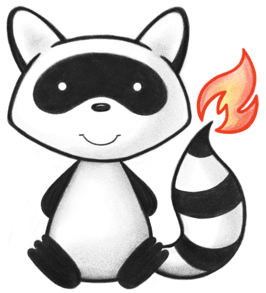
001package org.hl7.fhir.r5.fhirpath; 002 003import java.util.ArrayList; 004import java.util.List; 005 006import org.hl7.fhir.exceptions.FHIRException; 007import org.hl7.fhir.r5.model.Base; 008import org.hl7.fhir.r5.model.Element; 009import org.hl7.fhir.r5.model.ElementDefinition; 010import org.hl7.fhir.r5.model.Property; 011import org.hl7.fhir.r5.model.Resource; 012import org.hl7.fhir.r5.model.StringType; 013import org.hl7.fhir.r5.model.StructureDefinition; 014import org.hl7.fhir.r5.model.ElementDefinition.TypeRefComponent; 015import org.hl7.fhir.utilities.FhirPublication; 016import org.hl7.fhir.utilities.Utilities; 017 018public class FHIRPathUtilityClasses { 019 020 public static class FHIRConstant extends Base { 021 022 private static final long serialVersionUID = -8933773658248269439L; 023 private String value; 024 025 public FHIRConstant(String value) { 026 this.value = value; 027 } 028 029 @Override 030 public String fhirType() { 031 return "%constant"; 032 } 033 034 @Override 035 protected void listChildren(List<Property> result) { 036 } 037 038 @Override 039 public String getIdBase() { 040 return null; 041 } 042 043 @Override 044 public void setIdBase(String value) { 045 } 046 047 public String getValue() { 048 return value; 049 } 050 051 @Override 052 public String primitiveValue() { 053 return value; 054 } 055 056 @Override 057 public Base copy() { 058 throw new Error("Not Implemented"); 059 } 060 061 public FhirPublication getFHIRPublicationVersion() { 062 return FhirPublication.R5; 063 } 064 } 065 066 public static class ClassTypeInfo extends Base { 067 private static final long serialVersionUID = 4909223114071029317L; 068 private Base instance; 069 070 public ClassTypeInfo(Base instance) { 071 super(); 072 this.instance = instance; 073 } 074 075 @Override 076 public String fhirType() { 077 return "ClassInfo"; 078 } 079 080 @Override 081 protected void listChildren(List<Property> result) { 082 } 083 084 @Override 085 public String getIdBase() { 086 return null; 087 } 088 089 @Override 090 public void setIdBase(String value) { 091 } 092 093 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 094 if (name.equals("name")) { 095 return new Base[]{new StringType(getName())}; 096 } else if (name.equals("namespace")) { 097 return new Base[]{new StringType(getNamespace())}; 098 } else { 099 return super.getProperty(hash, name, checkValid); 100 } 101 } 102 103 private String getNamespace() { 104 if ((instance instanceof Resource)) { 105 return "FHIR"; 106 } else if (!(instance instanceof Element) || ((Element)instance).isDisallowExtensions()) { 107 return "System"; 108 } else { 109 return "FHIR"; 110 } 111 } 112 113 private String getName() { 114 if ((instance instanceof Resource)) { 115 return instance.fhirType(); 116 } else if (!(instance instanceof Element) || ((Element)instance).isDisallowExtensions()) { 117 return Utilities.capitalize(instance.fhirType()); 118 } else { 119 return instance.fhirType(); 120 } 121 } 122 123 @Override 124 public Base copy() { 125 throw new Error("Not Implemented"); 126 } 127 128 public FhirPublication getFHIRPublicationVersion() { 129 return FhirPublication.R5; 130 } 131 } 132 133 public static class TypedElementDefinition { 134 private ElementDefinition element; 135 private String type; 136 private StructureDefinition src; 137 public TypedElementDefinition(StructureDefinition src, ElementDefinition element, String type) { 138 super(); 139 this.element = element; 140 this.type = type; 141 this.src = src; 142 } 143 public TypedElementDefinition(ElementDefinition element) { 144 super(); 145 this.element = element; 146 } 147 public ElementDefinition getElement() { 148 return element; 149 } 150 public String getType() { 151 return type; 152 } 153 public List<TypeRefComponent> getTypes() { 154 List<TypeRefComponent> res = new ArrayList<ElementDefinition.TypeRefComponent>(); 155 for (TypeRefComponent tr : element.getType()) { 156 if (type == null || type.equals(tr.getCode())) { 157 res.add(tr); 158 } 159 } 160 return res; 161 } 162 public boolean hasType(String tn) { 163 if (type != null) { 164 return tn.equals(type); 165 } else { 166 for (TypeRefComponent t : element.getType()) { 167 if (tn.equals(t.getCode())) { 168 return true; 169 } 170 } 171 return false; 172 } 173 } 174 public StructureDefinition getSrc() { 175 return src; 176 } 177 } 178 179 180 public static class FunctionDetails { 181 private String description; 182 private int minParameters; 183 private int maxParameters; 184 public FunctionDetails(String description, int minParameters, int maxParameters) { 185 super(); 186 this.description = description; 187 this.minParameters = minParameters; 188 this.maxParameters = maxParameters; 189 } 190 public String getDescription() { 191 return description; 192 } 193 public int getMinParameters() { 194 return minParameters; 195 } 196 public int getMaxParameters() { 197 return maxParameters; 198 } 199 200 } 201}