains("/xml") || mt.contains("+xml")) { 109 return FhirFormat.XML; 110 } 111 if (mt.contains("/json") || mt.contains("+json")) { 112 return FhirFormat.JSON; 113 } 114 return null; 115 } 116 117 public static FhirFormat fromCode(String code) { 118 FhirFormat fmt = getFhirFormat(code); 119 if (fmt == null) { 120 fmt = readFromMimeType(code); 121 } 122 return fmt; 123 } 124 } 125 126 public static List<ValidatedFragment> parse(IWorkerContext context, InputStream source, FhirFormat inputFormat) throws FHIRFormatError, DefinitionException, IOException, FHIRException { 127 return makeParser(context, inputFormat).parse(source); 128 } 129 130 public static Element parseSingle(IWorkerContext context, InputStream source, FhirFormat inputFormat) throws FHIRFormatError, DefinitionException, IOException, FHIRException { 131 return makeParser(context, inputFormat).parseSingle(source, null); 132 } 133 134 135 public static void compose(IWorkerContext context, Element e, OutputStream destination, FhirFormat outputFormat, OutputStyle style, String base) throws FHIRException, IOException { 136 makeParser(context, outputFormat).compose(e, destination, style, base); 137 } 138 139 public static ParserBase makeParser(IWorkerContext context, FhirFormat format) { 140 if (format == null) { 141 throw new Error("Programming logic error: no format known"); 142 } 143 switch (format) { 144 case JSON : return new JsonParser(context); 145 case NDJSON : return new NDJsonParser(context); 146 case XML : return new XmlParser(context); 147 case TURTLE : return new TurtleParser(context); 148 case VBAR : return new VerticalBarParser(context); 149 case SHC : return new SHCParser(context); 150 case SHL : return new SHLParser(context); 151 case FML : return new FmlParser(context); 152 case TEXT : throw new Error("Programming logic error: do not call makeParser for a text resource"); 153 } 154 return null; 155 } 156 157 public static Element build(IWorkerContext context, StructureDefinition sd) { 158 return build(context, sd, new ProfileUtilities(context, null, null)); 159 } 160 161 public static Element build(IWorkerContext context, StructureDefinition sd, ProfileUtilities profileUtilities) { 162 Property p = new Property(context, sd.getSnapshot().getElementFirstRep(), sd, profileUtilities, new ContextUtilities(context)); 163 Element e = new Element(p.getName(), p); 164 e.setPath(sd.getType()); 165 return e; 166 } 167 168}