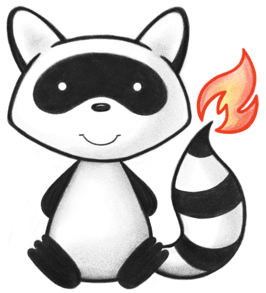
001/* 002 * #%L 003 * HAPI FHIR Structures - DSTU2 (FHIR v1.0.0) 004 * %% 005 * Copyright (C) 2014 - 2015 University Health Network 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package org.hl7.fhir.r5.hapi.rest.server; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.context.api.BundleInclusionRule; 024import ca.uhn.fhir.model.api.Include; 025import ca.uhn.fhir.model.api.ResourceMetadataKeyEnum; 026import ca.uhn.fhir.model.valueset.BundleEntrySearchModeEnum; 027import ca.uhn.fhir.model.valueset.BundleEntryTransactionMethodEnum; 028import ca.uhn.fhir.model.valueset.BundleTypeEnum; 029import ca.uhn.fhir.rest.api.BundleLinks; 030import ca.uhn.fhir.rest.api.Constants; 031import ca.uhn.fhir.rest.api.IVersionSpecificBundleFactory; 032import ca.uhn.fhir.rest.server.RestfulServerUtils; 033import ca.uhn.fhir.util.ResourceReferenceInfo; 034import jakarta.annotation.Nonnull; 035import org.hl7.fhir.instance.model.api.IAnyResource; 036import org.hl7.fhir.instance.model.api.IBaseResource; 037import org.hl7.fhir.instance.model.api.IIdType; 038import org.hl7.fhir.instance.model.api.IPrimitiveType; 039import org.hl7.fhir.r5.model.Bundle; 040import org.hl7.fhir.r5.model.Bundle.BundleEntryComponent; 041import org.hl7.fhir.r5.model.Bundle.BundleLinkComponent; 042import org.hl7.fhir.r5.model.Bundle.SearchEntryMode; 043import org.hl7.fhir.r5.model.DomainResource; 044import org.hl7.fhir.r5.model.IdType; 045import org.hl7.fhir.r5.model.Resource; 046 047import java.math.BigDecimal; 048import java.util.ArrayList; 049import java.util.Date; 050import java.util.HashSet; 051import java.util.List; 052import java.util.Set; 053import java.util.UUID; 054 055import static org.apache.commons.lang3.StringUtils.isNotBlank; 056 057@SuppressWarnings("Duplicates") 058public class R5BundleFactory implements IVersionSpecificBundleFactory { 059 private String myBase; 060 private Bundle myBundle; 061 private FhirContext myContext; 062 063 public R5BundleFactory(FhirContext theContext) { 064 myContext = theContext; 065 } 066 067 @Override 068 public void addResourcesToBundle( 069 List<IBaseResource> theResult, 070 BundleTypeEnum theBundleType, 071 String theServerBase, 072 BundleInclusionRule theBundleInclusionRule, 073 Set<Include> theIncludes) { 074 ensureBundle(); 075 076 List<IAnyResource> includedResources = new ArrayList<IAnyResource>(); 077 Set<IIdType> addedResourceIds = new HashSet<IIdType>(); 078 079 for (IBaseResource next : theResult) { 080 if (next.getIdElement().isEmpty() == false) { 081 addedResourceIds.add(next.getIdElement()); 082 } 083 } 084 085 for (IBaseResource next : theResult) { 086 087 Set<String> containedIds = new HashSet<String>(); 088 089 if (next instanceof DomainResource) { 090 for (Resource nextContained : ((DomainResource) next).getContained()) { 091 if (isNotBlank(nextContained.getId())) { 092 containedIds.add(nextContained.getId()); 093 } 094 } 095 } 096 097 List<ResourceReferenceInfo> references = myContext.newTerser().getAllResourceReferences(next); 098 do { 099 List<IAnyResource> addedResourcesThisPass = new ArrayList<IAnyResource>(); 100 101 for (ResourceReferenceInfo nextRefInfo : references) { 102 if (theBundleInclusionRule != null 103 && !theBundleInclusionRule.shouldIncludeReferencedResource(nextRefInfo, theIncludes)) { 