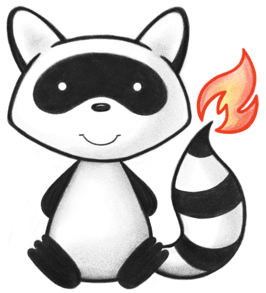
001package org.hl7.fhir.r5.elementmodel; 002 003import java.io.ByteArrayInputStream; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032 */ 033 034import java.io.IOException; 035import java.io.InputStream; 036import java.io.OutputStream; 037import java.nio.charset.StandardCharsets; 038import java.util.ArrayList; 039import java.util.List; 040 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.r5.conformance.profile.ProfileUtilities; 043import org.hl7.fhir.r5.context.IWorkerContext; 044import org.hl7.fhir.r5.formats.IParser.OutputStyle; 045import org.hl7.fhir.r5.formats.JsonCreator; 046import org.hl7.fhir.utilities.FileUtilities; 047import org.hl7.fhir.utilities.MarkedToMoveToAdjunctPackage; 048import org.hl7.fhir.utilities.Utilities; 049import org.hl7.fhir.utilities.i18n.I18nConstants; 050import org.hl7.fhir.utilities.validation.ValidationMessage; 051import org.hl7.fhir.utilities.validation.ValidationMessage.IssueSeverity; 052import org.hl7.fhir.utilities.validation.ValidationMessage.IssueType; 053 054 055@MarkedToMoveToAdjunctPackage 056public class NDJsonParser extends ParserBase { 057 058 public NDJsonParser(IWorkerContext context, ProfileUtilities utilities) { 059 super(context, utilities); 060 } 061 062 public NDJsonParser(IWorkerContext context) { 063 super(context); 064 } 065 066 private ValidatedFragment processLine(int lineCount, String line) throws FHIRException, IOException { 067 List<ValidatedFragment> list = new JsonParser(context).parse(new ByteArrayInputStream(line.getBytes(StandardCharsets.UTF_8)), lineCount); 068 return list.get(0); 069 } 070 071 @Override 072 public List<ValidatedFragment> parse(InputStream inStream) throws IOException, FHIRException { 073 String source = FileUtilities.streamToString(inStream); 074 int length = source.length(); 075 int start = 0; 076 int cursor = start; 077 int lineCount = 0; 078 079 List<ValidatedFragment> res = new ArrayList<>(); 080 while (cursor < length) { 081 while (cursor < length && source.charAt(cursor) != '\n') { 082 cursor++; 083 } 084 if (cursor < length) { 085 String line = source.substring(start, cursor); 086 if (line.endsWith("\r")) { 087 line = line.substring(0, line.length()-1); 088 } 089 if (Utilities.noString(line.trim())) { 090 ValidatedFragment vf = new ValidatedFragment(ValidatedFragment.ITEM_NAME, null, null, false); 091 logError(vf.getErrors(), "2024-06-30", lineCount+1, 1, null, IssueType.INFORMATIONAL, context.formatMessage(I18nConstants.NDJSON_EMPTY_LINE_WARNING), IssueSeverity.WARNING); 092 res.add(vf); 093 } else { 094 res.add(processLine(lineCount, line)); 095 } 096 start = cursor+1; 097 } else if (cursor > start) { 098 String line = source.substring(start, cursor); 099 if (line.endsWith("\r")) { 100 line = line.substring(0, line.length()-1); 101 } 102 if (Utilities.noString(line.trim())) { 103 ValidatedFragment vf = new ValidatedFragment(ValidatedFragment.ITEM_NAME, null, null, false); 104 logError(vf.getErrors(), "2024-06-30", lineCount+1, 1, null, IssueType.INFORMATIONAL, context.formatMessage(I18nConstants.NDJSON_EMPTY_LINE_WARNING), IssueSeverity.WARNING); 105 res.add(vf); 106 } else { 107 res.add(processLine(lineCount, line)); 108 } 109 start = cursor+1; 110 } 111 lineCount++; 112 cursor++; 113 } 114 return res; 115 } 116 117 @Override 118 public void compose(Element e, OutputStream stream, OutputStyle style, String identity) throws FHIRException, IOException { 119 throw new Error("Not done yet"); 120 } 121 122}