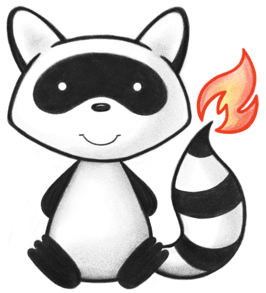
001package org.hl7.fhir.r5.elementmodel; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032import java.io.ByteArrayInputStream; 033import java.io.ByteArrayOutputStream; 034import java.io.IOException; 035import java.util.ArrayList; 036import java.util.List; 037 038import org.hl7.fhir.exceptions.FHIRException; 039import org.hl7.fhir.r5.conformance.profile.ProfileUtilities; 040import org.hl7.fhir.r5.conformance.profile.ProfileUtilities.SourcedChildDefinitions; 041import org.hl7.fhir.r5.context.IWorkerContext; 042import org.hl7.fhir.r5.formats.IParser.OutputStyle; 043import org.hl7.fhir.r5.model.Base; 044import org.hl7.fhir.r5.model.CodeableConcept; 045import org.hl7.fhir.r5.model.Coding; 046import org.hl7.fhir.r5.model.DataType; 047import org.hl7.fhir.r5.model.ElementDefinition; 048import org.hl7.fhir.r5.model.Factory; 049import org.hl7.fhir.r5.model.Identifier; 050import org.hl7.fhir.r5.model.PrimitiveType; 051import org.hl7.fhir.r5.model.Reference; 052import org.hl7.fhir.r5.model.Resource; 053import org.hl7.fhir.r5.model.StructureDefinition; 054import org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionKind; 055import org.hl7.fhir.utilities.MarkedToMoveToAdjunctPackage; 056 057 058@MarkedToMoveToAdjunctPackage 059public class ObjectConverter { 060 061 private IWorkerContext context; 062 private ProfileUtilities profileUtilities; 063 064 public ObjectConverter(IWorkerContext context) { 065 this.context = context; 066 profileUtilities = new ProfileUtilities(context, null, null); 067 } 068 069 public Element convert(Resource ig) throws IOException, FHIRException { 070 if (ig == null) 071 return null; 072 ByteArrayOutputStream bs = new ByteArrayOutputStream(); 073 org.hl7.fhir.r5.formats.JsonParser jp = new org.hl7.fhir.r5.formats.JsonParser(); 074 jp.compose(bs, ig); 075 ByteArrayInputStream bi = new ByteArrayInputStream(bs.toByteArray()); 076 List<ValidatedFragment> list = new JsonParser(context).parse(bi); 077 if (list.size() != 1) { 078 throw new FHIRException("Unable to convert because the source contains multiple resources"); 079 } 080 return list.get(0).getElement(); 081 } 082 083 public Element convert(Property property, DataType type) throws FHIRException { 084 return convertElement(property, type); 085 } 086 087 private Element convertElement(Property property, Base base) throws FHIRException { 088 if (base == null) 089 return null; 090 String tn = base.fhirType(); 091 StructureDefinition sd = context.fetchResource(StructureDefinition.class, ProfileUtilities.sdNs(tn, null)); 092 if (sd == null) 093 throw new FHIRException("Unable to find definition for type "+tn); 094 Element res = new Element(property.getName(), property); 095 if (sd.getKind() == StructureDefinitionKind.PRIMITIVETYPE) 096 res.setValue(((PrimitiveType) base).asStringValue()); 097 098 SourcedChildDefinitions children = profileUtilities.getChildMap(sd, sd.getSnapshot().getElementFirstRep(), true); 099 for (ElementDefinition child : children.getList()) { 100 String n = tail(child.getPath()); 101 if (sd.getKind() != StructureDefinitionKind.PRIMITIVETYPE || !"value".equals(n)) { 102 Base[] values = base.getProperty(n.hashCode(), n, false); 103 if (values != null) 104 for (Base value : values) { 105 res.getChildren().add(convertElement(new Property(context, child, sd), value)); 106 } 107 } 108 } 109 return res; 110 } 111 112 private String tail(String path) { 113 if (path.contains(".")) 114 return path.substring(path.lastIndexOf('.')+1); 115 else 116 return path; 117 } 118 119 public DataType convertToType(Element element) throws FHIRException { 120 DataType b = new Factory().create(element.fhirType()); 121 if (b instanceof PrimitiveType) { 122 ((PrimitiveType) b).setValueAsString(element.primitiveValue()); 123 } else { 124 for (Element child : element.getChildren()) { 125 b.setProperty(child.getName(), convertToType(child)); 126 } 127 } 128 return b; 129 } 130 131 public Resource convert(Element element) throws FHIRException { 132 ByteArrayOutputStream bo = new ByteArrayOutputStream(); 133 try { 134 new JsonParser(context).compose(element, bo, OutputStyle.NORMAL, null); 135 return new org.hl7.fhir.r5.formats.JsonParser().parse(bo.toByteArray()); 136 } catch (IOException e) { 137 // won't happen 138 throw new FHIRException(e); 139 } 140 141 } 142 143 public static CodeableConcept readAsCodeableConcept(Element element) { 144 if (element == null) { 145 return null; 146 } 147 CodeableConcept cc = new CodeableConcept(); 148 List<Element> list = new ArrayList<Element>(); 149 element.getNamedChildren("coding", list); 150 for (Element item : list) 151 cc.addCoding(readAsCoding(item)); 152 cc.setText(element.getNamedChildValue("text")); 153 return cc; 154 } 155 156 public static Coding readAsCoding(Element item) { 157 Coding c = new Coding(); 158 c.setSystem(item.getNamedChildValue("system")); 159 c.setVersion(item.getNamedChildValue("version")); 160 c.setCode(item.getNamedChildValue("code")); 161 c.setDisplay(item.getNamedChildValue("display")); 162 return c; 163 } 164 165 public static Identifier readAsIdentifier(Element item) { 166 Identifier r = new Identifier(); 167 r.setSystem(item.getNamedChildValue("system")); 168 r.setValue(item.getNamedChildValue("value")); 169 return r; 170 } 171 172 public static Reference readAsReference(Element item) { 173 Reference r = new Reference(); 174 r.setDisplay(item.getNamedChildValue("display")); 175 r.setReference(item.getNamedChildValue("reference")); 176 r.setType(item.getNamedChildValue("type")); 177 if (!r.hasType()) { 178 Element ext = item.getExtension("http://hl7.org/fhir/4.0/StructureDefinition/extension-Reference.type"); 179 if (ext != null) { 180 r.setType(ext.getChildValue("valueUri")); 181 } 182 } 183 List<Element> identifier = item.getChildrenByName("identifier"); 184 if (identifier.isEmpty() == false) { 185 r.setIdentifier(readAsIdentifier(identifier.get(0))); 186 } 187 return r; 188 } 189 190}