104 continue; 105 } 106 107 IAnyResource nextRes = 108 (IAnyResource) nextRefInfo.getResourceReference().getResource(); 109 if (nextRes != null) { 110 if (nextRes.getIdElement().hasIdPart()) { 111 if (containedIds.contains(nextRes.getIdElement().getValue())) { 112 // Don't add contained IDs as top level resources 113 continue; 114 } 115 116 IIdType id = nextRes.getIdElement(); 117 if (id.hasResourceType() == false) { 118 String resName = myContext.getResourceType(nextRes); 119 id = id.withResourceType(resName); 120 } 121 122 if (!addedResourceIds.contains(id)) { 123 addedResourceIds.add(id); 124 addedResourcesThisPass.add(nextRes); 125 } 126 } 127 } 128 } 129 130 includedResources.addAll(addedResourcesThisPass); 131 132 // Linked resources may themselves have linked resources 133 references = new ArrayList<>(); 134 for (IAnyResource iResource : addedResourcesThisPass) { 135 List<ResourceReferenceInfo> newReferences = 136 myContext.newTerser().getAllResourceReferences(iResource); 137 references.addAll(newReferences); 138 } 139 } while (references.isEmpty() == false); 140 141 BundleEntryComponent entry = myBundle.addEntry().setResource((Resource) next); 142 Resource nextAsResource = (Resource) next; 143 IIdType id = populateBundleEntryFullUrl(next, entry); 144 145 // Populate Request 146 BundleEntryTransactionMethodEnum httpVerb = 147 ResourceMetadataKeyEnum.ENTRY_TRANSACTION_METHOD.get(nextAsResource); 148 if (httpVerb != null) { 149 entry.getRequest().getMethodElement().setValueAsString(httpVerb.name()); 150 if (id != null) { 151 entry.getRequest().setUrl(id.toUnqualified().getValue()); 152 } 153 } 154 if (BundleEntryTransactionMethodEnum.DELETE.equals(httpVerb)) { 155 entry.setResource(null); 156 } 157 158 // Populate Bundle.entry.response 159 if (theBundleType != null) { 160 switch (theBundleType) { 161 case BATCH_RESPONSE: 162 case TRANSACTION_RESPONSE: 163 case HISTORY: 164 if ("1".equals(id.getVersionIdPart())) { 165 entry.getResponse().setStatus("201 Created"); 166 } else if (isNotBlank(id.getVersionIdPart())) { 167 entry.getResponse().setStatus("200 OK"); 168 } 169 if (isNotBlank(id.getVersionIdPart())) { 170 entry.getResponse().setEtag(RestfulServerUtils.createEtag(id.getVersionIdPart())); 171 } 172 break; 173 } 174 } 175 176 // Populate Bundle.entry.search 177 BundleEntrySearchModeEnum searchMode = ResourceMetadataKeyEnum.ENTRY_SEARCH_MODE.get(nextAsResource); 178 if (searchMode != null) { 179 entry.getSearch().getModeElement().setValueAsString(searchMode.getCode()); 180 } 181 BigDecimal searchScore = ResourceMetadataKeyEnum.ENTRY_SEARCH_SCORE.get(nextAsResource); 182 if (searchScore != null) { 183 entry.getSearch().getScoreElement().setValue(searchScore); 184 } 185 } 186 187 /* 188 * Actually add the resources to the bundle 189 */ 190 for (IAnyResource next : includedResources) { 191 BundleEntryComponent entry = myBundle.addEntry(); 192 entry.setResource((Resource) next).getSearch().setMode(SearchEntryMode.INCLUDE); 193 populateBundleEntryFullUrl(next, entry); 194 } 195 } 196 197 @Override 198 public void addRootPropertiesToBundle( 199 String theId, 200 @Nonnull BundleLinks theBundleLinks, 201 Integer theTotalResults, 202 IPrimitiveType<Date> theLastUpdated) { 203 ensureBundle(); 204 205 myBase = theBundleLinks.serverBase; 206 207 