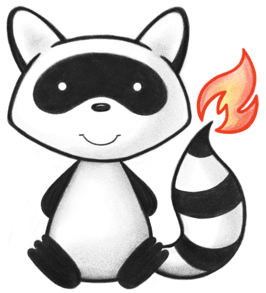
001package org.hl7.fhir.r5.formats; 002 003 004 005 006 007import java.io.FileInputStream; 008import java.io.IOException; 009 010/* 011 Copyright (c) 2011+, HL7, Inc. 012 All rights reserved. 013 014 Redistribution and use in source and binary forms, with or without modification, 015 are permitted provided that the following conditions are met: 016 017 * Redistributions of source code must retain the above copyright notice, this 018 list of conditions and the following disclaimer. 019 * Redistributions in binary form must reproduce the above copyright notice, 020 this list of conditions and the following disclaimer in the documentation 021 and/or other materials provided with the distribution. 022 * Neither the name of HL7 nor the names of its contributors may be used to 023 endorse or promote products derived from this software without specific 024 prior written permission. 025 026 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 027 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 028 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 029 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 030 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 031 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 032 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 033 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 034 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 035 POSSIBILITY OF SUCH DAMAGE. 036 037*/ 038 039 040import java.io.InputStream; 041import java.io.OutputStream; 042import java.io.UnsupportedEncodingException; 043 044import org.hl7.fhir.exceptions.FHIRFormatError; 045import org.hl7.fhir.r5.model.DataType; 046import org.hl7.fhir.r5.model.Resource; 047import org.hl7.fhir.r5.model.SearchParameter; 048import org.xmlpull.v1.XmlPullParserException; 049 050 051/** 052 * General interface - either an XML or JSON parser: read or write instances 053 * 054 * Defined to allow a factory to create a parser of the right type 055 */ 056public interface IParser { 057 058 /** 059 * check what kind of parser this is 060 * 061 * @return what kind of parser this is 062 */ 063 public ParserType getType(); 064 065 // -- Parser Configuration ---------------------------------- 066 /** 067 * Whether to parse or ignore comments - either reading or writing 068 */ 069 public boolean getHandleComments(); 070 public IParser setHandleComments(boolean value); 071 072 /** 073 * @param allowUnknownContent Whether to throw an exception if unknown content is found (or just skip it) when parsing 074 */ 075 public boolean isAllowUnknownContent(); 076 public IParser setAllowUnknownContent(boolean value); 077 078 079 public enum OutputStyle { 080 /** 081 * Produce normal output - no whitespace, except in HTML where whitespace is untouched 082 */ 083 NORMAL, 084 085 /** 086 * Produce pretty output - human readable whitespace, HTML whitespace untouched 087 */ 088 PRETTY, 089 090 /** 091 * Produce canonical output - no comments, no whitspace, HTML whitespace normlised, JSON attributes sorted alphabetically (slightly slower) 092 */ 093 CANONICAL, 094 } 095 096 /** 097 * Writing: 098 */ 099 public OutputStyle getOutputStyle(); 100 public IParser setOutputStyle(OutputStyle value); 101 102 /** 103 * This method is used by the publication tooling to stop the xhrtml narrative being generated. 104 * It is not valid to use in production use. The tooling uses it to generate json/xml representations in html that are not cluttered by escaped html representations of the html representation 105 */ 106 public IParser setSuppressXhtml(String message); 107 108 // -- Reading methods ---------------------------------------- 109 110 /** 111 * parse content that is known to be a resource 112 * @throws XmlPullParserException 113 * @throws FHIRFormatError 114 * @throws IOException 115 */ 116 public Resource parse(InputStream input) throws IOException, FHIRFormatError; 117 118 public Resource parseAndClose(InputStream input) throws IOException, FHIRFormatError; 119 120 /** 121 * parse content that is known to be a resource 122 * @throws UnsupportedEncodingException 123 * @throws IOException 124 * @throws FHIRFormatError 125 */ 126 public Resource parse(String input) throws UnsupportedEncodingException, FHIRFormatError, IOException; 127 128 /** 129 * parse content that is known to be a resource 130 * @throws IOException 131 * @throws FHIRFormatError 132 */ 133 public Resource parse(byte[] bytes) throws FHIRFormatError, IOException; 134 135 /** 136 * This is used to parse a type - a fragment of a resource. 137 * There's no reason to use this in production - it's used 138 * in the build tools 139 * 140 * Not supported by all implementations 141 * 142 * @param input 143 * @param knownType. if this is blank, the parser may try to infer the type (xml only) 144 * @return 145 * @throws XmlPullParserException 146 * @throws FHIRFormatError 147 * @throws IOException 148 */ 149 public DataType parseType(InputStream input, String knownType) throws IOException, FHIRFormatError; 150 public DataType parseAnyType(InputStream input, String knownType) throws IOException, FHIRFormatError; 151 152 /** 153 * This is used to parse a type - a fragment of a resource. 154 * There's no reason to use this in production - it's used 155 * in the build tools 156 * 157 * Not supported by all implementations 158 * 159 * @param input 160 * @param knownType. if this is blank, the parser may try to infer the type (xml only) 161 * @return 162 * @throws UnsupportedEncodingException 163 * @throws IOException 164 * @throws FHIRFormatError 165 */ 166 public DataType parseType(String input, String knownType) throws UnsupportedEncodingException, FHIRFormatError, IOException; 167 /** 168 * This is used to parse a type - a fragment of a resource. 169 * There's no reason to use this in production - it's used 170 * in the build tools 171 * 172 * Not supported by all implementations 173 * 174 * @param input 175 * @param knownType. if this is blank, the parser may try to infer the type (xml only) 176 * @return 177 * @throws IOException 178 * @throws FHIRFormatError 179 */ 180 public DataType parseType(byte[] bytes, String knownType) throws FHIRFormatError, IOException; 181 182 // -- Writing methods ---------------------------------------- 183 184 /** 185 * Compose a resource to a stream, possibly using pretty presentation for a human reader (used in the spec, for example, but not normally in production) 186 * @throws IOException 187 */ 188 public void compose(OutputStream stream, Resource resource) throws IOException; 189 190 /** 191 * Compose a resource to a stream, possibly using pretty presentation for a human reader (used in the spec, for example, but not normally in production) 192 * @throws IOException 193 */ 194 public String composeString(Resource resource) throws IOException; 195 196 /** 197 * Compose a resource to a stream, possibly using pretty presentation for a human reader (used in the spec, for example, but not normally in production) 198 * @throws IOException 199 */ 200 public byte[] composeBytes(Resource resource) throws IOException; 201 202 203 /** 204 * Compose a type to a stream, possibly using pretty presentation for a human reader (used in the spec, for example, but not normally in production) 205 * 206 * Not supported by all implementations. rootName is ignored in the JSON format 207 * @throws XmlPullParserException 208 * @throws FHIRFormatError 209 * @throws IOException 210 */ 211 public void compose(OutputStream stream, DataType type, String rootName) throws IOException; 212 213 /** 214 * Compose a type to a stream, possibly using pretty presentation for a human reader (used in the spec, for example, but not normally in production) 215 * 216 * Not supported by all implementations. rootName is ignored in the JSON format 217 * @throws IOException 218 */ 219 public String composeString(DataType type, String rootName) throws IOException; 220 221 /** 222 * Compose a type to a stream, possibly using pretty presentation for a human reader (used in the spec, for example, but not normally in production) 223 * 224 * Not supported by all implementations. rootName is ignored in the JSON format 225 * @throws IOException 226 */ 227 public byte[] composeBytes(DataType type, String rootName) throws IOException; 228 229 230}