if (myBundle.getIdElement().isEmpty()) { 208 myBundle.setId(theId); 209 } 210 211 if (myBundle.getMeta().getLastUpdated() == null && theLastUpdated != null) { 212 myBundle.getMeta().getLastUpdatedElement().setValueAsString(theLastUpdated.getValueAsString()); 213 } 214 215 if (!hasLink(Constants.LINK_SELF, myBundle) && isNotBlank(theBundleLinks.getSelf())) { 216 myBundle.addLink().setRelation(Bundle.LinkRelationTypes.SELF).setUrl(theBundleLinks.getSelf()); 217 } 218 if (!hasLink(Constants.LINK_NEXT, myBundle) && isNotBlank(theBundleLinks.getNext())) { 219 myBundle.addLink().setRelation(Bundle.LinkRelationTypes.NEXT).setUrl(theBundleLinks.getNext()); 220 } 221 if (!hasLink(Constants.LINK_PREVIOUS, myBundle) && isNotBlank(theBundleLinks.getPrev())) { 222 myBundle.addLink().setRelation(Bundle.LinkRelationTypes.PREV).setUrl(theBundleLinks.getPrev()); 223 } 224 225 addTotalResultsToBundle(theTotalResults, theBundleLinks.bundleType); 226 } 227 228 @Override 229 public void addTotalResultsToBundle(Integer theTotalResults, BundleTypeEnum theBundleType) { 230 ensureBundle(); 231 232 if (myBundle.getIdElement().isEmpty()) { 233 myBundle.setId(UUID.randomUUID().toString()); 234 } 235 236 if (myBundle.getTypeElement().isEmpty() && theBundleType != null) { 237 myBundle.getTypeElement().setValueAsString(theBundleType.getCode()); 238 } 239 240 if (myBundle.getTotalElement().isEmpty() && theTotalResults != null) { 241 myBundle.getTotalElement().setValue(theTotalResults); 242 } 243 } 244 245 private void ensureBundle() { 246 if (myBundle == null) { 247 myBundle = new Bundle(); 248 } 249 } 250 251 @Override 252 public IBaseResource getResourceBundle() { 253 return myBundle; 254 } 255 256 private boolean hasLink(String theLinkType, Bundle theBundle) { 257 for (BundleLinkComponent next : theBundle.getLink()) { 258 if (theLinkType.equals(next.getRelation())) { 259 return true; 260 } 261 } 262 return false; 263 } 264 265 @Override 266 public void initializeWithBundleResource(IBaseResource theBundle) { 267 myBundle = (Bundle) theBundle; 268 } 269 270 private IIdType populateBundleEntryFullUrl(IBaseResource next, BundleEntryComponent entry) { 271 IIdType idElement = null; 272 if (next.getIdElement().hasBaseUrl()) { 273 idElement = next.getIdElement(); 274 entry.setFullUrl(idElement.toVersionless().getValue()); 275 } else { 276 if (isNotBlank(myBase) && next.getIdElement().hasIdPart()) { 277 idElement = next.getIdElement(); 278 idElement = idElement.withServerBase(myBase, myContext.getResourceType(next)); 279 entry.setFullUrl(idElement.toVersionless().getValue()); 280 } 281 } 282 return idElement; 283 } 284 285 @Override 286 public List<IBaseResource> toListOfResources() { 287 ArrayList<IBaseResource> retVal = new ArrayList<IBaseResource>(); 288 for (BundleEntryComponent next : myBundle.getEntry()) { 289 if (next.getResource() != null) { 290 retVal.add(next.getResource()); 291 } else if (next.getResponse().getLocationElement().isEmpty() == false) { 292 IdType id = new IdType(next.getResponse().getLocation()); 293 String resourceType = id.getResourceType(); 294 if (isNotBlank(resourceType)) { 295 IAnyResource res = (IAnyResource) 296 myContext.getResourceDefinition(resourceType).newInstance(); 297 res.setId(id); 298 retVal.add(res); 299 } 300 } 301 } 302 return retVal; 303 } 304}